
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_update.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef SOURCE_PAL_IMPL_SERVICES_API_PAL_UPDATE_H_ 00018 #define SOURCE_PAL_IMPL_SERVICES_API_PAL_UPDATE_H_ 00019 00020 #include "pal_macros.h" 00021 #include "pal_types.h" 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 /*! \file pal_update.h 00028 * \brief PAL update. 00029 * This file contains the firmware update APIs and is a part of the PAL service API. 00030 * It provides the read/write and activation functionalities for the firmware. 00031 */ 00032 00033 00034 typedef uint32_t palImageId_t; 00035 00036 typedef struct _palImageHeaderDeails_t 00037 { 00038 size_t imageSize; 00039 palBuffer_t hash; 00040 uint64_t version; 00041 } palImageHeaderDeails_t; 00042 00043 typedef enum _palImagePlatformData_t 00044 { 00045 PAL_IMAGE_DATA_FIRST = 0, 00046 PAL_IMAGE_DATA_HASH = PAL_IMAGE_DATA_FIRST, 00047 PAL_IMAGE_DATA_LAST 00048 } palImagePlatformData_t; 00049 00050 typedef enum _palImageEvents_t 00051 { 00052 PAL_IMAGE_EVENT_FIRST = -1, 00053 PAL_IMAGE_EVENT_ERROR = PAL_IMAGE_EVENT_FIRST, 00054 PAL_IMAGE_EVENT_INIT , 00055 PAL_IMAGE_EVENT_PREPARE, 00056 PAL_IMAGE_EVENT_WRITE, 00057 PAL_IMAGE_EVENT_FINALIZE, 00058 PAL_IMAGE_EVENT_READTOBUFFER, 00059 PAL_IMAGE_EVENT_ACTIVATE, 00060 PAL_IMAGE_EVENT_ACTIVATE_ERROR, 00061 PAL_IMAGE_EVENT_GETACTIVEHASH, 00062 PAL_IMAGE_EVENT_GETACTIVEVERSION, 00063 PAL_IMAGE_EVENT_WRITEDATATOMEMORY, 00064 PAL_IMAGE_EVENT_LAST 00065 } palImageEvents_t; 00066 00067 typedef void (*palImageSignalEvent_t)( palImageEvents_t event); 00068 00069 #define SIZEOF_SHA256 256/8 00070 #define FIRMWARE_HEADER_MAGIC 0x5a51b3d4UL 00071 #define FIRMWARE_HEADER_VERSION 1 00072 #define IMAGE_COUNT_MAX 1 00073 #define ACTIVE_IMAGE_INDEX 0xFFFFFFFF 00074 00075 typedef struct FirmwareHeader { 00076 uint32_t magic; /** Metadata-header specific magic code. */ 00077 uint32_t version; /** Revision number for this generic metadata header. */ 00078 uint32_t checksum; /** A checksum of this header. This field should be considered to be zeroed out for 00079 * the sake of computing the checksum. */ 00080 uint32_t totalSize; /** Total space (in bytes) occupied by the firmware BLOB, including headers and any padding. */ 00081 uint64_t firmwareVersion; /** Version number for the accompanying firmware. Larger numbers imply more preferred (recent) 00082 * versions. This defines the selection order when multiple versions are available. */ 00083 uint8_t firmwareSHA256[SIZEOF_SHA256]; /** A SHA-2 using a block-size of 256-bits of the firmware, including any firmware-padding. */ 00084 } FirmwareHeader_t; 00085 00086 00087 typedef FirmwareHeader_t palFirmwareHeader_t; 00088 /*! Sets the callback function that is called before the end of each API (except `imageGetDirectMemAccess`). 00089 * 00090 * WARNING: Do not change this function! 00091 * This function loads a callback function received from the upper layer (service). 00092 * The callback should be called at the end of each function (except `pal_plat_imageGetDirectMemAccess`). 00093 * The callback receives the event type that just occurred, defined by the ENUM `palImageEvents_t`. 00094 * 00095 * If you do not call the callback at the end, the service behaviour will be undefined. 00096 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00097 * 00098 * @param[in] CBfunction A pointer to the callback function. 00099 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00100 */ 00101 palStatus_t pal_imageInitAPI(palImageSignalEvent_t CBfunction); 00102 00103 00104 /*! Clears all the resources used by the `pal_update` APIs. 00105 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00106 */ 00107 palStatus_t pal_imageDeInit(void); 00108 00109 /*! Prepares to write an image with an ID (`imageId`) and size (`imageSize`) in a suitable memory region. The space available is verified and reserved. 00110 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00111 * @param[in] imageId The image ID. 00112 * @param[in] headerDetails The size of the image. 00113 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00114 */ 00115 palStatus_t pal_imagePrepare(palImageId_t imageId, palImageHeaderDeails_t* headerDetails); 00116 00117 /*! Writes the data to the chunk buffer with the chunk `bufferLength` in the location of `imageId` adding the relative offset. 00118 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00119 * @param[in] imageId The image ID. 00120 * @param[in] offset The offset to write the data into. 00121 * @param[in] chunk A pointer to struct containing the data and the data length to write. 00122 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00123 */ 00124 palStatus_t pal_imageWrite (palImageId_t imageId, size_t offset, palConstBuffer_t *chunk); 00125 00126 /*! Flushes the image data and sets the version of `imageId` to `imageVersion`. 00127 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00128 * @param[in] imageId The image ID. 00129 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00130 */ 00131 palStatus_t pal_imageFinalize(palImageId_t imageId); 00132 00133 /*! Verifies whether the image (`imageId`) is readable and sets `imagePtr` to point to the beginning of the image in the memory and `imageSizeInBytes` to the image size. 00134 * In case of failure, sets `imagePtr` to NULL and returns the relevant `palStatus_t` error. 00135 * @param[in] imageId The image ID. 00136 * @param[out] imagePtr A pointer to the beginning of the image. 00137 * @param[out] imageSizeInBytes The size of the image. 00138 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00139 */ 00140 palStatus_t pal_imageGetDirectMemoryAccess(palImageId_t imageId, void** imagePtr, size_t *imageSizeInBytes); 00141 00142 /*! Reads the maximum of chunk (`maxBufferLength`) bytes from the image (`imageId`) with relative offset and stores it in the chunk buffer. 00143 * Also sets the chunk `bufferLength` value to the actual number of bytes read. 00144 * \note Use this API in the case image is not directly accessible via the `imageGetDirectMemAccess` function. 00145 * 00146 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00147 * 00148 * @param[in] imageId The image ID. 00149 * @param[in] offset The offset to start reading from. 00150 * @param[out] chunk A struct containing the data and actual bytes read. 00151 */ 00152 palStatus_t pal_imageReadToBuffer(palImageId_t imageId, size_t offset, palBuffer_t* chunk); 00153 00154 /*! Sets an image (`imageId`) as the active image (after the device reset). 00155 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00156 * 00157 * @param[in] imageId The image ID. 00158 */ 00159 palStatus_t pal_imageActivate(palImageId_t imageId); 00160 00161 /*! Retrieves the hash value of the active image to the hash buffer with the max size hash (`maxBufferLength`) and sets the hash size to hash `bufferLength`. 00162 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00163 * @param[out] hash A struct containing the hash and actual size of hash read. 00164 */ 00165 palStatus_t pal_imageGetActiveHash(palBuffer_t* hash); 00166 00167 00168 /*! Retrieves the data value of the image header. 00169 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00170 * @param[in] imageId The image ID. 00171 * @param[out] headerData A struct containing the headerData and actual size of header. 00172 */ 00173 palStatus_t pal_imageGetFirmwareHeaderData(palImageId_t imageId, palBuffer_t *headerData); 00174 00175 00176 /*! Retrieves the version of the active image to the version buffer with the size set to version `bufferLength`. 00177 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00178 * @param[out] version A struct containing the version and actual size of version read. 00179 */ 00180 palStatus_t pal_imageGetActiveVersion(palBuffer_t* version); 00181 00182 /*! Writes the data of `dataId` stored in `dataBuffer` to a memory accessible to the bootloader. Currently, only hash is available. 00183 * The function must call `g_palUpdateServiceCBfunc` before completing an event. 00184 * @param[in] dataId One of the members of the `palImagePlatformData_t` enum. 00185 * @param[in] dataBuffer A struct containing the data and actual bytes to be written. 00186 */ 00187 palStatus_t pal_imageWriteDataToMemory(palImagePlatformData_t dataId, const palConstBuffer_t* const dataBuffer); 00188 00189 00190 /*! \brief This function gets the firmware working directory. 00191 * 00192 * \return full path of the firmware working folder 00193 */ 00194 char* pal_imageGetFolder(void); 00195 00196 #ifdef __cplusplus 00197 } 00198 #endif 00199 #endif /* SOURCE_PAL_IMPL_SERVICES_API_PAL_UPDATE_H_ */
Generated on Tue Jul 12 2022 16:22:10 by
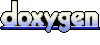