
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_types.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef _PAL_TYPES_H 00019 #define _PAL_TYPES_H 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 #include <stdint.h> 00025 #include <stdbool.h> 00026 #include <stddef.h> 00027 #if defined __cplusplus && !defined __STDC_FORMAT_MACROS 00028 #define UNDEF__STDC_FORMAT_MACROS 00029 #define __STDC_FORMAT_MACROS 00030 #endif 00031 #include <inttypes.h> 00032 #ifdef UNDEF__STDC_FORMAT_MACROS 00033 #undef UNDEF__STDC_FORMAT_MACROS 00034 #undef __STDC_FORMAT_MACROS 00035 #endif 00036 #include <stdlib.h> 00037 00038 /*! \file pal_types.h 00039 * \brief PAL types. 00040 * This file contains PAL generic types. 00041 */ 00042 00043 00044 #define NULLPTR 0 00045 00046 #define TRUE 1 00047 #define FALSE 0 00048 00049 #define PAL_INVALID_THREAD 0xFFFFFFFF 00050 00051 typedef int32_t palStatus_t; 00052 00053 typedef struct _palBuffer_t 00054 { 00055 uint32_t maxBufferLength; 00056 uint32_t bufferLength; 00057 uint8_t *buffer; 00058 } palBuffer_t; 00059 00060 typedef struct _palConstBuffer_t 00061 { 00062 const uint32_t maxBufferLength; 00063 const uint32_t bufferLength; 00064 const uint8_t *buffer; 00065 } palConstBuffer_t; 00066 00067 typedef struct sotpAreaData 00068 { 00069 uint32_t address; /*\brief the address of the starting sector for the given area*/ 00070 size_t size; /*\brief the size of the area*/ 00071 }palSotpAreaData_t; 00072 00073 00074 #ifdef __cplusplus 00075 } 00076 #endif 00077 #endif //_PAL_TYPES_H
Generated on Tue Jul 12 2022 16:22:10 by
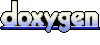