
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_rtos_test_utils.c
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "pal_rtos_test_utils.h" 00018 #include "pal_rtos.h" 00019 #include "pal_test_main.h" 00020 #include "unity_fixture.h" 00021 00022 #include "pal.h" 00023 00024 00025 extern threadsArgument_t g_threadsArg; 00026 extern palThreadLocalStore_t g_threadStorage; 00027 timerArgument_t timerArgs; 00028 00029 void palThreadFunc1(void const *argument) 00030 { 00031 volatile palThreadID_t threadID; 00032 palThreadLocalStore_t * threadStorage = NULL; 00033 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00034 #ifdef MUTEX_UNITY_TEST 00035 palStatus_t status = PAL_SUCCESS; 00036 PAL_PRINTF("palThreadFunc1::before mutex\n"); 00037 status = pal_osMutexWait(mutex1, 100); 00038 PAL_PRINTF("palThreadFunc1::after mutex: 0x%08x\n", status); 00039 PAL_PRINTF("palThreadFunc1::after mutex (expected): 0x%08x\n", PAL_ERR_RTOS_TIMEOUT ); 00040 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_TIMEOUT , status); 00041 return; // for Mutex scenario, this should end here 00042 #endif //MUTEX_UNITY_TEST 00043 00044 tmp->arg1 = 10; 00045 threadID = pal_osThreadGetId(); 00046 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00047 PAL_PRINTF("palThreadFunc1::Thread ID is %d\n", threadID); 00048 00049 threadStorage = pal_osThreadGetLocalStore(); 00050 if (threadStorage == &g_threadStorage) 00051 { 00052 PAL_PRINTF("Thread storage updated as expected\n"); 00053 } 00054 TEST_ASSERT_EQUAL_HEX((uintptr_t)threadStorage, (uintptr_t)(&g_threadStorage)); 00055 00056 #ifdef MUTEX_UNITY_TEST 00057 status = pal_osMutexRelease(mutex1); 00058 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00059 #endif //MUTEX_UNITY_TEST 00060 PAL_PRINTF("palThreadFunc1::STAAAAM\n"); 00061 } 00062 00063 void palThreadFunc2(void const *argument) 00064 { 00065 volatile palThreadID_t threadID; 00066 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00067 #ifdef MUTEX_UNITY_TEST 00068 palStatus_t status = PAL_SUCCESS; 00069 PAL_PRINTF("palThreadFunc2::before mutex\n"); 00070 status = pal_osMutexWait(mutex2, 300); 00071 PAL_PRINTF("palThreadFunc2::after mutex: 0x%08x\n", status); 00072 PAL_PRINTF("palThreadFunc2::after mutex (expected): 0x%08x\n", PAL_SUCCESS); 00073 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00074 #endif //MUTEX_UNITY_TEST 00075 00076 tmp->arg2 = 20; 00077 threadID = pal_osThreadGetId(); 00078 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00079 PAL_PRINTF("palThreadFunc2::Thread ID is %d\n", threadID); 00080 #ifdef MUTEX_UNITY_TEST 00081 status = pal_osMutexRelease(mutex2); 00082 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00083 #endif //MUTEX_UNITY_TEST 00084 PAL_PRINTF("palThreadFunc2::STAAAAM\n"); 00085 } 00086 00087 void palThreadFunc3(void const *argument) 00088 { 00089 volatile palThreadID_t threadID; 00090 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00091 00092 #ifdef SEMAPHORE_UNITY_TEST 00093 palStatus_t status = PAL_SUCCESS; 00094 uint32_t semaphoresAvailable = 10; 00095 status = pal_osSemaphoreWait(semaphore1, 200, &semaphoresAvailable); 00096 00097 if (PAL_SUCCESS == status) 00098 { 00099 PAL_PRINTF("palThreadFunc3::semaphoresAvailable: %d\n", semaphoresAvailable); 00100 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00101 } 00102 else if(PAL_ERR_RTOS_TIMEOUT == status) 00103 { 00104 PAL_PRINTF("palThreadFunc3::semaphoresAvailable: %d\n", semaphoresAvailable); 00105 PAL_PRINTF("palThreadFunc3::status: 0x%08x\n", status); 00106 PAL_PRINTF("palThreadFunc3::failed to get Semaphore as expected\n", status); 00107 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_TIMEOUT , status); 00108 return; 00109 } 00110 pal_osDelay(6000); 00111 #endif //SEMAPHORE_UNITY_TEST 00112 tmp->arg3 = 30; 00113 threadID = pal_osThreadGetId(); 00114 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00115 PAL_PRINTF("palThreadFunc3::Thread ID is %d\n", threadID); 00116 00117 #ifdef SEMAPHORE_UNITY_TEST 00118 status = pal_osSemaphoreRelease(semaphore1); 00119 PAL_PRINTF("palThreadFunc3::pal_osSemaphoreRelease res: 0x%08x\n", status); 00120 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00121 #endif //SEMAPHORE_UNITY_TEST 00122 PAL_PRINTF("palThreadFunc3::STAAAAM\n"); 00123 } 00124 00125 void palThreadFunc4(void const *argument) 00126 { 00127 volatile palThreadID_t threadID; 00128 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00129 #ifdef MUTEX_UNITY_TEST 00130 palStatus_t status = PAL_SUCCESS; 00131 PAL_PRINTF("palThreadFunc4::before mutex\n"); 00132 status = pal_osMutexWait(mutex1, 200); 00133 PAL_PRINTF("palThreadFunc4::after mutex: 0x%08x\n", status); 00134 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00135 pal_osDelay(3500); //wait 3.5 seconds to make sure that the next thread arrive to this point 00136 #endif //MUTEX_UNITY_TEST 00137 00138 tmp->arg4 = 40; 00139 threadID = pal_osThreadGetId(); 00140 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00141 PAL_PRINTF("Thread ID is %d\n", threadID); 00142 00143 #ifdef MUTEX_UNITY_TEST 00144 status = pal_osMutexRelease(mutex1); 00145 PAL_PRINTF("palThreadFunc4::after release mutex: 0x%08x\n", status); 00146 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00147 #endif //MUTEX_UNITY_TEST 00148 PAL_PRINTF("palThreadFunc4::STAAAAM\n"); 00149 } 00150 00151 void palThreadFunc5(void const *argument) 00152 { 00153 volatile palThreadID_t threadID; 00154 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00155 #ifdef MUTEX_UNITY_TEST 00156 palStatus_t status = PAL_SUCCESS; 00157 PAL_PRINTF("palThreadFunc5::before mutex\n"); 00158 status = pal_osMutexWait(mutex1, 4500); 00159 PAL_PRINTF("palThreadFunc5::after mutex: 0x%08x\n", status); 00160 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00161 #endif //MUTEX_UNITY_TEST 00162 tmp->arg5 = 50; 00163 threadID = pal_osThreadGetId(); 00164 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00165 PAL_PRINTF("Thread ID is %d\n", threadID); 00166 #ifdef MUTEX_UNITY_TEST 00167 status = pal_osMutexRelease(mutex1); 00168 PAL_PRINTF("palThreadFunc5::after release mutex: 0x%08x\n", status); 00169 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00170 #endif //MUTEX_UNITY_TEST 00171 PAL_PRINTF("palThreadFunc5::STAAAAM\n"); 00172 } 00173 00174 void palThreadFunc6(void const *argument) 00175 { 00176 volatile palThreadID_t threadID; 00177 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00178 #ifdef SEMAPHORE_UNITY_TEST 00179 palStatus_t status = PAL_SUCCESS; 00180 uint32_t semaphoresAvailable = 10; 00181 status = pal_osSemaphoreWait(123456, 200, &semaphoresAvailable); //MUST fail, since there is no semaphore with ID=3 00182 PAL_PRINTF("palThreadFunc6::semaphoresAvailable: %d\n", semaphoresAvailable); 00183 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_PARAMETER, status); 00184 return; 00185 #endif //SEMAPHORE_UNITY_TEST 00186 tmp->arg6 = 60; 00187 threadID = pal_osThreadGetId(); 00188 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00189 PAL_PRINTF("Thread ID is %d\n", threadID); 00190 #ifdef SEMAPHORE_UNITY_TEST 00191 status = pal_osSemaphoreRelease(123456); 00192 PAL_PRINTF("palThreadFunc6::pal_osSemaphoreRelease res: 0x%08x\n", status); 00193 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_PARAMETER, status); 00194 #endif //SEMAPHORE_UNITY_TEST 00195 PAL_PRINTF("palThreadFunc6::STAAAAM\n"); 00196 } 00197 00198 00199 void palTimerFunc1(void const *argument) 00200 { 00201 g_timerArgs.ticksInFunc1 = pal_osKernelSysTick(); 00202 PAL_PRINTF("ticks in palTimerFunc1: 0 - %" PRIu32 "\n", g_timerArgs.ticksInFunc1); 00203 PAL_PRINTF("Once Timer function was called\n"); 00204 } 00205 00206 void palTimerFunc2(void const *argument) 00207 { 00208 g_timerArgs.ticksInFunc2 = pal_osKernelSysTick(); 00209 PAL_PRINTF("ticks in palTimerFunc2: 0 - %" PRIu32 "\n", g_timerArgs.ticksInFunc2); 00210 PAL_PRINTF("Periodic Timer function was called\n"); 00211 } 00212 00213 void palTimerFunc3(void const *argument) 00214 { 00215 static int counter =0; 00216 counter++; 00217 } 00218 00219 void palTimerFunc4(void const *argument) 00220 { 00221 static int counter =0; 00222 counter++; 00223 g_timerArgs.ticksInFunc1 = counter; 00224 } 00225 00226 void palTimerFunc5(void const *argument) // function to count calls + wait alternatin short and long periods for timer drift test 00227 { 00228 static int counter = 0; 00229 counter++; 00230 g_timerArgs.ticksInFunc1 = counter; 00231 if (counter % 2 == 0) 00232 { 00233 pal_osDelay(PAL_TIMER_TEST_TIME_TO_WAIT_MS_LONG); 00234 } 00235 else 00236 { 00237 pal_osDelay(PAL_TIMER_TEST_TIME_TO_WAIT_MS_SHORT); 00238 } 00239 } 00240 00241 00242 void palThreadFuncCustom1(void const *argument) 00243 { 00244 PAL_PRINTF("palThreadFuncCustom1 was called\n"); 00245 } 00246 00247 void palThreadFuncCustom2(void const *argument) 00248 { 00249 PAL_PRINTF("palThreadFuncCustom2 was called\n"); 00250 } 00251 00252 void palThreadFuncCustom3(void const *argument) 00253 { 00254 palStatus_t status = PAL_SUCCESS; 00255 PAL_PRINTF("palThreadFuncCustom3 was called\n"); 00256 status = pal_osMutexWait(mutex1, PAL_RTOS_WAIT_FOREVER); 00257 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00258 00259 status = pal_osMutexRelease(mutex1); 00260 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00261 } 00262 00263 void palThreadFuncCustom4(void const *argument) 00264 { 00265 PAL_PRINTF("palThreadFuncCustom4 was called\n"); 00266 } 00267 00268 void palThreadFuncWaitForEverTest(void const *argument) 00269 { 00270 pal_osDelay(PAL_TIME_TO_WAIT_MS/2); 00271 pal_osSemaphoreRelease(*((palSemaphoreID_t*)(argument))); 00272 } 00273 00274 void palRunThreads() 00275 { 00276 palStatus_t status = PAL_SUCCESS; 00277 palThreadID_t threadID1 = NULLPTR; 00278 palThreadID_t threadID2 = NULLPTR; 00279 palThreadID_t threadID3 = NULLPTR; 00280 palThreadID_t threadID4 = NULLPTR; 00281 palThreadID_t threadID5 = NULLPTR; 00282 palThreadID_t threadID6 = NULLPTR; 00283 00284 status = pal_osThreadCreateWithAlloc(palThreadFunc1, &g_threadsArg, PAL_osPriorityIdle, PAL_TEST_THREAD_STACK_SIZE, &g_threadStorage, &threadID1); 00285 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00286 00287 status = pal_osThreadCreateWithAlloc(palThreadFunc2, &g_threadsArg, PAL_osPriorityLow, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID2); 00288 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00289 00290 status = pal_osThreadCreateWithAlloc(palThreadFunc3, &g_threadsArg, PAL_osPriorityNormal, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID3); 00291 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00292 00293 status = pal_osThreadCreateWithAlloc(palThreadFunc4, &g_threadsArg, PAL_osPriorityBelowNormal, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID4); 00294 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00295 00296 status = pal_osDelay(PAL_RTOS_THREAD_CLEANUP_TIMER_MILISEC * 2); // dealy to work around mbedOS timer issue (starting more than 6 timers at once will cause a hang) 00297 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00298 00299 status = pal_osThreadCreateWithAlloc(palThreadFunc5, &g_threadsArg, PAL_osPriorityAboveNormal, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID5); 00300 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00301 00302 status = pal_osThreadCreateWithAlloc(palThreadFunc6, &g_threadsArg, PAL_osPriorityHigh, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID6); 00303 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00304 00305 pal_osDelay(PAL_TIME_TO_WAIT_MS/5); 00306 00307 status = pal_osThreadTerminate(&threadID1); 00308 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00309 00310 status = pal_osThreadTerminate(&threadID2); 00311 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00312 00313 status = pal_osThreadTerminate(&threadID3); 00314 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00315 00316 status = pal_osThreadTerminate(&threadID4); 00317 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00318 00319 status = pal_osThreadTerminate(&threadID5); 00320 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00321 00322 status = pal_osThreadTerminate(&threadID6); 00323 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00324 } 00325 00326 void RecursiveLockThread(void const *param) 00327 { 00328 size_t i = 0; 00329 palStatus_t status; 00330 palRecursiveMutexParam_t *actualParams = (palRecursiveMutexParam_t*)param; 00331 size_t countbeforeStart = 0; 00332 volatile palThreadID_t threadID = 10; 00333 00334 status = pal_osSemaphoreWait(actualParams->sem, PAL_RTOS_WAIT_FOREVER, NULL); 00335 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00336 00337 for (i = 0; i < 100; ++i) 00338 { 00339 status = pal_osMutexWait(actualParams->mtx, PAL_RTOS_WAIT_FOREVER); 00340 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00341 if (i == 0) 00342 { 00343 countbeforeStart = actualParams->count; 00344 TEST_ASSERT_EQUAL_HEX(NULLPTR, actualParams->activeThread); 00345 actualParams->activeThread = pal_osThreadGetId(); 00346 } 00347 actualParams->count++; 00348 threadID = pal_osThreadGetId(); 00349 TEST_ASSERT_NOT_EQUAL(NULLPTR, threadID); 00350 TEST_ASSERT_EQUAL(actualParams->activeThread, threadID); 00351 pal_osDelay(1); 00352 } 00353 00354 pal_osDelay(50); 00355 TEST_ASSERT_EQUAL(100, actualParams->count - countbeforeStart); 00356 for (i = 0; i < 100; ++i) 00357 { 00358 threadID = pal_osThreadGetId(); 00359 TEST_ASSERT_NOT_EQUAL(NULLPTR, threadID); 00360 TEST_ASSERT_EQUAL(actualParams->activeThread, threadID); 00361 actualParams->count++; 00362 if (i == 99) 00363 { 00364 TEST_ASSERT_EQUAL(200, actualParams->count - countbeforeStart); 00365 actualParams->activeThread = NULLPTR; 00366 } 00367 00368 status = pal_osMutexRelease(actualParams->mtx); 00369 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00370 pal_osDelay(1); 00371 } 00372 00373 status = pal_osSemaphoreRelease(actualParams->sem); 00374 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00375 } 00376
Generated on Tue Jul 12 2022 16:22:10 by
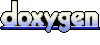