
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_rtos.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef _PAL_RTOS_H 00019 #define _PAL_RTOS_H 00020 00021 #include <stdint.h> 00022 #include <string.h> //memcpy 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 #include "pal_macros.h" 00029 #include "pal_types.h" 00030 #include "pal_configuration.h" //added for PAL_INITIAL_RANDOM_SIZE value 00031 00032 /*! \file pal_rtos.h 00033 * \brief PAL RTOS. 00034 * This file contains the real-time OS APIs and is a part of the PAL service API. 00035 * It provides thread, timers, semaphores, mutexes and memory pool management APIs. 00036 * Random API and ROT (root of trust) are also provided. 00037 */ 00038 00039 00040 //! Wait forever define. Used for semaphores and mutexes. 00041 #define PAL_TICK_TO_MILLI_FACTOR 1000 00042 00043 //! Primitive ID type declarations. 00044 typedef uintptr_t palThreadID_t; 00045 typedef uintptr_t palTimerID_t; 00046 typedef uintptr_t palMutexID_t; 00047 typedef uintptr_t palSemaphoreID_t; 00048 typedef uintptr_t palMemoryPoolID_t; 00049 typedef uintptr_t palMessageQID_t; 00050 00051 //! Timer types supported in PAL. 00052 typedef enum palTimerType { 00053 palOsTimerOnce = 0, /*! One shot timer. */ 00054 palOsTimerPeriodic = 1 /*! Periodic (repeating) timer. */ 00055 } palTimerType_t; 00056 00057 00058 PAL_DEPRECATED(palOsStorageEncryptionKey and palOsStorageSignatureKey are deprecated please use palOsStorageEncryptionKey128Bit palOsStorageSignatureKey128Bit) 00059 #define palOsStorageEncryptionKey palOsStorageEncryptionKey128Bit 00060 #define palOsStorageSignatureKey palOsStorageSignatureKey128Bit 00061 00062 00063 //! Device key types supported in PAL. 00064 typedef enum palDeviceKeyType { 00065 palOsStorageEncryptionKey128Bit = 0, /*! 128bit storage encryption key derived from RoT. */ 00066 palOsStorageSignatureKey128Bit = 1, /*! 128bit storage signature key derived from RoT. */ 00067 palOsStorageHmacSha256 = 2 00068 } palDevKeyType_t; 00069 00070 //! PAL timer function prototype. 00071 typedef void(*palTimerFuncPtr)(void const *funcArgument); 00072 00073 //! PAL thread function prototype. 00074 typedef void(*palThreadFuncPtr)(void const *funcArgument); 00075 00076 #define PAL_NUMBER_OF_THREADS_PRIORITIES (PAL_osPrioritylast-PAL_osPriorityFirst+1) 00077 //! Available priorities in PAL implementation, each priority can appear only once. 00078 typedef enum pal_osPriority { 00079 PAL_osPriorityFirst = -3, 00080 PAL_osPriorityIdle = PAL_osPriorityFirst, 00081 PAL_osPriorityLow = -2, 00082 PAL_osPriorityBelowNormal = -1, 00083 PAL_osPriorityNormal = 0, 00084 PAL_osPriorityAboveNormal = +1, 00085 PAL_osPriorityHigh = +2, 00086 PAL_osPriorityRealtime = +3, 00087 PAL_osPrioritylast = PAL_osPriorityRealtime, 00088 PAL_osPriorityError = 0x84 00089 } palThreadPriority_t; /*! Thread priority levels for PAL threads - each thread must have a different priority. */ 00090 00091 //! Thread local store struct. 00092 //! Can be used to hold for example state and configurations inside the thread. 00093 typedef struct pal_threadLocalStore{ 00094 void* storeData; 00095 } palThreadLocalStore_t; 00096 00097 typedef struct pal_timeVal{ 00098 int32_t pal_tv_sec; /*! Seconds. */ 00099 int32_t pal_tv_usec; /*! Microseconds. */ 00100 } pal_timeVal_t; 00101 00102 00103 //------- system general functions 00104 /*! Initiates a system reboot. 00105 */ 00106 void pal_osReboot(void); 00107 00108 //------- system tick functions 00109 /*! Get the RTOS kernel system timer counter. 00110 * \note The system needs to supply a 64-bit tick counter. If only a 32-bit counter is supported, 00111 * \note the counter wraps around very often (for example, once every 42 sec for 100Mhz). 00112 * \return The RTOS kernel system timer counter. 00113 */ 00114 uint64_t pal_osKernelSysTick(void); 00115 00116 00117 /*! Converts a value from microseconds to kernel system ticks. 00118 * 00119 * @param[in] microseconds The number of microseconds to convert into system ticks. 00120 * 00121 * \return Converted value in system ticks. 00122 */ 00123 uint64_t pal_osKernelSysTickMicroSec(uint64_t microseconds); 00124 00125 /*! Converts value from kernel system ticks to milliseconds. 00126 * 00127 * @param[in] sysTicks The number of kernel system ticks to convert into milliseconds. 00128 * 00129 * \return Converted value in system milliseconds. 00130 */ 00131 uint64_t pal_osKernelSysMilliSecTick(uint64_t sysTicks); 00132 00133 /*! Get the system tick frequency. 00134 * \return The system tick frequency. 00135 * 00136 * \note The system tick frequency MUST be more than 1KHz (at least one tick per millisecond). 00137 */ 00138 uint64_t pal_osKernelSysTickFrequency(void); 00139 00140 00141 /*! Get the system time. 00142 * \return The system 64-bit counter indicating the current system time in seconds on success. 00143 * Zero value when the time is not set in the system. 00144 */ 00145 uint64_t pal_osGetTime(void); 00146 00147 /*! \brief Set the current system time by accepting seconds since January 1st 1970 UTC+0. 00148 * 00149 * @param[in] seconds Seconds from January 1st 1970 UTC+0. 00150 * 00151 * \return PAL_SUCCESS when the time was set successfully. \n 00152 * PAL_ERR_INVALID_TIME when there is a failure setting the system time. 00153 */ 00154 palStatus_t pal_osSetTime(uint64_t seconds); 00155 00156 00157 /*! \brief <b>-----This function shall be deprecated in the next PAL release!!------ </b> 00158 * This function creates and starts the thread function (inside the PAL platform wrapper function). 00159 * @param[in] function A function pointer to the thread callback function. 00160 * @param[in] funcArgument An argument for the thread function. 00161 * @param[in] priority The priority of the thread. 00162 * @param[in] stackSize The stack size of the thread, can NOT be 0. 00163 * @param[in] stackPtr A pointer to the thread's stack. <b> ----Shall not be used, The user need to free this resource------ </b> 00164 * @param[in] store A pointer to thread's local store, can be NULL. 00165 * @param[out] threadID The created thread ID handle. In case of error, this value is NULL. 00166 * 00167 * \return PAL_SUCCESS when the thread was created successfully. \n 00168 * PAL_ERR_RTOS_PRIORITY when the given priority is already used in the system. 00169 * 00170 * \note Each thread MUST have a unique priority. 00171 * \note When the priority of the created thread function is higher than the current running thread, the 00172 * created thread function starts instantly and becomes the new running thread. 00173 */ 00174 palStatus_t pal_osThreadCreate(palThreadFuncPtr function, void* funcArgument, palThreadPriority_t priority, uint32_t stackSize, uint32_t* stackPtr, palThreadLocalStore_t* store, palThreadID_t* threadID); 00175 00176 /*! \brief Allocates memory for the thread stack, creates and starts the thread function (inside the PAL platform wrapper function). 00177 * 00178 * @param[in] function A function pointer to the thread callback function. 00179 * @param[in] funcArgument An argument for the thread function. 00180 * @param[in] priority The priority of the thread. 00181 * @param[in] stackSize The stack size of the thread, can NOT be 0. 00182 * @param[in] store A pointer to thread's local store, can be NULL. 00183 * @param[out] threadID: The created thread ID handle. In case of error, this value is NULL. 00184 * 00185 * \return PAL_SUCCESS when the thread was created successfully. \n 00186 * PAL_ERR_RTOS_PRIORITY when the given priority is already used in the system. 00187 * 00188 * \note Each thread MUST have a unique priority. 00189 * \note When the priority of the created thread function is higher than the current running thread, the 00190 * created thread function starts instantly and becomes the new running thread. 00191 * \note Calling \c pal_osThreadTerminate() releases the thread stack. 00192 */ 00193 palStatus_t pal_osThreadCreateWithAlloc(palThreadFuncPtr function, void* funcArgument, palThreadPriority_t priority, uint32_t stackSize, palThreadLocalStore_t* store, palThreadID_t* threadID); 00194 00195 /*! Terminate and free allocated data for the thread. 00196 * 00197 * @param[in] threadID The thread ID to stop and terminate. 00198 * 00199 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. \n 00200 * PAL_ERR_RTOS_RESOURCE if the thread ID is not correct. 00201 * \note pal_osThreadTerminate is a non blocking operation, pal_osThreadTerminate sends cancellation request to the thread, 00202 * usually the thread exits immediately, but the system does not always guarantee this 00203 */ 00204 palStatus_t pal_osThreadTerminate(palThreadID_t* threadID); 00205 00206 /*! Get the ID of the current thread. 00207 * \return The ID of the current thread. In case of error, return PAL_MAX_UINT32. 00208 * \note For a thread with real time priority, the function always returns PAL_MAX_UINT32. 00209 */ 00210 palThreadID_t pal_osThreadGetId(void); 00211 00212 /*! Get the storage of the current thread. 00213 * \return The storage of the current thread. 00214 */ 00215 palThreadLocalStore_t* pal_osThreadGetLocalStore(void); 00216 00217 /*! Wait for a specified time period in milliseconds. 00218 * 00219 * @param[in] milliseconds The number of milliseconds to wait before proceeding. 00220 * 00221 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00222 */ 00223 palStatus_t pal_osDelay(uint32_t milliseconds); 00224 00225 /*! Create a timer. 00226 * 00227 * @param[in] function A function pointer to the timer callback function. 00228 * @param[in] funcArgument An argument for the timer callback function. 00229 * @param[in] timerType The timer type to be created, periodic or oneShot. 00230 * @param[out] timerID The created timer ID handle. In case of error, this value is NULL. 00231 * 00232 * \return PAL_SUCCESS when the timer was created successfully. \n 00233 * PAL_ERR_NO_MEMORY when there is no memory resource available to create a timer object. 00234 * 00235 * \note The timer function runs according to the platform resources of stack-size and priority. 00236 */ 00237 palStatus_t pal_osTimerCreate(palTimerFuncPtr function, void* funcArgument, palTimerType_t timerType, palTimerID_t* timerID); 00238 00239 /*! Start or restart a timer. 00240 * 00241 * @param[in] timerID The handle for the timer to start. 00242 * @param[in] millisec The amount of time in milliseconds to set the timer to. MUST be larger than 0. 00243 * In case of 0 value, the error PAL_ERR_RTOS_VALUE is returned. 00244 * 00245 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00246 */ 00247 palStatus_t pal_osTimerStart(palTimerID_t timerID, uint32_t millisec); 00248 00249 /*! Stop a timer. 00250 * @param[in] timerID The handle for the timer to stop. 00251 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00252 */ 00253 palStatus_t pal_osTimerStop(palTimerID_t timerID); 00254 00255 /*! Delete the timer object. 00256 * 00257 * @param[inout] timerID The handle for the timer to delete. In success, `*timerID` = NULL. 00258 * 00259 * \return PAL_SUCCESS when timer was deleted successfully. \n 00260 * PAL_ERR_RTOS_PARAMETER when the `timerID` is incorrect. 00261 */ 00262 palStatus_t pal_osTimerDelete(palTimerID_t* timerID); 00263 00264 /*! Create and initialize a mutex object. 00265 * 00266 * @param[out] mutexID The created mutex ID handle. In case of error, this value is NULL. 00267 * 00268 * \return PAL_SUCCESS when the mutex was created successfully. \n 00269 * PAL_ERR_NO_MEMORY when there is no memory resource available to create a mutex object. 00270 */ 00271 palStatus_t pal_osMutexCreate(palMutexID_t* mutexID); 00272 00273 /*! Wait until a mutex becomes available. 00274 * 00275 * @param[in] mutexID The handle for the mutex. 00276 * @param[in] millisec The timeout for waiting to the mutex to be available. PAL_RTOS_WAIT_FOREVER can be used as a parameter. 00277 * 00278 * \return PAL_SUCCESS(0) in case of success or one of the following error codes in case of failure: \n 00279 * PAL_ERR_RTOS_RESOURCE - mutex was not availabe but no timeout was set. \n 00280 * PAL_ERR_RTOS_TIMEOUT - mutex was not available until the timeout. \n 00281 * PAL_ERR_RTOS_PARAMETER - mutex ID is invalid. \n 00282 * PAL_ERR_RTOS_ISR - cannot be called from the interrupt service routines. 00283 */ 00284 palStatus_t pal_osMutexWait(palMutexID_t mutexID, uint32_t millisec); 00285 00286 /*! Release a mutex that was obtained by `osMutexWait`. 00287 * 00288 * @param[in] mutexID The handle for the mutex. 00289 * \return PAL_SUCCESS(0) in case of success and another negative value indicating a specific error code in case of failure. 00290 */ 00291 palStatus_t pal_osMutexRelease(palMutexID_t mutexID); 00292 00293 /*!Delete a mutex object. 00294 * 00295 * @param[inout] mutexID The mutex handle to delete. In success, `*mutexID` = NULL. 00296 * 00297 * \return PAL_SUCCESS when the mutex was deleted successfully. \n 00298 * PAL_ERR_RTOS_RESOURCE - mutex is already released. \n 00299 * PAL_ERR_RTOS_PARAMETER - mutex ID is invalid. \n 00300 * PAL_ERR_RTOS_ISR - cannot be called from the interrupt service routines. \n 00301 * \note After this call, the `mutex_id` is no longer valid and cannot be used. 00302 */ 00303 palStatus_t pal_osMutexDelete(palMutexID_t* mutexID); 00304 00305 /*! Create and initialize a semaphore object. 00306 * 00307 * @param[in] count The number of available resources. 00308 * @param[out] semaphoreID The created semaphore ID handle. In case of error, this value is NULL. 00309 * 00310 * \return PAL_SUCCESS when the semaphore was created successfully. \n 00311 * PAL_ERR_NO_MEMORY when there is no memory resource available to create a semaphore object. 00312 */ 00313 palStatus_t pal_osSemaphoreCreate(uint32_t count, palSemaphoreID_t* semaphoreID); 00314 00315 /*! Wait until a semaphore token becomes available. 00316 * 00317 * @param[in] semaphoreID The handle for the semaphore. 00318 * @param[in] millisec The timeout for the waiting operation if the timeout 00319 expires before the semaphore is released and error is 00320 returned from the function. PAL_RTOS_WAIT_FOREVER can be used. 00321 * @param[out] countersAvailable The number of semaphores available at the call if a 00322 semaphore is available. If the semaphore is not available (timeout/error) zero is returned. This parameter can be NULL 00323 * \return PAL_SUCCESS(0) in case of success and one of the following error codes in case of failure: \n 00324 * PAL_ERR_RTOS_TIMEOUT - the semaphore was not available until timeout. \n 00325 * PAL_ERR_RTOS_PARAMETER - the semaphore ID is invalid. 00326 */ 00327 palStatus_t pal_osSemaphoreWait(palSemaphoreID_t semaphoreID, uint32_t millisec, int32_t* countersAvailable); 00328 00329 /*! Release a semaphore token. 00330 * 00331 * @param[in] semaphoreID The handle for the semaphore 00332 * 00333 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00334 */ 00335 palStatus_t pal_osSemaphoreRelease(palSemaphoreID_t semaphoreID); 00336 00337 /*! Delete a semaphore object. 00338 * 00339 * @param[inout] semaphoreID The semaphore handle to delete. In success, `*semaphoreID` = NULL. 00340 * 00341 * \return PAL_SUCCESS when the semaphore was deleted successfully. \n 00342 * PAL_ERR_RTOS_RESOURCE - the semaphore was already released. \n 00343 * PAL_ERR_RTOS_PARAMETER - the semaphore ID is invalid. 00344 * \note After this call, the `semaphore_id` is no longer valid and cannot be used. 00345 */ 00346 palStatus_t pal_osSemaphoreDelete(palSemaphoreID_t* semaphoreID); 00347 00348 /*! Create and initialize a memory pool. 00349 * 00350 * @param[in] blockSize The size of a single block in bytes. 00351 * @param[in] blockCount The maximum number of blocks in the memory pool. 00352 * @param[out] memoryPoolID The created memory pool ID handle. In case of error, this value is NULL. 00353 * 00354 * \return PAL_SUCCESS when the memory pool was created successfully. \n 00355 * PAL_ERR_NO_MEMORY when there is no memory resource available to create a memory pool object. 00356 */ 00357 palStatus_t pal_osPoolCreate(uint32_t blockSize, uint32_t blockCount, palMemoryPoolID_t* memoryPoolID); 00358 00359 /*! Allocate a single memory block from the memory pool. 00360 * 00361 * @param[in] memoryPoolID The handle for the memory pool. 00362 * 00363 * \return A pointer to a single allocated memory from the pool or NULL in case of failure. 00364 */ 00365 void* pal_osPoolAlloc(palMemoryPoolID_t memoryPoolID); 00366 00367 /*! Allocate a single memory block from the memory pool and set it to zero. 00368 * 00369 * @param[in] memoryPoolID The handle for the memory pool. 00370 * 00371 * \return A pointer to a single allocated memory from the pool or NULL in case of failure. 00372 */ 00373 void* pal_osPoolCAlloc(palMemoryPoolID_t memoryPoolID); 00374 00375 /*! Return a memory block back to a specific memory pool. 00376 * 00377 * @param[in] memoryPoolHandle The handle for the memory pool. 00378 * @param[in] block The block to free. 00379 * 00380 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00381 */ 00382 palStatus_t pal_osPoolFree(palMemoryPoolID_t memoryPoolID, void* block); 00383 00384 /*! Delete a memory pool object. 00385 * 00386 * @param[inout] memoryPoolID The handle for the memory pool. In success, `*memoryPoolID` = NULL. 00387 * 00388 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00389 */ 00390 palStatus_t pal_osPoolDestroy(palMemoryPoolID_t* memoryPoolID); 00391 00392 00393 /*! Create and initialize a message queue of `uint32_t` messages. 00394 * 00395 * @param[in] messageQCount The maximum number of messages in the queue. 00396 * @param[out] messageQID: The created message queue ID handle. In case of error, this value is NULL. 00397 * 00398 * \return PAL_SUCCESS when the message queue was created successfully. \n 00399 * PAL_ERR_NO_MEMORY when there is no memory resource available to create a message queue object. 00400 */ 00401 palStatus_t pal_osMessageQueueCreate(uint32_t messageQCount, palMessageQID_t* messageQID); 00402 00403 /*! Put a message to a queue. 00404 * 00405 * @param[in] messageQID The handle for the message queue. 00406 * @param[in] info The data to send. 00407 * @param[in] timeout The timeout in milliseconds. Value zero means that the function returns instantly. \n 00408 The value PAL_RTOS_WAIT_FOREVER means that the function waits for an infinite time until the message can be put to the queue. 00409 * 00410 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00411 */ 00412 palStatus_t pal_osMessagePut(palMessageQID_t messageQID, uint32_t info, uint32_t timeout); 00413 00414 /*! Get a message or wait for a message from a queue. 00415 * 00416 * @param[in] messageQID The handle for the memory pool. 00417 * @param[in] timeout The timeout in milliseconds. Value zero means that the function returns instantly \n 00418 The value PAL_RTOS_WAIT_FOREVER means that the funtion waits for an infinite time until message can be put to the queue. 00419 * @param[out] messageValue The message value from the message queue. 00420 * 00421 * \return PAL_SUCCESS(0) in case of success and one of the following error codes in case of failure: \n 00422 * PAL_ERR_RTOS_TIMEOUT - no message arrived during the given timeout period. Can also be returned in case of timeout zero value, which means that there are no messages in the queue. 00423 */ 00424 palStatus_t pal_osMessageGet(palMessageQID_t messageQID, uint32_t timeout, uint32_t* messageValue); 00425 00426 /*! Delete a message queue object. 00427 * 00428 * @param[in,out] messageQID The handle for the message queue. In success, `*messageQID` = NULL. 00429 * 00430 * \return PAL_SUCCESS(0) in case of success and a negative value indicating a specific error code in case of failure. 00431 */ 00432 palStatus_t pal_osMessageQueueDestroy(palMessageQID_t* messageQID); 00433 00434 /*! Perform an atomic increment for a signed 32-bit value. 00435 * 00436 * @param[in,out] valuePtr The address of the value to increment. 00437 * @param[in] increment The number by which to increment. 00438 * 00439 * \return The value of `valuePtr` after the increment operation. 00440 */ 00441 int32_t pal_osAtomicIncrement(int32_t* valuePtr, int32_t increment); 00442 00443 /*! Generate a 32-bit random number. 00444 * 00445 * @param[out] random A 32-bit buffer to hold the generated number. 00446 * 00447 \note `pal_init()` MUST be called before this function. 00448 \return PAL_SUCCESS on success, a negative value indicating a specific error code in case of failure. 00449 */ 00450 palStatus_t pal_osRandom32bit(uint32_t *random); 00451 00452 /*! Generate random number into given buffer with given size in bytes. 00453 * 00454 * @param[out] randomBuf A buffer to hold the generated number. 00455 * @param[in] bufSizeBytes The size of the buffer and the size of the required random number to generate. 00456 * 00457 \note `pal_init()` MUST be called before this function 00458 \return PAL_SUCCESS on success, a negative value indicating a specific error code in case of failure. 00459 */ 00460 palStatus_t pal_osRandomBuffer(uint8_t *randomBuf, size_t bufSizeBytes); 00461 00462 /*! Generate a 32-bit random number uniformly distributed less than `upperBound`. 00463 * 00464 * @param[out] random A pointer to a 32-bit buffer to hold the generated number. 00465 * 00466 \note `pal_init()` MUST be called before this function 00467 \note For the first phase, the `upperBound` parameter is ignored. 00468 \return PAL_SUCCESS on success, a negative value indicating a specific error code in case of failure. 00469 */ 00470 palStatus_t pal_osRandomUniform(uint32_t upperBound, uint32_t *random); 00471 00472 /*! Return a device unique key derived from the root of trust. 00473 * 00474 * @param[in] keyType The type of key to derive. 00475 * @param[in,out] key A 128-bit OR 256-bit buffer to hold the derived key, size is defined according to the `keyType`. 00476 * @param[in] keyLenBytes The size of buffer to hold the 128-bit OR 256-bit key. 00477 * \return PAL_SUCCESS in case of success and one of the following error codes in case of failure: \n 00478 * PAL_ERR_GET_DEV_KEY - an error in key derivation. 00479 */ 00480 PAL_DEPRECATED(pal_osGetDeviceKey128Bit is deprecated please use pal_osGetDeviceKey) 00481 #define pal_osGetDeviceKey128Bit pal_osGetDeviceKey 00482 00483 palStatus_t pal_osGetDeviceKey(palDevKeyType_t keyType, uint8_t *key, size_t keyLenBytes); 00484 00485 00486 /*! Initialize the RTOS module for PAL. 00487 * This function can be called only once before running the system. 00488 * To remove PAL from the system, call `pal_RTOSDestroy`. 00489 * After calling `pal_RTOSDestroy`, you can call `pal_RTOSInitialize` again. 00490 * 00491 * @param[in] opaqueContext: context to be passed to the platform if needed. 00492 * 00493 * \return PAL_SUCCESS upon success. 00494 */ 00495 palStatus_t pal_RTOSInitialize(void* opaqueContext); 00496 00497 00498 /*! This function removes PAL from the system and can be called after `pal_RTOSInitialize`. 00499 ** 00500 * \return PAL_SUCCESS upon success. \n 00501 * PAL_ERR_NOT_INITIALIZED - if the user did not call `pal_RTOSInitialize()` first. 00502 */ 00503 palStatus_t pal_RTOSDestroy(void); 00504 00505 00506 00507 #ifdef __cplusplus 00508 } 00509 #endif 00510 #endif //_PAL_RTOS_H
Generated on Tue Jul 12 2022 16:22:10 by
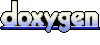