
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_plat_update.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef PAL_PLAT_UPDATE_HEADER 00019 #define PAL_PLAT_UPDATE_HEADER 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #include "pal_rtos.h" 00026 #include "pal_update.h" 00027 00028 00029 /*! \file pal_plat_update.h 00030 * \brief PAL update - platform. 00031 * This file contains the firmware update APIs that need to be implemented in the platform layer. 00032 */ 00033 00034 00035 /*! Set the callback function that is called before the end of each API (except `imageGetDirectMemAccess`). 00036 * @param[in] CBfunction A pointer to the callback function. 00037 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00038 */ 00039 palStatus_t pal_plat_imageInitAPI (palImageSignalEvent_t CBfunction); 00040 00041 00042 /*! Clear all the resources used by the `pal_update` APIs. 00043 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00044 */ 00045 palStatus_t pal_plat_imageDeInit (void); 00046 00047 /*! Set the `imageNumber` to the number of available images. You can do this through the hard coded define inside the linker script. 00048 * @param[out] imageNumber The total number of images the system supports. 00049 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00050 */ 00051 palStatus_t pal_plat_imageGetMaxNumberOfImages (uint8_t* imageNumber); 00052 00053 /*! Claim space in the relevant storage region for `imageId` with the size of the image. 00054 * @param[in] imageId The image ID. 00055 * @param[in] imageSize The size of the images. 00056 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00057 */ 00058 palStatus_t pal_plat_imageReserveSpace (palImageId_t imageId, size_t imageSize); 00059 00060 00061 /*! Set up the details for the image header. The data is written when the image write is called for the first time. 00062 * @param[in] imageId The image ID. 00063 * @param[in] details The data needed to build the image header. 00064 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00065 */ 00066 palStatus_t pal_plat_imageSetHeader (palImageId_t imageId,palImageHeaderDeails_t* details); 00067 00068 /*! Write the data in the chunk buffer with the size written in chunk `bufferLength` in the location of `imageId` adding the relative offset. 00069 * @param[in] imageId The image ID. 00070 * @param[in] offset The relative offset to write the data into. 00071 * @param[in] chunk A pointer to the struct containing the data and the data length to write. 00072 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00073 */ 00074 palStatus_t pal_plat_imageWrite (palImageId_t imageId, size_t offset, palConstBuffer_t* chunk); 00075 00076 /*! Update the image version of `imageId` to the version written in version buffer with version `bufferLength`. 00077 * @param[in] imageId The image ID. 00078 * @param[in] version The image version and its length. 00079 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00080 */ 00081 palStatus_t pal_plat_imageSetVersion (palImageId_t imageId, const palConstBuffer_t* version); 00082 00083 /*! Flush the entire image data after writing ends for `imageId`. 00084 * @param[in] imageId The image ID. 00085 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00086 */ 00087 palStatus_t pal_plat_imageFlush (palImageId_t imageId); 00088 00089 /*! Verify whether the `imageId` is readable and set `imagePtr` to point to the beginning of the image in the memory and `imageSizeInBytes` to the image size. \n 00090 * @param[in] imageId The image ID. 00091 * @param[out] imagePtr A pointer to the start of the image. 00092 * @param[out] imageSizeInBytes The size of the image. 00093 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure and sets `imagePtr` to NULL. 00094 */ 00095 palStatus_t pal_plat_imageGetDirectMemAccess (palImageId_t imageId, void** imagePtr, size_t* imageSizeInBytes); 00096 00097 00098 /*! Read the max of chunk `maxBufferLength` bytes from the `imageId` with relative offset and store it in chunk buffer. \n 00099 * Set the chunk `bufferLength` value to the actual number of bytes read. 00100 * \note Please use this API in case the image is not directly accessible via the `imageGetDirectMemAccess` function. 00101 * @param[in] imageId The image ID. 00102 * @param[in] offset The offset to start reading from. 00103 * @param[out] chunk The data and actual bytes read. 00104 */ 00105 palStatus_t pal_plat_imageReadToBuffer (palImageId_t imageId, size_t offset, palBuffer_t* chunk); 00106 00107 /*! Set the `imageId` to be the active image (after device reset). 00108 * @param[in] imageId The image ID. 00109 */ 00110 palStatus_t pal_plat_imageActivate (palImageId_t imageId); 00111 00112 /*! Retrieve the hash value of the active image to the hash buffer with the max size hash `maxBufferLength` and set the hash `bufferLength` to the hash size. 00113 * @param[out] hash The hash and actual size of hash read. 00114 */ 00115 palStatus_t pal_plat_imageGetActiveHash (palBuffer_t* hash); 00116 00117 /*! Retrieve the version of the active image to the version buffer with the size set to version `bufferLength`. 00118 * @param[out] version The version and actual size of version read. 00119 */ 00120 palStatus_t pal_plat_imageGetActiveVersion (palBuffer_t* version); 00121 00122 /*! Write the `dataId` stored in `dataBuffer` to the memory accessible to the bootloader. Currently, only HASH is available. 00123 * @param[in] hashValue The data and size of the HASH. 00124 */ 00125 palStatus_t pal_plat_imageWriteHashToMemory (const palConstBuffer_t* const hashValue); 00126 00127 00128 00129 00130 #endif /* SOURCE_PAL_IMPL_MODULES_UPDATE_PAL_PALT_UPDATE_H_ */ 00131 #ifdef __cplusplus 00132 } 00133 #endif
Generated on Tue Jul 12 2022 16:22:10 by
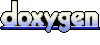