
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_plat_internalFlash.h
00001 #ifndef PAL_PLAT_FLASH_H_ 00002 #define PAL_PLAT_FLASH_H_ 00003 00004 #include "pal_internalFlash.h" 00005 00006 #ifdef __cplusplus 00007 extern "C" { 00008 #endif 00009 00010 /*! \brief This function initialized the flash API module, 00011 * And should be called prior flash APIs calls 00012 * 00013 * \return PAL_SUCCESS upon successful operation. \n 00014 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00015 * 00016 * \note should be called only once unless \c pal_InternalFlashDeinit function is called 00017 * \note This function is Blocking till completion!! 00018 * 00019 */ 00020 palStatus_t pal_plat_internalFlashInit(void); 00021 00022 /*! \brief This function destroy the flash module 00023 * 00024 * \return PAL_SUCCESS upon successful operation. \n 00025 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00026 * 00027 * \note Should be called only after \c pal_InternalFlashinit() is called. 00028 * \note Flash APIs will not work after calling this function 00029 * \note This function is Blocking till completion!! 00030 * 00031 */ 00032 palStatus_t pal_plat_internalFlashDeInit(void); 00033 00034 /*! \brief This function writes to the internal flash 00035 * 00036 * @param[in] buffer - pointer to the buffer to be written 00037 * @param[in] size - the size of the buffer in bytes, must be aligned to minimum writing unit (page size). 00038 * @param[in] address - the address of the internal flash. 00039 * 00040 * \return PAL_SUCCESS upon successful operation. \n 00041 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00042 * 00043 * \note This function is Blocking till completion!! 00044 * \note This function is Thread Safe!! 00045 */ 00046 palStatus_t pal_plat_internalFlashWrite(const size_t size, const uint32_t address, const uint32_t * buffer); 00047 00048 /*! \brief This function copies the memory data into the user given buffer 00049 * 00050 * @param[in] size - the size of the buffer in bytes. 00051 * @param[in] address - the address of the internal flash. 00052 * @param[out] buffer - pointer to the buffer to write to 00053 * 00054 * \return PAL_SUCCESS upon successful operation. \n 00055 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00056 * \note This function is Blocking till completion!! 00057 * \note This function is Thread Safe!! 00058 * 00059 */ 00060 palStatus_t pal_plat_internalFlashRead(const size_t size, const uint32_t address, uint32_t * buffer); 00061 00062 /*! \brief This function Erase the sector 00063 * 00064 * @param[in] size - the size to be erased, must be align to sector. 00065 * @param[in] address - sector start address to be erased, must be align to sector. 00066 * 00067 * \return PAL_SUCCESS upon successful operation. \n 00068 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00069 * 00070 * \note ALL sectors can be erased!! No protection to bootloader, program or other... 00071 * \note This function is Blocking till completion!! 00072 * \note Only one sector can be erased in each function call 00073 * \note This function is Thread Safe!! 00074 */ 00075 palStatus_t pal_plat_internalFlashErase(uint32_t address, size_t size); 00076 00077 /*! \brief This function returns the minimum writing unit to the flash 00078 * 00079 * \return size_t the 2, 4, 8.... 00080 */ 00081 size_t pal_plat_internalFlashGetPageSize(void); 00082 00083 00084 /*! \brief This function returns the sector size 00085 * 00086 * @param[in] address - the starting address of the sector is question 00087 * 00088 * \return size of sector, 0 if error 00089 */ 00090 size_t pal_plat_internalFlashGetSectorSize(uint32_t address); 00091 00092 00093 00094 /////////////////////////////////////////////////////////////// 00095 ////-------------------SOTP functions------------------------// 00096 /////////////////////////////////////////////////////////////// 00097 /*! \brief This function return the SOTP section data 00098 * 00099 * @param[in] section - the section number (0 or 1) 00100 * @param[out] data - the information about the section 00101 * 00102 * \return PAL_SUCCESS upon successful operation. \n 00103 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00104 * 00105 */ 00106 palStatus_t pal_plat_internalFlashGetAreaInfo(bool section, palSotpAreaData_t *data); 00107 00108 00109 #ifdef __cplusplus 00110 } 00111 #endif 00112 00113 #endif /* PAL_PLAT_FLASH_H_ */
Generated on Tue Jul 12 2022 16:22:09 by
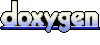