
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_network.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef _PAL_SOCKET_H 00019 #define _PAL_SOCKET_H 00020 00021 #include "pal_rtos.h" 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 /*! \file pal_network.h 00028 * \brief PAL network. 00029 * This file contains the network APIs and it is a part of the PAL service API. 00030 * It provides network functionalities for UDP and TCP sockets and connections. 00031 */ 00032 00033 //! PAL network socket API \n 00034 //! PAL network sockets configurations options: \n 00035 //! Set PAL_NET_TCP_AND_TLS_SUPPORT to true if TCP is supported by the platform and is required. \n 00036 //! Set PAL_NET_ASYNCHRONOUS_SOCKET_API to true if asynchronous socket API is supported by the platform and is required: Currently MANDATORY. 00037 //! Set PAL_NET_DNS_SUPPORT to true if DNS URL lookup API is supported. 00038 00039 typedef uint32_t palSocketLength_t; /*! The length of data. */ 00040 typedef void* palSocket_t ; /*! PAL socket handle type. */ 00041 00042 #define PAL_NET_MAX_ADDR_SIZE 32 // check if we can make this more efficient 00043 00044 typedef struct palSocketAddress { 00045 unsigned short addressType; /*! Address family for the socket. */ 00046 char addressData[PAL_NET_MAX_ADDR_SIZE]; /*! Address (based on protocol). */ 00047 } palSocketAddress_t; /*! Address data structure with enough room to support IPV4 and IPV6. */ 00048 00049 typedef struct palNetInterfaceInfo { 00050 char interfaceName[16]; //15 + ‘\0’ 00051 palSocketAddress_t address; 00052 uint32_t addressSize; 00053 } palNetInterfaceInfo_t ; 00054 00055 typedef enum { 00056 PAL_AF_UNSPEC = 0, 00057 PAL_AF_INET = 2, /*! Internet IP Protocol. */ 00058 PAL_AF_INET6 = 10, /*! IP version 6. */ 00059 } palSocketDomain_t ;/*! Network domains supported by PAL. */ 00060 00061 typedef enum { 00062 #if PAL_NET_TCP_AND_TLS_SUPPORT 00063 PAL_SOCK_STREAM = 1, /*! Stream socket. */ 00064 PAL_SOCK_STREAM_SERVER = 99, /*! Stream socket. */ 00065 #endif //PAL_NET_TCP_AND_TLS_SUPPORT 00066 PAL_SOCK_DGRAM = 2 /*! Datagram socket. */ 00067 } palSocketType_t ;/*! Socket types supported by PAL. */ 00068 00069 00070 typedef enum { 00071 PAL_SO_REUSEADDR = 0x0004, /*! Allow local address reuse. */ 00072 #if PAL_NET_TCP_AND_TLS_SUPPORT // Socket options below supported only if TCP is supported. 00073 PAL_SO_KEEPALIVE = 0x0008, /*! Keep TCP connection open even if idle using periodic messages. */ 00074 PAL_SO_KEEPIDLE = 0x0009, /*! The time (in seconds) the connection needs to remain idle before TCP starts sending keepalive probes, if the socket option SO_KEEPALIVE has been set on this socket. */ 00075 PAL_SO_KEEPINTVL = 0x0010, /*! The time (in seconds) between individual keepalive probes */ 00076 #endif //PAL_NET_TCP_AND_TLS_SUPPORT 00077 PAL_SO_SNDTIMEO = 0x1005, /*! Send timeout. */ 00078 PAL_SO_RCVTIMEO = 0x1006, /*! Receive timeout. */ 00079 } palSocketOptionName_t ;/*! Socket options supported by PAL. */ 00080 00081 #define PAL_NET_DEFAULT_INTERFACE 0xFFFFFFFF 00082 00083 #define PAL_IPV4_ADDRESS_SIZE 4 00084 #define PAL_IPV6_ADDRESS_SIZE 16 00085 00086 typedef uint8_t palIpV4Addr_t[PAL_IPV4_ADDRESS_SIZE]; 00087 typedef uint8_t palIpV6Addr_t[PAL_IPV6_ADDRESS_SIZE]; 00088 00089 00090 /*! Register a network interface for use with PAL sockets. Must be called before other socket functions. Most APIs will not work before a single interface is added. 00091 * @param[in] networkInterfaceContext The network interface to be added (OS specific. For example in mbed OS, this is the `NetworkInterface` object pointer for the network adapter [**Note:** We assume that connect has already been called on this]). If not available use NULL. 00092 * @param[out] interfaceIndex Contains the index assigned to the interface in case it has been assigned successfully. This index can be used, when creating a socket, to bind the socket to the interface. 00093 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00094 */ 00095 palStatus_t pal_registerNetworkInterface(void* networkInterfaceContext, uint32_t* interfaceIndex); 00096 00097 /*! Set a port to `palSocketAddress_t`. \n 00098 * You can set it either directly or via the `palSetSockAddrIPV4Addr` or `palSetSockAddrIPV6Addr` functions. 00099 * @param[in,out] address The address to set. 00100 * @param[in] port The port number to set. 00101 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00102 \note To set the socket correctly, the `addressType` field of the address must be set correctly. 00103 */ 00104 palStatus_t pal_setSockAddrPort(palSocketAddress_t* address, uint16_t port); 00105 00106 /*! Set an IPv4 address to `palSocketAddress_t` and `addressType` to IPv4. 00107 * @param[in,out] address The address to set. 00108 * @param[in] ipV4Addr The address value to set. 00109 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00110 */ 00111 palStatus_t pal_setSockAddrIPV4Addr(palSocketAddress_t* address, palIpV4Addr_t ipV4Addr); 00112 00113 /*! Set an IPv6 address to `palSocketAddress_t` and the `addressType` to IPv6. 00114 * @param[in,out] address The address to set. 00115 * @param[in] ipV6Addr The address value to set. 00116 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00117 */ 00118 palStatus_t pal_setSockAddrIPV6Addr(palSocketAddress_t* address, palIpV6Addr_t ipV6Addr); 00119 00120 /*! Get an IPv4 address from `palSocketAddress_t`. 00121 * @param[in] address The address to set. 00122 * @param[out] ipV4Addr The address that is set in `address`. 00123 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00124 */ 00125 palStatus_t pal_getSockAddrIPV4Addr(const palSocketAddress_t* address, palIpV4Addr_t ipV4Addr); 00126 00127 /*! Get an IPv6 address from `palSocketAddress_t`. 00128 * @param[in] address The address to set. 00129 * @param[out] ipV6Addr The address that is set in `address`. 00130 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00131 */ 00132 palStatus_t pal_getSockAddrIPV6Addr(const palSocketAddress_t* address, palIpV6Addr_t ipV6Addr); 00133 00134 /*! Get a port from `palSocketAddress_t`. 00135 * @param[in] address The address to set. 00136 * @param[out] port The port that is set in `address`. 00137 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00138 */ 00139 palStatus_t pal_getSockAddrPort(const palSocketAddress_t* address, uint16_t* port); 00140 00141 /*! Get a network socket. 00142 * @param[in] domain The domain for the created socket (see `palSocketDomain_t` for supported types). 00143 * @param[in] type The type of the created socket (see `palSocketType_t` for supported types). 00144 * @param[in] nonBlockingSocket If true, the socket is created as non-blocking (with O_NONBLOCK set). 00145 * @param[in] interfaceNum The number of the network interface used for this socket (info in interfaces supported via `pal_getNumberOfNetInterfaces` and `pal_getNetInterfaceInfo`). Select PAL_NET_DEFAULT_INTERFACE for the default interface. 00146 * @param[out] socket The socket is returned through this output parameter. 00147 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00148 */ 00149 palStatus_t pal_socket(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palSocket_t* socket); 00150 00151 /*! Get the value for a given socket option on a given network socket. 00152 * @param[in] socket The socket for which to get options. 00153 * @param[in] optionName The identification of the socket option for which we are getting the value (see enum palSocketOptionName_t for supported types). 00154 * @param[out] optionValue A buffer holding the option value returned by the function. 00155 * @param[in, out] optionLength The size of the buffer provided for `optionValue` when calling the function. After the call, it contains the length of data actually written to the `optionValue` buffer. 00156 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00157 */ 00158 palStatus_t pal_getSocketOptions(palSocket_t socket, palSocketOptionName_t optionName, void* optionValue, palSocketLength_t* optionLength); 00159 00160 /*! Set the value for a given socket option on a given network socket. 00161 * @param[in] socket The socket for which to get options. 00162 * @param[in] optionName The identification of the socket option for which we are getting the value (see enum palSocketOptionName_t for supported types). 00163 * @param[in] optionValue The buffer holding the option value to set for the given option. 00164 * @param[in] optionLength The size of the buffer provided for `optionValue`. 00165 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00166 */ 00167 palStatus_t pal_setSocketOptions(palSocket_t socket, int optionName, const void* optionValue, palSocketLength_t optionLength); 00168 00169 /*! Check if a given socket is non-blocking. 00170 * @param[in] socket The socket to check. 00171 * @param[out] isNonBlocking True if the socket is non-blocking, otherwise false. 00172 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00173 */ 00174 palStatus_t pal_isNonBlocking(palSocket_t socket, bool* isNonBlocking); 00175 00176 00177 /*! Bind a given socket to a local address. 00178 * @param[in] socket The socket to bind. 00179 * @param[in] myAddress The address to bind to. 00180 * @param[in] addressLength The length of the address passed in `myAddress`. 00181 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00182 */ 00183 palStatus_t pal_bind(palSocket_t socket, palSocketAddress_t* myAddress, palSocketLength_t addressLength); 00184 00185 /*! Receive a payload from the given socket. 00186 * @param[in] socket The socket to receive from. [The sockets passed to this function should be of type PAL_SOCK_DGRAM (the implementation may support other types as well).] 00187 * @param[out] buffer The buffer for the payload data. 00188 * @param[in] length The length of the buffer for the payload data. 00189 * @param[out] from The address that sent the payload. 00190 * @param[in, out] fromLength The length of the `from` address. Contains the amount of data actually written to the `from` address. 00191 * @param[out] bytesReceived The actual amount of payload data received in the buffer. 00192 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00193 */ 00194 palStatus_t pal_receiveFrom(palSocket_t socket, void* buffer, size_t length, palSocketAddress_t* from, palSocketLength_t* fromLength, size_t* bytesReceived); 00195 00196 /*! Send a payload to the given address using the given socket. 00197 * @param[in] socket The socket to use for sending the payload. [The sockets passed to this function should be of type PAL_SOCK_DGRAM (the implementation may support other types as well).] 00198 * @param[in] buffer The buffer for the payload data. 00199 * @param[in] length The length of the buffer for the payload data. 00200 * @param[in] to The address to which the payload should be sent. 00201 * @param[in] toLength The length of the `to` address. 00202 * @param[out] bytesSent The actual amount of payload data sent. 00203 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00204 */ 00205 palStatus_t pal_sendTo(palSocket_t socket, const void* buffer, size_t length, const palSocketAddress_t* to, palSocketLength_t toLength, size_t* bytesSent); 00206 00207 /*! Close a network socket. 00208 * @param[in,out] The socket to be closed. 00209 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00210 \note Receives `palSocket_t*`, NOT `palSocket_t`, so that it can zero the socket to avoid re-use. 00211 */ 00212 palStatus_t pal_close(palSocket_t* socket); 00213 00214 /*! Get the number of current network interfaces. 00215 * @param[out] numInterfaces The number of interfaces. 00216 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00217 */ 00218 palStatus_t pal_getNumberOfNetInterfaces(uint32_t* numInterfaces); 00219 00220 /*! Get information regarding the socket at the index/interface number given (this number is returned when registering the socket). 00221 * @param[in] interfaceNum The number of the interface to get information for. 00222 * @param[out] interfaceInfo Set to the information for the given interface number. 00223 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00224 */ 00225 palStatus_t pal_getNetInterfaceInfo(uint32_t interfaceNum, palNetInterfaceInfo_t * interfaceInfo); 00226 00227 00228 #define PAL_NET_SOCKET_SELECT_MAX_SOCKETS 8 00229 #define PAL_NET_SOCKET_SELECT_RX_BIT (1) 00230 #define PAL_NET_SOCKET_SELECT_TX_BIT (2) 00231 #define PAL_NET_SOCKET_SELECT_ERR_BIT (4) 00232 00233 #define PAL_NET_SELECT_IS_RX(socketStatus, index) ((socketStatus[index] & PAL_NET_SOCKET_SELECT_RX_BIT) != 0) /*! Check if RX bit is set in select result for a given socket index. */ 00234 #define PAL_NET_SELECT_IS_TX(socketStatus, index) ((socketStatus[index] & PAL_NET_SOCKET_SELECT_TX_BIT) != 0) /*! Check if TX bit is set in select result for a given socket index. */ 00235 #define PAL_NET_SELECT_IS_ERR(socketStatus, index) ((socketStatus[index] & PAL_NET_SOCKET_SELECT_ERR_BIT) != 0) /*! Check if ERR bit is set in select result for a given socket index. */ 00236 00237 /*! Check if one or more (up to PAL_NET_SOCKET_SELECT_MAX_SOCKETS) sockets given has data available for reading/writing/error. The function will block until data is available for one of the given sockets or the timeout expires. \n 00238 To use the function, set the sockets you want to check in the `socketsToCheck` array and set a timeout. When it returns the `socketStatus` output indicates the status of each socket passed in. \n 00239 \note If the timeout expires, the returned `palStatus_t` is PAL_SUCCESS and `numberOfSocketsSet` is 0. 00240 * @param[in] socketsToCheck On input, the array of up to 8 sockets handles to check. 00241 * @param[in] numberOfSockets The number of sockets set in the input `socketsToCheck` array. 00242 * @param[in] timeout The amount of time until timeout if no socket activity is detected 00243 * @param[out] socketStatus Information on each socket in the input array, indicating which event was set (none, rx, tx, err). Check the desired event using macros. 00244 * @param[out] numberOfSocketsSet The total number of sockets set in all three data sets (tx, rx, err). 00245 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00246 \note The entry in index x in the `socketStatus` array corresponds to the socket at index x in the sockets to check array. 00247 */ 00248 palStatus_t pal_socketMiniSelect(const palSocket_t socketsToCheck[PAL_NET_SOCKET_SELECT_MAX_SOCKETS], uint32_t numberOfSockets, pal_timeVal_t* timeout, 00249 uint8_t palSocketStatus[PAL_NET_SOCKET_SELECT_MAX_SOCKETS], uint32_t* numberOfSocketsSet); 00250 00251 00252 #if PAL_NET_TCP_AND_TLS_SUPPORT // The functionality below is supported only if TCP is supported. 00253 00254 00255 /*! Use the given socket to listen for incoming connections. This may also limit the queue of incoming connections. 00256 * @param[in] socket The socket to listen to. [The sockets passed to this function should be of type PAL_SOCK_STREAM_SERVER (the implementation may support other types as well).] 00257 * @param[in] backlog The amount of pending connections that can be saved for the socket. 00258 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00259 */ 00260 palStatus_t pal_listen(palSocket_t socket, int backlog); 00261 00262 /*! Accept a connection on the given socket. 00263 * @param[in] socket The socket on which to accept the connection. (The socket must be already created and bound and listen has must have been called on it.) [The sockets passed to this function should be of type PAL_SOCK_STREAM_SERVER (the implementation may support other types as well).] 00264 * @param[out] address The source address of the incoming connection. 00265 * @param[in, out] addressLen The length of the address field on input, the length of the data returned on output. 00266 * @param[out] acceptedSocket The socket of the accepted connection. 00267 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00268 */ 00269 palStatus_t pal_accept(palSocket_t socket, palSocketAddress_t* address, palSocketLength_t* addressLen, palSocket_t* acceptedSocket); 00270 00271 /*! Open a connection from the given socket to the given address. 00272 * @param[in] socket The socket to use for connection to the given address. [The sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well).] 00273 * @param[in] address The destination address of the connection. 00274 * @param[in] addressLen The length of the address field. 00275 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00276 */ 00277 palStatus_t pal_connect(palSocket_t socket, const palSocketAddress_t* address, palSocketLength_t addressLen); 00278 00279 /*! Receive data from the given connected socket. 00280 * @param[in] socket The connected socket on which to receive data. [The sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well).] 00281 * @param[out] buf The output buffer for the message data. 00282 * @param[in] len The length of the input data buffer. 00283 * @param[out] recievedDataSize The length of the data actually received. 00284 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00285 */ 00286 palStatus_t pal_recv(palSocket_t socket, void* buf, size_t len, size_t* recievedDataSize); 00287 00288 /*! Send a given buffer via the given connected socket. 00289 * @param[in] socket The connected socket on which to send data. [The sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well).] 00290 * @param[in] buf The output buffer for the message data. 00291 * @param[in] len The length of the input data buffer. 00292 * @param[out] sentDataSize The length of the data sent. 00293 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00294 */ 00295 palStatus_t pal_send(palSocket_t socket, const void* buf, size_t len, size_t* sentDataSize); 00296 00297 00298 #endif //PAL_NET_TCP_AND_TLS_SUPPORT 00299 00300 00301 #if PAL_NET_ASYNCHRONOUS_SOCKET_API 00302 00303 /*! The type of the callback funciton passed when creating asynchronous sockets. 00304 * @param[in] argument The user provided argument passed to the callback function. 00305 */ 00306 typedef void(*palAsyncSocketCallback_t )(void*); 00307 00308 /*! Get an asynchronous network socket. 00309 * @param[in] domain The domain for the created socket (see enum `palSocketDomain_t` for supported types). 00310 * @param[in] type The type for the created socket (see enum `palSocketType_t` for supported types). 00311 * @param[in] nonBlockingSocket If true, the socket is created as non-blocking (with O_NONBLOCK set). 00312 * @param[in] interfaceNum The number of the network interface used for this socket (info in interfaces supported via `pal_getNumberOfNetInterfaces` and `pal_getNetInterfaceInfo`). Select PAL_NET_DEFAULT_INTERFACE for the default interface. 00313 * @param[in] callback A callback function that is called when any supported event happens in the given asynchronous socket (see `palAsyncSocketCallbackType` enum for the types of events supported). 00314 * @param[out] socket The socket is returned through this output parameter. 00315 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00316 */ 00317 palStatus_t pal_asynchronousSocket(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, palSocket_t* socket); 00318 00319 /*! Get an asynchronous network socket that passes the provided `callbackArgument` to the provided callback on callback events. 00320 * @param[in] domain The domain for the created socket (see enum `palSocketDomain_t` for supported types). 00321 * @param[in] type The type for the created socket (see enum `palSocketType_t` for supported types). 00322 * @param[in] nonBlockingSocket If true, the socket is created as non-blocking (with O_NONBLOCK set). 00323 * @param[in] interfaceNum The number of the network interface used for this socket (info in interfaces supported via `pal_getNumberOfNetInterfaces` and `pal_getNetInterfaceInfo`). Select PAL_NET_DEFAULT_INTERFACE for the default interface. 00324 * @param[in] callback A callback function that is called when any supported event happens in the given asynchronous socket. 00325 * @param[in] callbackArgument The argument with which the callback function is called when any supported event happens in the given asynchronous socket. 00326 * @param[out] socket The socket is returned through this output parameter. 00327 \return PAL_SUCCESS (0) in case of success or a specific negative error code in case of failure. 00328 */ 00329 palStatus_t pal_asynchronousSocketWithArgument(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback,void* callbackArgument, palSocket_t* socket); 00330 00331 00332 00333 #endif 00334 00335 #if PAL_NET_DNS_SUPPORT 00336 00337 /*! This function translates from a URL to `palSocketAddress_t` which can be used with PAL sockets. It supports both IP address as strings and URLs (using DNS lookup). 00338 * @param[in] url The URL (or IP address string) to be translated to a `palSocketAddress_t`. 00339 * @param[out] address The address for the output of the translation. 00340 */ 00341 palStatus_t pal_getAddressInfo(const char* url, palSocketAddress_t* address, palSocketLength_t* addressLength); 00342 00343 #endif 00344 00345 #ifdef __cplusplus 00346 } 00347 #endif 00348 #endif //_PAL_SOCKET_H 00349 00350
Generated on Tue Jul 12 2022 16:22:09 by
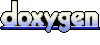