
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_network.c
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "pal.h" 00019 #include "pal_network.h" 00020 #include "pal_plat_network.h" 00021 00022 typedef struct pal_in_addr { 00023 uint32_t s_addr; // that's a 32-bit int (4 bytes) 00024 } pal_in_addr_t; 00025 00026 typedef struct pal_socketAddressInternal { 00027 short int pal_sin_family; // address family 00028 unsigned short int pal_sin_port; // port 00029 pal_in_addr_t pal_sin_addr; // ipv4 address 00030 unsigned char pal_sin_zero[8]; // 00031 } pal_socketAddressInternal_t; 00032 00033 typedef struct pal_socketAddressInternal6{ 00034 uint16_t pal_sin6_family; // address family, 00035 uint16_t pal_sin6_port; // port number, Network Byte Order 00036 uint32_t pal_sin6_flowinfo; // IPv6 flow information 00037 palIpV6Addr_t pal_sin6_addr; // IPv6 address 00038 uint32_t pal_sin6_scope_id; // Scope ID 00039 } pal_socketAddressInternal6_t; 00040 00041 00042 00043 palStatus_t pal_registerNetworkInterface(void* networkInterfaceContext, uint32_t* interfaceIndex) 00044 { 00045 palStatus_t result = PAL_SUCCESS; 00046 if (networkInterfaceContext != NULL && interfaceIndex != NULL) 00047 { 00048 result = pal_plat_registerNetworkInterface (networkInterfaceContext, interfaceIndex); 00049 } 00050 else 00051 { 00052 result = PAL_ERR_INVALID_ARGUMENT ; 00053 } 00054 00055 return result; 00056 } 00057 00058 palStatus_t pal_setSockAddrPort(palSocketAddress_t* address, uint16_t port) 00059 { 00060 palStatus_t result = PAL_SUCCESS; 00061 if (NULL == address) 00062 { 00063 return PAL_ERR_INVALID_ARGUMENT ; 00064 } 00065 00066 if (address->addressType == PAL_AF_INET) 00067 { 00068 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00069 // Set Linux format 00070 innerAddr->pal_sin_port = PAL_HTONS(port); 00071 } 00072 else if (address->addressType == PAL_AF_INET6 ) 00073 { 00074 pal_socketAddressInternal6_t * innerAddr = (pal_socketAddressInternal6_t*)address; 00075 // Set Linux format 00076 innerAddr->pal_sin6_port = PAL_HTONS(port); 00077 } 00078 else 00079 { 00080 result = PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00081 } 00082 00083 return result; 00084 } 00085 00086 00087 palStatus_t pal_setSockAddrIPV4Addr(palSocketAddress_t* address, palIpV4Addr_t ipV4Addr) 00088 { 00089 if ((NULL == address) || (NULL == ipV4Addr)) 00090 { 00091 return PAL_ERR_INVALID_ARGUMENT ; 00092 } 00093 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00094 innerAddr->pal_sin_family = PAL_AF_INET; 00095 innerAddr->pal_sin_addr.s_addr = (ipV4Addr[0]) | (ipV4Addr[1] << 8) | (ipV4Addr[2] << 16) | (ipV4Addr[3] << 24); 00096 return PAL_SUCCESS; 00097 } 00098 00099 00100 palStatus_t pal_setSockAddrIPV6Addr(palSocketAddress_t* address, palIpV6Addr_t ipV6Addr) 00101 { 00102 int index; 00103 00104 if ((NULL == address) || (NULL == ipV6Addr)) 00105 { 00106 return PAL_ERR_INVALID_ARGUMENT ; 00107 } 00108 pal_socketAddressInternal6_t* innerAddr = (pal_socketAddressInternal6_t*)address; 00109 innerAddr->pal_sin6_family = PAL_AF_INET6 ; 00110 for (index = 0; index < PAL_IPV6_ADDRESS_SIZE; index++) // TODO: use mem copy? 00111 { 00112 innerAddr->pal_sin6_addr[index] = ipV6Addr[index]; 00113 } 00114 return PAL_SUCCESS; 00115 } 00116 00117 00118 palStatus_t pal_getSockAddrIPV4Addr(const palSocketAddress_t* address, palIpV4Addr_t ipV4Addr) 00119 { 00120 palStatus_t result = PAL_SUCCESS; 00121 if (NULL == address) 00122 { 00123 return PAL_ERR_INVALID_ARGUMENT ; 00124 } 00125 if (address->addressType == PAL_AF_INET) 00126 { 00127 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00128 ipV4Addr[0] = (innerAddr->pal_sin_addr.s_addr) & 0xFF; 00129 ipV4Addr[1] = (innerAddr->pal_sin_addr.s_addr >> 8) & 0xFF; 00130 ipV4Addr[2] = (innerAddr->pal_sin_addr.s_addr >> 16) & 0xFF; 00131 ipV4Addr[3] = (innerAddr->pal_sin_addr.s_addr >> 24) & 0xFF; 00132 00133 } 00134 else 00135 { 00136 result = PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00137 } 00138 return result; 00139 } 00140 00141 00142 palStatus_t pal_getSockAddrIPV6Addr(const palSocketAddress_t* address, palIpV6Addr_t ipV6Addr) 00143 { 00144 palStatus_t result = PAL_SUCCESS; 00145 int index = 0; 00146 if (address->addressType == PAL_AF_INET6 ) 00147 { 00148 pal_socketAddressInternal6_t * innerAddr = (pal_socketAddressInternal6_t*)address; 00149 for (index = 0; index < PAL_IPV6_ADDRESS_SIZE; index++) // TODO: use mem copy? 00150 { 00151 ipV6Addr[index] = innerAddr->pal_sin6_addr[index]; 00152 } 00153 } 00154 else 00155 { 00156 result = PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00157 } 00158 return result; 00159 } 00160 00161 00162 palStatus_t pal_getSockAddrPort(const palSocketAddress_t* address, uint16_t* port) 00163 { 00164 palStatus_t result = PAL_SUCCESS; 00165 if ((NULL == address) || (NULL == port)) 00166 { 00167 return PAL_ERR_INVALID_ARGUMENT ; 00168 } 00169 00170 if (address->addressType == PAL_AF_INET) 00171 { 00172 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00173 // Set numeric formal 00174 *port = PAL_NTOHS(innerAddr->pal_sin_port); 00175 } 00176 else if (address->addressType == PAL_AF_INET6 ) 00177 { 00178 pal_socketAddressInternal6_t * innerAddr = (pal_socketAddressInternal6_t*)address; 00179 // Set numeric formal 00180 *port = PAL_NTOHS(innerAddr->pal_sin6_port); 00181 } 00182 else 00183 { 00184 result = PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00185 } 00186 00187 return result; 00188 } 00189 00190 00191 palStatus_t pal_socket(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palSocket_t* socket) 00192 { 00193 palStatus_t result = PAL_SUCCESS; 00194 if (NULL == socket) 00195 { 00196 return PAL_ERR_INVALID_ARGUMENT ; 00197 } 00198 result = pal_plat_socket (domain, type, nonBlockingSocket, interfaceNum, socket); 00199 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00200 } 00201 00202 00203 palStatus_t pal_getSocketOptions(palSocket_t socket, palSocketOptionName_t optionName, void* optionValue, palSocketLength_t* optionLength) 00204 { 00205 palStatus_t result = PAL_SUCCESS; 00206 if ((NULL == optionValue) || (NULL == optionLength)) 00207 { 00208 return PAL_ERR_INVALID_ARGUMENT ; 00209 } 00210 result = pal_plat_getSocketOptions (socket, optionName, optionValue, optionLength); 00211 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00212 } 00213 00214 00215 palStatus_t pal_setSocketOptions(palSocket_t socket, int optionName, const void* optionValue, palSocketLength_t optionLength) 00216 { 00217 palStatus_t result = PAL_SUCCESS; 00218 if (NULL == optionValue) 00219 { 00220 return PAL_ERR_INVALID_ARGUMENT ; 00221 } 00222 result = pal_plat_setSocketOptions ( socket, optionName, optionValue, optionLength); 00223 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00224 } 00225 00226 palStatus_t pal_isNonBlocking(palSocket_t socket, bool* isNonBlocking) 00227 { 00228 palStatus_t result = PAL_SUCCESS; 00229 if (NULL == isNonBlocking) 00230 { 00231 return PAL_ERR_INVALID_ARGUMENT ; 00232 } 00233 result = pal_plat_isNonBlocking (socket, isNonBlocking); 00234 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00235 } 00236 00237 00238 palStatus_t pal_bind(palSocket_t socket, palSocketAddress_t* myAddress, palSocketLength_t addressLength) 00239 { 00240 palStatus_t result = PAL_SUCCESS; 00241 if (NULL == myAddress) 00242 { 00243 return PAL_ERR_INVALID_ARGUMENT ; 00244 } 00245 result = pal_plat_bind (socket, myAddress, addressLength); 00246 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00247 } 00248 00249 00250 palStatus_t pal_receiveFrom(palSocket_t socket, void* buffer, size_t length, palSocketAddress_t* from, palSocketLength_t* fromLength, size_t* bytesReceived) 00251 { 00252 palStatus_t result = PAL_SUCCESS; 00253 if ((NULL == buffer) || (NULL == bytesReceived)) 00254 { 00255 return PAL_ERR_INVALID_ARGUMENT ; 00256 } 00257 result = pal_plat_receiveFrom (socket, buffer, length, from, fromLength, bytesReceived); 00258 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00259 } 00260 00261 00262 palStatus_t pal_sendTo(palSocket_t socket, const void* buffer, size_t length, const palSocketAddress_t* to, palSocketLength_t toLength, size_t* bytesSent) 00263 { 00264 palStatus_t result = PAL_SUCCESS; 00265 if ((NULL == buffer) || (NULL == bytesSent) || (NULL == to)) 00266 { 00267 return PAL_ERR_INVALID_ARGUMENT ; 00268 } 00269 result = pal_plat_sendTo (socket, buffer, length, to, toLength, bytesSent); 00270 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00271 } 00272 00273 00274 palStatus_t pal_close(palSocket_t* socket) 00275 { 00276 palStatus_t result = PAL_SUCCESS; 00277 if (NULL == socket ) 00278 { 00279 return PAL_ERR_INVALID_ARGUMENT ; 00280 } 00281 result = pal_plat_close (socket); 00282 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00283 } 00284 00285 00286 palStatus_t pal_getNumberOfNetInterfaces( uint32_t* numInterfaces) 00287 { 00288 palStatus_t result = PAL_SUCCESS; 00289 if (NULL == numInterfaces) 00290 { 00291 return PAL_ERR_INVALID_ARGUMENT ; 00292 } 00293 result = pal_plat_getNumberOfNetInterfaces (numInterfaces); 00294 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00295 } 00296 00297 00298 palStatus_t pal_getNetInterfaceInfo(uint32_t interfaceNum, palNetInterfaceInfo_t * interfaceInfo) 00299 { 00300 palStatus_t result = PAL_SUCCESS; 00301 00302 if (NULL == interfaceInfo) 00303 { 00304 return PAL_ERR_INVALID_ARGUMENT ; 00305 } 00306 result = pal_plat_getNetInterfaceInfo (interfaceNum, interfaceInfo); 00307 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00308 } 00309 00310 00311 palStatus_t pal_socketMiniSelect(const palSocket_t socketsToCheck[PAL_NET_SOCKET_SELECT_MAX_SOCKETS], uint32_t numberOfSockets, 00312 pal_timeVal_t* timeout, uint8_t palSocketStatus[PAL_NET_SOCKET_SELECT_MAX_SOCKETS], uint32_t * numberOfSocketsSet) 00313 { 00314 palStatus_t result = PAL_SUCCESS; 00315 if ((NULL == socketsToCheck) || (NULL == numberOfSocketsSet) || (PAL_NET_SOCKET_SELECT_MAX_SOCKETS < numberOfSockets)) 00316 { 00317 return PAL_ERR_INVALID_ARGUMENT ; 00318 } 00319 result = pal_plat_socketMiniSelect (socketsToCheck, numberOfSockets, timeout, palSocketStatus, numberOfSocketsSet); 00320 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00321 } 00322 00323 00324 #if PAL_NET_TCP_AND_TLS_SUPPORT // functionality below supported only in case TCP is supported. 00325 00326 palStatus_t pal_listen(palSocket_t socket, int backlog) 00327 { 00328 palStatus_t result = PAL_SUCCESS; 00329 result = pal_plat_listen (socket, backlog); 00330 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00331 } 00332 00333 00334 palStatus_t pal_accept(palSocket_t socket, palSocketAddress_t* address, palSocketLength_t* addressLen, palSocket_t* acceptedSocket) 00335 { 00336 palStatus_t result = PAL_SUCCESS; 00337 if ((NULL == acceptedSocket) || (NULL == address)|| (NULL == addressLen)) 00338 { 00339 return PAL_ERR_INVALID_ARGUMENT ; 00340 } 00341 result = pal_plat_accept (socket, address, addressLen, acceptedSocket); 00342 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00343 } 00344 00345 00346 palStatus_t pal_connect(palSocket_t socket, const palSocketAddress_t* address, palSocketLength_t addressLen) 00347 { 00348 palStatus_t result = PAL_SUCCESS; 00349 if (NULL == address) 00350 { 00351 return PAL_ERR_INVALID_ARGUMENT ; 00352 } 00353 result = pal_plat_connect ( socket, address, addressLen); 00354 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00355 } 00356 00357 00358 palStatus_t pal_recv(palSocket_t socket, void* buf, size_t len, size_t* recievedDataSize) 00359 { 00360 palStatus_t result = PAL_SUCCESS; 00361 if ((NULL == recievedDataSize) || (NULL == buf)) 00362 { 00363 return PAL_ERR_INVALID_ARGUMENT ; 00364 } 00365 result = pal_plat_recv (socket, buf, len, recievedDataSize); 00366 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00367 } 00368 00369 00370 palStatus_t pal_send(palSocket_t socket, const void* buf, size_t len, size_t* sentDataSize) 00371 { 00372 palStatus_t result = PAL_SUCCESS; 00373 if ((NULL == buf) || (NULL == sentDataSize)) 00374 { 00375 return PAL_ERR_INVALID_ARGUMENT ; 00376 } 00377 result = pal_plat_send ( socket, buf, len, sentDataSize); 00378 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00379 } 00380 00381 00382 #endif //PAL_NET_TCP_AND_TLS_SUPPORT 00383 00384 00385 #if PAL_NET_ASYNCHRONOUS_SOCKET_API 00386 00387 palStatus_t pal_asynchronousSocket(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, palSocket_t* socket) 00388 { 00389 palStatus_t result = PAL_SUCCESS; 00390 if ((NULL == socket) || (NULL == callback)) 00391 { 00392 return PAL_ERR_INVALID_ARGUMENT ; 00393 } 00394 result = pal_plat_asynchronousSocket (domain, type, nonBlockingSocket, interfaceNum, callback, NULL, socket); 00395 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00396 } 00397 00398 palStatus_t pal_asynchronousSocketWithArgument(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, void* callbackArgument, palSocket_t* socket) 00399 { 00400 palStatus_t result = PAL_SUCCESS; 00401 if ((NULL == socket) || (NULL == callback)) 00402 { 00403 return PAL_ERR_INVALID_ARGUMENT ; 00404 } 00405 result = pal_plat_asynchronousSocket (domain, type, nonBlockingSocket, interfaceNum, callback, callbackArgument, socket); 00406 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00407 } 00408 00409 #endif 00410 00411 #if PAL_NET_DNS_SUPPORT 00412 00413 palStatus_t pal_getAddressInfo(const char *url, palSocketAddress_t *address, palSocketLength_t* addressLength) 00414 { 00415 palStatus_t result = PAL_SUCCESS; 00416 if ((NULL == url) || (NULL == address) || (NULL == addressLength)) 00417 { 00418 return PAL_ERR_INVALID_ARGUMENT ; 00419 } 00420 result = pal_plat_getAddressInfo (url, address, addressLength); 00421 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00422 } 00423 00424 #endif 00425 00426 00427 00428 00429
Generated on Tue Jul 12 2022 16:22:09 by
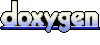