
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_init.c
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "pal.h" 00019 #include "pal_plat_network.h" 00020 #include "pal_plat_TLS.h" 00021 #include "pal_plat_Crypto.h" 00022 #include "pal_macros.h" 00023 00024 00025 00026 //this variable must be a int32_t for using atomic increment 00027 PAL_PRIVATE int32_t g_palIntialized = 0; 00028 00029 00030 PAL_PRIVATE void pal_modulesCleanup(void) 00031 { 00032 DEBUG_PRINT("Destroying modules\r\n"); 00033 pal_plat_cleanupTLS (); 00034 pal_plat_socketsTerminate (NULL); 00035 pal_RTOSDestroy(); 00036 pal_plat_cleanupCrypto (); 00037 pal_internalFlashDeInit(); 00038 } 00039 00040 00041 palStatus_t pal_init(void) 00042 { 00043 00044 palStatus_t status = PAL_SUCCESS; 00045 int32_t currentInitValue; 00046 // get the return value of g_palIntialized+1 to save it locally 00047 currentInitValue = pal_osAtomicIncrement(&g_palIntialized,1); 00048 // if increased for the 1st time 00049 if (1 == currentInitValue) 00050 { 00051 DEBUG_PRINT("\nInit for the 1st time, initializing the modules\r\n"); 00052 status = pal_RTOSInitialize(NULL); 00053 if (PAL_SUCCESS == status) 00054 { 00055 DEBUG_PRINT("\n1. Network init\r\n"); 00056 status = pal_plat_socketsInit(NULL); 00057 if (PAL_SUCCESS != status) 00058 { 00059 DEBUG_PRINT("init of network module has failed with status %" PRIu32 "\r\n",status); 00060 } 00061 else //socket init succeeded 00062 { 00063 DEBUG_PRINT("\n2. TLS init\r\n"); 00064 status = pal_plat_initTLSLibrary (); 00065 if (PAL_SUCCESS != status) 00066 { 00067 DEBUG_PRINT("init of tls module has failed with status %" PRIu32 "\r\n",status); 00068 } 00069 else 00070 { 00071 DEBUG_PRINT("\n3. Crypto init\r\n"); 00072 status = pal_plat_initCrypto (); 00073 if (PAL_SUCCESS != status) 00074 { 00075 DEBUG_PRINT("init of crypto module has failed with status %" PRIu32 "\r\n",status); 00076 } 00077 else 00078 { 00079 DEBUG_PRINT("\n4. Internal Flash init\r\n"); 00080 status = pal_internalFlashInit(); 00081 if (PAL_SUCCESS != status) 00082 { 00083 DEBUG_PRINT("init of Internal Flash module has failed with status %" PRIu32 "\r\n",status); 00084 } 00085 } 00086 } 00087 } 00088 } 00089 else 00090 { 00091 DEBUG_PRINT("init of RTOS module has failed with status %" PRIu32 "\r\n",status); 00092 } 00093 00094 // if failed decrease the value of g_palIntialized 00095 if (PAL_SUCCESS != status) 00096 { 00097 pal_modulesCleanup(); 00098 pal_osAtomicIncrement(&g_palIntialized, -1); 00099 PAL_LOG(ERR,"\nInit failed\r\n"); 00100 } 00101 } 00102 00103 00104 return status; 00105 } 00106 00107 00108 int32_t pal_destroy(void) 00109 { 00110 int32_t currentInitValue; 00111 // get the current value of g_palIntialized locally 00112 currentInitValue = pal_osAtomicIncrement(&g_palIntialized, 0); 00113 if(currentInitValue != 0) 00114 { 00115 currentInitValue = pal_osAtomicIncrement(&g_palIntialized, -1); 00116 if (0 == currentInitValue) 00117 { 00118 pal_modulesCleanup(); 00119 } 00120 } 00121 return currentInitValue; 00122 }
Generated on Tue Jul 12 2022 16:22:09 by
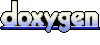