
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_configuration.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef _PAL_COFIGURATION_H 00019 #define _PAL_COFIGURATION_H 00020 #include "limits.h" 00021 00022 00023 #ifdef PAL_USER_DEFINED_CONFIGURATION 00024 #include PAL_USER_DEFINED_CONFIGURATION 00025 #endif 00026 00027 /*! \brief let the user choose its platform configuration file. 00028 \note if the user does not specify a platform configuration file, 00029 \note PAL uses a default configuration set that can be found at \b Configs/pal_config folder 00030 */ 00031 00032 #ifdef PAL_PLATFORM_DEFINED_CONFIGURATION 00033 #include PAL_PLATFORM_DEFINED_CONFIGURATION 00034 #elif defined(__LINUX__) 00035 #include "palInclude_Linux.h" 00036 #elif defined(__FREERTOS__) 00037 #include "palInclude_FreeRTOS.h" 00038 #elif defined(__MBED__) 00039 #include "palInclude_mbedOS.h" 00040 #else 00041 #error "Please specify the platform PAL_PLATFORM_DEFINED_CONFIGURATION" 00042 #endif 00043 00044 /*! \file pal_configuration.h 00045 * \brief PAL Configuration. 00046 * This file contains PAL configuration information including the following: 00047 * 1. The flags to enable or disable features. 00048 * 2. The configuration of the number of objects provided by PAL (such as the number of threads supported) or their sizes. 00049 * 3. The configuration of supported cipher suites. 00050 * 4. The configuration for flash memory usage. 00051 * 5. The configuration for the root of trust. 00052 */ 00053 00054 00055 /* 00056 * Network configuration 00057 */ 00058 //! PAL configuration options 00059 #ifndef PAL_NET_TCP_AND_TLS_SUPPORT 00060 #define PAL_NET_TCP_AND_TLS_SUPPORT true/* Add PAL support for TCP. */ 00061 #endif 00062 00063 #ifndef PAL_NET_ASYNCHRONOUS_SOCKET_API 00064 #define PAL_NET_ASYNCHRONOUS_SOCKET_API true/* Add PAL support for asynchronous sockets. */ 00065 #endif 00066 00067 #ifndef PAL_NET_DNS_SUPPORT 00068 #define PAL_NET_DNS_SUPPORT true/* Add PAL support for DNS lookup. */ 00069 #endif 00070 00071 //values for PAL_NET_DNS_IP_SUPPORT 00072 #define PAL_NET_DNS_ANY 0 /* if PAL_NET_DNS_IP_SUPPORT is set to PAL_NET_DNS_ANY pal_getAddressInfo will return the first available IPV4 or IPV6 address*/ 00073 #define PAL_NET_DNS_IPV4_ONLY 2 /* if PAL_NET_DNS_IP_SUPPORT is set to PAL_NET_DNS_IPV4_ONLY pal_getAddressInfo will return the first available IPV4 address*/ 00074 #define PAL_NET_DNS_IPV6_ONLY 4 /* if PAL_NET_DNS_IP_SUPPORT is set to PAL_NET_DNS_IPV6_ONLY pal_getAddressInfo will return the first available IPV6 address*/ 00075 00076 #ifndef PAL_NET_DNS_IP_SUPPORT 00077 #define PAL_NET_DNS_IP_SUPPORT 0 /* sets the type of IP addresses returned by pal_getAddressInfo*/ 00078 #endif 00079 00080 //! The maximum number of interfaces that can be supported at a time. 00081 #ifndef PAL_MAX_SUPORTED_NET_INTERFACES 00082 #define PAL_MAX_SUPORTED_NET_INTERFACES 10 00083 #endif 00084 00085 /* 00086 * RTOS configuration 00087 */ 00088 00089 #ifndef PAL_IGNORE_UNIQUE_THREAD_PRIORITY 00090 #define PAL_UNIQUE_THREAD_PRIORITY true 00091 #endif 00092 00093 //! The number of valid priorities limits the number of threads. If priorities are added this value should be increased. 00094 #ifndef PAL_MAX_NUMBER_OF_THREADS 00095 #define PAL_MAX_NUMBER_OF_THREADS 7 00096 #endif 00097 00098 //! initial time until thread stack cleanup (mbedOs only). This is the amount of time we wait before checking that a thread has completed so we can free it's stack. 00099 #ifndef PAL_RTOS_THREAD_CLEANUP_TIMER_MILISEC 00100 #define PAL_RTOS_THREAD_CLEANUP_TIMER_MILISEC 200 00101 #endif 00102 00103 //! This define is used to determine the size of the initial random buffer (in bytes) held by PAL for random the algorithm. 00104 #ifndef PAL_INITIAL_RANDOM_SIZE 00105 #define PAL_INITIAL_RANDOM_SIZE 48 00106 #endif 00107 00108 #ifndef PAL_RTOS_WAIT_FOREVER 00109 #define PAL_RTOS_WAIT_FOREVER UINT_MAX 00110 #endif 00111 00112 /* 00113 * TLS configuration 00114 */ 00115 //! The the maximum number of TLS contexts supported. 00116 #ifndef PAL_MAX_NUM_OF_TLS_CTX 00117 #define PAL_MAX_NUM_OF_TLS_CTX 1 00118 #endif 00119 00120 //! The maximum number of supported cipher suites. 00121 #ifndef PAL_MAX_ALLOWED_CIPHER_SUITES 00122 #define PAL_MAX_ALLOWED_CIPHER_SUITES 1 00123 #endif 00124 00125 //! This value is in milliseconds. 1000 = 1 second. 00126 #ifndef PAL_DTLS_PEER_MIN_TIMEOUT 00127 #define PAL_DTLS_PEER_MIN_TIMEOUT 1000 00128 #endif 00129 00130 //! The debug threshold for TLS API. 00131 #ifndef PAL_TLS_DEBUG_THRESHOLD 00132 #define PAL_TLS_DEBUG_THRESHOLD 5 00133 #endif 00134 00135 //! Define the cipher suites for TLS (only one cipher suite per device available). 00136 #define PAL_TLS_PSK_WITH_AES_128_CBC_SHA256_SUITE 0x01 00137 #define PAL_TLS_PSK_WITH_AES_128_CCM_8_SUITE 0x02 00138 #define PAL_TLS_PSK_WITH_AES_256_CCM_8_SUITE 0x04 00139 #define PAL_TLS_ECDHE_ECDSA_WITH_AES_128_CCM_8_SUITE 0x08 00140 #define PAL_TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256_SUITE 0x10 00141 #define PAL_TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384_SUITE 0x20 00142 00143 //! Use the default cipher suite for TLS/DTLS operations 00144 #define PAL_TLS_CIPHER_SUITE PAL_TLS_ECDHE_ECDSA_WITH_AES_128_CCM_8_SUITE 00145 00146 /* 00147 * UPDATE configuration 00148 */ 00149 00150 #define PAL_UPDATE_USE_FLASH 1 00151 #define PAL_UPDATE_USE_FS 2 00152 00153 #ifndef PAL_UPDATE_IMAGE_LOCATION 00154 #define PAL_UPDATE_IMAGE_LOCATION PAL_UPDATE_USE_FS //!< Choose the storage correct Storage option, File System or Flash 00155 #endif 00156 00157 //! Certificate date validation in Unix time format. 00158 #ifndef PAL_CRYPTO_CERT_DATE_LENGTH 00159 #define PAL_CRYPTO_CERT_DATE_LENGTH sizeof(uint64_t) 00160 #endif 00161 00162 00163 /* 00164 * FS configuration 00165 */ 00166 00167 00168 /* !\brief file system configurations 00169 * PAL_NUMBER_OF_PARTITIONS 00170 * 0 - Default behavior for the platform (Described by either 1 or 2 below). 00171 * 1 - There is a single partition in which the ARM client applications create and remove files (but do not format it). 00172 * 2 - There are two partitions in which ARM client applications may format or create and remove files, 00173 * depending on PAL_PRIMARY_PARTITION_PRIVATE and PAL_SECONDARY_PARTITION_PRIVATE 00174 */ 00175 #ifndef PAL_NUMBER_OF_PARTITIONS 00176 #define PAL_NUMBER_OF_PARTITIONS 1 // Default partitions 00177 #endif 00178 00179 #if (PAL_NUMBER_OF_PARTITIONS > 2) 00180 #error "PAL_NUMBER_OF_PARTITIONS cannot be more then 2" 00181 #endif 00182 00183 // PAL_PRIMARY_PARTITION_PRIVATE 00184 // 1 if the primary partition is exclusively dedicated to the ARM client applications. 00185 // 0 if the primary partition is used for storing other files as well. 00186 #ifndef PAL_PRIMARY_PARTITION_PRIVATE 00187 #define PAL_PRIMARY_PARTITION_PRIVATE 0 00188 #endif 00189 00190 //! PAL_SECONDARY_PARTITION_PRIVATE 00191 //! 1 if the secondary partition is exclusively dedicated to the ARM client applications. 00192 //! 0 if the secondary partition is used for storing other files as well. 00193 #ifndef PAL_SECONDARY_PARTITION_PRIVATE 00194 #define PAL_SECONDARY_PARTITION_PRIVATE 0 00195 #endif 00196 00197 //! This define is the location of the primary mount point for the file system 00198 #ifndef PAL_FS_MOUNT_POINT_PRIMARY 00199 #define PAL_FS_MOUNT_POINT_PRIMARY "" 00200 #endif 00201 00202 //! This define is the location of the secondary mount point for the file system 00203 #ifndef PAL_FS_MOUNT_POINT_SECONDARY 00204 #define PAL_FS_MOUNT_POINT_SECONDARY "" 00205 #endif 00206 00207 00208 // Update 00209 00210 #ifndef PAL_UPDATE_FIRMWARE_MOUNT_POINT 00211 #define PAL_UPDATE_FIRMWARE_MOUNT_POINT PAL_FS_MOUNT_POINT_PRIMARY 00212 #endif 00213 //! The location of the firmware update folder 00214 #ifndef PAL_UPDATE_FIRMWARE_DIR 00215 #define PAL_UPDATE_FIRMWARE_DIR PAL_UPDATE_FIRMWARE_MOUNT_POINT "/firmware" 00216 #endif 00217 00218 #endif //_PAL_COFIGURATION_H
Generated on Tue Jul 12 2022 16:22:08 by
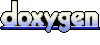