
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
pal_Crypto.c
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "pal.h" 00017 #include "pal_plat_Crypto.h" 00018 00019 00020 palStatus_t pal_initAes(palAesHandle_t *aes) 00021 { 00022 palStatus_t status = PAL_SUCCESS; 00023 00024 if (NULLPTR == aes) 00025 { 00026 return PAL_ERR_INVALID_ARGUMENT ; 00027 } 00028 00029 status = pal_plat_initAes (aes); 00030 return status; 00031 } 00032 00033 palStatus_t pal_freeAes(palAesHandle_t *aes) 00034 { 00035 palStatus_t status = PAL_SUCCESS; 00036 00037 if (NULL == aes || (uintptr_t)NULL == *aes) 00038 { 00039 return PAL_ERR_INVALID_ARGUMENT ; 00040 } 00041 00042 status = pal_plat_freeAes (aes); 00043 return status; 00044 } 00045 00046 palStatus_t pal_setAesKey(palAesHandle_t aes, const unsigned char* key, uint32_t keybits, palAesKeyType_t keyTarget) 00047 { 00048 palStatus_t status = PAL_SUCCESS; 00049 00050 if (NULLPTR == aes || NULL == key) 00051 { 00052 return PAL_ERR_INVALID_ARGUMENT ; 00053 } 00054 00055 status = pal_plat_setAesKey (aes, key, keybits, keyTarget); 00056 return status; 00057 } 00058 00059 palStatus_t pal_aesCTR(palAesHandle_t aes, const unsigned char* input, unsigned char* output, size_t inLen, unsigned char iv[16]) 00060 { 00061 palStatus_t status = PAL_SUCCESS; 00062 00063 if (NULLPTR == aes || NULL == input || NULL == output || NULL == iv) 00064 { 00065 return PAL_ERR_INVALID_ARGUMENT ; 00066 } 00067 00068 status = pal_plat_aesCTR (aes, input, output, inLen, iv, false); 00069 return status; 00070 } 00071 00072 palStatus_t pal_aesCTRWithZeroOffset(palAesHandle_t aes, const unsigned char* input, unsigned char* output, size_t inLen, unsigned char iv[16]) 00073 { 00074 palStatus_t status = PAL_SUCCESS; 00075 00076 if (NULLPTR == aes || NULL == input || NULL == output || NULL == iv) 00077 { 00078 return PAL_ERR_INVALID_ARGUMENT ; 00079 } 00080 00081 status = pal_plat_aesCTR (aes, input, output, inLen, iv, true); 00082 return status; 00083 } 00084 00085 palStatus_t pal_aesECB(palAesHandle_t aes, const unsigned char input[PAL_CRYPT_BLOCK_SIZE], unsigned char output[PAL_CRYPT_BLOCK_SIZE], palAesMode_t mode) 00086 { 00087 palStatus_t status = PAL_SUCCESS; 00088 00089 if (NULLPTR == aes || NULL == input || NULL == output) 00090 { 00091 return PAL_ERR_INVALID_ARGUMENT ; 00092 } 00093 00094 status = pal_plat_aesECB (aes, input, output, mode); 00095 return status; 00096 } 00097 00098 palStatus_t pal_sha256 (const unsigned char* input, size_t inLen, unsigned char* output) 00099 { 00100 palStatus_t status = PAL_SUCCESS; 00101 00102 if (NULL == input || NULL == output) 00103 { 00104 return PAL_ERR_INVALID_ARGUMENT ; 00105 } 00106 00107 status = pal_plat_sha256 (input, inLen, output); 00108 return status; 00109 } 00110 00111 palStatus_t pal_x509Initiate(palX509Handle_t* x509Cert) 00112 { 00113 palStatus_t status = PAL_SUCCESS; 00114 00115 if (NULL == x509Cert) 00116 { 00117 return PAL_ERR_INVALID_ARGUMENT ; 00118 } 00119 00120 status = pal_plat_x509Initiate (x509Cert); 00121 return status; 00122 } 00123 00124 palStatus_t pal_x509CertParse(palX509Handle_t x509Cert, const unsigned char* input, size_t inLen) 00125 { 00126 palStatus_t status = PAL_SUCCESS; 00127 00128 if (NULLPTR == x509Cert || NULL == input) 00129 { 00130 return PAL_ERR_INVALID_ARGUMENT ; 00131 } 00132 00133 status = pal_plat_x509CertParse (x509Cert, input, inLen); 00134 return status; 00135 } 00136 00137 palStatus_t pal_x509CertGetAttribute(palX509Handle_t x509Cert, palX509Attr_t attr, void* output, size_t outLenBytes, size_t* actualOutLenBytes) 00138 { 00139 palStatus_t status = PAL_SUCCESS; 00140 00141 if (NULLPTR == x509Cert || NULL == output || NULL == actualOutLenBytes) 00142 { 00143 return PAL_ERR_INVALID_ARGUMENT ; 00144 } 00145 00146 status = pal_plat_x509CertGetAttribute (x509Cert, attr, output, outLenBytes, actualOutLenBytes); 00147 return status; 00148 } 00149 00150 palStatus_t pal_x509CertVerify(palX509Handle_t x509Cert, palX509Handle_t x509CertChain) 00151 { 00152 palStatus_t status = PAL_SUCCESS; 00153 00154 if (NULLPTR == x509Cert) 00155 { 00156 return PAL_ERR_INVALID_ARGUMENT ; 00157 } 00158 00159 status = pal_plat_x509CertVerify (x509Cert, x509CertChain); 00160 return status; 00161 } 00162 00163 palStatus_t pal_x509Free(palX509Handle_t* x509Cert) 00164 { 00165 palStatus_t status = PAL_SUCCESS; 00166 00167 if (NULLPTR == x509Cert || NULLPTR == *x509Cert) 00168 { 00169 return PAL_ERR_INVALID_ARGUMENT ; 00170 } 00171 00172 status = pal_plat_x509Free (x509Cert); 00173 return status; 00174 } 00175 00176 palStatus_t pal_mdInit(palMDHandle_t* md, palMDType_t mdType) 00177 { 00178 palStatus_t status = PAL_SUCCESS; 00179 00180 if (NULLPTR == md) 00181 { 00182 return PAL_ERR_INVALID_ARGUMENT ; 00183 } 00184 00185 status = pal_plat_mdInit (md, mdType); 00186 return status; 00187 } 00188 00189 palStatus_t pal_mdUpdate(palMDHandle_t md, const unsigned char* input, size_t inLen) 00190 { 00191 palStatus_t status = PAL_SUCCESS; 00192 00193 if (NULLPTR == md || NULL == input) 00194 { 00195 return PAL_ERR_INVALID_ARGUMENT ; 00196 } 00197 00198 status = pal_plat_mdUpdate (md, input, inLen); 00199 return status; 00200 } 00201 00202 palStatus_t pal_mdGetOutputSize(palMDHandle_t md, size_t* bufferSize) 00203 { 00204 palStatus_t status = PAL_SUCCESS; 00205 00206 if (NULLPTR == md || NULL == bufferSize) 00207 { 00208 return PAL_ERR_INVALID_ARGUMENT ; 00209 } 00210 00211 status = pal_plat_mdGetOutputSize (md, bufferSize); 00212 return status; 00213 } 00214 00215 palStatus_t pal_mdFinal(palMDHandle_t md, unsigned char* output) 00216 { 00217 palStatus_t status = PAL_SUCCESS; 00218 00219 if (NULLPTR == md || NULL == output) 00220 { 00221 return PAL_ERR_INVALID_ARGUMENT ; 00222 } 00223 00224 status = pal_plat_mdFinal (md, output); 00225 return status; 00226 } 00227 00228 palStatus_t pal_mdFree(palMDHandle_t* md) 00229 { 00230 palStatus_t status = PAL_SUCCESS; 00231 00232 if (NULLPTR == md || NULLPTR == *md) 00233 { 00234 return PAL_ERR_INVALID_ARGUMENT ; 00235 } 00236 00237 status = pal_plat_mdFree (md); 00238 return status; 00239 } 00240 00241 palStatus_t pal_verifySignature(palX509Handle_t x509, palMDType_t mdType, const unsigned char *hash, size_t hashLen, const unsigned char *sig, size_t sigLen) 00242 { 00243 palStatus_t status = PAL_SUCCESS; 00244 00245 if (NULLPTR == x509 || NULL == hash || NULL == sig) 00246 { 00247 return PAL_ERR_INVALID_ARGUMENT ; 00248 } 00249 00250 status = pal_plat_verifySignature (x509, mdType, hash, hashLen, sig, sigLen); 00251 return status; 00252 } 00253 00254 palStatus_t pal_ASN1GetTag(unsigned char **position, const unsigned char *end, size_t *len, uint8_t tag ) 00255 { 00256 palStatus_t status = PAL_SUCCESS; 00257 00258 if (NULL == position || NULL == end || NULL == len) 00259 { 00260 return PAL_ERR_INVALID_ARGUMENT ; 00261 } 00262 00263 status = pal_plat_ASN1GetTag (position, end, len, tag); 00264 return status; 00265 } 00266 00267 palStatus_t pal_CCMInit(palCCMHandle_t* ctx) 00268 { 00269 palStatus_t status = PAL_SUCCESS; 00270 00271 if (NULL == ctx) 00272 { 00273 return PAL_ERR_INVALID_ARGUMENT ; 00274 } 00275 00276 status = pal_plat_CCMInit (ctx); 00277 return status; 00278 } 00279 00280 palStatus_t pal_CCMFree(palCCMHandle_t* ctx) 00281 { 00282 palStatus_t status = PAL_SUCCESS; 00283 00284 if (NULL == ctx || NULLPTR == *ctx) 00285 { 00286 return PAL_ERR_INVALID_ARGUMENT ; 00287 } 00288 00289 status = pal_plat_CCMFree (ctx); 00290 return status; 00291 } 00292 00293 palStatus_t pal_CCMSetKey(palCCMHandle_t ctx, const unsigned char *key, uint32_t keybits, palCipherID_t id) 00294 { 00295 palStatus_t status = PAL_SUCCESS; 00296 00297 if (NULLPTR == ctx || NULL == key) 00298 { 00299 return PAL_ERR_INVALID_ARGUMENT ; 00300 } 00301 00302 status = pal_plat_CCMSetKey (ctx, id, key, keybits); 00303 return status; 00304 } 00305 00306 palStatus_t pal_CCMDecrypt(palCCMHandle_t ctx, unsigned char* input, size_t inLen, 00307 unsigned char* iv, size_t ivLen, unsigned char* add, 00308 size_t addLen, unsigned char* tag, size_t tagLen, 00309 unsigned char* output) 00310 { 00311 palStatus_t status = PAL_SUCCESS; 00312 00313 if (NULLPTR == ctx || NULL == input || NULL == iv || NULL == add || NULL == tag || NULL == output) 00314 { 00315 return PAL_ERR_INVALID_ARGUMENT ; 00316 } 00317 00318 status = pal_plat_CCMDecrypt (ctx, input, inLen, iv, ivLen, add, addLen, tag, tagLen, output); 00319 return status; 00320 } 00321 00322 palStatus_t pal_CCMEncrypt(palCCMHandle_t ctx, unsigned char* input, 00323 size_t inLen, unsigned char* iv, size_t ivLen, 00324 unsigned char* add, size_t addLen, unsigned char* output, 00325 unsigned char* tag, size_t tagLen) 00326 { 00327 palStatus_t status = PAL_SUCCESS; 00328 00329 if (NULLPTR == ctx || NULL == input || NULL == iv || NULL == add || NULL == tag || NULL == output) 00330 { 00331 return PAL_ERR_INVALID_ARGUMENT ; 00332 } 00333 00334 status = pal_plat_CCMEncrypt (ctx, input, inLen, iv, ivLen, add, addLen, output, tag, tagLen); 00335 return status; 00336 } 00337 00338 palStatus_t pal_CtrDRBGInit(palCtrDrbgCtxHandle_t* ctx, const void* seed, size_t len) 00339 { 00340 palStatus_t status = PAL_SUCCESS; 00341 00342 if (NULL == ctx || NULL == seed) 00343 { 00344 return PAL_ERR_INVALID_ARGUMENT ; 00345 } 00346 00347 status = pal_plat_CtrDRBGInit (ctx); 00348 if (PAL_SUCCESS == status) 00349 { 00350 status = pal_plat_CtrDRBGSeed (*ctx, seed, len); 00351 } 00352 00353 return status; 00354 } 00355 00356 palStatus_t pal_CtrDRBGGenerate(palCtrDrbgCtxHandle_t ctx, unsigned char* out, size_t len) 00357 { 00358 palStatus_t status = PAL_SUCCESS; 00359 00360 if (NULLPTR == ctx || NULL == out) 00361 { 00362 return PAL_ERR_INVALID_ARGUMENT ; 00363 } 00364 00365 status = pal_plat_CtrDRBGGenerate (ctx, out, len); 00366 return status; 00367 } 00368 00369 palStatus_t pal_CtrDRBGFree(palCtrDrbgCtxHandle_t* ctx) 00370 { 00371 palStatus_t status = PAL_SUCCESS; 00372 00373 if (NULL == ctx || NULLPTR == *ctx) 00374 { 00375 return PAL_ERR_INVALID_ARGUMENT ; 00376 } 00377 00378 status = pal_plat_CtrDRBGFree (ctx); 00379 return status; 00380 } 00381 00382 palStatus_t pal_cipherCMAC(const unsigned char *key, size_t keyLenInBits, const unsigned char *input, size_t inputLenInBytes, unsigned char *output) 00383 { 00384 palStatus_t status = PAL_SUCCESS; 00385 if (NULL == key || NULL == input || NULL == output) 00386 { 00387 return PAL_ERR_INVALID_ARGUMENT ; 00388 } 00389 00390 status = pal_plat_cipherCMAC (key, keyLenInBits, input, inputLenInBytes, output); 00391 return status; 00392 } 00393 00394 palStatus_t pal_CMACStart(palCMACHandle_t *ctx, const unsigned char *key, size_t keyLenBits, palCipherID_t cipherID) 00395 { 00396 palStatus_t status = PAL_SUCCESS; 00397 00398 if (NULLPTR == ctx || NULL == key) 00399 { 00400 return PAL_ERR_INVALID_ARGUMENT ; 00401 } 00402 00403 status = pal_plat_CMACStart (ctx, key, keyLenBits, cipherID); 00404 return status; 00405 } 00406 00407 palStatus_t pal_CMACUpdate(palCMACHandle_t ctx, const unsigned char *input, size_t inLen) 00408 { 00409 palStatus_t status = PAL_SUCCESS; 00410 00411 if (NULLPTR == ctx || NULL == input) 00412 { 00413 return PAL_ERR_INVALID_ARGUMENT ; 00414 } 00415 00416 status = pal_plat_CMACUpdate (ctx, input, inLen); 00417 return status; 00418 } 00419 00420 palStatus_t pal_CMACFinish(palCMACHandle_t *ctx, unsigned char *output, size_t* outLen) 00421 { 00422 palStatus_t status = PAL_SUCCESS; 00423 00424 if (NULLPTR == ctx || NULLPTR == *ctx || NULL == output || NULL == outLen) 00425 { 00426 return PAL_ERR_INVALID_ARGUMENT ; 00427 } 00428 00429 status = pal_plat_CMACFinish (ctx, output, outLen); 00430 return status; 00431 } 00432 00433 palStatus_t pal_mdHmacSha256(const unsigned char *key, size_t keyLenInBytes, const unsigned char *input, size_t inputLenInBytes, unsigned char *output, size_t* outputLenInBytes) 00434 { 00435 palStatus_t status = PAL_SUCCESS; 00436 00437 if (NULL == key || NULL == input || NULL == output) 00438 { 00439 return PAL_ERR_INVALID_ARGUMENT ; 00440 } 00441 00442 status = pal_plat_mdHmacSha256 (key, keyLenInBytes, input, inputLenInBytes, output, outputLenInBytes); 00443 return status; 00444 } 00445 00446 palStatus_t pal_ECCheckKey(palCurveHandle_t grp, palECKeyHandle_t key, uint32_t type, bool *verified) 00447 { 00448 palStatus_t status = PAL_SUCCESS; 00449 00450 if (NULLPTR == grp || NULLPTR == key || NULL == verified) 00451 { 00452 return PAL_ERR_INVALID_ARGUMENT ; 00453 } 00454 00455 status = pal_plat_ECCheckKey (grp, key, type, verified); 00456 return status; 00457 } 00458 00459 palStatus_t pal_ECKeyNew(palECKeyHandle_t* key) 00460 { 00461 palStatus_t status = PAL_SUCCESS; 00462 00463 if (NULL == key) 00464 { 00465 return PAL_ERR_INVALID_ARGUMENT ; 00466 } 00467 00468 status = pal_plat_ECKeyNew (key); 00469 return status; 00470 } 00471 00472 palStatus_t pal_ECKeyFree(palECKeyHandle_t* key) 00473 { 00474 palStatus_t status = PAL_SUCCESS; 00475 00476 if (NULL == key || NULLPTR == *key) 00477 { 00478 return PAL_ERR_INVALID_ARGUMENT ; 00479 } 00480 00481 status = pal_plat_ECKeyFree (key); 00482 return status; 00483 } 00484 00485 palStatus_t pal_parseECPrivateKeyFromDER(const unsigned char* prvDERKey, size_t keyLen, palECKeyHandle_t key) 00486 { 00487 palStatus_t status = PAL_SUCCESS; 00488 00489 if (NULL == prvDERKey || NULLPTR == key) 00490 { 00491 return PAL_ERR_INVALID_ARGUMENT ; 00492 } 00493 00494 status = pal_plat_parseECPrivateKeyFromDER (prvDERKey, keyLen, key); 00495 return status; 00496 } 00497 00498 palStatus_t pal_parseECPublicKeyFromDER(const unsigned char* pubDERKey, size_t keyLen, palECKeyHandle_t key) 00499 { 00500 palStatus_t status = PAL_SUCCESS; 00501 00502 if (NULL == pubDERKey || NULLPTR == key) 00503 { 00504 return PAL_ERR_INVALID_ARGUMENT ; 00505 } 00506 00507 status = pal_plat_parseECPublicKeyFromDER (pubDERKey, keyLen, key); 00508 return status; 00509 } 00510 00511 palStatus_t pal_writePrivateKeyToDer(palECKeyHandle_t key, unsigned char* derBuffer, size_t bufferSize, size_t* actualSize) 00512 { 00513 palStatus_t status = PAL_SUCCESS; 00514 00515 if (NULLPTR == key || NULL == derBuffer || NULL == actualSize) 00516 { 00517 return PAL_ERR_INVALID_ARGUMENT ; 00518 } 00519 00520 status = pal_plat_writePrivateKeyToDer (key, derBuffer, bufferSize, actualSize); 00521 return status; 00522 } 00523 00524 palStatus_t pal_writePublicKeyToDer(palECKeyHandle_t key, unsigned char* derBuffer, size_t bufferSize, size_t* actualSize) 00525 { 00526 palStatus_t status = PAL_SUCCESS; 00527 00528 if (NULLPTR == key || NULL == derBuffer || NULL == actualSize) 00529 { 00530 return PAL_ERR_INVALID_ARGUMENT ; 00531 } 00532 00533 status = pal_plat_writePublicKeyToDer (key, derBuffer, bufferSize, actualSize); 00534 return status; 00535 } 00536 palStatus_t pal_ECGroupInitAndLoad(palCurveHandle_t* grp, palGroupIndex_t index) 00537 { 00538 palStatus_t status = PAL_SUCCESS; 00539 00540 if (NULLPTR == grp) 00541 { 00542 return PAL_ERR_INVALID_ARGUMENT ; 00543 } 00544 00545 status = pal_plat_ECGroupInitAndLoad (grp, index); 00546 return status; 00547 } 00548 00549 palStatus_t pal_ECGroupFree(palCurveHandle_t* grp) 00550 { 00551 palStatus_t status = PAL_SUCCESS; 00552 00553 if (NULL == grp || NULLPTR == *grp) 00554 { 00555 return PAL_ERR_INVALID_ARGUMENT ; 00556 } 00557 00558 status = pal_plat_ECGroupFree (grp); 00559 return status; 00560 } 00561 00562 palStatus_t pal_ECKeyGenerateKey(palGroupIndex_t grpID, palECKeyHandle_t key) 00563 { 00564 palStatus_t status = PAL_SUCCESS; 00565 00566 if (NULLPTR == key) 00567 { 00568 return PAL_ERR_INVALID_ARGUMENT ; 00569 } 00570 00571 status = pal_plat_ECKeyGenerateKey (grpID, key); 00572 return status; 00573 } 00574 00575 palStatus_t pal_ECKeyGetCurve(palECKeyHandle_t key, palGroupIndex_t* grpID) 00576 { 00577 palStatus_t status = PAL_SUCCESS; 00578 00579 if (NULLPTR == key || NULL == grpID) 00580 { 00581 return PAL_ERR_INVALID_ARGUMENT ; 00582 } 00583 00584 status = pal_plat_ECKeyGetCurve (key, grpID); 00585 return status; 00586 } 00587 00588 palStatus_t pal_x509CSRInit(palx509CSRHandle_t *x509CSR) 00589 { 00590 palStatus_t status = PAL_SUCCESS; 00591 00592 if (NULL == x509CSR) 00593 { 00594 return PAL_ERR_INVALID_ARGUMENT ; 00595 } 00596 00597 status = pal_plat_x509CSRInit (x509CSR); 00598 return status; 00599 } 00600 00601 palStatus_t pal_x509CSRSetSubject(palx509CSRHandle_t x509CSR, const char* subjectName) 00602 { 00603 palStatus_t status = PAL_SUCCESS; 00604 00605 if (NULLPTR == x509CSR || NULL == subjectName) 00606 { 00607 return PAL_ERR_INVALID_ARGUMENT ; 00608 } 00609 00610 status = pal_plat_x509CSRSetSubject (x509CSR, subjectName); 00611 return status; 00612 } 00613 00614 palStatus_t pal_x509CSRSetKey(palx509CSRHandle_t x509CSR, palECKeyHandle_t pubKey, palECKeyHandle_t prvKey) 00615 { 00616 palStatus_t status = PAL_SUCCESS; 00617 00618 if (NULLPTR == x509CSR || NULLPTR == pubKey) 00619 { 00620 return PAL_ERR_INVALID_ARGUMENT ; 00621 } 00622 00623 status = pal_plat_x509CSRSetKey (x509CSR, pubKey, prvKey); 00624 return status; 00625 } 00626 00627 palStatus_t pal_x509CSRSetMD(palx509CSRHandle_t x509CSR, palMDType_t mdType) 00628 { 00629 palStatus_t status = PAL_SUCCESS; 00630 00631 if (NULLPTR == x509CSR) 00632 { 00633 return PAL_ERR_INVALID_ARGUMENT ; 00634 } 00635 00636 status = pal_plat_x509CSRSetMD (x509CSR, mdType); 00637 return status; 00638 } 00639 00640 palStatus_t pal_x509CSRSetKeyUsage(palx509CSRHandle_t x509CSR, uint32_t keyUsage) 00641 { 00642 palStatus_t status = PAL_SUCCESS; 00643 00644 if (NULLPTR == x509CSR) 00645 { 00646 return PAL_ERR_INVALID_ARGUMENT ; 00647 } 00648 00649 status = pal_plat_x509CSRSetKeyUsage (x509CSR, keyUsage); 00650 return status; 00651 } 00652 00653 palStatus_t pal_x509CSRSetExtension(palx509CSRHandle_t x509CSR,const char* oid, size_t oidLen, const unsigned char* value, size_t valueLen) 00654 { 00655 palStatus_t status = PAL_SUCCESS; 00656 00657 if (NULLPTR == x509CSR || NULL == oid || NULL == value) 00658 { 00659 return PAL_ERR_INVALID_ARGUMENT ; 00660 } 00661 00662 status = pal_plat_x509CSRSetExtension (x509CSR, oid, oidLen, value, valueLen); 00663 return status; 00664 } 00665 00666 palStatus_t pal_x509CSRWriteDER(palx509CSRHandle_t x509CSR, unsigned char* derBuf, size_t derBufLen, size_t* actualDerLen) 00667 { 00668 palStatus_t status = PAL_SUCCESS; 00669 00670 if (NULLPTR == x509CSR || NULL == derBuf) 00671 { 00672 return PAL_ERR_INVALID_ARGUMENT ; 00673 } 00674 00675 status = pal_plat_x509CSRWriteDER (x509CSR, derBuf, derBufLen, actualDerLen); 00676 return status; 00677 } 00678 00679 palStatus_t pal_x509CSRFree(palx509CSRHandle_t *x509CSR) 00680 { 00681 palStatus_t status = PAL_SUCCESS; 00682 00683 if (NULL == x509CSR || NULLPTR == *x509CSR) 00684 { 00685 return PAL_ERR_INVALID_ARGUMENT ; 00686 } 00687 00688 status = pal_plat_x509CSRFree (x509CSR); 00689 return status; 00690 } 00691 00692 palStatus_t pal_ECDHComputeKey(const palCurveHandle_t grp, const palECKeyHandle_t peerPublicKey, 00693 const palECKeyHandle_t privateKey, palECKeyHandle_t outKey) 00694 { 00695 palStatus_t status = PAL_SUCCESS; 00696 00697 if (NULLPTR == grp || NULLPTR == peerPublicKey || NULLPTR == privateKey || NULLPTR == outKey) 00698 { 00699 return PAL_ERR_INVALID_ARGUMENT ; 00700 } 00701 00702 status = pal_plat_ECDHComputeKey (grp, peerPublicKey, privateKey, outKey); 00703 return status; 00704 } 00705 00706 palStatus_t pal_ECDSASign(palCurveHandle_t grp, palMDType_t mdType, palECKeyHandle_t prvKey, unsigned char* dgst, 00707 uint32_t dgstLen, unsigned char *sig, size_t *sigLen) 00708 { 00709 palStatus_t status = PAL_SUCCESS; 00710 00711 if (NULLPTR == grp || NULLPTR == prvKey || NULL == dgst || NULL == sig || NULL == sigLen) 00712 { 00713 return PAL_ERR_INVALID_ARGUMENT ; 00714 } 00715 00716 status = pal_plat_ECDSASign (grp, mdType, prvKey, dgst, dgstLen, sig, sigLen); 00717 return status; 00718 } 00719 00720 palStatus_t pal_ECDSAVerify(palECKeyHandle_t pubKey, unsigned char* dgst, uint32_t dgstLen, 00721 unsigned char* sig, size_t sigLen, bool* verified) 00722 { 00723 palStatus_t status = PAL_SUCCESS; 00724 00725 if (NULLPTR == pubKey || NULL == dgst || NULL == sig || NULL == verified) 00726 { 00727 return PAL_ERR_INVALID_ARGUMENT ; 00728 } 00729 00730 status = pal_plat_ECDSAVerify (pubKey, dgst, dgstLen, sig, sigLen, verified); 00731 return status; 00732 } 00733
Generated on Tue Jul 12 2022 16:22:08 by
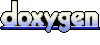