
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
m2minterfaceimpl.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_INTERFACE_IMPL_H 00017 #define M2M_INTERFACE_IMPL_H 00018 00019 #include "mbed-client/m2minterface.h" 00020 #include "mbed-client/m2mserver.h" 00021 #include "mbed-client/m2mconnectionobserver.h" 00022 #include "mbed-client/m2mconnectionsecurity.h" 00023 #include "include/m2mnsdlobserver.h" 00024 #include "include/m2mnsdlinterface.h" 00025 #include "mbed-client/m2mtimerobserver.h" 00026 #include "mbed-client/m2mtimer.h" 00027 #include "mbed-client/m2mconnectionhandler.h" 00028 #include "mbed-client/m2mconstants.h" 00029 00030 //FORWARD DECLARATION 00031 class M2MConnectionSecurity; 00032 class EventData; 00033 class M2MUpdateRegisterData; 00034 /** 00035 * @brief M2MInterfaceImpl. 00036 * This class implements handling of all mbed Client Interface operations 00037 * defined in OMA LWM2M specifications. 00038 * This includes Bootstrapping, Client Registration, Device Management & 00039 * Service Enablement and Information Reporting. 00040 */ 00041 00042 class M2MInterfaceImpl : public M2MInterface, 00043 public M2MNsdlObserver, 00044 public M2MConnectionObserver, 00045 public M2MTimerObserver 00046 { 00047 private: 00048 // Prevents the use of assignment operator by accident. 00049 M2MInterfaceImpl& operator=( const M2MInterfaceImpl& /*other*/ ); 00050 00051 // Prevents the use of copy constructor by accident 00052 M2MInterfaceImpl( const M2MInterfaceImpl& /*other*/ ); 00053 00054 friend class M2MInterfaceFactory; 00055 00056 private: 00057 00058 /** 00059 * @brief Constructor 00060 * @param observer, Observer to pass the event callbacks for various 00061 * interface operations. 00062 * @param endpoint_name Endpoint name of the client. 00063 * @param endpoint_type Endpoint type of the client. 00064 * @param life_time Life time of the client in seconds 00065 * @param listen_port Listening port for the endpoint, default is 8000. 00066 * @param domain Domain of the client. 00067 * @param mode Binding mode of the client, default is UDP 00068 * @param stack Network stack to be used for connection, default is LwIP_IPv4. 00069 * @param context_address Context address, default is empty. 00070 */ 00071 M2MInterfaceImpl(M2MInterfaceObserver& observer, 00072 const String &endpoint_name, 00073 const String &endpoint_type, 00074 const int32_t life_time, 00075 const uint16_t listen_port, 00076 const String &domain = "", 00077 BindingMode mode = M2MInterface::NOT_SET, 00078 M2MInterface::NetworkStack stack = M2MInterface::LwIP_IPv4, 00079 const String &context_address = ""); 00080 00081 public: 00082 00083 /** 00084 * @brief Destructor 00085 */ 00086 virtual ~M2MInterfaceImpl(); 00087 00088 /** 00089 * @brief Initiates bootstrapping of the client with the provided Bootstrap 00090 * server information. 00091 * @param security_object Security object which contains information 00092 * required for successful bootstrapping of the client. 00093 */ 00094 virtual void bootstrap(M2MSecurity *security); 00095 00096 /** 00097 * @brief Cancels on going bootstrapping operation of the client. If the client has 00098 * already successfully bootstrapped then this function deletes existing 00099 * bootstrap information from the client. 00100 */ 00101 virtual void cancel_bootstrap(); 00102 00103 /** 00104 * @brief Initiates registration of the provided Security object to the 00105 * corresponding LWM2M server. 00106 * @param security_object Security object which contains information 00107 * required for registering to the LWM2M server. 00108 * If client wants to register to multiple LWM2M servers then it has call 00109 * this function once for each of LWM2M server object separately. 00110 * @param object_list Objects which contains information 00111 * which the client want to register to the LWM2M server. 00112 */ 00113 virtual void register_object(M2MSecurity *security_object, const M2MObjectList &object_list); 00114 00115 /** 00116 * @brief Updates or refreshes the client's registration on the LWM2M 00117 * server. 00118 * @param security_object Security object from which the device object 00119 * needs to update registration, if there is only one LWM2M server registered 00120 * then this parameter can be NULL. 00121 * @param lifetime Lifetime for the endpoint client in seconds. 00122 */ 00123 virtual void update_registration(M2MSecurity *security_object, const uint32_t lifetime = 0); 00124 00125 /** 00126 * @brief Updates or refreshes the client's registration on the LWM2M 00127 * server. Use this function to publish new objects to LWM2M server. 00128 * @param security_object The security object from which the device object 00129 * needs to update the registration. If there is only one LWM2M server registered, 00130 * this parameter can be NULL. 00131 * @param object_list Objects that contain information about the 00132 * client attempting to register to the LWM2M server. 00133 * @param lifetime The lifetime of the endpoint client in seconds. If the same value 00134 * has to be passed, set the default value to 0. 00135 */ 00136 virtual void update_registration(M2MSecurity *security_object, const M2MObjectList &object_list, 00137 const uint32_t lifetime = 0); 00138 00139 /** 00140 * @brief Unregisters the registered object from the LWM2M server 00141 * @param security_object Security object from which the device object 00142 * needs to be unregistered. If there is only one LWM2M server registered 00143 * this parameter can be NULL. 00144 */ 00145 virtual void unregister_object(M2MSecurity* security = NULL); 00146 00147 /** 00148 * @brief Sets the function which will be called indicating client 00149 * is going to sleep when the Binding mode is selected with Queue mode. 00150 * @param callback A function pointer that will be called when client 00151 * goes to sleep. 00152 */ 00153 virtual void set_queue_sleep_handler(callback_handler handler); 00154 00155 /** 00156 * @brief Sets the network interface handler that is used by client to connect 00157 * to a network over IP. 00158 * @param handler A network interface handler that is used by client to connect. 00159 * This API is optional but provides a mechanism for different platforms to 00160 * manage usage of underlying network interface by client. 00161 */ 00162 virtual void set_platform_network_handler(void *handler = NULL); 00163 00164 /** 00165 * \brief Sets the function callback that will be called by mbed-client for 00166 * fetching random number from application for ensuring strong entropy. 00167 * \param random_callback A function pointer that will be called by mbed-client 00168 * while performing secure handshake. 00169 * Function signature should be uint32_t (*random_number_callback)(void); 00170 */ 00171 virtual void set_random_number_callback(random_number_cb callback); 00172 00173 /** 00174 * \brief Sets the function callback that will be called by mbed-client for 00175 * providing entropy source from application for ensuring strong entropy. 00176 * \param entropy_callback A function pointer that will be called by mbed-client 00177 * while performing secure handshake. 00178 * Function signature , if using mbed-client-mbedtls should be 00179 * int (*mbedtls_entropy_f_source_ptr)(void *data, unsigned char *output, 00180 * size_t len, size_t *olen); 00181 */ 00182 virtual void set_entropy_callback(entropy_cb callback); 00183 00184 /** 00185 * @brief Updates the endpoint name. 00186 * @param name New endpoint name 00187 */ 00188 virtual void update_endpoint(String &name); 00189 00190 /** 00191 * @brief Updates the domain name. 00192 * @param domain New domain name 00193 */ 00194 virtual void update_domain(String &domain); 00195 00196 /** 00197 * @brief Return internal endpoint name 00198 * @return internal endpoint name 00199 */ 00200 virtual const String internal_endpoint_name() const; 00201 00202 /** 00203 * @brief Return error description for the latest error code 00204 * @return Error description string 00205 */ 00206 virtual const char *error_description() const; 00207 00208 /** 00209 * @brief Sends the CoAP GET request to the server. 00210 * @uri Uri path to the data. 00211 * @offset Data offset. 00212 * @async In async mode application must call this API again with the updated offset. 00213 * If set to false then client will automatically download the whole package. 00214 * @get_data_cb Callback which is triggered once there is data available. 00215 * @get_data_error_cb Callback which is trigged in case of any error. 00216 */ 00217 virtual void get_data_request(const char *uri, 00218 const size_t offset, 00219 const bool async, 00220 get_data_cb data_cb, 00221 get_data_error_cb error_cb, 00222 void *context); 00223 00224 protected: // From M2MNsdlObserver 00225 00226 virtual void coap_message_ready(uint8_t *data_ptr, 00227 uint16_t data_len, 00228 sn_nsdl_addr_s *address_ptr); 00229 00230 virtual void client_registered(M2MServer *server_object); 00231 00232 virtual void registration_updated(const M2MServer &server_object); 00233 00234 virtual void registration_error(uint8_t error_code, bool retry = false); 00235 00236 virtual void client_unregistered(); 00237 00238 virtual void bootstrap_done(); 00239 00240 virtual void bootstrap_wait(); 00241 00242 virtual void bootstrap_error_wait(const char *reason); 00243 00244 virtual void bootstrap_error(const char *reason); 00245 00246 virtual void coap_data_processed(); 00247 00248 virtual void value_updated(M2MBase *base); 00249 00250 protected: // From M2MConnectionObserver 00251 00252 virtual void data_available(uint8_t* data, 00253 uint16_t data_size, 00254 const M2MConnectionObserver::SocketAddress &address); 00255 00256 virtual void socket_error(uint8_t error_code, bool retry = true); 00257 00258 virtual void address_ready(const M2MConnectionObserver::SocketAddress &address, 00259 M2MConnectionObserver::ServerType server_type, 00260 const uint16_t server_port); 00261 00262 virtual void data_sent(); 00263 00264 protected: // from M2MTimerObserver 00265 00266 virtual void timer_expired(M2MTimerObserver::Type type); 00267 00268 00269 private: // state machine state functions 00270 00271 /** 00272 * When the state is Idle. 00273 */ 00274 void state_idle(EventData* data); 00275 00276 /** 00277 * When the client starts bootstrap. 00278 */ 00279 void state_bootstrap( EventData *data); 00280 00281 /** 00282 * When the bootstrap server address is resolved. 00283 */ 00284 void state_bootstrap_address_resolved( EventData *data); 00285 00286 /** 00287 * When the bootstrap resource is created. 00288 */ 00289 void state_bootstrap_resource_created( EventData *data); 00290 00291 /** 00292 * When the server has sent response and bootstrapping is done. 00293 */ 00294 void state_bootstrapped( EventData *data); 00295 00296 /** 00297 * When the client starts register. 00298 */ 00299 void state_register( EventData *data); 00300 00301 /** 00302 * When the server address for register is resolved. 00303 */ 00304 void state_register_address_resolved( EventData *data); 00305 00306 /** 00307 * When the client is registered. 00308 */ 00309 void state_registered( EventData *data); 00310 00311 /** 00312 * When the client is updating registration. 00313 */ 00314 void state_update_registration( EventData *data); 00315 00316 /** 00317 * When the client starts unregister. 00318 */ 00319 void state_unregister( EventData *data); 00320 00321 /** 00322 * When the client has been unregistered. 00323 */ 00324 void state_unregistered( EventData *data); 00325 00326 /** 00327 * When the coap data is been sent through socket. 00328 */ 00329 void state_sending_coap_data( EventData *data); 00330 00331 /** 00332 * When the coap data is sent successfully. 00333 */ 00334 void state_coap_data_sent( EventData *data); 00335 00336 /** 00337 * When the socket is receiving coap data. 00338 */ 00339 void state_receiving_coap_data( EventData *data); 00340 00341 /** 00342 * When the socket has received coap data. 00343 */ 00344 void state_coap_data_received( EventData *data); 00345 00346 /** 00347 * When the coap message is being processed. 00348 */ 00349 void state_processing_coap_data( EventData *data); 00350 00351 /** 00352 * When the coap message has been processed. 00353 */ 00354 void state_coap_data_processed( EventData *data); 00355 00356 /** 00357 * When the client is waiting to receive or send data. 00358 */ 00359 void state_waiting( EventData *data); 00360 00361 /** 00362 * Start registration update. 00363 */ 00364 void start_register_update(M2MUpdateRegisterData *data); 00365 00366 /** 00367 * State enumeration order must match the order of state 00368 * method entries in the state map 00369 */ 00370 enum E_States { 00371 STATE_IDLE = 0, 00372 STATE_BOOTSTRAP, 00373 STATE_BOOTSTRAP_ADDRESS_RESOLVED, 00374 STATE_BOOTSTRAP_RESOURCE_CREATED, 00375 STATE_BOOTSTRAP_WAIT, 00376 STATE_BOOTSTRAP_ERROR_WAIT, // 5 00377 STATE_BOOTSTRAPPED, 00378 STATE_REGISTER, 00379 STATE_REGISTER_ADDRESS_RESOLVED, 00380 STATE_REGISTERED, 00381 STATE_UPDATE_REGISTRATION, // 10 00382 STATE_UNREGISTER, 00383 STATE_UNREGISTERED, 00384 STATE_SENDING_COAP_DATA, 00385 STATE_COAP_DATA_SENT, 00386 STATE_COAP_DATA_RECEIVED, // 15 00387 STATE_PROCESSING_COAP_DATA, 00388 STATE_COAP_DATA_PROCESSED, 00389 STATE_WAITING, 00390 STATE_MAX_STATES 00391 }; 00392 00393 /** 00394 * @brief Redirects the state machine to right function. 00395 * @param current_state Current state to be set. 00396 * @param data Data to be passed to the state function. 00397 */ 00398 void state_function( uint8_t current_state, EventData* data ); 00399 00400 /** 00401 * @brief State Engine maintaining state machine logic. 00402 */ 00403 void state_engine(void); 00404 00405 /** 00406 * External event which can trigger the state machine. 00407 * @param New The state to which the state machine should go. 00408 * @param data The data to be passed to the state machine. 00409 */ 00410 void external_event(uint8_t, EventData* = NULL); 00411 00412 /** 00413 * Internal event generated by state machine. 00414 * @param New State which the state machine should go to. 00415 * @param data The data to be passed to the state machine. 00416 */ 00417 void internal_event(uint8_t, EventData* = NULL); 00418 00419 /** 00420 * Queue mode enabled or not. 00421 * @return True if queue mode otherwise false. 00422 */ 00423 bool queue_mode() const; 00424 00425 enum 00426 { 00427 EVENT_IGNORED = 0xFE, 00428 CANNOT_HAPPEN 00429 }; 00430 00431 /** 00432 * Helper method for extracting the IP address part and port from the 00433 * given server address. 00434 * @param server_address Source URL (without "coap" or "coaps" prefix). 00435 * @param ip_address The extracted IP. 00436 * @param port The extracted port. 00437 */ 00438 static void process_address(const String& server_address, String& ip_address, uint16_t& port); 00439 00440 /** 00441 * Helper method for storing the error description to _error_description if the feature 00442 * has not been turned off. 00443 * @param error description 00444 */ 00445 void set_error_description(const char *description); 00446 00447 enum ReconnectionState{ 00448 None, 00449 WithUpdate, 00450 FullRegistration, 00451 Unregistration 00452 }; 00453 00454 private: 00455 00456 EventData *_event_data; 00457 M2MTimer *_registration_flow_timer; 00458 uint16_t _server_port; 00459 uint16_t _listen_port; 00460 int32_t _life_time; 00461 String _server_ip_address; 00462 M2MSecurity *_register_server; //TODO: to be the list not owned 00463 M2MTimer _queue_sleep_timer; 00464 M2MTimer _retry_timer; 00465 callback_handler _callback_handler; 00466 const uint8_t _max_states; 00467 bool _event_ignored; 00468 bool _event_generated; 00469 bool _reconnecting; 00470 bool _retry_timer_expired; 00471 bool _bootstrapped; 00472 bool _queue_mode_timer_ongoing; 00473 uint8_t _current_state; 00474 BindingMode _binding_mode; 00475 ReconnectionState _reconnection_state; 00476 M2MInterfaceObserver &_observer; 00477 M2MConnectionSecurity *_security_connection; // Doesn't own 00478 M2MConnectionHandler _connection_handler; 00479 M2MNsdlInterface _nsdl_interface; 00480 M2MSecurity *_security; 00481 00482 #ifndef DISABLE_ERROR_DESCRIPTION 00483 // The DISABLE_ERROR_DESCRIPTION macro will reduce the flash usage by ~1800 bytes. 00484 char _error_description[MAX_ALLOWED_ERROR_STRING_LENGTH]; 00485 #endif 00486 00487 uint8_t _initial_reconnection_time; 00488 uint64_t _reconnection_time; 00489 00490 friend class Test_M2MInterfaceImpl; 00491 00492 }; 00493 00494 #define BEGIN_TRANSITION_MAP \ 00495 static const uint8_t TRANSITIONS[] = {\ 00496 00497 #define TRANSITION_MAP_ENTRY(entry)\ 00498 entry, 00499 00500 #define END_TRANSITION_MAP(data) \ 00501 0 };\ 00502 external_event(TRANSITIONS[_current_state], data); 00503 00504 #endif //M2M_INTERFACE_IMPL_H 00505 00506
Generated on Tue Jul 12 2022 16:22:06 by
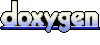