
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
lwm2m-monitor.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "update-client-lwm2m/lwm2m-monitor.h" 00020 #include "update-client-lwm2m/FirmwareUpdateResource.h" 00021 #include "update-client-lwm2m/DeviceMetadataResource.h" 00022 00023 /** 00024 * @brief Get driver version. 00025 * @return Driver version. 00026 */ 00027 uint32_t ARM_UCS_LWM2M_MONITOR_GetVersion(void) 00028 { 00029 return 0; 00030 } 00031 00032 /** 00033 * @brief Get Source capabilities. 00034 * @return Struct containing capabilites. See definition above. 00035 */ 00036 ARM_MONITOR_CAPABILITIES ARM_UCS_LWM2M_MONITOR_GetCapabilities(void) 00037 { 00038 ARM_MONITOR_CAPABILITIES result = { .state = 1, 00039 .result = 1, 00040 .version = 1 }; 00041 00042 return result; 00043 } 00044 00045 /** 00046 * @brief Initialize Monitor. 00047 * @return Error code. 00048 */ 00049 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_Initialize(void (*notification_handler)(void)) 00050 { 00051 arm_uc_error_t retval = { .code = SRCE_ERR_NONE }; 00052 00053 FirmwareUpdateResource::Initialize(); 00054 FirmwareUpdateResource::addNotificationCallback(notification_handler); 00055 00056 DeviceMetadataResource::Initialize(); 00057 00058 return retval; 00059 } 00060 00061 /** 00062 * @brief Uninitialized Monitor. 00063 * @return Error code. 00064 */ 00065 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_Uninitialize(void) 00066 { 00067 arm_uc_error_t retval = { .code = SRCE_ERR_NONE }; 00068 00069 return retval; 00070 } 00071 00072 /** 00073 * @brief Send Update Client state. 00074 * @details From the OMA LWM2M Technical Specification: 00075 * 00076 * Indicates current state with respect to this firmware update. 00077 * This value is set by the LWM2M Client. 00078 * 0: Idle (before downloading or after successful updating) 00079 * 1: Downloading (The data sequence is on the way) 00080 * 2: Downloaded 00081 * 3: Updating 00082 * 00083 * If writing the firmware package to Package Resource is done, 00084 * or, if the device has downloaded the firmware package from the 00085 * Package URI the state changes to Downloaded. 00086 * 00087 * If writing an empty string to Package Resource is done or 00088 * writing an empty string to Package URI is done, the state 00089 * changes to Idle. 00090 * 00091 * When in Downloaded state, and the executable Resource Update is 00092 * triggered, the state changes to Updating. 00093 * If the Update Resource failed, the state returns at Downloaded. 00094 * If performing the Update Resource was successful, the state 00095 * changes from Updating to Idle. 00096 * 00097 * @param state Valid states: ARM_UC_MONITOR_STATE_IDLE 00098 * ARM_UC_MONITOR_STATE_DOWNLOADING 00099 * ARM_UC_MONITOR_STATE_DOWNLOADED 00100 * ARM_UC_MONITOR_STATE_UPDATING 00101 * 00102 * @return Error code. 00103 */ 00104 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_SendState(arm_uc_monitor_state_t state) 00105 { 00106 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00107 00108 int32_t retval = -1; 00109 00110 switch (state) 00111 { 00112 case ARM_UC_MONITOR_STATE_IDLE: 00113 retval = FirmwareUpdateResource::sendState( 00114 FirmwareUpdateResource::ARM_UCS_LWM2M_STATE_IDLE); 00115 break; 00116 case ARM_UC_MONITOR_STATE_DOWNLOADING: 00117 retval = FirmwareUpdateResource::sendState( 00118 FirmwareUpdateResource::ARM_UCS_LWM2M_STATE_DOWNLOADING); 00119 break; 00120 case ARM_UC_MONITOR_STATE_DOWNLOADED: 00121 retval = FirmwareUpdateResource::sendState( 00122 FirmwareUpdateResource::ARM_UCS_LWM2M_STATE_DOWNLOADED); 00123 break; 00124 case ARM_UC_MONITOR_STATE_UPDATING: 00125 retval = FirmwareUpdateResource::sendState( 00126 FirmwareUpdateResource::ARM_UCS_LWM2M_STATE_UPDATING); 00127 break; 00128 default: 00129 break; 00130 } 00131 00132 if (retval == 0) 00133 { 00134 result.code = ERR_NONE; 00135 } 00136 00137 return result; 00138 } 00139 00140 /** 00141 * @brief Send update result. 00142 * @details From the OMA LWM2M Technical Specification: 00143 * 00144 * Contains the result of downloading or updating the firmware 00145 * 0: Initial value. Once the updating process is initiated 00146 * (Download /Update), this Resource MUST be reset to Initial 00147 * value. 00148 * 1: Firmware updated successfully, 00149 * 2: Not enough storage for the new firmware package. 00150 * 3. Out of memory during downloading process. 00151 * 4: Connection lost during downloading process. 00152 * 5: CRC check failure for new downloaded package. 00153 * 6: Unsupported package type. 00154 * 7: Invalid URI 00155 * 8: Firmware update failed 00156 * 00157 * This Resource MAY be reported by sending Observe operation. 00158 * 00159 * @param result Valid results: ARM_UC_MONITOR_RESULT_INITIAL 00160 * ARM_UC_MONITOR_RESULT_SUCCESS 00161 * ARM_UC_MONITOR_RESULT_ERROR_STORAGE 00162 * ARM_UC_MONITOR_RESULT_ERROR_MEMORY 00163 * ARM_UC_MONITOR_RESULT_ERROR_CONNECTION 00164 * ARM_UC_MONITOR_RESULT_ERROR_CRC 00165 * ARM_UC_MONITOR_RESULT_ERROR_TYPE 00166 * ARM_UC_MONITOR_RESULT_ERROR_URI 00167 * ARM_UC_MONITOR_RESULT_ERROR_UPDATE 00168 * 00169 * @return Error code. 00170 */ 00171 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_SendUpdateResult(arm_uc_monitor_result_t updateResult) 00172 { 00173 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00174 00175 int32_t retval = -1; 00176 00177 switch (updateResult) 00178 { 00179 case ARM_UC_MONITOR_RESULT_INITIAL: 00180 retval = FirmwareUpdateResource::sendUpdateResult( 00181 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_INITIAL); 00182 break; 00183 case ARM_UC_MONITOR_RESULT_SUCCESS: 00184 retval = FirmwareUpdateResource::sendUpdateResult( 00185 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_SUCCESS); 00186 break; 00187 case ARM_UC_MONITOR_RESULT_ERROR_STORAGE: 00188 retval = FirmwareUpdateResource::sendUpdateResult( 00189 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_STORAGE); 00190 break; 00191 case ARM_UC_MONITOR_RESULT_ERROR_MEMORY: 00192 retval = FirmwareUpdateResource::sendUpdateResult( 00193 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_MEMORY); 00194 break; 00195 case ARM_UC_MONITOR_RESULT_ERROR_CONNECTION: 00196 retval = FirmwareUpdateResource::sendUpdateResult( 00197 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_CONNECTION); 00198 break; 00199 case ARM_UC_MONITOR_RESULT_ERROR_CRC: 00200 retval = FirmwareUpdateResource::sendUpdateResult( 00201 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_CRC); 00202 break; 00203 case ARM_UC_MONITOR_RESULT_ERROR_TYPE: 00204 retval = FirmwareUpdateResource::sendUpdateResult( 00205 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_TYPE); 00206 break; 00207 case ARM_UC_MONITOR_RESULT_ERROR_URI: 00208 retval = FirmwareUpdateResource::sendUpdateResult( 00209 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_URI); 00210 break; 00211 case ARM_UC_MONITOR_RESULT_ERROR_UPDATE: 00212 retval = FirmwareUpdateResource::sendUpdateResult( 00213 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_UPDATE); 00214 break; 00215 case ARM_UC_MONITOR_RESULT_ERROR_HASH: 00216 retval = FirmwareUpdateResource::sendUpdateResult( 00217 FirmwareUpdateResource::ARM_UCS_LWM2M_RESULT_ERROR_HASH); 00218 break; 00219 default: 00220 break; 00221 } 00222 00223 if (retval == 0) 00224 { 00225 result.code = ERR_NONE; 00226 } 00227 00228 return result; 00229 } 00230 00231 /** 00232 * @brief Send current firmware name. 00233 * @details The firmware name is the SHA256 hash. 00234 * 00235 * @param name Pointer to buffer struct. Hash is stored as byte array. 00236 * @return Error code. 00237 */ 00238 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_SendName(arm_uc_buffer_t* name) 00239 { 00240 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00241 00242 int32_t retval = -1; 00243 00244 if (name && name->ptr) 00245 { 00246 retval = FirmwareUpdateResource::sendPkgName(name->ptr, name->size); 00247 } 00248 00249 if (retval == 0) 00250 { 00251 result.code = ERR_NONE; 00252 } 00253 00254 return result; 00255 } 00256 00257 /** 00258 * @brief Send current firmware version. 00259 * @details The firmware version is the timestamp from the manifest that 00260 * authorized the firmware. 00261 * 00262 * @param version Timestamp, 64 bit unsigned integer. 00263 * @return Error code. 00264 */ 00265 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_SendVersion(uint64_t version) 00266 { 00267 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00268 00269 int32_t retval = FirmwareUpdateResource::sendPkgVersion(version); 00270 00271 if (retval == 0) 00272 { 00273 result.code = ERR_NONE; 00274 } 00275 00276 return result; 00277 } 00278 00279 /** 00280 * @brief Set the bootloader hash. 00281 * @details The bootloader hash is a hash of the bootloader. This is 00282 * used for tracking the version of the bootloader used. 00283 * 00284 * @param name Pointer to buffer struct. Hash is stored as byte array. 00285 * @return Error code. 00286 */ 00287 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_SetBootloaderHash(arm_uc_buffer_t* hash) 00288 { 00289 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00290 00291 int32_t retval = DeviceMetadataResource::setBootloaderHash(hash); 00292 00293 if (retval == 0) 00294 { 00295 result.code = ERR_NONE; 00296 } 00297 00298 return result; 00299 } 00300 00301 /** 00302 * @brief Set the OEM bootloader hash. 00303 * @details If the end-user has modified the bootloader the hash of the 00304 * modified bootloader can be set here. 00305 * 00306 * @param name Pointer to buffer struct. Hash is stored as byte array. 00307 * @return Error code. 00308 */ 00309 arm_uc_error_t ARM_UCS_LWM2M_MONITOR_SetOEMBootloaderHash(arm_uc_buffer_t* hash) 00310 { 00311 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00312 00313 int32_t retval = DeviceMetadataResource::setOEMBootloaderHash(hash); 00314 00315 if (retval == 0) 00316 { 00317 result.code = ERR_NONE; 00318 } 00319 00320 return result; 00321 } 00322 00323 ARM_UPDATE_MONITOR ARM_UCS_LWM2M_MONITOR = 00324 { 00325 .GetVersion = ARM_UCS_LWM2M_MONITOR_GetVersion, 00326 .GetCapabilities = ARM_UCS_LWM2M_MONITOR_GetCapabilities, 00327 .Initialize = ARM_UCS_LWM2M_MONITOR_Initialize, 00328 .Uninitialize = ARM_UCS_LWM2M_MONITOR_Uninitialize, 00329 00330 .SendState = ARM_UCS_LWM2M_MONITOR_SendState, 00331 .SendUpdateResult = ARM_UCS_LWM2M_MONITOR_SendUpdateResult, 00332 .SendName = ARM_UCS_LWM2M_MONITOR_SendName, 00333 .SendVersion = ARM_UCS_LWM2M_MONITOR_SendVersion, 00334 00335 .SetBootloaderHash = ARM_UCS_LWM2M_MONITOR_SetBootloaderHash, 00336 .SetOEMBootloaderHash = ARM_UCS_LWM2M_MONITOR_SetOEMBootloaderHash 00337 };
Generated on Tue Jul 12 2022 16:22:06 by
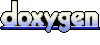