
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
ftcd_comm_serial.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 // Note: this macro is needed on armcc to get the the PRI*32 macros 00018 // from inttypes.h in a C++ code. 00019 #ifndef __STDC_FORMAT_MACROS 00020 #define __STDC_FORMAT_MACROS 00021 #endif 00022 00023 #include <stdlib.h> 00024 #include "pv_endian.h" 00025 #include "pal.h" 00026 #include "pv_log.h" 00027 #include "ftcd_comm_serial.h" 00028 00029 #define TRACE_GROUP "fcsr" 00030 00031 FtcdCommSerial::FtcdCommSerial(PinName TX, PinName RX, uint32_t baud) : mbed::Serial(TX, RX, baud) 00032 { 00033 } 00034 00035 FtcdCommSerial::~FtcdCommSerial() 00036 { 00037 } 00038 00039 size_t FtcdCommSerial::_serial_read(char *buffOut, size_t buffSize) 00040 { 00041 size_t count; 00042 //TODO: 00043 //getc is blocking. There is currently no way to check if there is anything left to read. seems readable() us not working 00044 //Once determined, the relevant check should be added to this code. 00045 for (count = 0; count < buffSize; count++) { 00046 buffOut[count] = getc(); 00047 } 00048 return count; 00049 } 00050 00051 size_t FtcdCommSerial::_serial_write(const char *buff, size_t buffSize) 00052 { 00053 lock(); 00054 00055 for (size_t i = 0; i < buffSize; i++) { 00056 putc(buff[i]); 00057 } 00058 00059 unlock(); 00060 00061 return buffSize; 00062 } 00063 00064 ftcd_comm_status_e FtcdCommSerial::is_token_detected() 00065 { 00066 char c; 00067 char expected_token[] = FTCD_MSG_HEADER_TOKEN; 00068 size_t idx = 0; 00069 00070 //read char by char to detect token 00071 while (idx < FTCD_MSG_HEADER_TOKEN_SIZE_BYTES) { 00072 _serial_read(&c, 1); 00073 if (c == expected_token[idx]) { 00074 idx++; 00075 } else { 00076 idx = 0; 00077 } 00078 } 00079 return FTCD_COMM_STATUS_SUCCESS; 00080 } 00081 00082 uint32_t FtcdCommSerial::read_message_size() 00083 { 00084 uint32_t message_size = 0; 00085 00086 size_t read_chars = _serial_read(reinterpret_cast<char*>(&message_size), sizeof(message_size)); 00087 if (read_chars != sizeof(message_size)) { 00088 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Failed reading message size (read %d bytes out of %d)\r", read_chars, sizeof(message_size)); 00089 return 0; 00090 } 00091 00092 return message_size; 00093 } 00094 00095 bool FtcdCommSerial::read_message(uint8_t *message_out, size_t message_size) 00096 { 00097 if (message_out == NULL) { 00098 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Invalid message buffer\r"); 00099 return false; 00100 } 00101 00102 // Read CBOR message bytes 00103 // We assume that LENGTH is NOT bigger than INT_MAX 00104 size_t read_chars = _serial_read(reinterpret_cast<char*>(message_out), message_size); 00105 if (read_chars != message_size) { 00106 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Failed reading message bytes (read %d bytes out of %d)\r", read_chars, message_size); 00107 return false; 00108 } 00109 00110 return true; 00111 } 00112 00113 bool FtcdCommSerial::read_message_signature(uint8_t *sig, size_t sig_size) 00114 { 00115 if (sig == NULL) { 00116 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Invalid sig buffer\r"); 00117 return false; 00118 } 00119 00120 // Read signature from medium 00121 size_t read_chars = _serial_read(reinterpret_cast<char*>(sig), sig_size); 00122 if (read_chars != sig_size) { 00123 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Failed reading message signature bytes (read %d bytes out of %d)\r", read_chars, sig_size); 00124 return false; 00125 } 00126 00127 return true; 00128 } 00129 00130 bool FtcdCommSerial::send(const uint8_t *data, uint32_t data_size) 00131 { 00132 if (data == NULL) { 00133 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Invalid response_message\r"); 00134 return false; 00135 } 00136 if (data_size == 0) { 00137 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Got an empty message\r"); 00138 return false; 00139 } 00140 00141 // Send data on the serial medium 00142 size_t write_chars = _serial_write(reinterpret_cast<const char*>(data), data_size); 00143 if (write_chars != data_size) { 00144 mbed_tracef(TRACE_LEVEL_CMD, TRACE_GROUP,"Failed writing message bytes (wrote %" PRIu32 " bytes out of %" PRIu32 ")\r", (uint32_t)write_chars, data_size); 00145 return false; 00146 } 00147 00148 return true; 00149 }
Generated on Tue Jul 12 2022 16:22:06 by
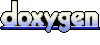