
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
fcc_sotp.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FCC_SOTP_H__ 00018 #define __FCC_SOTP_H__ 00019 00020 #include "factory_configurator_client.h" 00021 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif 00025 00026 /* Enum representing the different types of data that can be stored in SOTP */ 00027 typedef enum fcc_sotp_type_ { 00028 FCC_SOTP_TYPE_ROT = 1, 00029 FCC_SOTP_TYPE_FACTORY_DISABLE = 2, 00030 FCC_SOTP_TYPE_ENTROPY = 5 // Note that the values 3 and 4 are reserved for backward time and secure time - not used by us. 00031 } fcc_sotp_type_e; 00032 00033 00034 // Size of factory disabled flag in SOTP - internal use only. 00035 #define FCC_FACTORY_DISABLE_FLAG_SIZE sizeof(int64_t) 00036 00037 /** Writes data to SOTP 00038 * 00039 * @param data[in] The data to store in SOTP. 00040 * @param data_size[in] The size of buf in bytes. Must be divisible by 8, and less than or equal to 1024. Must be according to sotp_type. 00041 * @param sotp_type[in] Enum representing the type of the item to be stored in SOTP. 00042 * 00043 * @return 00044 * FCC_STATUS_SUCCESS for success or one of the `::fcc_status_e` errors otherwise. 00045 */ 00046 fcc_status_e fcc_sotp_data_store(const uint8_t *data, size_t data_size, fcc_sotp_type_e sotp_type); 00047 00048 /** Reads data from SOTP 00049 * 00050 * @param data_out[out] A buffer to retrieve data from SOTP. 00051 * @param data_size_max[in] The size of data_out max. bytes. 00052 * @param data_actual_size_out[out] The actual size of bytes returned by this function, should be less or equal to data_size_max. 00053 * @param sotp_type[in] Enum representing the type of the item to be stored in SOTP. 00054 * 00055 * @return 00056 * FCC_STATUS_SUCCESS for success or one of the `::fcc_status_e` errors otherwise. 00057 */ 00058 fcc_status_e fcc_sotp_data_retrieve(uint8_t *data_out, size_t data_size_max, size_t *data_actual_size_out, fcc_sotp_type_e sotp_type); 00059 00060 00061 // FIXME: The following should all be removed once SOTP APIs are implemented 00062 #define MAX_SOTP_BUFFER_SIZE FCC_ENTROPY_SIZE 00063 00064 typedef struct sotp_entree_ { 00065 bool write_disabled; 00066 uint8_t type; 00067 int64_t data[MAX_SOTP_BUFFER_SIZE / 8]; 00068 size_t data_size_in_bytes; 00069 } sotp_entree_s; 00070 00071 extern sotp_entree_s g_sotp_rot; 00072 extern sotp_entree_s g_sotp_factory_disabled; 00073 extern sotp_entree_s g_sotp_entropy; 00074 00075 bool SOTP_Set(uint8_t type, uint8_t size, const int64_t *data); 00076 00077 00078 /** Gets the soft OTP's info by the given owner and type. 00079 * 00080 * @param owner [in] The module owner. 00081 * @param type [in] Type specific to the given owner 00082 * @param revision_out [out] The revision number (none zero value) 00083 * @param data_out [out] Array of int64 represents the data stored in the SOTP 00084 * @param data_size_out [out] Size of data, must be in the range of 0-128 00085 * 00086 * @returns 00087 * status true/false. 00088 */ 00089 bool SOTP_Get(uint8_t type, int64_t *data_out, uint8_t *data_size_out); 00090 00091 // FIXME: remove this TestOnly function when SOTP will be implemented 00092 // The storage_reset() should reset also the SOTP 00093 void SOTP_TestOnly_reset(void); 00094 00095 #ifdef __cplusplus 00096 } 00097 #endif 00098 00099 #endif //__FCC_SOTP_H__
Generated on Tue Jul 12 2022 16:22:05 by
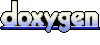