
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "WizFi310Interface.h" 00003 #include "UDPSocket.h" 00004 #include "greentea-client/test_env.h" 00005 #include "unity/unity.h" 00006 #include "utest.h" 00007 00008 using namespace utest::v1; 00009 00010 00011 #ifndef MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE 00012 #define MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE 64 00013 #endif 00014 00015 #ifndef MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT 00016 #define MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT 500 00017 #endif 00018 00019 #ifndef MBED_CFG_WIZFI310_TX 00020 #define MBED_CFG_WIZFI310_TX D1 00021 #endif 00022 00023 #ifndef MBED_CFG_WIZFI310_RX 00024 #define MBED_CFG_WIZFI310_RX D0 00025 #endif 00026 00027 #ifndef MBED_CFG_WIZFI310_DEBUG 00028 #define MBED_CFG_WIZFI310_DEBUG false 00029 #endif 00030 00031 #define STRINGIZE(x) STRINGIZE2(x) 00032 #define STRINGIZE2(x) #x 00033 00034 00035 namespace { 00036 char tx_buffer[MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE] = {0}; 00037 char rx_buffer[MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE] = {0}; 00038 const char ASCII_MAX = '~' - ' '; 00039 const int ECHO_LOOPS = 16; 00040 char uuid[48] = {0}; 00041 } 00042 00043 void prep_buffer(char *uuid, char *tx_buffer, size_t tx_size) { 00044 size_t i = 0; 00045 00046 memcpy(tx_buffer, uuid, strlen(uuid)); 00047 i += strlen(uuid); 00048 00049 tx_buffer[i++] = ' '; 00050 00051 for (; i<tx_size; ++i) { 00052 tx_buffer[i] = (rand() % 10) + '0'; 00053 } 00054 } 00055 00056 void test_udp_echo() { 00057 WizFi310Interface net(MBED_CFG_WIZFI310_TX, MBED_CFG_WIZFI310_RX, MBED_CFG_WIZFI310_DEBUG); 00058 00059 int err = net.connect(STRINGIZE(MBED_CFG_WIZFI310_SSID), STRINGIZE(MBED_CFG_WIZFI310_PASS)); 00060 TEST_ASSERT_EQUAL(0, err); 00061 00062 if (err) { 00063 printf("MBED: failed to connect with an error of %d\r\n", err); 00064 TEST_ASSERT_EQUAL(0, err); 00065 } 00066 00067 printf("UDP client IP Address is %s\n", net.get_ip_address()); 00068 00069 greentea_send_kv("target_ip", net.get_ip_address()); 00070 00071 char recv_key[] = "host_port"; 00072 char ipbuf[60] = {0}; 00073 char portbuf[16] = {0}; 00074 unsigned int port = 0; 00075 00076 UDPSocket sock; 00077 sock.open(&net); 00078 sock.set_timeout(MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT); 00079 00080 greentea_send_kv("host_ip", " "); 00081 greentea_parse_kv(recv_key, ipbuf, sizeof(recv_key), sizeof(ipbuf)); 00082 00083 greentea_send_kv("host_port", " "); 00084 greentea_parse_kv(recv_key, portbuf, sizeof(recv_key), sizeof(ipbuf)); 00085 sscanf(portbuf, "%u", &port); 00086 00087 printf("MBED: UDP Server IP address received: %s:%d \n", ipbuf, port); 00088 SocketAddress udp_addr(ipbuf, port); 00089 00090 int success = 0; 00091 for (int i = 0; success < ECHO_LOOPS; i++) { 00092 prep_buffer(uuid, tx_buffer, sizeof(tx_buffer)); 00093 const int ret = sock.sendto(udp_addr, tx_buffer, sizeof(tx_buffer)); 00094 if (ret >= 0) { 00095 printf("[%02d] sent %d bytes - %.*s \n", i, ret, ret, tx_buffer); 00096 } else { 00097 printf("[%02d] Network error %d\n", i, ret); 00098 continue; 00099 } 00100 00101 SocketAddress temp_addr; 00102 const int n = sock.recvfrom(&temp_addr, rx_buffer, sizeof(rx_buffer)); 00103 if (n >= 0) { 00104 printf("[%02d] recv %d bytes - %.*s \n", i, n, n, tx_buffer); 00105 } else { 00106 printf("[%02d] Network error %d\n", i, n); 00107 continue; 00108 } 00109 00110 if ((temp_addr == udp_addr && 00111 n == sizeof(tx_buffer) && 00112 memcmp(rx_buffer, tx_buffer, sizeof(rx_buffer)) == 0)) { 00113 success += 1; 00114 00115 printf("[%02d] success #%d\n", i, success); 00116 continue; 00117 } 00118 00119 // failed, clean out any remaining bad packets 00120 sock.set_timeout(0); 00121 while (true) { 00122 err = sock.recvfrom(NULL, NULL, 0); 00123 if (err == NSAPI_ERROR_WOULD_BLOCK) { 00124 break; 00125 } 00126 } 00127 sock.set_timeout(MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT); 00128 } 00129 00130 sock.close(); 00131 net.disconnect(); 00132 TEST_ASSERT_EQUAL(ECHO_LOOPS, success); 00133 } 00134 00135 00136 // Test setup 00137 utest::v1::status_t test_setup(const size_t number_of_cases) { 00138 GREENTEA_SETUP(120, "udp_echo"); 00139 return verbose_test_setup_handler(number_of_cases); 00140 } 00141 00142 Case cases[] = { 00143 Case("UDP echo", test_udp_echo), 00144 }; 00145 00146 Specification specification(test_setup, cases); 00147 00148 int main() { 00149 return !Harness::run(specification); 00150 } 00151
Generated on Tue Jul 12 2022 16:22:07 by
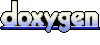