
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ESP8266Interface.h" 00003 #include "UDPSocket.h" 00004 #include "greentea-client/test_env.h" 00005 #include "unity/unity.h" 00006 #include "utest.h" 00007 00008 using namespace utest::v1; 00009 00010 00011 #ifndef MBED_CFG_UDP_DTLS_HANDSHAKE_BUFFER_SIZE 00012 #define MBED_CFG_UDP_DTLS_HANDSHAKE_BUFFER_SIZE 512 00013 #endif 00014 00015 #ifndef MBED_CFG_UDP_DTLS_HANDSHAKE_RETRIES 00016 #define MBED_CFG_UDP_DTLS_HANDSHAKE_RETRIES 16 00017 #endif 00018 00019 #ifndef MBED_CFG_UDP_DTLS_HANDSHAKE_PATTERN 00020 #define MBED_CFG_UDP_DTLS_HANDSHAKE_PATTERN 112, 384, 200, 219, 25 00021 #endif 00022 00023 #ifndef MBED_CFG_UDP_DTLS_HANDSHAKE_TIMEOUT 00024 #define MBED_CFG_UDP_DTLS_HANDSHAKE_TIMEOUT 1500 00025 #endif 00026 00027 #ifndef MBED_CFG_ESP8266_TX 00028 #define MBED_CFG_ESP8266_TX D1 00029 #endif 00030 00031 #ifndef MBED_CFG_ESP8266_RX 00032 #define MBED_CFG_ESP8266_RX D0 00033 #endif 00034 00035 #ifndef MBED_CFG_ESP8266_DEBUG 00036 #define MBED_CFG_ESP8266_DEBUG false 00037 #endif 00038 00039 #define STRINGIZE(x) STRINGIZE2(x) 00040 #define STRINGIZE2(x) #x 00041 00042 uint8_t buffer[MBED_CFG_UDP_DTLS_HANDSHAKE_BUFFER_SIZE] = {0}; 00043 int udp_dtls_handshake_pattern[] = {MBED_CFG_UDP_DTLS_HANDSHAKE_PATTERN}; 00044 const int udp_dtls_handshake_count = sizeof(udp_dtls_handshake_pattern) / sizeof(int); 00045 00046 void test_udp_dtls_handshake() { 00047 ESP8266Interface net(MBED_CFG_ESP8266_TX, MBED_CFG_ESP8266_RX, MBED_CFG_ESP8266_DEBUG); 00048 int err = net.connect(STRINGIZE(MBED_CFG_ESP8266_SSID), STRINGIZE(MBED_CFG_ESP8266_PASS)); 00049 TEST_ASSERT_EQUAL(0, err); 00050 00051 printf("MBED: UDPClient IP address is '%s'\n", net.get_ip_address()); 00052 printf("MBED: UDPClient waiting for server IP and port...\n"); 00053 00054 greentea_send_kv("target_ip", net.get_ip_address()); 00055 00056 bool result = false; 00057 00058 char recv_key[] = "host_port"; 00059 char ipbuf[60] = {0}; 00060 char portbuf[16] = {0}; 00061 unsigned int port = 0; 00062 00063 greentea_send_kv("host_ip", " "); 00064 greentea_parse_kv(recv_key, ipbuf, sizeof(recv_key), sizeof(ipbuf)); 00065 00066 greentea_send_kv("host_port", " "); 00067 greentea_parse_kv(recv_key, portbuf, sizeof(recv_key), sizeof(ipbuf)); 00068 sscanf(portbuf, "%u", &port); 00069 00070 printf("MBED: UDP Server IP address received: %s:%d \n", ipbuf, port); 00071 00072 // align each size to 4-bits 00073 for (int i = 0; i < udp_dtls_handshake_count; i++) { 00074 udp_dtls_handshake_pattern[i] = (~0xf & udp_dtls_handshake_pattern[i]) + 0x10; 00075 } 00076 00077 printf("MBED: DTLS pattern ["); 00078 for (int i = 0; i < udp_dtls_handshake_count; i++) { 00079 printf("%d", udp_dtls_handshake_pattern[i]); 00080 if (i != udp_dtls_handshake_count-1) { 00081 printf(", "); 00082 } 00083 } 00084 printf("]\r\n"); 00085 00086 UDPSocket sock; 00087 SocketAddress udp_addr(ipbuf, port); 00088 sock.set_timeout(MBED_CFG_UDP_DTLS_HANDSHAKE_TIMEOUT); 00089 00090 for (int attempt = 0; attempt < MBED_CFG_UDP_DTLS_HANDSHAKE_RETRIES; attempt++) { 00091 err = sock.open(&net); 00092 TEST_ASSERT_EQUAL(0, err); 00093 00094 for (int i = 0; i < udp_dtls_handshake_count; i++) { 00095 buffer[i] = udp_dtls_handshake_pattern[i] >> 4; 00096 } 00097 00098 err = sock.sendto(udp_addr, buffer, udp_dtls_handshake_count); 00099 printf("UDP: tx -> %d\r\n", err); 00100 TEST_ASSERT_EQUAL(udp_dtls_handshake_count, err); 00101 00102 int step = 0; 00103 while (step < udp_dtls_handshake_count) { 00104 err = sock.recvfrom(NULL, buffer, sizeof(buffer)); 00105 printf("UDP: rx <- %d ", err); 00106 00107 // check length 00108 if (err != udp_dtls_handshake_pattern[step]) { 00109 printf("x (expected %d)\r\n", udp_dtls_handshake_pattern[step]); 00110 break; 00111 } 00112 00113 // check quick xor of packet 00114 uint8_t check = 0; 00115 for (int j = 0; j < udp_dtls_handshake_pattern[step]; j++) { 00116 check ^= buffer[j]; 00117 } 00118 00119 if (check != 0) { 00120 printf("x (checksum 0x%02x)\r\n", check); 00121 break; 00122 } 00123 00124 // successfully got a packet 00125 printf("\r\n"); 00126 step += 1; 00127 } 00128 00129 err = sock.close(); 00130 TEST_ASSERT_EQUAL(0, err); 00131 00132 // got through all steps, test passed 00133 if (step == udp_dtls_handshake_count) { 00134 result = true; 00135 break; 00136 } 00137 } 00138 00139 net.disconnect(); 00140 TEST_ASSERT_EQUAL(true, result); 00141 } 00142 00143 00144 // Test setup 00145 utest::v1::status_t test_setup(const size_t number_of_cases) { 00146 GREENTEA_SETUP(120, "udp_shotgun"); 00147 return verbose_test_setup_handler(number_of_cases); 00148 } 00149 00150 Case cases[] = { 00151 Case("UDP DTLS handshake", test_udp_dtls_handshake), 00152 }; 00153 00154 Specification specification(test_setup, cases); 00155 00156 int main() { 00157 return !Harness::run(specification); 00158 } 00159
Generated on Tue Jul 12 2022 16:22:07 by
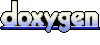