
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
atomic.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2015-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef ATOMIC_QUEUE_ATOMIC_H 00020 #define ATOMIC_QUEUE_ATOMIC_H 00021 #include <stdint.h> 00022 00023 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 enum { 00030 AQ_ATOMIC_CAS_DEREF_SUCCESS = 0, 00031 AQ_ATOMIC_CAS_DEREF_NULLPTR, 00032 AQ_ATOMIC_CAS_DEREF_VALUE, 00033 AQ_ATOMIC_CAS_DEREF_INTERUPTED, 00034 }; 00035 00036 int aq_atomic_cas_uintptr(uintptr_t *ptr, uintptr_t oldval, uintptr_t newval); 00037 00038 /** 00039 * @brief Atomically compares the value of a dereferenced pointer, replacing the pointer on success. 00040 * @detail aq_atomic_cas_deref_uintptr provides a mechanism to atomically change a pointer based on a value contained 00041 * in the structure referenced by the pointer. This is done in the following sequence of operations: 00042 * 00043 * 1. Load the value of `*ptrAddr` 00044 * 2. Optionally store the current value of `*ptrAddr` 00045 * 3. Check that the pointer is valid: `*ptrAddr != NULL` 00046 * 4. Check the value of the referenced location: `*(*ptrAddr + valueOffset) == expectedDerefValue` (casts omitted) 00047 * 5. If 3 and 4 succeeded, store newPtrValue: `*ptrAddr = newPtrValue` (NOTE: in non-blocking implementations, this step can fail) 00048 * 6. Return a status code based on the results of 3, 4, 5. 00049 * 00050 * @param[in,out] ptrAddr The address of the pointer to manipulate 00051 * @param[out] currentPtrValue A pointer to a container for the current value of *ptrAddr 00052 * @param[in] expectedDerefValue This is the value that is expected at *(uintptr_t *)((uintptr_t)*ptrAddr + valueOffset) 00053 * @param[in] newPtrValue The value to store to *ptrAddr if the comparison is successful 00054 * @param[in] valueOffset The offset of the target value from *ptrAddr 00055 * 00056 * @retval AQ_ATOMIC_CAS_DEREF_SUCCESS The compare and set has succeeded 00057 * @retval AQ_ATOMIC_CAS_DEREF_NULLPTR `*ptrAddr` was `NULL` 00058 * @retval AQ_ATOMIC_CAS_DEREF_VALUE The value test failed (*(*ptrAddr + valueOffset) != expectedDerefValue`) 00059 * @retval AQ_ATOMIC_CAS_DEREF_INTERUPTED Another context modified `*ptrAddr` 00060 */ 00061 int aq_atomic_cas_deref_uintptr(uintptr_t* volatile * ptrAddr, 00062 uintptr_t** currentPtrValue, 00063 uintptr_t expectedDerefValue, 00064 uintptr_t* newPtrValue, 00065 uintptr_t valueOffset); 00066 00067 #ifdef __cplusplus 00068 } // extern "C" 00069 #endif 00070 #endif // ATOMIC_QUEUE_ATOMIC_H
Generated on Tue Jul 12 2022 16:22:04 by
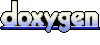