
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
arm_uc_scheduler.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef ARM_UPDATE_SCHEDULER_H 00020 #define ARM_UPDATE_SCHEDULER_H 00021 00022 #include <stddef.h> 00023 #include <stdint.h> 00024 #include <stdbool.h> 00025 00026 /** 00027 * Simple scheduler useful for collecting events from different threads and 00028 * interrupt context so they can be executed from the same thread/context. 00029 * 00030 * This can reduce the calldepth, ensure functions are executed from the same 00031 * thread, and provide a method for breaking out of interrupt context. 00032 * 00033 * Callbacks are executed FIFO. 00034 * 00035 * The notification handler should be used for processing the queue whenever it 00036 * is non-empty. 00037 */ 00038 00039 /** 00040 * Use custom struct for the lockfree queue. 00041 * Struct contains function pointer callback and uint32_t parameter. 00042 */ 00043 #define ATOMIC_QUEUE_CUSTOM_ELEMENT 00044 00045 struct lockfree_queue_element { 00046 struct lockfree_queue_element * volatile next; 00047 uintptr_t lock; 00048 void (*callback)(uint32_t); 00049 uint32_t parameter; 00050 }; 00051 00052 #include "atomic-queue/atomic-queue.h" 00053 00054 #ifdef __cplusplus 00055 extern "C" { 00056 #endif 00057 00058 typedef struct lockfree_queue_element arm_uc_callback_t; 00059 00060 /** 00061 * @brief Add function to be executed to the queue. 00062 * @details The caller is responsible for managing the memory for each element 00063 * in the queue, i.e., allocating enough struct lockfree_queue_element 00064 * to hold the number of outstanding callbacks in the queue. 00065 * 00066 * @param storage Pointer to struct lockfree_queue_element. 00067 * @param callback Function pointer to function being scheduled to run later. 00068 * @param parameter uint32_t value to be passed as parameter to the callback function. 00069 * @return True when the callback was successfully scheduled. 00070 */ 00071 bool ARM_UC_PostCallback(arm_uc_callback_t* storage, void (*callback)(uint32_t), uint32_t parameter); 00072 00073 /** 00074 * @brief Calling this function processes all callbacks in the queue. 00075 */ 00076 void ARM_UC_ProcessQueue(void); 00077 00078 /** 00079 * @brief Calling this function processes a single callback in the queue. 00080 * @details The return value indicates whether there are more callbacks 00081 * in the queue that needs handling. 00082 * @return True when there are more callbacks in the queue, false otherwise. 00083 */ 00084 bool ARM_UC_ProcessSingleCallback(void); 00085 00086 /** 00087 * @brief Register callback function for when callbacks are added to an empty queue. 00088 * @details This function is called at least once (maybe more) when callbacks are 00089 * added to an empty queue. Useful for scheduling when the queue needs 00090 * to be processed. 00091 * @param handler Function pointer to function to be called when elements are 00092 * added to an empty queue. 00093 */ 00094 void ARM_UC_AddNotificationHandler(void (*handler)(void)); 00095 00096 #ifdef __cplusplus 00097 } 00098 #endif 00099 00100 #endif // ARM_UPDATE_SCHEDULER_H
Generated on Tue Jul 12 2022 16:22:03 by
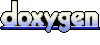