
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
WNCIO.h
Go to the documentation of this file.
00001 /** 00002 * copyright (c) 2017-2018, James Flynn 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 /* 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 00021 /** 00022 * @file WNCIO.h 00023 * @brief A class that WNCInterface uses for input/output 00024 * 00025 * @author James Flynn 00026 * 00027 * @date 1-Feb-2018 00028 * 00029 */ 00030 00031 #ifndef __WNCIO__ 00032 #define __WNCIO__ 00033 #include <stdio.h> 00034 #include "mbed.h" 00035 00036 /** WncIO class 00037 * Used to read/write the WNC UART using FILE I/O. 00038 */ 00039 00040 class WncIO 00041 { 00042 public: 00043 //! Create class with either stdio or a pointer to a uart 00044 WncIO( UARTSerial * uart): m_puart(uart) {;} 00045 ~WncIO() {}; 00046 00047 //! standard printf() functionallity 00048 int printf( char * fmt, ...) { 00049 char buffer[256]; 00050 int ret=0; 00051 va_list args; 00052 va_start (args, fmt); 00053 vsnprintf(buffer, sizeof(buffer), fmt, args); 00054 prt.lock(); 00055 ret=m_puart->write(buffer,strlen(buffer)); 00056 prt.unlock(); 00057 va_end (args); 00058 return ret; 00059 } 00060 00061 //! standard putc() functionallity 00062 int putc( int c ) { 00063 int ret=0; 00064 prt.lock(); 00065 ret=m_puart->write((const void*)&c,1); 00066 prt.unlock(); 00067 return ret; 00068 } 00069 00070 //! standard puts() functionallity 00071 int puts( const char * str ) { 00072 int ret=0; 00073 prt.lock(); 00074 ret=m_puart->write(str,strlen(str)); 00075 prt.unlock(); 00076 return ret; 00077 } 00078 00079 //! return true when data is available, false otherwise 00080 bool readable( void ) { 00081 return m_puart->readable(); 00082 } 00083 00084 //! get the next character available from the uart 00085 int getc( void ) { 00086 char c; 00087 m_puart->read( &c, 1 ); 00088 return c; 00089 } 00090 00091 //! set the uart baud rate 00092 void baud( int baud ) { 00093 m_puart->set_baud(baud); 00094 } 00095 00096 private: 00097 UARTSerial *m_puart; 00098 Mutex prt; 00099 }; 00100 00101 #endif 00102
Generated on Tue Jul 12 2022 16:22:13 by
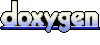