
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
FileSystemInit.c
00001 #include "pal.h" 00002 #include <errno.h> 00003 #include <sys/stat.h> 00004 #include <sys/types.h> 00005 #include <sys/mount.h> 00006 00007 #ifndef PRIMARY_PARTITION_NAME 00008 #define PRIMARY_PARTITION_NAME "/dev/mmcblk0p3" 00009 #endif 00010 00011 #ifndef SECONDARY_PARTITION_NAME 00012 #define SECONDARY_PARTITION_NAME "/dev/mmcblk0p4" 00013 #endif 00014 00015 #ifndef PAL_PARTITION_FORMAT_TYPE 00016 #define PAL_PARTITION_FORMAT_TYPE "ext4" 00017 #endif 00018 00019 #ifndef PARTITION_FORMAT_ADDITIONAL_PARAMS 00020 #define PARTITION_FORMAT_ADDITIONAL_PARAMS NULL 00021 #endif 00022 // Desktop Linux 00023 // In order for tests to pass for all partition configurations we need to simulate the case of multiple 00024 // partitions using a single folder. We do this by creating one or two different sub-folders, depending on 00025 // the configuration. 00026 palStatus_t fileSystemCreateRootFolders(void) 00027 { 00028 palStatus_t status = PAL_SUCCESS; 00029 char folder[PAL_MAX_FILE_AND_FOLDER_LENGTH] = {0}; 00030 00031 // Get default mount point. 00032 status = pal_fsGetMountPoint(PAL_FS_PARTITION_PRIMARY, PAL_MAX_FILE_AND_FOLDER_LENGTH, folder); 00033 if(status != PAL_SUCCESS) 00034 { 00035 return PAL_ERR_GENERIC_FAILURE; 00036 } 00037 printf("Mount point for primary partition: %s\r\n",folder); 00038 // Make the sub-folder 00039 int res = mkdir(folder,0744); 00040 if(res) 00041 { 00042 // Ignore error if it exists 00043 if( errno != EEXIST) 00044 { 00045 printf("mkdir failed errno= %d\r\n",errno); 00046 return PAL_ERR_GENERIC_FAILURE; 00047 } 00048 } 00049 00050 res = mount(PRIMARY_PARTITION_NAME, folder, PAL_PARTITION_FORMAT_TYPE, 0 ,PARTITION_FORMAT_ADDITIONAL_PARAMS); 00051 if (res) 00052 { 00053 if( errno != EBUSY) 00054 { 00055 printf("mounting failed %d\r\n",errno); 00056 return PAL_ERR_GENERIC_FAILURE; 00057 } 00058 } 00059 // Get default mount point. 00060 memset(folder,0,sizeof(folder)); 00061 status = pal_fsGetMountPoint(PAL_FS_PARTITION_SECONDARY, PAL_MAX_FILE_AND_FOLDER_LENGTH, folder); 00062 printf("Mount point for secondary partition: %s\r\n",folder); 00063 if(status != PAL_SUCCESS) 00064 { 00065 return PAL_ERR_GENERIC_FAILURE; 00066 } 00067 00068 // Make the sub-folder 00069 res = mkdir(folder,0744); 00070 if(res) 00071 { 00072 // Ignore error if it exists 00073 if( errno != EEXIST) 00074 { 00075 printf("mkdir failed errno= %d\r\n",errno); 00076 return PAL_ERR_GENERIC_FAILURE; 00077 } 00078 } 00079 00080 res = mount(SECONDARY_PARTITION_NAME, folder,PAL_PARTITION_FORMAT_TYPE ,0 ,PARTITION_FORMAT_ADDITIONAL_PARAMS); 00081 if (res) 00082 { 00083 if( errno != EBUSY) 00084 { 00085 printf("mounting failed %d\r\n",errno); 00086 return PAL_ERR_GENERIC_FAILURE; 00087 } 00088 } 00089 00090 if(status != PAL_SUCCESS) 00091 { 00092 return PAL_ERR_GENERIC_FAILURE; 00093 } 00094 00095 return PAL_SUCCESS; 00096 }
Generated on Tue Jul 12 2022 16:22:05 by
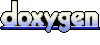