
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
FileSystemInit.c
00001 #include "pal.h" 00002 #include "FreeRTOS.h" 00003 #include "task.h" 00004 #include "fsl_mpu.h" 00005 #include "ff.h" 00006 #include "diskio.h" 00007 #include "sdhc_config.h" 00008 #include "fsl_debug_console.h" 00009 00010 00011 //uncomment this to create the partitions 00012 //#define PAL_EXAMPLE_GENERATE_PARTITION 1 00013 00014 #if (PAL_NUMBER_OF_PARTITIONS > 0) 00015 00016 #ifndef _MULTI_PARTITION 00017 #error "Please Define _MULTI_PARTITION in ffconf.h" 00018 #endif 00019 00020 #if ((PAL_NUMBER_OF_PARTITIONS > 2) || (PAL_NUMBER_OF_PARTITIONS < 0)) 00021 #error "Pal partition number is not supported, please set to a number between 0 and 2" 00022 #endif 00023 00024 PARTITION VolToPart[] = { 00025 #if (PAL_NUMBER_OF_PARTITIONS > 0) 00026 {SDDISK,1}, /* 0: */ 00027 #endif 00028 #if (PAL_NUMBER_OF_PARTITIONS > 1) 00029 {SDDISK,2} /* 1: */ 00030 #endif 00031 }; 00032 #endif 00033 00034 bool FileSystemInit = false; 00035 #define MAX_SD_READ_RETRIES 5 00036 #define LABEL_LENGTH 66 00037 /*! 00038 * @brief Get event instance. 00039 * @param eventType The event type 00040 * @return The event instance's pointer. 00041 */ 00042 PAL_PRIVATE volatile uint32_t *EVENT_GetInstance(event_t eventType); 00043 00044 /*! @brief Transfer complete event. */ 00045 PAL_PRIVATE volatile uint32_t g_eventTransferComplete; 00046 00047 PAL_PRIVATE volatile uint32_t g_eventSDReady; 00048 00049 /*! @brief Time variable unites as milliseconds. */ 00050 PAL_PRIVATE volatile uint32_t g_timeMilliseconds; 00051 00052 /*! @brief Preallocated Work area (file system object) for logical drive, should NOT be free or lost*/ 00053 PAL_PRIVATE FATFS fileSystem[2]; 00054 00055 /*! \brief CallBack function for SD card initialization 00056 * Set systick reload value to generate 1ms interrupt 00057 * @param void 00058 * 00059 * \return void 00060 * 00061 */ 00062 void EVENT_InitTimer(void) 00063 { 00064 /* Set systick reload value to generate 1ms interrupt */ 00065 SysTick_Config(CLOCK_GetFreq(kCLOCK_CoreSysClk) / 1000U); 00066 } 00067 00068 00069 /*! \brief CallBack function for SD card initialization 00070 * 00071 * @param void 00072 * 00073 * \return pointer to the requested instance 00074 * 00075 */ 00076 PAL_PRIVATE volatile uint32_t *EVENT_GetInstance(event_t eventType) 00077 { 00078 volatile uint32_t *event; 00079 00080 switch (eventType) 00081 { 00082 case kEVENT_TransferComplete: 00083 event = &g_eventTransferComplete; 00084 break; 00085 default: 00086 event = NULL; 00087 break; 00088 } 00089 00090 return event; 00091 } 00092 00093 /*! \brief CallBack function for SD card initialization 00094 * 00095 * @param event_t 00096 * 00097 * \return TRUE if instance was found 00098 * 00099 */ 00100 bool EVENT_Create(event_t eventType) 00101 { 00102 volatile uint32_t *event = EVENT_GetInstance(eventType); 00103 00104 if (event) 00105 { 00106 *event = 0; 00107 return true; 00108 } 00109 else 00110 { 00111 return false; 00112 } 00113 } 00114 00115 /*! \brief blockDelay - Blocks the task and count the number of ticks given 00116 * 00117 * @param void 00118 * 00119 * \return TRUE - on success 00120 * 00121 */ 00122 void blockDelay(uint32_t Ticks) 00123 { 00124 uint32_t tickCounts = 0; 00125 for(tickCounts = 0; tickCounts < Ticks; tickCounts++){} 00126 } 00127 00128 00129 /*! \brief CallBack function for SD card initialization 00130 * 00131 * @param void 00132 * 00133 * \return TRUE - on success 00134 * 00135 */ 00136 bool EVENT_Wait(event_t eventType, uint32_t timeoutMilliseconds) 00137 { 00138 uint32_t startTime; 00139 uint32_t elapsedTime; 00140 00141 volatile uint32_t *event = EVENT_GetInstance(eventType); 00142 00143 if (timeoutMilliseconds && event) 00144 { 00145 startTime = g_timeMilliseconds; 00146 do 00147 { 00148 elapsedTime = (g_timeMilliseconds - startTime); 00149 } while ((*event == 0U) && (elapsedTime < timeoutMilliseconds)); 00150 *event = 0U; 00151 00152 return ((elapsedTime < timeoutMilliseconds) ? true : false); 00153 } 00154 else 00155 { 00156 return false; 00157 } 00158 } 00159 00160 /*! \brief CallBack function for SD card initialization 00161 * 00162 * @param eventType 00163 * 00164 * \return TRUE if instance was found 00165 * 00166 */ 00167 bool EVENT_Notify(event_t eventType) 00168 { 00169 volatile uint32_t *event = EVENT_GetInstance(eventType); 00170 00171 if (event) 00172 { 00173 *event = 1U; 00174 return true; 00175 } 00176 else 00177 { 00178 return false; 00179 } 00180 } 00181 00182 /*! \brief CallBack function for SD card initialization 00183 * 00184 * @param eventType 00185 * 00186 * \return void 00187 * 00188 */ 00189 void EVENT_Delete(event_t eventType) 00190 { 00191 volatile uint32_t *event = EVENT_GetInstance(eventType); 00192 00193 if (event) 00194 { 00195 *event = 0U; 00196 } 00197 } 00198 00199 00200 /*! \brief This function mount the fatfs on and SD card 00201 * 00202 * @param void 00203 * 00204 * \return palStatus_t - PAL_SUCCESS when mount point succeeded 00205 * 00206 */ 00207 00208 void fileSystemMountDrive(void) 00209 { 00210 char folder1[PAL_MAX_FILE_AND_FOLDER_LENGTH] = {0}; 00211 char folder2[PAL_MAX_FILE_AND_FOLDER_LENGTH] = {0}; 00212 PRINTF("%s : Creating FileSystem SetUp thread!\r\n",__FUNCTION__); 00213 FRESULT fatResult; 00214 int count = 0; 00215 palStatus_t status = PAL_SUCCESS; 00216 00217 if (FileSystemInit == false) 00218 { 00219 //Detected SD card inserted 00220 while (!(GPIO_ReadPinInput(BOARD_SDHC_CD_GPIO_BASE, BOARD_SDHC_CD_GPIO_PIN))) 00221 { 00222 blockDelay(1000U); 00223 if (count++ > MAX_SD_READ_RETRIES) 00224 { 00225 break; 00226 } 00227 } 00228 00229 if(count < MAX_SD_READ_RETRIES) 00230 { 00231 /* Delay some time to make card stable. */ 00232 blockDelay(10000000U); 00233 #ifdef PAL_EXAMPLE_GENERATE_PARTITION 00234 #if (PAL_NUMBER_OF_PARTITIONS == 1) 00235 DWORD plist[] = {100,0,0,0}; 00236 #elif (PAL_NUMBER_OF_PARTITIONS == 2) //else of (PAL_NUMBER_OF_PARTITIONS == 1) 00237 DWORD plist[] = {50,50,0,0}; 00238 #endif //(PAL_NUMBER_OF_PARTITIONS == 1) 00239 BYTE work[_MAX_SS]; 00240 00241 fatResult= f_fdisk(SDDISK,plist, work); 00242 PRINTF("f_fdisk fatResult=%d\r\n",fatResult); 00243 if (FR_OK != fatResult) 00244 { 00245 PRINTF("Failed to create partitions in disk\r\n"); 00246 } 00247 #endif //PAL_EXAMPLE_GENERATE_PARTITION 00248 00249 00250 status = pal_fsGetMountPoint(PAL_FS_PARTITION_PRIMARY,PAL_MAX_FILE_AND_FOLDER_LENGTH,folder1); 00251 if (PAL_SUCCESS == status) 00252 { 00253 fatResult = f_mount(&fileSystem[0], folder1, 1U); 00254 if (FR_OK != fatResult) 00255 { 00256 PRINTF("Failed to mount partition %s in disk\r\n",folder1); 00257 } 00258 } 00259 else 00260 { 00261 PRINTF("Failed to get mount point for primary partition\r\n"); 00262 } 00263 00264 status = pal_fsGetMountPoint(PAL_FS_PARTITION_SECONDARY,PAL_MAX_FILE_AND_FOLDER_LENGTH,folder2); 00265 if (PAL_SUCCESS == status) 00266 { 00267 //if there is a different root folder for partition 1 and 2, mount the 2nd partition 00268 if (strncmp(folder1,folder2,PAL_MAX_FILE_AND_FOLDER_LENGTH)) 00269 { 00270 fatResult = f_mount(&fileSystem[1], folder2, 1U); 00271 if (FR_OK != fatResult) 00272 { 00273 PRINTF("Failed to mount partition %s in disk\r\n",folder2); 00274 } 00275 } 00276 } 00277 else 00278 { 00279 PRINTF("Failed to get mount point for secondary partition\r\n"); 00280 } 00281 00282 if (fatResult == FR_OK) 00283 { 00284 FileSystemInit = true; 00285 PRINTF("%s : Exit FileSystem SetUp thread!\r\n",__FUNCTION__); 00286 } 00287 } 00288 } 00289 vTaskDelete( NULL ); 00290 }
Generated on Tue Jul 12 2022 16:22:05 by
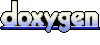