
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
ISM43362.cpp
00001 /* ISM43362 Example 00002 * 00003 * Copyright (c) STMicroelectronics 2018 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include <string.h> 00018 #include "ISM43362.h" 00019 #include "mbed_debug.h" 00020 00021 // activate / de-activate debug 00022 #define ism_debug false 00023 00024 ISM43362::ISM43362(PinName mosi, PinName miso, PinName sclk, PinName nss, PinName resetpin, PinName datareadypin, PinName wakeup, bool debug) 00025 : _bufferspi(mosi, miso, sclk, nss, datareadypin), _parser(_bufferspi), _resetpin(resetpin), 00026 _packets(0), _packets_end(&_packets) 00027 { 00028 DigitalOut wakeup_pin(wakeup); 00029 ISM43362::setTimeout((uint32_t)5000); 00030 _bufferspi.format(16, 0); /* 16bits, ploarity low, phase 1Edge, master mode */ 00031 _bufferspi.frequency(20000000); /* up to 20 MHz */ 00032 _active_id = 0xFF; 00033 00034 reset(); 00035 00036 _ism_debug = debug || ism_debug; 00037 _parser.debugOn(debug); 00038 } 00039 00040 /** 00041 * @brief Parses and returns number from string. 00042 * @param ptr: pointer to string 00043 * @param cnt: pointer to the number of parsed digit 00044 * @retval integer value. 00045 */ 00046 #define CHARISHEXNUM(x) (((x) >= '0' && (x) <= '9') || \ 00047 ((x) >= 'a' && (x) <= 'f') || \ 00048 ((x) >= 'A' && (x) <= 'F')) 00049 #define CHARISNUM(x) ((x) >= '0' && (x) <= '9') 00050 #define CHAR2NUM(x) ((x) - '0') 00051 00052 00053 extern "C" int32_t ParseNumber(char* ptr, uint8_t* cnt) 00054 { 00055 uint8_t minus = 0, i = 0; 00056 int32_t sum = 0; 00057 00058 if (*ptr == '-') { /* Check for minus character */ 00059 minus = 1; 00060 ptr++; 00061 i++; 00062 } 00063 while (CHARISNUM(*ptr) || (*ptr=='.')) { /* Parse number */ 00064 if (*ptr == '.') { 00065 ptr++; // next char 00066 } else { 00067 sum = 10 * sum + CHAR2NUM(*ptr); 00068 ptr++; 00069 i++; 00070 } 00071 } 00072 00073 if (cnt != NULL) { /* Save number of characters used for number */ 00074 *cnt = i; 00075 } 00076 if (minus) { /* Minus detected */ 00077 return 0 - sum; 00078 } 00079 return sum; /* Return number */ 00080 } 00081 00082 const char *ISM43362::get_firmware_version(void) 00083 { 00084 char tmp_buffer[250]; 00085 char *ptr, *ptr2; 00086 00087 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00088 if(!(_parser.send("I?") && _parser.recv("%[^\n^\r]\r\n", tmp_buffer) && check_response())) { 00089 debug_if(_ism_debug, "ISM43362: get_firmware_version is FAIL\r\n"); 00090 return 0; 00091 } 00092 00093 // Get the first version in the string 00094 ptr = strtok((char *)tmp_buffer, ","); 00095 ptr = strtok(NULL, ","); 00096 ptr2 = strtok(NULL, ","); 00097 if (ptr == NULL) { 00098 debug_if(_ism_debug, "ISM43362: get_firmware_version decoding is FAIL\r\n"); 00099 return 0; 00100 } 00101 strncpy(_fw_version, ptr , ptr2-ptr); 00102 00103 debug_if(_ism_debug, "ISM43362: get_firmware_version = [%s]\r\n", _fw_version); 00104 00105 return _fw_version; 00106 } 00107 00108 bool ISM43362::reset(void) 00109 { 00110 char tmp_buffer[100]; 00111 debug_if(_ism_debug,"ISM43362: Reset Module\r\n"); 00112 _resetpin = 0; 00113 wait_ms(10); 00114 _resetpin = 1; 00115 wait_ms(500); 00116 00117 /* Wait for prompt line : the string is "> ". */ 00118 /* As the space char is not detected by sscanf function in parser.recv, */ 00119 /* we need to use %[\n] */ 00120 if (!_parser.recv(">%[^\n]", tmp_buffer)) { 00121 debug_if(_ism_debug,"ISM43362: Reset Module failed\r\n"); 00122 return false; 00123 } 00124 return true; 00125 } 00126 00127 void ISM43362::print_rx_buff(void) { 00128 char tmp[150] = {0}; 00129 uint16_t i = 0; 00130 debug_if(_ism_debug,"ISM43362: "); 00131 while(i < 150) { 00132 int c = _parser.getc(); 00133 if (c < 0) 00134 break; 00135 tmp[i] = c; 00136 debug_if(_ism_debug,"0x%2X ",c); 00137 i++; 00138 } 00139 debug_if(_ism_debug,"\n"); 00140 debug_if(_ism_debug,"ISM43362: Buffer content =====%s=====\r\n",tmp); 00141 } 00142 00143 /* checks the standard OK response of the WIFI module, shouldbe: 00144 * \r\nDATA\r\nOK\r\n>sp 00145 * or 00146 * \r\nERROR\r\nUSAGE\r\n>sp 00147 * function returns true if OK, false otherwise. In case of error, 00148 * print error content then flush buffer */ 00149 bool ISM43362::check_response(void) 00150 { 00151 char tmp_buffer[100]; 00152 if(!_parser.recv("OK\r\n")) { 00153 print_rx_buff(); 00154 _parser.flush(); 00155 return false; 00156 } 00157 00158 /* Then we should get the prompt: "> " */ 00159 /* As the space char is not detected by sscanf function in parser.recv, */ 00160 /* we need to use %[\n] */ 00161 if (!_parser.recv(">%[^\n]", tmp_buffer)) { 00162 debug_if(_ism_debug, "ISM43362: Missing prompt in WIFI resp\r\n"); 00163 print_rx_buff(); 00164 _parser.flush(); 00165 return false; 00166 } 00167 00168 /* Inventek module do stuffing / padding of data with 0x15, 00169 * in case buffer contains such */ 00170 while(1) { 00171 int c = _parser.getc(); 00172 if ( c == 0x15) { 00173 debug_if(_ism_debug, "ISM43362: Flush char 0x%x\n", c); 00174 continue; 00175 } else { 00176 /* How to put it back if needed ? */ 00177 break; 00178 } 00179 } 00180 return true; 00181 } 00182 00183 bool ISM43362::dhcp(bool enabled) 00184 { 00185 return (_parser.send("C4=%d", enabled ? 1:0) && check_response()); 00186 } 00187 00188 int ISM43362::connect(const char *ap, const char *passPhrase, ism_security_t ap_sec) 00189 { 00190 char tmp[256]; 00191 00192 if (!(_parser.send("C1=%s", ap) && check_response())) { 00193 return NSAPI_ERROR_PARAMETER; 00194 } 00195 00196 if (!(_parser.send("C2=%s", passPhrase) && check_response())) { 00197 return NSAPI_ERROR_PARAMETER; 00198 } 00199 00200 /* Check security level is acceptable */ 00201 if (ap_sec > ISM_SECURITY_WPA_WPA2 ) { 00202 debug_if(_ism_debug, "ISM43362: Unsupported security level %d\n", ap_sec); 00203 return NSAPI_ERROR_UNSUPPORTED; 00204 } 00205 00206 if (!(_parser.send("C3=%d", ap_sec) && check_response())) { 00207 return NSAPI_ERROR_PARAMETER; 00208 } 00209 00210 if(_parser.send("C0")) { 00211 while (_parser.recv("%[^\n]\n", tmp)) { 00212 if(strstr(tmp, "OK")) { 00213 _parser.flush(); 00214 return NSAPI_ERROR_OK; 00215 } 00216 if(strstr(tmp, "JOIN")) { 00217 if(strstr(tmp, "Failed")) { 00218 _parser.flush(); 00219 return NSAPI_ERROR_AUTH_FAILURE; 00220 } 00221 } 00222 } 00223 } 00224 00225 return NSAPI_ERROR_NO_CONNECTION; 00226 } 00227 00228 bool ISM43362::disconnect(void) 00229 { 00230 return (_parser.send("CD") && check_response()); 00231 } 00232 00233 const char *ISM43362::getIPAddress(void) 00234 { 00235 char tmp_ip_buffer[250]; 00236 char *ptr, *ptr2; 00237 00238 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00239 if(!(_parser.send("C?") 00240 && _parser.recv("%[^\n^\r]\r\n", tmp_ip_buffer) 00241 && check_response())) { 00242 debug_if(_ism_debug,"ISM43362: getIPAddress LINE KO: %s\n", tmp_ip_buffer); 00243 return 0; 00244 } 00245 00246 /* Get the IP address in the result */ 00247 /* TODO : check if the begining of the string is always = "eS-WiFi_AP_C47F51011231," */ 00248 ptr = strtok((char *)tmp_ip_buffer, ","); 00249 ptr = strtok(NULL, ","); 00250 ptr = strtok(NULL, ","); 00251 ptr = strtok(NULL, ","); 00252 ptr = strtok(NULL, ","); 00253 ptr = strtok(NULL, ","); 00254 ptr2 = strtok(NULL, ","); 00255 if (ptr == NULL) return 0; 00256 strncpy(_ip_buffer, ptr , ptr2-ptr); 00257 00258 tmp_ip_buffer[59] = 0; 00259 debug_if(_ism_debug,"ISM43362: receivedIPAddress: %s\n", _ip_buffer); 00260 00261 return _ip_buffer; 00262 } 00263 00264 const char *ISM43362::getMACAddress(void) 00265 { 00266 if(!(_parser.send("Z5") && _parser.recv("%s\r\n", _mac_buffer) && check_response())) { 00267 debug_if(_ism_debug,"ISM43362: receivedMacAddress LINE KO: %s\n", _mac_buffer); 00268 return 0; 00269 } 00270 00271 debug_if(_ism_debug,"ISM43362: receivedMacAddress:%s, size=%d\r\n", _mac_buffer, sizeof(_mac_buffer)); 00272 00273 return _mac_buffer; 00274 } 00275 00276 const char *ISM43362::getGateway() 00277 { 00278 char tmp[250]; 00279 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00280 if(!(_parser.send("C?") && _parser.recv("%[^\n^\r]\r\n", tmp) && check_response())) { 00281 debug_if(_ism_debug,"ISM43362: getGateway LINE KO: %s\r\n", tmp); 00282 return 0; 00283 } 00284 00285 /* Extract the Gateway in the received buffer */ 00286 char *ptr; 00287 ptr = strtok(tmp,","); 00288 for (int i = 0; i< 7;i++) { 00289 if (ptr == NULL) break; 00290 ptr = strtok(NULL,","); 00291 } 00292 00293 strncpy(_gateway_buffer, ptr, sizeof(_gateway_buffer)); 00294 00295 debug_if(_ism_debug,"ISM43362: getGateway: %s\r\n", _gateway_buffer); 00296 00297 return _gateway_buffer; 00298 } 00299 00300 const char *ISM43362::getNetmask() 00301 { 00302 char tmp[250]; 00303 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00304 if(!(_parser.send("C?") && _parser.recv("%[^\n^\r]\r\n", tmp) && check_response())) { 00305 debug_if(_ism_debug,"ISM43362: getNetmask LINE KO: %s\n", tmp); 00306 return 0; 00307 } 00308 00309 /* Extract Netmask in the received buffer */ 00310 char *ptr; 00311 ptr = strtok(tmp,","); 00312 for (int i = 0; i< 6;i++) { 00313 if (ptr == NULL) break; 00314 ptr = strtok(NULL,","); 00315 } 00316 00317 strncpy(_netmask_buffer, ptr, sizeof(_netmask_buffer)); 00318 00319 debug_if(_ism_debug,"ISM43362: getNetmask: %s\r\n", _netmask_buffer); 00320 00321 return _netmask_buffer; 00322 } 00323 00324 int8_t ISM43362::getRSSI() 00325 { 00326 int8_t rssi; 00327 char tmp[25]; 00328 00329 if(!(_parser.send("CR") && _parser.recv("%s\r\n", tmp) && check_response())) { 00330 debug_if(_ism_debug,"ISM43362: getRSSI LINE KO: %s\r\n", tmp); 00331 return 0; 00332 } 00333 00334 rssi = ParseNumber(tmp, NULL); 00335 00336 debug_if(_ism_debug,"ISM43362: getRSSI: %d\r\n", rssi); 00337 00338 return rssi; 00339 } 00340 /** 00341 * @brief Parses Security type. 00342 * @param ptr: pointer to string 00343 * @retval Encryption type. 00344 */ 00345 extern "C" nsapi_security_t ParseSecurity(char* ptr) 00346 { 00347 if(strstr(ptr,"Open")) return NSAPI_SECURITY_NONE; 00348 else if(strstr(ptr,"WEP")) return NSAPI_SECURITY_WEP; 00349 else if(strstr(ptr,"WPA2 AES")) return NSAPI_SECURITY_WPA2; 00350 else if(strstr(ptr,"WPA WPA2")) return NSAPI_SECURITY_WPA_WPA2; 00351 else if(strstr(ptr,"WPA2 TKIP")) return NSAPI_SECURITY_UNKNOWN; // no match in mbed 00352 else if(strstr(ptr,"WPA2")) return NSAPI_SECURITY_WPA2; // catch any other WPA2 formula 00353 else if(strstr(ptr,"WPA")) return NSAPI_SECURITY_WPA; 00354 else return NSAPI_SECURITY_UNKNOWN; 00355 } 00356 00357 /** 00358 * @brief Convert char in Hex format to integer. 00359 * @param a: character to convert 00360 * @retval integer value. 00361 */ 00362 extern "C" uint8_t Hex2Num(char a) 00363 { 00364 if (a >= '0' && a <= '9') { /* Char is num */ 00365 return a - '0'; 00366 } else if (a >= 'a' && a <= 'f') { /* Char is lowercase character A - Z (hex) */ 00367 return (a - 'a') + 10; 00368 } else if (a >= 'A' && a <= 'F') { /* Char is uppercase character A - Z (hex) */ 00369 return (a - 'A') + 10; 00370 } 00371 00372 return 0; 00373 } 00374 00375 /** 00376 * @brief Extract a hex number from a string. 00377 * @param ptr: pointer to string 00378 * @param cnt: pointer to the number of parsed digit 00379 * @retval Hex value. 00380 */ 00381 extern "C" uint32_t ParseHexNumber(char* ptr, uint8_t* cnt) 00382 { 00383 uint32_t sum = 0; 00384 uint8_t i = 0; 00385 00386 while (CHARISHEXNUM(*ptr)) { /* Parse number */ 00387 sum <<= 4; 00388 sum += Hex2Num(*ptr); 00389 ptr++; 00390 i++; 00391 } 00392 00393 if (cnt != NULL) { /* Save number of characters used for number */ 00394 *cnt = i; 00395 } 00396 return sum; /* Return number */ 00397 } 00398 00399 bool ISM43362::isConnected(void) 00400 { 00401 return getIPAddress() != 0; 00402 } 00403 00404 int ISM43362::scan(WiFiAccessPoint *res, unsigned limit) 00405 { 00406 unsigned cnt = 0, num=0; 00407 char *ptr; 00408 char tmp[256]; 00409 00410 if(!(_parser.send("F0"))) { 00411 debug_if(_ism_debug,"ISM43362: scan error\r\n"); 00412 return 0; 00413 } 00414 00415 /* Parse the received buffer and fill AP buffer */ 00416 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00417 while (_parser.recv("#%[^\n]\n", tmp)) { 00418 if (limit != 0 && cnt >= limit) { 00419 /* reached end */ 00420 break; 00421 } 00422 nsapi_wifi_ap_t ap = {0}; 00423 debug_if(_ism_debug,"ISM43362: received:%s\n", tmp); 00424 ptr = strtok(tmp, ","); 00425 num = 0; 00426 while (ptr != NULL) { 00427 switch (num++) { 00428 case 0: /* Ignore index */ 00429 case 4: /* Ignore Max Rate */ 00430 case 5: /* Ignore Network Type */ 00431 case 7: /* Ignore Radio Band */ 00432 break; 00433 case 1: 00434 ptr[strlen(ptr) - 1] = 0; 00435 strncpy((char *)ap.ssid, ptr+ 1, 32); 00436 break; 00437 case 2: 00438 for (int i=0; i<6; i++) { 00439 ap.bssid[i] = ParseHexNumber(ptr + (i*3), NULL); 00440 } 00441 break; 00442 case 3: 00443 ap.rssi = ParseNumber(ptr, NULL); 00444 break; 00445 case 6: 00446 ap.security = ParseSecurity(ptr); 00447 break; 00448 case 8: 00449 ap.channel = ParseNumber(ptr, NULL); 00450 num = 1; 00451 break; 00452 default: 00453 break; 00454 } 00455 ptr = strtok(NULL, ","); 00456 } 00457 res[cnt] = WiFiAccessPoint(ap); 00458 cnt++; 00459 } 00460 00461 /* We may stop before having read all the APs list, so flush the rest of 00462 * it as well as OK commands */ 00463 _parser.flush(); 00464 00465 debug_if(_ism_debug, "ISM43362: End of Scan: cnt=%d\n", cnt); 00466 00467 return cnt; 00468 00469 } 00470 00471 bool ISM43362::open(const char *type, int id, const char* addr, int port) 00472 { /* TODO : This is the implementation for the client socket, need to check if need to create openserver too */ 00473 //IDs only 0-3 00474 if((id < 0) ||(id > 3)) { 00475 debug_if(_ism_debug, "ISM43362: open: wrong id\n"); 00476 return false; 00477 } 00478 /* Set communication socket */ 00479 debug_if(_ism_debug, "ISM43362: OPEN socket\n"); 00480 _active_id = id; 00481 if (!(_parser.send("P0=%d", id) && check_response())) { 00482 return false; 00483 } 00484 /* Set protocol */ 00485 if (!(_parser.send("P1=%s", type) && check_response())) { 00486 return false; 00487 } 00488 /* Set address */ 00489 if (!(_parser.send("P3=%s", addr) && check_response())) { 00490 return false; 00491 } 00492 if (!(_parser.send("P4=%d", port) && check_response())) { 00493 return false; 00494 } 00495 /* Start client */ 00496 if (!(_parser.send("P6=1") && check_response())) { 00497 return false; 00498 } 00499 00500 /* request as much data as possible - i.e. module max size */ 00501 if (!(_parser.send("R1=%d", ES_WIFI_MAX_RX_PACKET_SIZE)&& check_response())) { 00502 return -1; 00503 } 00504 00505 return true; 00506 } 00507 00508 bool ISM43362::dns_lookup(const char* name, char* ip) 00509 { 00510 char tmp[30]; 00511 00512 if (!(_parser.send("D0=%s", name) && _parser.recv("%s\r\n", tmp) 00513 && check_response())) { 00514 debug_if(_ism_debug,"ISM43362: dns_lookup LINE KO: %s\n", tmp); 00515 return 0; 00516 } 00517 00518 strncpy(ip, tmp, sizeof(tmp)); 00519 00520 debug_if(_ism_debug, "ISM43362: ip of DNSlookup: %s\n", ip); 00521 return 1; 00522 } 00523 00524 bool ISM43362::send(int id, const void *data, uint32_t amount) 00525 { 00526 // The Size limit has to be checked on caller side. 00527 if (amount > ES_WIFI_MAX_RX_PACKET_SIZE) { 00528 return false; 00529 } 00530 00531 /* Activate the socket id in the wifi module */ 00532 if ((id < 0) ||(id > 3)) { 00533 return false; 00534 } 00535 debug_if(_ism_debug, "ISM43362: SEND socket amount %d\n", amount); 00536 if (_active_id != id) { 00537 _active_id = id; 00538 if (!(_parser.send("P0=%d",id) && check_response())) { 00539 return false; 00540 } 00541 } 00542 00543 /* Change the write timeout */ 00544 if (!(_parser.send("S2=%d", _timeout) && check_response())) { 00545 return false; 00546 } 00547 /* set Write Transport Packet Size */ 00548 int i = _parser.printf("S3=%d\r", (int)amount); 00549 if (i < 0) { 00550 return false; 00551 } 00552 i = _parser.write((const char *)data, amount, i); 00553 if (i < 0) { 00554 return false; 00555 } 00556 00557 if (!check_response()) { 00558 return false; 00559 } 00560 00561 return true; 00562 } 00563 00564 int ISM43362::check_recv_status(int id, void *data) 00565 { 00566 int read_amount; 00567 static int keep_to = 0; 00568 00569 debug_if(_ism_debug, "ISM43362: ISM43362 req check_recv_status\r\n"); 00570 /* Activate the socket id in the wifi module */ 00571 if ((id < 0) ||(id > 3)) { 00572 return -1; 00573 } 00574 00575 if (_active_id != id) { 00576 _active_id = id; 00577 if (!(_parser.send("P0=%d",id) && check_response())) { 00578 return -1; 00579 } 00580 } 00581 00582 00583 /* MBED wifi driver is meant to be non-blocking, but we need anyway to 00584 * wait for some data on the RECV side to avoid overflow on TX side, the 00585 * tiemout is defined in higher layer */ 00586 if (keep_to != _timeout) { 00587 if (!(_parser.send("R2=%d", _timeout) && check_response())) { 00588 return -1; 00589 } 00590 keep_to = _timeout; 00591 } 00592 00593 if (!_parser.send("R0")) { 00594 return -1; 00595 } 00596 read_amount = _parser.read((char *)data); 00597 00598 if(read_amount < 0) { 00599 debug_if(_ism_debug, "ISM43362: ERROR in data RECV, timeout?\r\n"); 00600 return -1; /* nothing to read */ 00601 } 00602 00603 /* If there are spurious 0x15 at the end of the data, this is an error 00604 * we hall can get rid off of them :-( 00605 * This should not happen, but let's try to clean-up anyway 00606 */ 00607 char *cleanup = (char *) data; 00608 while ((read_amount > 0) && (cleanup[read_amount-1] == 0x15)) { 00609 debug_if(_ism_debug, "ISM43362: spurious 0X15 trashed\r\n"); 00610 /* Remove the trailling char then search again */ 00611 read_amount--; 00612 } 00613 00614 if ((read_amount >= 6) && (strncmp("OK\r\n> ", (char *)data, 6) == 0)) { 00615 debug_if(_ism_debug, "ISM43362: recv 2 nothing to read=%d\r\n", read_amount); 00616 return 0; /* nothing to read */ 00617 } else if ((read_amount >= 8) && (strncmp((char *)((uint32_t) data + read_amount - 8), "\r\nOK\r\n> ", 8)) == 0) { 00618 /* bypass ""\r\nOK\r\n> " if present at the end of the chain */ 00619 read_amount -= 8; 00620 } else { 00621 debug_if(_ism_debug, "ISM43362: ERROR in data RECV?, flushing %d bytes\r\n", read_amount); 00622 int i = 0; 00623 debug_if(_ism_debug, "ISM43362: "); 00624 for (i = 0; i < read_amount; i++) { 00625 debug_if(_ism_debug, "%2X ", cleanup[i]); 00626 } 00627 cleanup[i] = 0; 00628 debug_if(_ism_debug, "\r\n%s\r\n", cleanup); 00629 return -1; /* nothing to read */ 00630 } 00631 00632 debug_if(_ism_debug, "ISM43362: read_amount=%d\r\n", read_amount); 00633 return read_amount; 00634 } 00635 00636 bool ISM43362::close(int id) 00637 { 00638 if ((id <0) || (id > 3)) { 00639 debug_if(_ism_debug,"ISM43362: Wrong socket number\n"); 00640 return false; 00641 } 00642 /* Set connection on this socket */ 00643 debug_if(_ism_debug,"ISM43362: CLOSE socket id=%d\n", id); 00644 _active_id = id; 00645 if (!(_parser.send("P0=%d", id) && check_response())) { 00646 return false; 00647 } 00648 /* close this socket */ 00649 if (!(_parser.send("P6=0") && check_response())) { 00650 return false; 00651 } 00652 return true; 00653 } 00654 00655 void ISM43362::setTimeout(uint32_t timeout_ms) 00656 { 00657 _timeout = timeout_ms; 00658 _parser.setTimeout(timeout_ms); 00659 } 00660 00661 bool ISM43362::readable() 00662 { 00663 /* not applicable with SPI api */ 00664 return true; 00665 } 00666 00667 bool ISM43362::writeable() 00668 { 00669 /* not applicable with SPI api */ 00670 return true; 00671 } 00672 00673 void ISM43362::attach(Callback<void()> func) 00674 { 00675 /* not applicable with SPI api */ 00676 } 00677
Generated on Tue Jul 12 2022 16:22:06 by
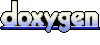