
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
FileSystemInit.cpp
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "pal.h" 00017 #include "mbed.h" 00018 #include "FATFileSystem.h" 00019 #include "SDBlockDevice.h" 00020 00021 #include "MBRBlockDevice.h" 00022 00023 #ifndef PRIMARY_PARTITION_NUMBER 00024 #define PRIMARY_PARTITION_NUMBER 1 00025 #endif 00026 00027 #ifndef PRIMARY_PARTITION_START 00028 #define PRIMARY_PARTITION_START 0 00029 #endif 00030 00031 #ifndef PRIMARY_PARTITION_SIZE 00032 #define PRIMARY_PARTITION_SIZE 512*1024 00033 #endif 00034 00035 #ifndef SECONDARY_PARTITION_NUMBER 00036 #define SECONDARY_PARTITION_NUMBER 2 00037 #endif 00038 00039 #ifndef SECONDARY_PARTITION_START 00040 #define SECONDARY_PARTITION_START PRIMARY_PARTITION_SIZE 00041 #endif 00042 00043 #ifndef SECONDARY_PARTITION_SIZE 00044 #define SECONDARY_PARTITION_SIZE PRIMARY_PARTITION_SIZE 00045 #endif 00046 00047 /*MBED_LIBRARY_VERSION 129 stands for mbed-os 5.2.2*/ 00048 #ifdef MBED_LIBRARY_VERSION 00049 #if (MBED_LIBRARY_VERSION < 129) 00050 #endif 00051 #endif //MBED_LIBRARY_VERSION 00052 00053 00054 //uncomment this to create the partitions 00055 #define PAL_EXAMPLE_GENERATE_PARTITION 1 00056 00057 // 00058 // See the mbed_lib.json in the sd-driver library for the definitions. 00059 // See the sd-driver library README.md for details with CI-shield etc. 00060 // Add also new boards/exceptions there rather than in code directly 00061 // OR 00062 // alternatively overload via your mbed_app.json (MBED_CONF_APP...) 00063 // 00064 00065 #if defined (MBED_CONF_APP_SPI_MOSI) && defined (MBED_CONF_APP_SPI_MISO) && defined (MBED_CONF_APP_SPI_CLK) && defined (MBED_CONF_APP_SPI_CS) 00066 SDBlockDevice sd(MBED_CONF_APP_SPI_MOSI, MBED_CONF_APP_SPI_MISO, MBED_CONF_APP_SPI_CLK, MBED_CONF_APP_SPI_CS); 00067 #else 00068 SDBlockDevice sd(MBED_CONF_SD_SPI_MOSI, MBED_CONF_SD_SPI_MISO, MBED_CONF_SD_SPI_CLK, MBED_CONF_SD_SPI_CS); 00069 #endif 00070 00071 //This trick (the adding of 1) is to skip the '/' that is needed for FS but not needed to init 00072 FATFileSystem fat1(((char*)PAL_FS_MOUNT_POINT_PRIMARY+1)); 00073 00074 #if (PAL_NUMBER_OF_PARTITIONS > 0) 00075 MBRBlockDevice part1(&sd,1); 00076 #if (PAL_NUMBER_OF_PARTITIONS == 2) 00077 MBRBlockDevice part2(&sd,2); 00078 //This trick (the adding of 1) is to skip the '/' that is needed for FS but not needed to init 00079 FATFileSystem fat2(((char*)PAL_FS_MOUNT_POINT_SECONDARY+1)); 00080 #endif 00081 #endif 00082 00083 00084 static int initPartition(uint8_t partitionNumber, BlockDevice* bd,FATFileSystem* fs) 00085 { 00086 int err = bd->init(); 00087 if (err < 0) 00088 { 00089 printf("Failed to initialize 1st partition cause %d\r\n",err); 00090 #ifdef PAL_EXAMPLE_GENERATE_PARTITION 00091 printf("Trying to create the partition\r\n"); 00092 if (PRIMARY_PARTITION_NUMBER == partitionNumber) 00093 { 00094 err = MBRBlockDevice::partition(&sd, PRIMARY_PARTITION_NUMBER, 0x83, PRIMARY_PARTITION_START, PRIMARY_PARTITION_START + PRIMARY_PARTITION_SIZE); 00095 } 00096 else if (SECONDARY_PARTITION_NUMBER == partitionNumber) 00097 { 00098 err = MBRBlockDevice::partition(&sd, SECONDARY_PARTITION_NUMBER, 0x83, SECONDARY_PARTITION_START, SECONDARY_PARTITION_START + SECONDARY_PARTITION_SIZE); 00099 } 00100 else 00101 { 00102 printf("Wrong partition number %d\r\n",partitionNumber); 00103 err = -1; 00104 } 00105 if (err < 0) 00106 { 00107 printf("Failed to create the partition cause %d\r\n",err); 00108 } 00109 else 00110 { 00111 err = bd->init(); 00112 } 00113 #endif 00114 } 00115 if (!err) 00116 { 00117 err = fs->mount(bd); 00118 if (err < 0) 00119 { 00120 err = FATFileSystem::format(bd); 00121 if (err < 0) 00122 { 00123 printf("failed to format part cause %d\r\n",err); 00124 } 00125 else 00126 { 00127 err = fs->mount(bd); 00128 if (err < 0) 00129 { 00130 printf("failed to mount cause %d\r\n",err); 00131 } 00132 } 00133 } 00134 } 00135 return err; 00136 } 00137 00138 int initSDcardAndFileSystem(void) 00139 { 00140 printf("Initializing the file system\r\n"); 00141 #if (PAL_NUMBER_OF_PARTITIONS == 0 ) 00142 int err = initPartition(0, &sd, &fat1); 00143 if (err < 0) 00144 { 00145 printf("Failed to initialize primary partition\r\n"); 00146 } 00147 #elif (PAL_NUMBER_OF_PARTITIONS > 0) // create and mount an additional partition 00148 int err = initPartition(PRIMARY_PARTITION_NUMBER, &part1,&fat1); 00149 if (err < 0) 00150 { 00151 printf("Failed to initialize primary partition\r\n"); 00152 } 00153 #if (PAL_NUMBER_OF_PARTITIONS == 2) 00154 else 00155 { 00156 err = initPartition(SECONDARY_PARTITION_NUMBER, &part2,&fat2); 00157 if (err < 0) 00158 { 00159 printf("Failed to initialize secondary partition\r\n"); 00160 } 00161 } 00162 #endif 00163 #endif 00164 return err; 00165 } 00166
Generated on Tue Jul 12 2022 16:22:05 by
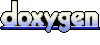