
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
DeviceMetadataResource.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "update-client-lwm2m/DeviceMetadataResource.h" 00020 00021 #include "update-client-common/arm_uc_common.h" 00022 #include "pal4life-device-identity/pal_device_identity.h" 00023 00024 #include <stdio.h> 00025 00026 #define ARM_UCS_LWM2M_INTERNAL_ERROR (-1) 00027 #define ARM_UCS_LWM2M_INTERNAL_SUCCESS (0) 00028 00029 namespace DeviceMetadataResource { 00030 00031 /* LWM2M Firmware Update Object */ 00032 static M2MObject* deviceMetadataObject = NULL; 00033 00034 /* LWM2M Firmware Update Object resources */ 00035 static M2MResource* protocolSupportedResource = NULL; // /10255/0/0 00036 static M2MResource* bootloaderHashResource = NULL; // /10255/0/1 00037 static M2MResource* OEMBootloaderHashResource = NULL; // /10255/0/2 00038 static M2MResource* vendorIdResource = NULL; // /10255/0/3 00039 static M2MResource* classIdResource = NULL; // /10255/0/4 00040 static M2MResource* deviceIdResource = NULL; // /10255/0/5 00041 } 00042 00043 00044 /** 00045 * @brief Initialize LWM2M Device Metadata Object 00046 * @details Sets up LWM2M object with accompanying resources. 00047 */ 00048 void DeviceMetadataResource::Initialize(void) 00049 { 00050 static bool initialized = false; 00051 00052 if (!initialized) 00053 { 00054 initialized = true; 00055 00056 /* The LWM2M Firmware Update Object is at /10255 */ 00057 deviceMetadataObject = M2MInterfaceFactory::create_object("10255"); 00058 00059 if (deviceMetadataObject) 00060 { 00061 /* Set object operating mode to GET_ALLOWED */ 00062 deviceMetadataObject->set_operation(M2MBase::GET_ALLOWED); 00063 /* Create first (and only) instance /10255/0 */ 00064 M2MObjectInstance* deviceMetadataInstance = deviceMetadataObject->create_object_instance(); 00065 00066 if (deviceMetadataInstance) 00067 { 00068 /* Default values are non-standard, but the standard has no 00069 values for indicating that the device is initializing. 00070 */ 00071 int64_t defaultVersion = 1; 00072 const uint8_t invalid_value[] = "INVALID"; 00073 const uint8_t invalid_value_size = sizeof(invalid_value) - 1; 00074 00075 arm_uc_error_t err = { .code = ERR_INVALID_PARAMETER }; 00076 arm_uc_guid_t guid = { 0 }; 00077 uint8_t* value = NULL; 00078 uint32_t value_length = 0; 00079 00080 /* Set instance operating mode to GET_ALLOWED */ 00081 deviceMetadataInstance->set_operation(M2MBase::GET_ALLOWED); 00082 00083 /* Create Update resource /10255/0/0 */ 00084 protocolSupportedResource = deviceMetadataInstance->create_dynamic_resource( 00085 "0", 00086 "ProtocolSupported", 00087 M2MResourceInstance::INTEGER, 00088 true); 00089 if (protocolSupportedResource) 00090 { 00091 protocolSupportedResource->set_operation(M2MBase::GET_ALLOWED); 00092 protocolSupportedResource->set_value(defaultVersion); 00093 } 00094 00095 /* Create Update resource /10255/0/1 */ 00096 bootloaderHashResource = deviceMetadataInstance->create_static_resource( 00097 "1", 00098 "BootloaderHash", 00099 M2MResourceInstance::OPAQUE, 00100 (uint8_t *) invalid_value, 00101 invalid_value_size); 00102 if (bootloaderHashResource) 00103 { 00104 bootloaderHashResource->set_operation(M2MBase::GET_ALLOWED); 00105 } 00106 00107 /* Create Update resource /10255/0/2 */ 00108 OEMBootloaderHashResource = deviceMetadataInstance->create_static_resource( 00109 "2", 00110 "OEMBootloaderHash", 00111 M2MResourceInstance::OPAQUE, 00112 (uint8_t *) invalid_value, 00113 invalid_value_size); 00114 if (OEMBootloaderHashResource) 00115 { 00116 OEMBootloaderHashResource->set_operation(M2MBase::GET_ALLOWED); 00117 } 00118 00119 /* get vendor ID */ 00120 err = pal_getVendorGuid(&guid); 00121 if (err.error == ERR_NONE) 00122 { 00123 value = (uint8_t *) &guid; 00124 value_length = sizeof(arm_uc_guid_t); 00125 } 00126 else 00127 { 00128 value = (uint8_t *) invalid_value; 00129 value_length = invalid_value_size; 00130 } 00131 00132 /* Create Update resource /10255/0/3 */ 00133 vendorIdResource = deviceMetadataInstance->create_dynamic_resource( 00134 "3", 00135 "Vendor", 00136 M2MResourceInstance::OPAQUE, 00137 true); 00138 00139 if (vendorIdResource) 00140 { 00141 vendorIdResource->set_operation(M2MBase::GET_ALLOWED); 00142 vendorIdResource->set_value(value, value_length); 00143 } 00144 00145 /* get class ID */ 00146 err = pal_getClassGuid(&guid); 00147 if (err.error == ERR_NONE) 00148 { 00149 value = (uint8_t *) &guid; 00150 value_length = sizeof(arm_uc_guid_t); 00151 } 00152 else 00153 { 00154 value = (uint8_t *) invalid_value; 00155 value_length = invalid_value_size; 00156 } 00157 00158 /* Create Update resource /10255/0/4 */ 00159 classIdResource = deviceMetadataInstance->create_dynamic_resource( 00160 "4", 00161 "Class", 00162 M2MResourceInstance::OPAQUE, 00163 true); 00164 00165 if (classIdResource) 00166 { 00167 classIdResource->set_operation(M2MBase::GET_ALLOWED); 00168 classIdResource->set_value(value, value_length); 00169 } 00170 00171 /* get device ID */ 00172 err = pal_getDeviceGuid(&guid); 00173 if (err.error == ERR_NONE) 00174 { 00175 value = (uint8_t *) &guid; 00176 value_length = sizeof(arm_uc_guid_t); 00177 } 00178 else 00179 { 00180 value = (uint8_t *) invalid_value; 00181 value_length = invalid_value_size; 00182 } 00183 00184 /* Create Update resource /10255/0/5 */ 00185 deviceIdResource = deviceMetadataInstance->create_static_resource( 00186 "5", 00187 "DeviceId", 00188 M2MResourceInstance::OPAQUE, 00189 value, 00190 value_length); 00191 if (deviceIdResource) 00192 { 00193 deviceIdResource->set_operation(M2MBase::GET_ALLOWED); 00194 } 00195 } 00196 } 00197 } 00198 } 00199 00200 int32_t DeviceMetadataResource::setBootloaderHash(arm_uc_buffer_t* hash) 00201 { 00202 UC_SRCE_TRACE("DeviceMetadataResource::setBootloaderHash ptr %p size %u", hash, hash->size); 00203 00204 int32_t result = ARM_UCS_LWM2M_INTERNAL_ERROR; 00205 00206 if (bootloaderHashResource && hash && hash->size > 0) 00207 { 00208 bool rt = bootloaderHashResource->set_value(hash->ptr, hash->size); 00209 if (rt == true) 00210 { 00211 result = ARM_UCS_LWM2M_INTERNAL_SUCCESS; 00212 } 00213 } 00214 00215 return result; 00216 } 00217 00218 int32_t DeviceMetadataResource::setOEMBootloaderHash(arm_uc_buffer_t* hash) 00219 { 00220 UC_SRCE_TRACE("DeviceMetadataResource::setOEMBootloaderHash ptr %p size %u", hash, hash->size); 00221 00222 int32_t result = ARM_UCS_LWM2M_INTERNAL_ERROR; 00223 00224 if (OEMBootloaderHashResource && hash && hash->size > 0) 00225 { 00226 bool rt = OEMBootloaderHashResource->set_value(hash->ptr, hash->size); 00227 if (rt == true) 00228 { 00229 result = ARM_UCS_LWM2M_INTERNAL_SUCCESS; 00230 } 00231 } 00232 00233 return result; 00234 } 00235 00236 M2MObject* DeviceMetadataResource::getObject() 00237 { 00238 Initialize(); 00239 00240 return deviceMetadataObject; 00241 }
Generated on Tue Jul 12 2022 16:22:04 by
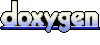