
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
ConnectorClient.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef __CONNECTOR_CLIENT_H__ 00020 #define __CONNECTOR_CLIENT_H__ 00021 00022 #include "mbed-client/functionpointer.h" 00023 #include "mbed-client/m2minterfacefactory.h" 00024 #include "mbed-client/m2mdevice.h" 00025 #include "mbed-client/m2minterfaceobserver.h" 00026 #include "mbed-client/m2minterface.h" 00027 #include "mbed-client/m2mobjectinstance.h" 00028 #include "mbed-client/m2mresource.h" 00029 #include "mbed-client/m2mtimerobserver.h" 00030 #include "mbed-client/m2mtimer.h" 00031 #include "include/CloudClientStorage.h" 00032 00033 class ConnectorClientCallback; 00034 00035 using namespace std; 00036 00037 00038 /** 00039 * \brief ConnectorClientEndpointInfo 00040 * A structure that contains the needed endpoint information to register with the Cloud service. 00041 * Note: this should be changed to a class instead of struct and/or members changed to "const char*". 00042 */ 00043 struct ConnectorClientEndpointInfo { 00044 00045 public: 00046 ConnectorClientEndpointInfo(M2MSecurity::SecurityModeType m) : mode(m) {}; 00047 ~ConnectorClientEndpointInfo() {}; 00048 00049 public: 00050 00051 String endpoint_name; 00052 String account_id; 00053 String internal_endpoint_name; 00054 M2MSecurity::SecurityModeType mode; 00055 }; 00056 00057 /** 00058 * \brief ConnectorClient 00059 * This class is an interface towards the M2MInterface client to handle all 00060 * data flow towards Connector through this client. 00061 * This class is intended to be used via ServiceClient, not directly. 00062 * This class contains also the bootstrap functionality. 00063 */ 00064 class ConnectorClient : public M2MInterfaceObserver, public M2MTimerObserver { 00065 00066 public: 00067 /** 00068 * \brief An enum defining the different states of 00069 * ConnectorClient during the client flow. 00070 */ 00071 enum StartupSubStateRegistration { 00072 State_Bootstrap_Start, 00073 State_Bootstrap_Started, 00074 State_Bootstrap_Success, 00075 State_Bootstrap_Failure, 00076 State_Registration_Start, 00077 State_Registration_Started, 00078 State_Registration_Success, 00079 State_Registration_Failure, 00080 State_Registration_Updated, 00081 State_Unregistered 00082 }; 00083 00084 public: 00085 00086 /** 00087 * \brief Constructor. 00088 * \param callback, A callback for the status from ConnectorClient. 00089 */ 00090 ConnectorClient(ConnectorClientCallback* callback); 00091 00092 /** 00093 * \brief Destructor. 00094 */ 00095 ~ConnectorClient(); 00096 00097 /** 00098 * \brief Starts the bootstrap sequence from the Service Client. 00099 */ 00100 void start_bootstrap(); 00101 00102 /** 00103 * \brief Starts the registration sequence from the Service Client. 00104 * \param client_objs, A list of objects to be registered with Cloud. 00105 */ 00106 void start_registration(M2MObjectList* client_objs); 00107 00108 /** 00109 * \brief Sends an update registration message to the LWM2M server. 00110 */ 00111 void update_registration(); 00112 00113 /** 00114 * \brief Returns the M2MInterface handler. 00115 * \return M2MInterface, Handled for M2MInterface. 00116 */ 00117 M2MInterface * m2m_interface(); 00118 00119 /** 00120 * \brief Checks whether to use Bootstrap or direct Connector mode. 00121 * \return True if bootstrap mode, False if direct Connector flow 00122 */ 00123 bool use_bootstrap(); 00124 00125 /** 00126 * \brief Checks whether to go connector registration flow 00127 * \return True if connector credentials available otherwise false. 00128 */ 00129 bool connector_credentials_available(); 00130 00131 /** 00132 * \brief A utility function to generate the key name. 00133 * \param key, The key to get the value for. 00134 * \param endpoint, The name of the endpoint to be appended 00135 * to the key. 00136 * \param key_name, The [OUT] final key name. 00137 * \return True if available, else false. 00138 */ 00139 bool get_key(const char *key, const char *endpoint, char *&key_name); 00140 00141 /** 00142 * \brief Returns pointer to the ConnectorClientEndpointInfo object. 00143 * \return ConnectorClientEndpointInfo pointer. 00144 */ 00145 const ConnectorClientEndpointInfo *endpoint_info() const; 00146 00147 public: 00148 // implementation of M2MInterfaceObserver: 00149 00150 /** 00151 * \brief A callback indicating that the bootstap has been performed successfully. 00152 * \param server_object, The server object that contains the information fetched 00153 * about the LWM2M server from the bootstrap server. This object can be used 00154 * to register with the LWM2M server. The object ownership is passed. 00155 */ 00156 virtual void bootstrap_done(M2MSecurity *server_object); 00157 00158 /** 00159 * \brief A callback indicating that the device object has been registered 00160 * successfully with the LWM2M server. 00161 * \param security_object, The server object on which the device object is 00162 * registered. The object ownership is passed. 00163 * \param server_object, An object containing information about the LWM2M server. 00164 * The client maintains the object. 00165 */ 00166 virtual void object_registered(M2MSecurity *security_object, const M2MServer &server_object); 00167 00168 /** 00169 * \brief A callback indicating that the device object has been successfully unregistered 00170 * from the LWM2M server. 00171 * \param server_object, The server object from which the device object is 00172 * unregistered. The object ownership is passed. 00173 */ 00174 virtual void object_unregistered(M2MSecurity *server_object); 00175 00176 /** 00177 * \brief A callback indicating that the device object registration has been successfully 00178 * updated on the LWM2M server. 00179 * \param security_object, The server object on which the device object registration is 00180 * updated. The object ownership is passed. 00181 * \param server_object, An object containing information about the LWM2M server. 00182 * The client maintains the object. 00183 */ 00184 virtual void registration_updated(M2MSecurity *security_object, const M2MServer & server_object); 00185 00186 /** 00187 * \brief A callback indicating that there was an error during the operation. 00188 * \param error, An error code for the occurred error. 00189 */ 00190 virtual void error(M2MInterface::Error error); 00191 00192 /** 00193 * \brief A callback indicating that the value of the resource object is updated by the server. 00194 * \param base, The object whose value is updated. 00195 * \param type, The type of the object. 00196 */ 00197 virtual void value_updated(M2MBase *base, M2MBase::BaseType type); 00198 00199 protected: // from M2MTimerObserver 00200 00201 virtual void timer_expired(M2MTimerObserver::Type type); 00202 00203 private: 00204 /** 00205 * \brief Redirects the state machine to right function. 00206 * \param current_state, The current state to be set. 00207 * \param data, The data to be passed to the state function. 00208 */ 00209 void state_function(StartupSubStateRegistration current_state); 00210 00211 /** 00212 * \brief The state engine maintaining the state machine logic. 00213 */ 00214 void state_engine(void); 00215 00216 /** 00217 * \brief An internal event generated by the state machine. 00218 * \param new_state, The new state to which the state machine should go. 00219 * \param data, The data to be passed to the state machine. 00220 */ 00221 void internal_event(StartupSubStateRegistration new_state); 00222 00223 /** 00224 * When the bootstrap starts. 00225 */ 00226 void state_bootstrap_start(); 00227 00228 /** 00229 * When the bootstrap is started. 00230 */ 00231 void state_bootstrap_started(); 00232 00233 /** 00234 * When the bootstrap is successful. 00235 */ 00236 void state_bootstrap_success(); 00237 00238 /** 00239 * When the bootstrap failed. 00240 */ 00241 void state_bootstrap_failure(); 00242 00243 /** 00244 * When the registration starts. 00245 */ 00246 void state_registration_start(); 00247 00248 /** 00249 * When the registration started. 00250 */ 00251 void state_registration_started(); 00252 00253 /** 00254 * When the registration is successful. 00255 */ 00256 void state_registration_success(); 00257 00258 /** 00259 * When the registration failed. 00260 */ 00261 void state_registration_failure(); 00262 00263 /** 00264 * When the client is unregistered. 00265 */ 00266 void state_unregistered(); 00267 00268 /** 00269 * \brief A utility function to create an M2MSecurity object 00270 * for registration. 00271 */ 00272 void create_register_object(); 00273 00274 /** 00275 * \brief A utility function to create an M2MSecurity object 00276 * for bootstrap. 00277 */ 00278 bool create_bootstrap_object(); 00279 00280 /** 00281 * \brief A utility function to set the connector credentials 00282 * in storage. This includes endpoint, domain, connector URI 00283 * and certificates. 00284 * \param security, The Connector certificates. 00285 */ 00286 ccs_status_e set_connector_credentials(M2MSecurity *security); 00287 00288 /** 00289 * \brief A utility function to set the bootstrap credentials 00290 * in storage. This includes Bootstrap URI and certificates. 00291 * \param security, The Bootstrap certificates. 00292 */ 00293 ccs_status_e set_bootstrap_credentials(M2MSecurity *security); 00294 00295 /** 00296 * \brief A utility function to set the bootstrap address in storage. 00297 * \param security, The bootstrap security object containing the address. 00298 */ 00299 ccs_status_e store_bootstrap_address(M2MSecurity *security); 00300 00301 /** 00302 * \brief A utility function to check whether bootstrap credentials are stored in KCM. 00303 */ 00304 bool bootstrap_credentials_stored_in_kcm(); 00305 00306 /** 00307 * \brief A utility function to check whether first to claim feature is configured. 00308 */ 00309 bool is_first_to_claim(); 00310 00311 /** 00312 * \brief A utility function to clear the first to claim parameter in storage. 00313 */ 00314 ccs_status_e clear_first_to_claim(); 00315 00316 /** 00317 * \brief Returns the binding mode selected by the client 00318 * through the configuration. 00319 * \return Binding mode of the client. 00320 */ 00321 static M2MInterface::BindingMode transport_mode(); 00322 00323 private: 00324 // A callback to be called after the sequence is complete. 00325 ConnectorClientCallback* _callback; 00326 StartupSubStateRegistration _current_state; 00327 bool _event_generated; 00328 bool _state_engine_running; 00329 M2MInterface *_interface; 00330 M2MSecurity *_security; 00331 ConnectorClientEndpointInfo _endpoint_info; 00332 M2MObjectList *_client_objs; 00333 M2MTimer _rebootstrap_timer; 00334 uint16_t _bootstrap_security_instance; 00335 uint16_t _lwm2m_security_instance; 00336 }; 00337 00338 /** 00339 * \brief ConnectorClientCallback 00340 * A callback class for passing the client progress and error condition to the 00341 * ServiceClient class object. 00342 */ 00343 class ConnectorClientCallback { 00344 public: 00345 00346 /** 00347 * \brief Indicates that the registration or unregistration operation is complete 00348 * with success or failure. 00349 * \param status, Indicates success or failure in terms of status code. 00350 */ 00351 virtual void registration_process_result(ConnectorClient::StartupSubStateRegistration status) = 0; 00352 00353 /** 00354 * \brief Indicates the Connector error condition of an underlying M2MInterface client. 00355 * \param error, Indicates an error code translated from M2MInterface::Error. 00356 * \param reason, Indicates human readable text for error description. 00357 */ 00358 virtual void connector_error(M2MInterface::Error error, const char *reason) = 0; 00359 00360 /** 00361 * \brief A callback indicating that the value of the resource object is updated 00362 * by the LWM2M Cloud server. 00363 * \param base, The object whose value is updated. 00364 * \param type, The type of the object. 00365 */ 00366 virtual void value_updated(M2MBase *base, M2MBase::BaseType type) = 0; 00367 }; 00368 00369 #endif // !__CONNECTOR_CLIENT_H__
Generated on Tue Jul 12 2022 16:22:04 by
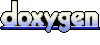