
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
ATParser.h
00001 /* Copyright (c) 2015 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 * 00015 * @section DESCRIPTION 00016 * 00017 * Parser for the AT command syntax 00018 * 00019 */ 00020 #ifndef AT_PARSER_H 00021 #define AT_PARSER_H 00022 00023 #include "mbed.h" 00024 #include <cstdarg> 00025 #include <vector> 00026 #include "BufferedSpi.h" 00027 #include "Callback.h" 00028 00029 00030 /** 00031 * Parser class for parsing AT commands 00032 * 00033 * Here are some examples: 00034 * @code 00035 * ATParser at = ATParser(serial, "\r\n"); 00036 * int value; 00037 * char buffer[100]; 00038 * 00039 * at.send("AT") && at.recv("OK"); 00040 * at.send("AT+CWMODE=%d", 3) && at.recv("OK"); 00041 * at.send("AT+CWMODE?") && at.recv("+CWMODE:%d\r\nOK", &value); 00042 * at.recv("+IPD,%d:", &value); 00043 * at.read(buffer, value); 00044 * at.recv("OK"); 00045 * @endcode 00046 */ 00047 class ATParser 00048 { 00049 private: 00050 // Serial information 00051 BufferedSpi *_serial_spi; 00052 int _buffer_size; 00053 char *_buffer; 00054 Mutex _bufferMutex; 00055 volatile int _timeout; 00056 00057 // Parsing information 00058 const char *_delimiter; 00059 int _delim_size; 00060 char _in_prev; 00061 bool dbg_on; 00062 volatile bool _aborted; 00063 00064 struct oob { 00065 unsigned len; 00066 const char *prefix; 00067 mbed::Callback<void()> cb; 00068 oob *next; 00069 }; 00070 oob *_oobs; 00071 00072 public: 00073 /** 00074 * Constructor 00075 * 00076 * @param serial spi interface to use for AT commands 00077 * @param buffer_size size of internal buffer for transaction 00078 * @param timeout timeout of the connection 00079 * @param delimiter string of characters to use as line delimiters 00080 */ 00081 ATParser(BufferedSpi &serial_spi, const char *delimiter = "\r\n", int buffer_size = 1440, int timeout = 8000, bool debug = false) : 00082 _serial_spi(&serial_spi), 00083 _buffer_size(buffer_size), _in_prev(0), _oobs(NULL) 00084 { 00085 _buffer = new char[buffer_size]; 00086 setTimeout(timeout); 00087 setDelimiter(delimiter); 00088 debugOn(debug); 00089 } 00090 00091 /** 00092 * Destructor 00093 */ 00094 ~ATParser() 00095 { 00096 while (_oobs) { 00097 struct oob *oob = _oobs; 00098 _oobs = oob->next; 00099 delete oob; 00100 } 00101 delete[] _buffer; 00102 } 00103 00104 /** 00105 * Allows timeout to be changed between commands 00106 * 00107 * @param timeout timeout of the connection 00108 */ 00109 void setTimeout(int timeout) 00110 { 00111 _timeout = timeout; 00112 _serial_spi->setTimeout(timeout); 00113 } 00114 00115 /** 00116 * Sets string of characters to use as line delimiters 00117 * 00118 * @param delimiter string of characters to use as line delimiters 00119 */ 00120 void setDelimiter(const char *delimiter) { 00121 _delimiter = delimiter; 00122 _delim_size = strlen(delimiter); 00123 } 00124 00125 /** 00126 * Allows echo to be on or off 00127 * 00128 * @param echo 1 for echo and 0 turns it off 00129 */ 00130 void debugOn(uint8_t on) { 00131 dbg_on = (on) ? 1 : 0; 00132 } 00133 00134 /** 00135 * Sends an AT command 00136 * 00137 * Sends a formatted command using printf style formatting 00138 * @see printf 00139 * 00140 * @param command printf-like format string of command to send which 00141 * is appended with a newline 00142 * @param ... all printf-like arguments to insert into command 00143 * @return true only if command is successfully sent 00144 */ 00145 bool send(const char *command, ...); 00146 00147 bool vsend(const char *command, va_list args); 00148 00149 /** 00150 * Receive an AT response 00151 * 00152 * Receives a formatted response using scanf style formatting 00153 * @see scanf 00154 * 00155 * Responses are parsed line at a time. 00156 * Any received data that does not match the response is ignored until 00157 * a timeout occurs. 00158 * 00159 * @param response scanf-like format string of response to expect 00160 * @param ... all scanf-like arguments to extract from response 00161 * @return true only if response is successfully matched 00162 */ 00163 bool recv(const char *response, ...); 00164 bool vrecv(const char *response, va_list args); 00165 00166 00167 /** 00168 * Write a single byte to the underlying stream 00169 * 00170 * @param c The byte to write 00171 * @return The byte that was written or -1 during a timeout 00172 */ 00173 int putc(char c); 00174 00175 /** 00176 * Get a single byte from the underlying stream 00177 * 00178 * @return The byte that was read or -1 during a timeout 00179 */ 00180 int getc(); 00181 00182 /** 00183 * Write an array of bytes to the underlying stream 00184 * assuming the header of the command is already in _txbuffer 00185 * 00186 * @param data the array of bytes to write 00187 * @param size_of_data number of bytes in data array 00188 * @param size_in_buff number of bytes already in the internal buff 00189 * @return number of bytes written or -1 on failure 00190 */ 00191 int write(const char *data, int size_of_data, int size_in_buff); 00192 00193 /** 00194 * Read an array of bytes from the underlying stream 00195 * 00196 * @param data the destination for the read bytes 00197 * @param size number of bytes to read 00198 * @return number of bytes read or -1 on failure 00199 */ 00200 int read(char *data); 00201 00202 /** 00203 * Direct printf to underlying stream 00204 * @see printf 00205 * 00206 * @param format format string to pass to printf 00207 * @param ... arguments to printf 00208 * @return number of bytes written or -1 on failure 00209 */ 00210 int printf(const char *format, ...); 00211 int vprintf(const char *format, va_list args); 00212 00213 /** 00214 * Direct scanf on underlying stream 00215 * @see ::scanf 00216 * 00217 * @param format format string to pass to scanf 00218 * @param ... arguments to scanf 00219 * @return number of bytes read or -1 on failure 00220 */ 00221 int scanf(const char *format, ...); 00222 00223 int vscanf(const char *format, va_list args); 00224 00225 /** 00226 * Attach a callback for out-of-band data 00227 * 00228 * @param prefix string on when to initiate callback 00229 * @param func callback to call when string is read 00230 * @note out-of-band data is only processed during a scanf call 00231 */ 00232 void oob(const char *prefix, mbed::Callback<void()> func); 00233 00234 /** 00235 * Flushes the underlying stream 00236 */ 00237 void flush(); 00238 00239 /** 00240 * Abort current recv 00241 * 00242 * Can be called from oob handler to interrupt the current 00243 * recv operation. 00244 */ 00245 void abort(); 00246 00247 /** 00248 * Process out-of-band data 00249 * 00250 * Process out-of-band data in the receive buffer. This function 00251 * returns immediately if there is no data to process. 00252 * 00253 * @return true if oob data processed, false otherwise 00254 */ 00255 bool process_oob(void); 00256 /** 00257 * Get buffer_size 00258 */ 00259 int get_size(void) { 00260 return _buffer_size; 00261 } 00262 00263 }; 00264 #endif
Generated on Tue Jul 12 2022 16:22:04 by
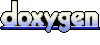