Fujitsu MB85RSxx SPI FRAM access library
Dependents: MB85RSxx_Hello TYBLE16_simple_data_logger
MB85RSxx_SPI.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file MB85RSxx_SPI.cpp 00004 * @author Toyomasa Watarai 00005 * @version V1.0.0 00006 * @date 24 April 2017 00007 * @brief MB85RSxx_SPI class implementation 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * Permission is hereby granted, free of charge, to any person obtaining a copy 00012 * of this software and associated documentation files (the "Software"), to deal 00013 * in the Software without restriction, including without limitation the rights 00014 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00015 * copies of the Software, and to permit persons to whom the Software is 00016 * furnished to do so, subject to the following conditions: 00017 * 00018 * The above copyright notice and this permission notice shall be included in 00019 * all copies or substantial portions of the Software. 00020 * 00021 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00022 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00023 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00024 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00025 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00026 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00027 * THE SOFTWARE. 00028 */ 00029 #include "mbed.h" 00030 #include "MB85RSxx_SPI.h" 00031 00032 MB85RSxx_SPI::MB85RSxx_SPI(PinName mosi, PinName miso, PinName sclk, PinName cs) 00033 : 00034 _spi(mosi, miso, sclk), 00035 _cs(cs) 00036 { 00037 uint8_t buf[4]; 00038 00039 _cs = 1; 00040 _spi.format(8, 0); 00041 read_device_id(buf); 00042 if ((buf[2] & 0x1F) > MB85_DENSITY_512K) { 00043 _address_bits = 24; 00044 } else { 00045 _address_bits = 16; 00046 } 00047 } 00048 00049 MB85RSxx_SPI::~MB85RSxx_SPI() 00050 { 00051 } 00052 00053 void MB85RSxx_SPI::frequency(int hz) 00054 { 00055 _spi.frequency(hz); 00056 } 00057 00058 void MB85RSxx_SPI::read_device_id(uint8_t* device_id) 00059 { 00060 _cs = 0; 00061 _spi.write(MB85_RDID); 00062 for (int i = 0; i < 4; i++) { 00063 *device_id++ = (uint8_t)_spi.write(0); 00064 } 00065 _cs = 1; 00066 } 00067 00068 uint8_t MB85RSxx_SPI::read_status() 00069 { 00070 _cs = 0; 00071 _spi.write(MB85_RDSR); 00072 uint8_t st = (uint8_t)_spi.write(0); 00073 _cs = 1; 00074 00075 return st; 00076 } 00077 00078 void MB85RSxx_SPI::read(uint32_t address, uint8_t* data, uint32_t length) 00079 { 00080 _cs = 0; 00081 _spi.write(MB85_READ); 00082 if (_address_bits == 24) { 00083 _spi.write((uint8_t)((address >> 16) & 0xFF)); 00084 } 00085 _spi.write((uint8_t)((address >> 8) & 0xFF)); 00086 _spi.write((uint8_t)((address >> 0) & 0xFF)); 00087 for (uint32_t i = 0; i < length; i++) { 00088 *data++ = (uint8_t)_spi.write(0); 00089 } 00090 _cs = 1; 00091 } 00092 00093 uint8_t MB85RSxx_SPI::read(uint32_t address) 00094 { 00095 uint8_t data; 00096 00097 _cs = 0; 00098 _spi.write(MB85_READ); 00099 if (_address_bits == 24) { 00100 _spi.write((uint8_t)((address >> 16) & 0xFF)); 00101 } 00102 _spi.write((uint8_t)((address >> 8) & 0xFF)); 00103 _spi.write((uint8_t)((address >> 0) & 0xFF)); 00104 data = (uint8_t)_spi.write(0); 00105 _cs = 1; 00106 00107 return data; 00108 } 00109 00110 void MB85RSxx_SPI::write(uint32_t address, uint8_t* data, uint32_t length) 00111 { 00112 _cs = 0; 00113 _spi.write(MB85_WRITE); 00114 if (_address_bits == 24) { 00115 _spi.write((uint8_t)((address >> 16) & 0xFF)); 00116 } 00117 _spi.write((uint8_t)((address >> 8) & 0xFF)); 00118 _spi.write((uint8_t)((address >> 0) & 0xFF)); 00119 for (uint32_t i = 0; i < length; i++) { 00120 _spi.write(*data++); 00121 } 00122 _cs = 1; 00123 } 00124 00125 void MB85RSxx_SPI::write(uint32_t address, uint8_t data) 00126 { 00127 _cs = 0; 00128 _spi.write(MB85_WRITE); 00129 if (_address_bits == 24) { 00130 _spi.write((uint8_t)((address >> 16) & 0xFF)); 00131 } 00132 _spi.write((uint8_t)((address >> 8) & 0xFF)); 00133 _spi.write((uint8_t)((address >> 0) & 0xFF)); 00134 _spi.write(data); 00135 _cs = 1; 00136 } 00137 00138 void MB85RSxx_SPI::fill(uint32_t address, uint8_t data, uint32_t length) 00139 { 00140 _cs = 0; 00141 _spi.write(MB85_WRITE); 00142 if (_address_bits == 24) { 00143 _spi.write((uint8_t)((address >> 16) & 0xFF)); 00144 } 00145 _spi.write((uint8_t)((address >> 8) & 0xFF)); 00146 _spi.write((uint8_t)((address >> 0) & 0xFF)); 00147 for (uint32_t i = 0; i < length; i++) { 00148 _spi.write(data); 00149 } 00150 _cs = 1; 00151 } 00152 00153 void MB85RSxx_SPI::write_enable() 00154 { 00155 _cs = 0; 00156 _spi.write(MB85_WREN); 00157 _cs = 1; 00158 } 00159 00160 void MB85RSxx_SPI::write_disable() 00161 { 00162 _cs = 0; 00163 _spi.write(MB85_WRDI); 00164 _cs = 1; 00165 }
Generated on Wed Jul 13 2022 19:22:52 by
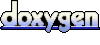