Headers for MAX32630FTHR Demo Board sample programs
Embed:
(wiki syntax)
Show/hide line numbers
generalinterface.cpp
00001 #include "generalinterface.h" 00002 00003 Serial daplink(P2_1,P2_0); 00004 USBSerial microUSB; 00005 SDBlockDevice bd(P0_5, P0_6, P0_4, P0_7); 00006 FATFileSystem fs("fs"); 00007 USBMSD_BD msd(&bd); 00008 00009 void clearSerialStream() 00010 { 00011 char c; 00012 while(daplink.readable()) { 00013 c = daplink.getc(); 00014 wait_ms(1); 00015 } 00016 } 00017 00018 bool getInput(int maxSize,char *inputArray) 00019 { 00020 int i; 00021 char c; 00022 clearSerialStream(); 00023 for(i=0; i<maxSize && c!='\r'; i++) { 00024 c = daplink.getc(); 00025 daplink.putc(c); 00026 inputArray[i] = c; 00027 } 00028 if(i == maxSize) { 00029 return 0; 00030 } else { 00031 return 1; 00032 } 00033 } 00034 00035 void startFileSystem() 00036 { 00037 printf("\f---STARTING FILESYSTEM...---\r\n"); 00038 00039 Thread::wait(100); 00040 00041 // Try to mount the filesystem 00042 printf("Mounting the filesystem... "); 00043 fflush(stdout); 00044 int err = fs.mount(&bd); 00045 printf("%s\r\n", (err ? "Fail :(" : "OK")); 00046 if (err) { 00047 // Reformat if we can't mount the filesystem 00048 // this should only happen on the first boot 00049 printf("No filesystem found, formatting... "); 00050 fflush(stdout); 00051 err = fs.reformat(&bd); 00052 printf("%s\r\n", (err ? "Fail :(" : "OK")); 00053 } 00054 00055 // Open the numbers file 00056 printf("Opening \"/fs/numbers.txt\"... "); 00057 fflush(stdout); 00058 FILE *f = fopen("/fs/numbers.txt", "r+"); 00059 printf("%s\r\n", (!f ? "Fail :(" : "OK")); 00060 if (!f) { 00061 // Create the numbers file if it doesn't exist 00062 printf("No file found, creating a new file... "); 00063 fflush(stdout); 00064 f = fopen("/fs/numbers.txt", "w+"); 00065 printf("%s\r\n", (!f ? "Fail :(" : "OK")); 00066 00067 for (int i = 0; i < 10; i++) { 00068 printf("\rWriting numbers (%d/%d)... ", i, 10); 00069 fflush(stdout); 00070 err = fprintf(f, " %d\r\n", i); 00071 if (err < 0) { 00072 printf("Fail :(\r\n"); 00073 } 00074 } 00075 printf("\rWriting numbers (%d/%d)... OK\r\n", 10, 10); 00076 00077 printf("Seeking file... "); 00078 fflush(stdout); 00079 err = fseek(f, 0, SEEK_SET); 00080 printf("%s\r\n", (err < 0 ? "Fail :(" : "OK")); 00081 } 00082 00083 // Go through and increment the numbers 00084 for (int i = 0; i < 10; i++) { 00085 printf("\rIncrementing numbers (%d/%d)... ", i, 10); 00086 fflush(stdout); 00087 00088 // Get current stream position 00089 long pos = ftell(f); 00090 00091 // Parse out the number and increment 00092 int32_t number; 00093 fscanf(f, "%d", &number); 00094 number += 1; 00095 00096 // Seek to beginning of number 00097 fseek(f, pos, SEEK_SET); 00098 00099 // Store number 00100 fprintf(f, " %d\r\n", number); 00101 } 00102 printf("\rIncrementing numbers (%d/%d)... OK\r\n", 10, 10); 00103 00104 // Close the file which also flushes any cached writes 00105 printf("Closing \"/fs/numbers.txt\"... "); 00106 fflush(stdout); 00107 err = fclose(f); 00108 printf("%s\r\n", (err < 0 ? "Fail :(" : "OK")); 00109 00110 // Display the root directory 00111 printf("Opening the root directory... "); 00112 fflush(stdout); 00113 DIR *d = opendir("/fs/"); 00114 printf("%s\r\n", (!d ? "Fail :(" : "OK")); 00115 00116 printf("root directory:\r\n"); 00117 while (true) { 00118 struct dirent *e = readdir(d); 00119 if (!e) { 00120 break; 00121 } 00122 printf(" %s\r\n", e->d_name); 00123 } 00124 00125 printf("Closing the root directory... "); 00126 fflush(stdout); 00127 err = closedir(d); 00128 printf("%s\r\n", (err < 0 ? "Fail :(" : "OK")); 00129 00130 // Display the numbers file 00131 printf("Opening \"/fs/numbers.txt\"... "); 00132 fflush(stdout); 00133 f = fopen("/fs/numbers.txt", "r"); 00134 printf("%s\r\n", (!f ? "Fail :(" : "OK")); 00135 00136 printf("numbers:\r\n"); 00137 while (!feof(f)) { 00138 int c = fgetc(f); 00139 printf("%c", c); 00140 } 00141 00142 printf("\rClosing \"/fs/numbers.txt\"... "); 00143 fflush(stdout); 00144 err = fclose(f); 00145 printf("%s\r\n", (err < 0 ? "Fail :(" : "OK")); 00146 00147 00148 00149 00150 // Switch to MSD 00151 // printf("Unmounting... "); 00152 // fflush(stdout); 00153 // err = fs.unmount(); 00154 // printf("%s\r\n", (err < 0 ? "Fail :(" : "OK")); 00155 00156 00157 printf("Starting MSD... "); 00158 msd.disk_initialize(); 00159 err = msd.connect(); 00160 printf("%s\r\n", (err < 0 ? "Fail :(" : "OK")); 00161 }
Generated on Sat Jul 23 2022 08:05:41 by
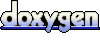