WatchDog Timer library, support for LPC1768 and LPC11U24
Dependents: ECGAFE_copy WatchDog
Fork of WatchDog by
WatchDog.h
00001 /* 00002 * Using code Simon Ford written in his example 00003 * to implement WDT. 00004 * It can run in seconds, milliseconds, and microseconds. 00005 * All there is to do, is keep feeding the watchdog. 00006 */ 00007 00008 #ifndef WATCHDOG_H 00009 #define WATCHDOG_H 00010 00011 #include "mbed.h" 00012 00013 /** Watchdog timer implementation (in seconds) */ 00014 class WatchDog { 00015 public: 00016 /** Creates Watchdog timer 00017 * 00018 * @param Sets the WDT in seconds 00019 */ 00020 WatchDog(float s); 00021 00022 /** Keep feeding the watchdog in routine 00023 * 00024 * xxx.feed(); does the trick 00025 */ 00026 void feed(); 00027 }; 00028 /** Watchdog timer implementation (in milliseconds) */ 00029 class WatchDog_ms { 00030 public: 00031 /** Creates Watchdog timer 00032 * 00033 * @param Sets the WDT in milliseconds 00034 */ 00035 WatchDog_ms(int ms); 00036 /** Keep feeding the watchdog in routine 00037 * 00038 * xxx.feed(); does the trick 00039 */ 00040 void feed(); 00041 }; 00042 /** Watchdog timer implementation (in microseconds) */ 00043 class WatchDog_us { 00044 public: 00045 /** Creates Watchdog timer 00046 * 00047 * @param Sets the WDT in microseconds 00048 */ 00049 WatchDog_us(int us); 00050 /** Keep feeding the watchdog in routine 00051 * 00052 * xxx.feed(); does the trick 00053 */ 00054 void feed(); 00055 }; 00056 00057 #endif
Generated on Fri Jul 15 2022 00:49:24 by
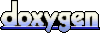