API for linking to the Huxley National Rail REST proxy for the retrieval of live National Rail data. Still needs work (sadly), but works well for the time being!
Dependencies: EthernetInterface
nr_network.h
00001 #ifndef NR_NETWORK_H 00002 #define NR_NETWORK_H 00003 00004 #include "arrival_board.h" 00005 #include "departure_board.h" 00006 #include "EthernetInterface.h" 00007 #include <string> 00008 00009 /* 00010 * This project is distributed by Leigh Brooks under the MIT license. 00011 * 00012 * MIT License 00013 * 00014 * Copyright (c) 2016 Leigh Brooks 00015 * 00016 * Permission is hereby granted, free of charge, to any person obtaining a copy 00017 * of this software and associated documentation files (the "Software"), to deal 00018 * in the Software without restriction, including without limitation the rights 00019 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00020 * copies of the Software, and to permit persons to whom the Software is 00021 * furnished to do so, subject to the following conditions: 00022 * 00023 * The above copyright notice and this permission notice shall be included in all 00024 * copies or substantial portions of the Software. 00025 * 00026 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00027 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00028 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00029 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00030 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00031 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00032 * SOFTWARE. 00033 */ 00034 00035 /** NR_Network_Conn 00036 * Used for establishing a connection to the Huxley NR SOAP proxy and to generate departure/arrivals data 00037 */ 00038 class NR_Network_Conn 00039 { 00040 private: 00041 void init(const char* address, const char* mask, const char* gateway); 00042 EthernetInterface conn; 00043 TCPSocketConnection socket; 00044 char _address[17]; 00045 char _sub_mask[17]; 00046 char _gateway[17]; 00047 00048 public: 00049 /** Make a connection. The empty constructor creates a connection using DHCP. 00050 */ 00051 NR_Network_Conn(); 00052 /** Make a connection. This constructor creates a connection with the specified IP address, subnet and gateway 00053 * @param address the IP address of this device (in the format "192.168.1.101" 00054 * @param mask the subnet mask of this device (in the format "255.255.255.0" 00055 * @param gateway the gatway of this device (in the format "192.168.1.1" 00056 */ 00057 NR_Network_Conn(const char* address, const char* mask, const char* gateway); 00058 /** Get the socket (an object of type TCPSocketConnection) 00059 */ 00060 TCPSocketConnection GetSocket(); 00061 /** Get the IP address of the current connection 00062 */ 00063 char* GetIP(); 00064 /** Establish a connection to the API 00065 */ 00066 int Connect(const char* url); 00067 /** Disconnect from the API 00068 */ 00069 void Disconnect(); 00070 /** Request a list of arrivals from the API and format them into an Arrival_Board object. 00071 * @param code_stn the station code to get arrivals from 00072 * @param number the number of arrivals to retrieve 00073 * @return an Arrival_Board object containing all the arrivals to this station 00074 */ 00075 Arrival_Board GetArrivals(const std::string& code_stn, const std::string& number); 00076 /** Request a list of departures from the API and format them into a Departure_Board object. 00077 * @param code_stn the station code to get departures from 00078 * @param number the number of departures to retrieve 00079 * @return a Departure_Board object containing all the departures from this station 00080 */ 00081 Departure_Board GetDepartures(const std::string& code_stn, const std::string& number); 00082 }; 00083 00084 #endif
Generated on Mon Jul 18 2022 22:01:04 by
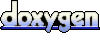