API for linking to the Huxley National Rail REST proxy for the retrieval of live National Rail data. Still needs work (sadly), but works well for the time being!
Dependencies: EthernetInterface
departure_board.cpp
00001 #include "MbedJSONValue.h" 00002 #include "departure_board.h" 00003 00004 00005 using std::string; 00006 00007 Departure_Board::Departure_Board(Departure* _departures, const int& _num_departures, std::string* _messages, const int& _num_messages) 00008 { 00009 departures = _departures; 00010 num_departures = _num_departures; 00011 messages = _messages; 00012 num_messages = _num_messages; 00013 } 00014 00015 Departure_Board::~Departure_Board() 00016 { 00017 delete departures; 00018 delete messages; 00019 } 00020 00021 Departure_Board depBoardFromJson(const string& jsonObject) 00022 { 00023 Departure_Board board; 00024 00025 MbedJSONValue json (jsonObject); 00026 00027 MbedJSONValue trainServices = json["trainServices"]; 00028 MbedJSONValue messages = json["nrccMessages"]; 00029 00030 board.departures = new Departure[trainServices.size()]; 00031 board.num_departures = trainServices.size(); 00032 00033 board.messages = new string[messages.size()]; 00034 board.num_messages = messages.size(); 00035 00036 for(int i = 0; i < trainServices.size(); ++i) { 00037 Departure d; 00038 00039 d.crs = trainServices[i]["destination"]["crs"].get<string>(); 00040 d.locationName = trainServices[i]["destination"]["locationName"].get<string>(); 00041 d.std = trainServices[i]["std"].get<string>(); 00042 d.etd = trainServices[i]["etd"].get<string>(); 00043 d.platform = trainServices[i]["platform"].get<string>(); 00044 d.operatorName = trainServices[i]["operator"].get<string>(); 00045 d.operatorCode = trainServices[i]["operatorCode"].get<string>(); 00046 00047 board.departures[i] = d; 00048 } 00049 00050 for(int i = 0; i < messages.size(); ++i) { 00051 board.messages[i] = messages[i]["value"].get<string>(); 00052 } 00053 00054 return board; 00055 }
Generated on Mon Jul 18 2022 22:01:04 by
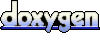