
forkd
Fork of LGstaandart by
Embed:
(wiki syntax)
Show/hide line numbers
core_cmFunc.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cmFunc.h 00003 * @brief CMSIS Cortex-M Core Function Access Header File 00004 * @version V2.01 00005 * @date 06. December 2010 00006 * 00007 * @note 00008 * Copyright (C) 2009-2010 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef __CORE_CMFUNC_H__ 00025 #define __CORE_CMFUNC_H__ 00026 00027 /* ########################### Core Function Access ########################### */ 00028 /** \ingroup CMSIS_Core_FunctionInterface 00029 \defgroup CMSIS_Core_RegAccFunctions CMSIS Core Register Access Functions 00030 @{ 00031 */ 00032 00033 #if defined ( __CC_ARM ) /*------------------ RealView Compiler ----------------*/ 00034 /* ARM armcc specific functions */ 00035 00036 /* intrinsic void __enable_irq(); */ 00037 /* intrinsic void __disable_irq(); */ 00038 00039 /** \brief Get Control Register 00040 00041 This function returns the content of the Control Register. 00042 00043 \return Control Register value 00044 */ 00045 #if (__ARMCC_VERSION < 400000) 00046 extern uint32_t __get_CONTROL(void); 00047 #else /* (__ARMCC_VERSION >= 400000) */ 00048 static __INLINE uint32_t __get_CONTROL(void) 00049 { 00050 register uint32_t __regControl __ASM("control"); 00051 return(__regControl); 00052 } 00053 #endif /* __ARMCC_VERSION */ 00054 00055 00056 /** \brief Set Control Register 00057 00058 This function writes the given value to the Control Register. 00059 00060 \param [in] control Control Register value to set 00061 */ 00062 #if (__ARMCC_VERSION < 400000) 00063 extern void __set_CONTROL(uint32_t control); 00064 #else /* (__ARMCC_VERSION >= 400000) */ 00065 static __INLINE void __set_CONTROL(uint32_t control) 00066 { 00067 register uint32_t __regControl __ASM("control"); 00068 __regControl = control; 00069 } 00070 #endif /* __ARMCC_VERSION */ 00071 00072 00073 /** \brief Get ISPR Register 00074 00075 This function returns the content of the ISPR Register. 00076 00077 \return ISPR Register value 00078 */ 00079 #if (__ARMCC_VERSION < 400000) 00080 extern uint32_t __get_IPSR(void); 00081 #else /* (__ARMCC_VERSION >= 400000) */ 00082 static __INLINE uint32_t __get_IPSR(void) 00083 { 00084 register uint32_t __regIPSR __ASM("ipsr"); 00085 return(__regIPSR); 00086 } 00087 #endif /* __ARMCC_VERSION */ 00088 00089 00090 /** \brief Get APSR Register 00091 00092 This function returns the content of the APSR Register. 00093 00094 \return APSR Register value 00095 */ 00096 #if (__ARMCC_VERSION < 400000) 00097 extern uint32_t __get_APSR(void); 00098 #else /* (__ARMCC_VERSION >= 400000) */ 00099 static __INLINE uint32_t __get_APSR(void) 00100 { 00101 register uint32_t __regAPSR __ASM("apsr"); 00102 return(__regAPSR); 00103 } 00104 #endif /* __ARMCC_VERSION */ 00105 00106 00107 /** \brief Get xPSR Register 00108 00109 This function returns the content of the xPSR Register. 00110 00111 \return xPSR Register value 00112 */ 00113 #if (__ARMCC_VERSION < 400000) 00114 extern uint32_t __get_xPSR(void); 00115 #else /* (__ARMCC_VERSION >= 400000) */ 00116 static __INLINE uint32_t __get_xPSR(void) 00117 { 00118 register uint32_t __regXPSR __ASM("xpsr"); 00119 return(__regXPSR); 00120 } 00121 #endif /* __ARMCC_VERSION */ 00122 00123 00124 /** \brief Get Process Stack Pointer 00125 00126 This function returns the current value of the Process Stack Pointer (PSP). 00127 00128 \return PSP Register value 00129 */ 00130 #if (__ARMCC_VERSION < 400000) 00131 extern uint32_t __get_PSP(void); 00132 #else /* (__ARMCC_VERSION >= 400000) */ 00133 static __INLINE uint32_t __get_PSP(void) 00134 { 00135 register uint32_t __regProcessStackPointer __ASM("psp"); 00136 return(__regProcessStackPointer); 00137 } 00138 #endif /* __ARMCC_VERSION */ 00139 00140 00141 /** \brief Set Process Stack Pointer 00142 00143 This function assigns the given value to the Process Stack Pointer (PSP). 00144 00145 \param [in] topOfProcStack Process Stack Pointer value to set 00146 */ 00147 #if (__ARMCC_VERSION < 400000) 00148 extern void __set_PSP(uint32_t topOfProcStack); 00149 #else /* (__ARMCC_VERSION >= 400000) */ 00150 static __INLINE void __set_PSP(uint32_t topOfProcStack) 00151 { 00152 register uint32_t __regProcessStackPointer __ASM("psp"); 00153 __regProcessStackPointer = topOfProcStack; 00154 } 00155 #endif /* __ARMCC_VERSION */ 00156 00157 00158 /** \brief Get Main Stack Pointer 00159 00160 This function returns the current value of the Main Stack Pointer (MSP). 00161 00162 \return MSP Register value 00163 */ 00164 #if (__ARMCC_VERSION < 400000) 00165 extern uint32_t __get_MSP(void); 00166 #else /* (__ARMCC_VERSION >= 400000) */ 00167 static __INLINE uint32_t __get_MSP(void) 00168 { 00169 register uint32_t __regMainStackPointer __ASM("msp"); 00170 return(__regMainStackPointer); 00171 } 00172 #endif /* __ARMCC_VERSION */ 00173 00174 00175 /** \brief Set Main Stack Pointer 00176 00177 This function assigns the given value to the Main Stack Pointer (MSP). 00178 00179 \param [in] topOfMainStack Main Stack Pointer value to set 00180 */ 00181 #if (__ARMCC_VERSION < 400000) 00182 extern void __set_MSP(uint32_t topOfMainStack); 00183 #else /* (__ARMCC_VERSION >= 400000) */ 00184 static __INLINE void __set_MSP(uint32_t topOfMainStack) 00185 { 00186 register uint32_t __regMainStackPointer __ASM("msp"); 00187 __regMainStackPointer = topOfMainStack; 00188 } 00189 #endif /* __ARMCC_VERSION */ 00190 00191 00192 /** \brief Get Priority Mask 00193 00194 This function returns the current state of the priority mask bit from the Priority Mask Register. 00195 00196 \return Priority Mask value 00197 */ 00198 #if (__ARMCC_VERSION < 400000) 00199 extern uint32_t __get_PRIMASK(void); 00200 #else /* (__ARMCC_VERSION >= 400000) */ 00201 static __INLINE uint32_t __get_PRIMASK(void) 00202 { 00203 register uint32_t __regPriMask __ASM("primask"); 00204 return(__regPriMask); 00205 } 00206 #endif /* __ARMCC_VERSION */ 00207 00208 00209 /** \brief Set Priority Mask 00210 00211 This function assigns the given value to the Priority Mask Register. 00212 00213 \param [in] priMask Priority Mask 00214 */ 00215 #if (__ARMCC_VERSION < 400000) 00216 extern void __set_PRIMASK(uint32_t priMask); 00217 #else /* (__ARMCC_VERSION >= 400000) */ 00218 static __INLINE void __set_PRIMASK(uint32_t priMask) 00219 { 00220 register uint32_t __regPriMask __ASM("primask"); 00221 __regPriMask = (priMask); 00222 } 00223 #endif /* __ARMCC_VERSION */ 00224 00225 00226 #if (__CORTEX_M >= 0x03) 00227 00228 /** \brief Enable FIQ 00229 00230 This function enables FIQ interrupts by clearing the F-bit in the CPSR. 00231 Can only be executed in Privileged modes. 00232 */ 00233 #define __enable_fault_irq __enable_fiq 00234 00235 00236 /** \brief Disable FIQ 00237 00238 This function disables FIQ interrupts by setting the F-bit in the CPSR. 00239 Can only be executed in Privileged modes. 00240 */ 00241 #define __disable_fault_irq __disable_fiq 00242 00243 00244 /** \brief Get Base Priority 00245 00246 This function returns the current value of the Base Priority register. 00247 00248 \return Base Priority register value 00249 */ 00250 #if (__ARMCC_VERSION < 400000) 00251 extern uint32_t __get_BASEPRI(void); 00252 #else /* (__ARMCC_VERSION >= 400000) */ 00253 static __INLINE uint32_t __get_BASEPRI(void) 00254 { 00255 register uint32_t __regBasePri __ASM("basepri"); 00256 return(__regBasePri); 00257 } 00258 #endif /* __ARMCC_VERSION */ 00259 00260 00261 /** \brief Set Base Priority 00262 00263 This function assigns the given value to the Base Priority register. 00264 00265 \param [in] basePri Base Priority value to set 00266 */ 00267 #if (__ARMCC_VERSION < 400000) 00268 extern void __set_BASEPRI(uint32_t basePri); 00269 #else /* (__ARMCC_VERSION >= 400000) */ 00270 static __INLINE void __set_BASEPRI(uint32_t basePri) 00271 { 00272 register uint32_t __regBasePri __ASM("basepri"); 00273 __regBasePri = (basePri & 0xff); 00274 } 00275 #endif /* __ARMCC_VERSION */ 00276 00277 00278 /** \brief Get Fault Mask 00279 00280 This function returns the current value of the Fault Mask register. 00281 00282 \return Fault Mask register value 00283 */ 00284 #if (__ARMCC_VERSION < 400000) 00285 extern uint32_t __get_FAULTMASK(void); 00286 #else /* (__ARMCC_VERSION >= 400000) */ 00287 static __INLINE uint32_t __get_FAULTMASK(void) 00288 { 00289 register uint32_t __regFaultMask __ASM("faultmask"); 00290 return(__regFaultMask); 00291 } 00292 #endif /* __ARMCC_VERSION */ 00293 00294 00295 /** \brief Set Fault Mask 00296 00297 This function assigns the given value to the Fault Mask register. 00298 00299 \param [in] faultMask Fault Mask value to set 00300 */ 00301 #if (__ARMCC_VERSION < 400000) 00302 extern void __set_FAULTMASK(uint32_t faultMask); 00303 #else /* (__ARMCC_VERSION >= 400000) */ 00304 static __INLINE void __set_FAULTMASK(uint32_t faultMask) 00305 { 00306 register uint32_t __regFaultMask __ASM("faultmask"); 00307 __regFaultMask = (faultMask & 1); 00308 } 00309 #endif /* __ARMCC_VERSION */ 00310 00311 #endif /* (__CORTEX_M >= 0x03) */ 00312 00313 00314 #if (__CORTEX_M == 0x04) 00315 00316 /** \brief Get FPSCR 00317 00318 This function returns the current value of the Floating Point Status/Control register. 00319 00320 \return Floating Point Status/Control register value 00321 */ 00322 static __INLINE uint32_t __get_FPSCR(void) 00323 { 00324 #if (__FPU_PRESENT == 1) 00325 register uint32_t __regfpscr __ASM("fpscr"); 00326 return(__regfpscr); 00327 #else 00328 return(0); 00329 #endif 00330 } 00331 00332 00333 /** \brief Set FPSCR 00334 00335 This function assigns the given value to the Floating Point Status/Control register. 00336 00337 \param [in] fpscr Floating Point Status/Control value to set 00338 */ 00339 static __INLINE void __set_FPSCR(uint32_t fpscr) 00340 { 00341 #if (__FPU_PRESENT == 1) 00342 register uint32_t __regfpscr __ASM("fpscr"); 00343 __regfpscr = (fpscr); 00344 #endif 00345 } 00346 00347 #endif /* (__CORTEX_M == 0x04) */ 00348 00349 00350 #elif (defined (__ICCARM__)) /*---------------- ICC Compiler ---------------------*/ 00351 /* IAR iccarm specific functions */ 00352 00353 #if defined (__ICCARM__) 00354 #include <intrinsics.h> /* IAR Intrinsics */ 00355 #endif 00356 00357 #pragma diag_suppress=Pe940 00358 00359 /** \brief Enable IRQ Interrupts 00360 00361 This function enables IRQ interrupts by clearing the I-bit in the CPSR. 00362 Can only be executed in Privileged modes. 00363 */ 00364 #define __enable_irq __enable_interrupt 00365 00366 00367 /** \brief Disable IRQ Interrupts 00368 00369 This function disables IRQ interrupts by setting the I-bit in the CPSR. 00370 Can only be executed in Privileged modes. 00371 */ 00372 #define __disable_irq __disable_interrupt 00373 00374 00375 /* intrinsic unsigned long __get_CONTROL( void ); (see intrinsic.h) */ 00376 /* intrinsic void __set_CONTROL( unsigned long ); (see intrinsic.h) */ 00377 00378 00379 /** \brief Get ISPR Register 00380 00381 This function returns the content of the ISPR Register. 00382 00383 \return ISPR Register value 00384 */ 00385 static uint32_t __get_IPSR(void) 00386 { 00387 __ASM("mrs r0, ipsr"); 00388 } 00389 00390 00391 /** \brief Get APSR Register 00392 00393 This function returns the content of the APSR Register. 00394 00395 \return APSR Register value 00396 */ 00397 static uint32_t __get_APSR(void) 00398 { 00399 __ASM("mrs r0, apsr"); 00400 } 00401 00402 00403 /** \brief Get xPSR Register 00404 00405 This function returns the content of the xPSR Register. 00406 00407 \return xPSR Register value 00408 */ 00409 static uint32_t __get_xPSR(void) 00410 { 00411 __ASM("mrs r0, psr"); // assembler does not know "xpsr" 00412 } 00413 00414 00415 /** \brief Get Process Stack Pointer 00416 00417 This function returns the current value of the Process Stack Pointer (PSP). 00418 00419 \return PSP Register value 00420 */ 00421 static uint32_t __get_PSP(void) 00422 { 00423 __ASM("mrs r0, psp"); 00424 } 00425 00426 00427 /** \brief Set Process Stack Pointer 00428 00429 This function assigns the given value to the Process Stack Pointer (PSP). 00430 00431 \param [in] topOfProcStack Process Stack Pointer value to set 00432 */ 00433 static void __set_PSP(uint32_t topOfProcStack) 00434 { 00435 __ASM("msr psp, r0"); 00436 } 00437 00438 00439 /** \brief Get Main Stack Pointer 00440 00441 This function returns the current value of the Main Stack Pointer (MSP). 00442 00443 \return MSP Register value 00444 */ 00445 static uint32_t __get_MSP(void) 00446 { 00447 __ASM("mrs r0, msp"); 00448 } 00449 00450 00451 /** \brief Set Main Stack Pointer 00452 00453 This function assigns the given value to the Main Stack Pointer (MSP). 00454 00455 \param [in] topOfMainStack Main Stack Pointer value to set 00456 */ 00457 static void __set_MSP(uint32_t topOfMainStack) 00458 { 00459 __ASM("msr msp, r0"); 00460 } 00461 00462 00463 /* intrinsic unsigned long __get_PRIMASK( void ); (see intrinsic.h) */ 00464 /* intrinsic void __set_PRIMASK( unsigned long ); (see intrinsic.h) */ 00465 00466 00467 #if (__CORTEX_M >= 0x03) 00468 00469 /** \brief Enable FIQ 00470 00471 This function enables FIQ interrupts by clearing the F-bit in the CPSR. 00472 Can only be executed in Privileged modes. 00473 */ 00474 static __INLINE void __enable_fault_irq(void) 00475 { 00476 __ASM ("cpsie f"); 00477 } 00478 00479 00480 /** \brief Disable FIQ 00481 00482 This function disables FIQ interrupts by setting the F-bit in the CPSR. 00483 Can only be executed in Privileged modes. 00484 */ 00485 static __INLINE void __disable_fault_irq(void) 00486 { 00487 __ASM ("cpsid f"); 00488 } 00489 00490 00491 /* intrinsic unsigned long __get_BASEPRI( void ); (see intrinsic.h) */ 00492 /* intrinsic void __set_BASEPRI( unsigned long ); (see intrinsic.h) */ 00493 /* intrinsic unsigned long __get_FAULTMASK( void ); (see intrinsic.h) */ 00494 /* intrinsic void __set_FAULTMASK(unsigned long); (see intrinsic.h) */ 00495 00496 #endif /* (__CORTEX_M >= 0x03) */ 00497 00498 00499 #if (__CORTEX_M == 0x04) 00500 00501 /** \brief Get FPSCR 00502 00503 This function returns the current value of the Floating Point Status/Control register. 00504 00505 \return Floating Point Status/Control register value 00506 */ 00507 static uint32_t __get_FPSCR(void) 00508 { 00509 #if (__FPU_PRESENT == 1) 00510 __ASM("vmrs r0, fpscr"); 00511 #else 00512 return(0); 00513 #endif 00514 } 00515 00516 00517 /** \brief Set FPSCR 00518 00519 This function assigns the given value to the Floating Point Status/Control register. 00520 00521 \param [in] fpscr Floating Point Status/Control value to set 00522 */ 00523 static void __set_FPSCR(uint32_t fpscr) 00524 { 00525 #if (__FPU_PRESENT == 1) 00526 __ASM("vmsr fpscr, r0"); 00527 #endif 00528 } 00529 00530 #endif /* (__CORTEX_M == 0x04) */ 00531 00532 #pragma diag_default=Pe940 00533 00534 00535 #elif (defined (__GNUC__)) /*------------------ GNU Compiler ---------------------*/ 00536 /* GNU gcc specific functions */ 00537 00538 /** \brief Enable IRQ Interrupts 00539 00540 This function enables IRQ interrupts by clearing the I-bit in the CPSR. 00541 Can only be executed in Privileged modes. 00542 */ 00543 __attribute__( ( always_inline ) ) static __INLINE void __enable_irq(void) 00544 { 00545 __ASM volatile ("cpsie i"); 00546 } 00547 00548 00549 /** \brief Disable IRQ Interrupts 00550 00551 This function disables IRQ interrupts by setting the I-bit in the CPSR. 00552 Can only be executed in Privileged modes. 00553 */ 00554 __attribute__( ( always_inline ) ) static __INLINE void __disable_irq(void) 00555 { 00556 __ASM volatile ("cpsid i"); 00557 } 00558 00559 00560 /** \brief Get Control Register 00561 00562 This function returns the content of the Control Register. 00563 00564 \return Control Register value 00565 */ 00566 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_CONTROL(void) 00567 { 00568 uint32_t result; 00569 00570 __ASM volatile ("MRS %0, control" : "=r" (result) ); 00571 return(result); 00572 } 00573 00574 00575 /** \brief Set Control Register 00576 00577 This function writes the given value to the Control Register. 00578 00579 \param [in] control Control Register value to set 00580 */ 00581 __attribute__( ( always_inline ) ) static __INLINE void __set_CONTROL(uint32_t control) 00582 { 00583 __ASM volatile ("MSR control, %0" : : "r" (control) ); 00584 } 00585 00586 00587 /** \brief Get ISPR Register 00588 00589 This function returns the content of the ISPR Register. 00590 00591 \return ISPR Register value 00592 */ 00593 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_IPSR(void) 00594 { 00595 uint32_t result; 00596 00597 __ASM volatile ("MRS %0, ipsr" : "=r" (result) ); 00598 return(result); 00599 } 00600 00601 00602 /** \brief Get APSR Register 00603 00604 This function returns the content of the APSR Register. 00605 00606 \return APSR Register value 00607 */ 00608 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_APSR(void) 00609 { 00610 uint32_t result; 00611 00612 __ASM volatile ("MRS %0, apsr" : "=r" (result) ); 00613 return(result); 00614 } 00615 00616 00617 /** \brief Get xPSR Register 00618 00619 This function returns the content of the xPSR Register. 00620 00621 \return xPSR Register value 00622 */ 00623 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_xPSR(void) 00624 { 00625 uint32_t result; 00626 00627 __ASM volatile ("MRS %0, xpsr" : "=r" (result) ); 00628 return(result); 00629 } 00630 00631 00632 /** \brief Get Process Stack Pointer 00633 00634 This function returns the current value of the Process Stack Pointer (PSP). 00635 00636 \return PSP Register value 00637 */ 00638 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_PSP(void) 00639 { 00640 register uint32_t result; 00641 00642 __ASM volatile ("MRS %0, psp\n" : "=r" (result) ); 00643 return(result); 00644 } 00645 00646 00647 /** \brief Set Process Stack Pointer 00648 00649 This function assigns the given value to the Process Stack Pointer (PSP). 00650 00651 \param [in] topOfProcStack Process Stack Pointer value to set 00652 */ 00653 __attribute__( ( always_inline ) ) static __INLINE void __set_PSP(uint32_t topOfProcStack) 00654 { 00655 __ASM volatile ("MSR psp, %0\n" : : "r" (topOfProcStack) ); 00656 } 00657 00658 00659 /** \brief Get Main Stack Pointer 00660 00661 This function returns the current value of the Main Stack Pointer (MSP). 00662 00663 \return MSP Register value 00664 */ 00665 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_MSP(void) 00666 { 00667 register uint32_t result; 00668 00669 __ASM volatile ("MRS %0, msp\n" : "=r" (result) ); 00670 return(result); 00671 } 00672 00673 00674 /** \brief Set Main Stack Pointer 00675 00676 This function assigns the given value to the Main Stack Pointer (MSP). 00677 00678 \param [in] topOfMainStack Main Stack Pointer value to set 00679 */ 00680 __attribute__( ( always_inline ) ) static __INLINE void __set_MSP(uint32_t topOfMainStack) 00681 { 00682 __ASM volatile ("MSR msp, %0\n" : : "r" (topOfMainStack) ); 00683 } 00684 00685 00686 /** \brief Get Priority Mask 00687 00688 This function returns the current state of the priority mask bit from the Priority Mask Register. 00689 00690 \return Priority Mask value 00691 */ 00692 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_PRIMASK(void) 00693 { 00694 uint32_t result; 00695 00696 __ASM volatile ("MRS %0, primask" : "=r" (result) ); 00697 return(result); 00698 } 00699 00700 00701 /** \brief Set Priority Mask 00702 00703 This function assigns the given value to the Priority Mask Register. 00704 00705 \param [in] priMask Priority Mask 00706 */ 00707 __attribute__( ( always_inline ) ) static __INLINE void __set_PRIMASK(uint32_t priMask) 00708 { 00709 __ASM volatile ("MSR primask, %0" : : "r" (priMask) ); 00710 } 00711 00712 00713 #if (__CORTEX_M >= 0x03) 00714 00715 /** \brief Enable FIQ 00716 00717 This function enables FIQ interrupts by clearing the F-bit in the CPSR. 00718 Can only be executed in Privileged modes. 00719 */ 00720 __attribute__( ( always_inline ) ) static __INLINE void __enable_fault_irq(void) 00721 { 00722 __ASM volatile ("cpsie f"); 00723 } 00724 00725 00726 /** \brief Disable FIQ 00727 00728 This function disables FIQ interrupts by setting the F-bit in the CPSR. 00729 Can only be executed in Privileged modes. 00730 */ 00731 __attribute__( ( always_inline ) ) static __INLINE void __disable_fault_irq(void) 00732 { 00733 __ASM volatile ("cpsid f"); 00734 } 00735 00736 00737 /** \brief Get Base Priority 00738 00739 This function returns the current value of the Base Priority register. 00740 00741 \return Base Priority register value 00742 */ 00743 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_BASEPRI(void) 00744 { 00745 uint32_t result; 00746 00747 __ASM volatile ("MRS %0, basepri_max" : "=r" (result) ); 00748 return(result); 00749 } 00750 00751 00752 /** \brief Set Base Priority 00753 00754 This function assigns the given value to the Base Priority register. 00755 00756 \param [in] basePri Base Priority value to set 00757 */ 00758 __attribute__( ( always_inline ) ) static __INLINE void __set_BASEPRI(uint32_t value) 00759 { 00760 __ASM volatile ("MSR basepri, %0" : : "r" (value) ); 00761 } 00762 00763 00764 /** \brief Get Fault Mask 00765 00766 This function returns the current value of the Fault Mask register. 00767 00768 \return Fault Mask register value 00769 */ 00770 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_FAULTMASK(void) 00771 { 00772 uint32_t result; 00773 00774 __ASM volatile ("MRS %0, faultmask" : "=r" (result) ); 00775 return(result); 00776 } 00777 00778 00779 /** \brief Set Fault Mask 00780 00781 This function assigns the given value to the Fault Mask register. 00782 00783 \param [in] faultMask Fault Mask value to set 00784 */ 00785 __attribute__( ( always_inline ) ) static __INLINE void __set_FAULTMASK(uint32_t faultMask) 00786 { 00787 __ASM volatile ("MSR faultmask, %0" : : "r" (faultMask) ); 00788 } 00789 00790 #endif /* (__CORTEX_M >= 0x03) */ 00791 00792 00793 #if (__CORTEX_M == 0x04) 00794 00795 /** \brief Get FPSCR 00796 00797 This function returns the current value of the Floating Point Status/Control register. 00798 00799 \return Floating Point Status/Control register value 00800 */ 00801 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_FPSCR(void) 00802 { 00803 #if (__FPU_PRESENT == 1) 00804 uint32_t result; 00805 00806 __ASM volatile ("MRS %0, fpscr" : "=r" (result) ); 00807 return(result); 00808 #else 00809 return(0); 00810 #endif 00811 } 00812 00813 00814 /** \brief Set FPSCR 00815 00816 This function assigns the given value to the Floating Point Status/Control register. 00817 00818 \param [in] fpscr Floating Point Status/Control value to set 00819 */ 00820 __attribute__( ( always_inline ) ) static __INLINE void __set_FPSCR(uint32_t fpscr) 00821 { 00822 #if (__FPU_PRESENT == 1) 00823 __ASM volatile ("MSR fpscr, %0" : : "r" (fpscr) ); 00824 #endif 00825 } 00826 00827 #endif /* (__CORTEX_M == 0x04) */ 00828 00829 00830 #elif (defined (__TASKING__)) /*--------------- TASKING Compiler -----------------*/ 00831 /* TASKING carm specific functions */ 00832 00833 /* 00834 * The CMSIS functions have been implemented as intrinsics in the compiler. 00835 * Please use "carm -?i" to get an up to date list of all instrinsics, 00836 * Including the CMSIS ones. 00837 */ 00838 00839 #endif 00840 00841 /*@} end of CMSIS_Core_RegAccFunctions */ 00842 00843 00844 #endif /* __CORE_CMFUNC_H__ */ 00845
Generated on Tue Jul 12 2022 17:13:50 by
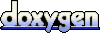