
n
Fork of LG by
Embed:
(wiki syntax)
Show/hide line numbers
PLC_reg.c
00001 /****************************************Copyright (c)**************************************************** 00002 **--------------File Info--------------------------------------------------------------------------------- 00003 ** File name: PLC_reg.c 00004 ** Last modified Date: 2011-09-26 00005 ** Last Version: V1.00 00006 ** Descriptions: Routines for system of perimeter regulating unit 00007 ** 00008 **-------------------------------------------------------------------------------------------------------- 00009 ** Created by: Electrooptica Incorp. 00010 ** Created date: 2011-09-26 00011 ** Version: V1.00 00012 ** Descriptions: 00013 ** 00014 **-------------------------------------------------------------------------------------------------------- 00015 *********************************************************************************************************/ 00016 #include "Global.h" 00017 00018 00019 #define CONFIG_HFO_REG //r. изменяем коэффициент передачи контура ГВЧ от номинального на время обнуления 00020 #define WP_TRANSITION_ENA // 00021 00022 //e.--- constants for the CPLC regulator ------------------------------------------------------- //r.--- константы для контура СРП ------------------------------------------------------- 00023 00024 #define PLC_SHIFT (6) 00025 #define PLC_PHASE_DET_SHIFT (18) //e. 18 - for analog output //r. 18 - для аналогового 00026 00027 #define PLC_RESET_THRESHOLD (-3276) //e. correspond to the voltage +1.2 Volts //r. соответствует напряжению +1.2 вольта 00028 #define WP_REG32MAX_SATURATION (32767 << PLC_SHIFT) 00029 #define WP_REG32MIN_NEW_SATURATION (PLC_RESET_THRESHOLD << PLC_SHIFT) 00030 #define WP_TMP_THRESHOLD (7) //e. temperature threshold, defining heats up or cool down the device //r. температурный порог, определяющий нагревается или охлаждается прибор 00031 00032 00033 #define debugPLC 00034 00035 int WP_reg32; 00036 int WP_Phase_Det; //e. output of the phase detector of the CPLC (in a digital kind)//r. выход фазового детектора СРП (в цифровом виде) 00037 int WP_reset_heating; //e. voltage of reset at heating //r. напряжение сброса при нагревании 00038 int WP_reset_cooling; //e. voltage of reset at cooling //r. напряжение сброса при охлаждении 00039 int MaxDelayPLC; 00040 int sin_func[100]; 00041 00042 int phase_Digital; 00043 00044 int WP_PhaseDetectorRate(int PhaseDetInput, int IntegrateTime); 00045 00046 /****************************************************************************** 00047 ** Function name: init_PLC 00048 ** 00049 ** Descriptions: Initialization procedure for PLC regulator 00050 ** 00051 ** Parameters: None 00052 ** Returned value: None 00053 ** 00054 ******************************************************************************/ 00055 void init_PLC(void) 00056 { 00057 int i; 00058 //( 1,2 вольта) 00059 if (Device_blk.Str.WP_reset < PLC_RESET_THRESHOLD) //e. напряжение после сброса на нагревателе не должно превышать 1,2 вольта. 00060 //(исходное значение регулятора СРП (после сброса)) < (-3276). 00061 { 00062 Device_blk.Str.WP_reset = PLC_RESET_THRESHOLD + 1;//(-3275) 00063 } 00064 //напряжение на СРП = (мин. значение на нагревателе + мах. значение на нагревателе)/2 00065 Output.Str.WP_reg = (Device_blk.Str.WP_rup + Device_blk.Str.WP_rdw) >> 1; //e. WP_reg start voltage is (WP_rup - WP_rdw)/2 00066 00067 // напряжение на СРП << 6 00068 WP_reg32 = Output.Str.WP_reg<<PLC_SHIFT; 00069 00070 if ((Device_blk.Str.PI_b3>100)||(Device_blk.Str.PI_b3<10)) //e. Если требуемая частота модулятора СРП больше 1kHz или меньше 100Hz 00071 Device_blk.Str.PI_b3 = 40; //e. Установить частоту в 250Hz (частота дребездения) 00072 00073 for (i = 0; i<Device_blk.Str.PI_b3; i++) { //e. Сканирование СРП сигнала 00074 float temp = sin((float)i*2.0*PI/(float)Device_blk.Str.PI_b3); /// вычисление значений синуса 00075 /// для частоты модулятора срп (PI_b3), 00076 sin_func[i] = (int)(temp*32767); /// и калибровка этих значений для АЦП. 00077 if (sin_func[i] < 0) 00078 sin_func[i] += 65536; 00079 } 00080 00081 //e. calculation of filter coefficients for PLC 00082 // 250 Hz 10 KHz 00083 init_BandPass( 1.0/(float)Device_blk.Str.PI_b3, 10.0/(float)(DEVICE_SAMPLE_RATE_HZ), PLC); //полософой фильтр для выделения частоты колебания модулятора 00084 //и определение коэфициентов(aPLC[0-2] и bPLC[0-2]) 00085 //(дребездение срп для определения греть или охлождать основной элемент управления.) 00086 00087 Device_blk.Str.WP_scl <<= 1; //e. during fist 10 seconds after start we state Device_blk.Str.WP_scl = 2*Device_blk.Str.WP_scl 00088 // первые 10 секунд работать с коэфициентом передачи * 2 00089 00090 MaxDelayPLC = Device_blk.Str.PI_b3>>1; //e. max expected delay for phase detector output 00091 } // init_PLC 00092 00093 /****************************************************************************** 00094 ** Function name: PLC_MeanderDelay 00095 ** 00096 ** Descriptions: Outgoing of the delayed meander signal for the PLC regulator 00097 ** 00098 ** parameters: Input value 00099 ** Returned value: Delayed value 00100 ** 00101 ******************************************************************************/ 00102 int PLC_MeanderDelay(int flag) 00103 { 00104 static int poz_counter = 0, neg_counter = 0, flg_delay; 00105 00106 if (Device_blk.Str.WP_ref == 0) { 00107 return (flag); 00108 } 00109 00110 //e. check whether delay exceeds the greatest possible value //r. проверка не превосходит ли задержка максимально возможную 00111 if (Device_blk.Str.WP_ref > MaxDelayPLC) { 00112 Device_blk.Str.WP_ref = MaxDelayPLC; 00113 } 00114 00115 if (flag) { //e. outgoing poz_sin_flag flag, which delayed by the WP_ref //r. формирование задержанного на величину WP_ref флага poz_sin_flag 00116 neg_counter = 0; 00117 poz_counter++; 00118 } else { 00119 poz_counter = 0; 00120 neg_counter++; 00121 } 00122 if (poz_counter == Device_blk.Str.WP_ref) { 00123 flg_delay = 0; 00124 } 00125 if (neg_counter == Device_blk.Str.WP_ref) { 00126 flg_delay = 1; 00127 } 00128 return (flg_delay); 00129 } 00130 /****************************************************************************** 00131 ** Function name: clc_PLC 00132 ** 00133 ** Descriptions: Procedure of initial processing for the CPLC regulator 00134 ** 00135 ** parameters: None 00136 ** Returned value: None 00137 ** 00138 ******************************************************************************/ 00139 void clc_PLC(void) 00140 { 00141 static int is_zeroing = 0; 00142 static int zero_delay = 0; 00143 // static int WP_DelaySin_Array[21] = {0}; 00144 // int phase_Digital; 00145 int poz_sin_flag; 00146 int poz_sin_flag_delayed; 00147 00148 00149 static int plc_reset32; 00150 static enum { 00151 //r. состояние линейного перехода при обнулении СРП 00152 FINISHED, //r. линейный переход завершен 00153 TRANS_HEATING, //r. переход выполняется при нагревании 00154 TRANS_COOLING //r. переход выполняется при охлаждении 00155 } plc_transiton = FINISHED; 00156 00157 // int i; 00158 00159 if (Output.Str.WP_sin >= 32768) { 00160 poz_sin_flag = 0; 00161 } else { 00162 poz_sin_flag = 1; 00163 } 00164 00165 //r. полосовой фильтр для контура СРП 00166 WP_Phase_Det = PLC_PhaseDetFilt(/*Output.Str.WP_sin*/Input.StrIn.WP_sel); 00167 00168 00169 if (WP_Phase_Det >0) { 00170 //r. WP_sel>0 00171 phase_Digital = 1; 00172 } else { 00173 phase_Digital = -1; 00174 } 00175 // from this WP_Phase_Det - modulated signal like LIM_DIG 00176 00177 poz_sin_flag_delayed = PLC_MeanderDelay(poz_sin_flag); 00178 00179 if(poz_sin_flag_delayed) { 00180 WP_Phase_Det = -WP_Phase_Det; 00181 phase_Digital = -phase_Digital; 00182 } 00183 // from this WP_Phase_Det - demodulated signal like LIDEM_DIG 00184 00185 if (!is_zeroing) { //r. Не пора выполнять обнуление 00186 //r. нет обнуления 00187 if ((WP_reg32 > (Device_blk.Str.WP_rup << PLC_SHIFT)) && IsHeating) { //r. происходит нагревание 00188 is_zeroing = 1; 00189 //r. напряжение сброса при нагревании 00190 WP_reset_heating = CPL_reset_calc(Device_blk.Str.WP_reset, Device_blk.Str.K_WP_rst_heating, Temp_Aver, Device_blk.Str.TemperNormal); 00191 plc_transiton = TRANS_HEATING; 00192 plc_reset32 = WP_reset_heating << PLC_SHIFT;; 00193 00194 Device_blk.Str.HF_scl = Device_blk.Str.HF_scl_2; //r. изменяем коэффициент передачи контура ГВЧ от номинального на время обнуления 00195 } else if ((WP_reg32 < (Device_blk.Str.WP_rdw << PLC_SHIFT)) && !IsHeating) { //r. охлаждение 00196 is_zeroing = 1; 00197 //r. напряжение сброса при охлаждении 00198 WP_reset_cooling = CPL_reset_calc(Device_blk.Str.WP_reset2, Device_blk.Str.K_WP_rst_cooling, Temp_Aver, Device_blk.Str.TemperNormal); 00199 00200 plc_transiton = TRANS_COOLING; 00201 plc_reset32 = WP_reset_cooling << PLC_SHIFT; 00202 00203 Device_blk.Str.HF_scl = Device_blk.Str.HF_scl_2; //r. изменяем коэффициент передачи контура ГВЧ от номинального на время обнуления 00204 } else //r. пороги не превышены, обычная работа контура 00205 WP_reg32 = L_mac(WP_reg32, phase_Digital, Device_blk.Str.WP_scl ); // WP_reg32 += phase_Digital * Device_blk.Str.WP_scl; 00206 00207 } else { //r. флаг установлен (1) - режим обнуления 00208 00209 if (plc_transiton != FINISHED) { 00210 if (plc_transiton == TRANS_HEATING) { 00211 00212 WP_reg32 = L_sub(WP_reg32, Device_blk.Str.WP_transition_step); // WP_reg32 -= Device_blk.Str.WP_transition_step; 00213 if (WP_reg32 < plc_reset32) { 00214 zero_delay = 0; 00215 plc_transiton = FINISHED; //r.false; 00216 WP_reg32 = plc_reset32; 00217 } 00218 } else { // plc_transiton == TRANS_COOLING 00219 WP_reg32 = L_add(WP_reg32, Device_blk.Str.WP_transition_step); // WP_reg32 += Device_blk.Str.WP_transition_step; 00220 if (WP_reg32 > plc_reset32) { 00221 zero_delay = 0; 00222 plc_transiton = FINISHED; //r.false; 00223 WP_reg32 = plc_reset32; 00224 } 00225 } 00226 } else 00227 00228 if (zero_delay < Device_blk.Str.WP_mdy) { 00229 zero_delay++; 00230 } else { //e. resetting was completed //r. обнуление закончилось 00231 is_zeroing = 0; 00232 //e. save the temperature for further comparison //r. запоминаем температуру для дальнейшего сравнения 00233 // TempOfReset = Temp_Aver; //r.x. Temp5_Aver; //r. Tmp_Out[TSENS_NUMB]; // T4; 00234 //r.x Zero_Numb_dbg++; // так можно подсчитывать число обнулений 00235 00236 // DithFreqRangeCalc(); //e. calculation of range of the division factor for the dither drive frequency, depending on current temperature //r. расчет границ коэффициента деления для частоты вибропривода, зависящих от текущей температуры 00237 } 00238 } 00239 00240 Saturation(WP_reg32, WP_REG32MAX_SATURATION, WP_REG32MIN_NEW_SATURATION); //e. the minimum corresponds to a small negative number, appropriate to PLC_RESET_THRESHOLD //r. минимум соответствует небольшому отрицательному числу, соотв-му PLC_RESET_THRESHOLD 00241 00242 00243 if ( loop_is_closed(WP_REG_ON) ) { //e. the regulator loop is closed //r. контур замкнут 00244 Output.Str.WP_reg = (int)(WP_reg32 >> PLC_SHIFT); //e. we use as controlling - voltages of the integrator //r. используем как управляющее - напряжения интегратора 00245 00246 } else { //e. the regulator loop is open //r. контур разомкнут 00247 WP_reg32 = Output.Str.WP_reg << PLC_SHIFT; //e. set the previous value of the WP_reg //r. присваиваем предыдущее значение WP_reg 00248 00249 } 00250 00251 //e. integartion of output of the PD of the CPLC regulator for the technological output on the Rate command //r. интегрирование выхода ФД контура СРП для технологического вывода по команде Rate 00252 00253 Output.Str.WP_pll = WP_PhaseDetectorRate( WP_Phase_Det, time_1_Sec); 00254 00255 } // clc_PLC 00256 00257 /****************************************************************************** 00258 ** Function name: Signal_2_Oscill 00259 ** 00260 ** Descriptions: Procedure of analog worm output 00261 ** 00262 ** parameters: Type of output 00263 ** Returned value: code to DAC 00264 ** 00265 ******************************************************************************/ 00266 int Signal_2_Oscill() //e. the signal for the control by scope on DAC output (was DS) //r. сигнал для контроля осциллографом на выходе ЦАП (бывший ДУП) 00267 { 00268 // Scope_Mode var not used now, reserved for future applications 00269 return (-WP_Phase_Det << 2); 00270 } // Signal_2_Oscill 00271 00272 /****************************************************************************** 00273 ** Function name: clc_WP_sin 00274 ** 00275 ** Descriptions: Procedure of scan signal generating 00276 ** 00277 ** parameters: None 00278 ** Returned value: Current code for scan signal DAC of PLC 00279 ** 00280 ******************************************************************************/ 00281 int clc_WP_sin(void) 00282 { 00283 static int index = 0; 00284 index++; 00285 00286 if (index >= 40/*Device_blk.Str.PI_b3*/) 00287 index = 0; 00288 /* if (index > 20) 00289 LPC_GPIO0->FIOSET = (1<<26); 00290 else 00291 LPC_GPIO0->FIOCLR = (1<<26); */ 00292 DAC_Output(sin_func[index]); //output to DAC 00293 00294 return (sin_func[index]); 00295 } // clc_WP_sin 00296 00297 /****************************************************************************** 00298 ** Function name: WP_PhaseDetectorRate 00299 ** 00300 ** Descriptions: Integartion of output of the PD of the CPLC regulator 00301 for the technological output on the Rate command 00302 ** 00303 ** Parameters: Current PD magnitude, period of integration 00304 ** Returned value: Integrated magnitude of PD 00305 ** 00306 ******************************************************************************/ 00307 int WP_PhaseDetectorRate(int PhaseDetInput, int IntegrateTime) 00308 { 00309 00310 static int SampleAndHoldOut = 0; 00311 static int WP_PhasDet_integr = 0;//, WP_PhasDetector = 0; 00312 00313 if (IntegrateTime == DEVICE_SAMPLE_RATE_uks) { 00314 SampleAndHoldOut = (int)(WP_PhasDet_integr >> PLC_PHASE_DET_SHIFT); 00315 WP_PhasDet_integr = 0; 00316 } else { 00317 WP_PhasDet_integr += PhaseDetInput; 00318 } 00319 return (SampleAndHoldOut); 00320 } // WP_PhaseDetectorRate
Generated on Tue Jul 12 2022 15:16:11 by
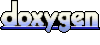