A library for Freescale MCU which contain TSI peripheral, just for Kinetis L version. Because they use "lighter" version of TSI peripheral.
Dependents: kl25z-tinyshell-demo tsi_slider_light_senso_LED frdm_tsi_slider_led_blend demo_slider ... more
tsi_sensor.h
00001 /* Freescale Semiconductor Inc. 00002 * 00003 * mbed Microcontroller Library 00004 * (c) Copyright 2009-2012 ARM Limited. 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00007 * and associated documentation files (the "Software"), to deal in the Software without 00008 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00009 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00010 * Software is furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in all copies or 00013 * substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00016 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00017 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00018 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00020 */ 00021 00022 #ifndef TSISENSOR_H 00023 #define TSISENSOR_H 00024 00025 /** 00026 * TSISensor example 00027 * 00028 * @code 00029 * #include "mbed.h" 00030 * #include "TSISensor.h" 00031 * 00032 * int main(void) { 00033 * DigitalOut led(LED_GREEN); 00034 * TSIElectrode elec0(9); 00035 * TSIElectrode elec1(10); 00036 * TSIAnalogSlider tsi(elec0, elec1, 40); 00037 * 00038 * while (true) { 00039 * printf("slider percentage: %f%\r\n", tsi.readPercentage()); 00040 * printf("slider distance: %dmm\r\n", tsi.readDistance()); 00041 * wait(1); 00042 * led = !led; 00043 * } 00044 * } 00045 * @endcode 00046 */ 00047 #define NO_TOUCH 0 00048 00049 /** TSI Electrode with simple data required for touch detection. 00050 */ 00051 class TSIElectrode { 00052 public: 00053 /** Initialize electrode. 00054 */ 00055 TSIElectrode(PinName pin) : _threshold(100) { 00056 _channel = getTSIChannel(pin); 00057 } 00058 00059 /** Initialize electrode. 00060 */ 00061 TSIElectrode(uint32_t tsi_channel) : _threshold(100) { 00062 _channel = (uint8_t)tsi_channel; 00063 } 00064 /** Set baseline. 00065 */ 00066 void setBaseline(uint32_t baseline) { 00067 _baseline = (uint16_t)baseline; 00068 } 00069 /** Set threshold. 00070 */ 00071 void setThreshold(uint32_t threshold) { 00072 _threshold = (uint16_t)threshold; 00073 } 00074 /** Set signal. 00075 */ 00076 void setSignal(uint32_t signal) { 00077 _signal = (uint16_t)signal; 00078 } 00079 /** Get baseline. 00080 */ 00081 uint32_t getBaseline() { 00082 return _baseline; 00083 } 00084 /** Get delta. 00085 */ 00086 uint32_t getDelta() { 00087 int32_t delta = getSignal() - getBaseline(); 00088 if (delta < 0) { 00089 return 0; 00090 } else { 00091 return delta; 00092 } 00093 } 00094 /** Get signal. 00095 */ 00096 uint32_t getSignal() { 00097 return _signal; 00098 } 00099 /** Get threshold. 00100 */ 00101 uint32_t getThreshold() { 00102 return _threshold; 00103 } 00104 /** Get channel. 00105 */ 00106 uint32_t getChannel() { 00107 return _channel; 00108 } 00109 /** Get TSI Channel for PinName. 00110 * 00111 * @returns TSI channel ID for use in constructor of TSIAnalogSlider and TSIElectrode. 00112 * @throws compile-time error if target is not supported, or runtime error if pin does not match any channel. 00113 */ 00114 static uint8_t getTSIChannel(PinName pin) { 00115 #if defined(TARGET_KL25Z) || defined(TARGET_KL46Z) || defined (TARGET_KL05Z) 00116 switch(pin) { 00117 //these are 00118 case PTA0: return 1; 00119 case PTA1: return 2; 00120 case PTA2: return 3; 00121 case PTA3: return 4; 00122 case PTA4: return 5; 00123 case PTB0: return 0; 00124 case PTB1: return 6; 00125 case PTB2: return 7; 00126 case PTB3: return 8; 00127 case PTB16: return 9; 00128 case PTB17: return 10; 00129 case PTB18: return 11; 00130 case PTB19: return 12; 00131 #if defined(TARGET_KL25Z) || defined(TARGET_KL46Z) 00132 case PTC0: return 13; 00133 case PTC1: return 14; 00134 case PTC2: return 15; 00135 #endif 00136 default: error("PinName provided to TSIElectrode::getTSIChannel() does not correspond to any known TSI channel."); 00137 } 00138 # else 00139 #error "Unknown target for TSIElectrode::getTSIChannel() - only supports KL25Z so far." 00140 # endif 00141 return 0xFF; //should never get here 00142 } 00143 00144 private: 00145 uint8_t _channel; 00146 uint16_t _signal; 00147 uint16_t _baseline; 00148 uint16_t _threshold; 00149 }; 00150 00151 /** Analog slider which consists of two electrodes. 00152 */ 00153 class TSIAnalogSlider { 00154 public: 00155 /** 00156 * 00157 * Initialize the TSI Touch Sensor with the given PinNames 00158 */ 00159 TSIAnalogSlider(PinName elec0, PinName elec1, uint32_t range); 00160 /** 00161 * Initialize the TSI Touch Sensor 00162 */ 00163 TSIAnalogSlider(uint32_t elec0, uint32_t elec1, uint32_t range); 00164 /** 00165 * Read Touch Sensor percentage value 00166 * 00167 * @returns percentage value between [0 ... 1] 00168 */ 00169 float readPercentage(); 00170 /** 00171 * Read Touch Sensor distance 00172 * 00173 * @returns distance in mm. The value is between [0 ... _range] 00174 */ 00175 uint32_t readDistance(); 00176 /** Get current electrode. 00177 */ 00178 TSIElectrode* getCurrentElectrode() { 00179 return _current_elec; 00180 } 00181 /** Set current electrode which is being measured. 00182 */ 00183 void setCurrentElectrode(TSIElectrode *elec){ 00184 _current_elec = elec; 00185 } 00186 /** Get next electrode. 00187 */ 00188 TSIElectrode* getNextElectrode(TSIElectrode* electrode) { 00189 if (electrode->getChannel() == _elec0.getChannel()) { 00190 return &_elec1; 00191 } else { 00192 return &_elec0; 00193 } 00194 } 00195 /** Return absolute distance position. 00196 */ 00197 uint32_t getAbsoluteDistance() { 00198 return _absolute_distance_pos; 00199 } 00200 /** Return absolute precentage position. 00201 */ 00202 uint32_t getAbsolutePosition() { 00203 return _absolute_percentage_pos; 00204 } 00205 /** Set value to the scan in progress flag. 00206 */ 00207 void setScan(uint32_t scan) { 00208 _scan_in_progress = scan; 00209 } 00210 /** Return instance to Analog slider. Used in tsi irq. 00211 */ 00212 static TSIAnalogSlider *getInstance() { 00213 return _instance; 00214 } 00215 private: 00216 void initObject(void); //shared constructor code 00217 void sliderRead(void); 00218 void selfCalibration(void); 00219 void setSliderPercPosition(uint32_t elec_num, uint32_t position) { 00220 _percentage_position[elec_num] = position; 00221 } 00222 void setSliderDisPosition(uint32_t elec_num, uint32_t position) { 00223 _distance_position[elec_num] = position; 00224 } 00225 void setAbsolutePosition(uint32_t position) { 00226 _absolute_percentage_pos = position; 00227 } 00228 void setAbsoluteDistance(uint32_t distance) { 00229 _absolute_distance_pos = distance; 00230 } 00231 private: 00232 TSIElectrode _elec0; 00233 TSIElectrode _elec1; 00234 uint8_t _scan_in_progress; 00235 TSIElectrode* _current_elec; 00236 uint8_t _percentage_position[2]; 00237 uint8_t _distance_position[2]; 00238 uint32_t _absolute_percentage_pos; 00239 uint32_t _absolute_distance_pos; 00240 uint8_t _range; 00241 protected: 00242 static TSIAnalogSlider *_instance; 00243 }; 00244 00245 #endif
Generated on Tue Jul 12 2022 11:17:42 by
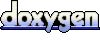