fork
Fork of cpputest by
Embed:
(wiki syntax)
Show/hide line numbers
TestFailure.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/TestFailure.h" 00030 #include "CppUTest/TestOutput.h" 00031 #include "CppUTest/PlatformSpecificFunctions.h" 00032 00033 static SimpleString removeAllPrintableCharactersFrom(const SimpleString& str) 00034 { 00035 size_t bufferSize = str.size()+1; 00036 char* buffer = (char*) PlatformSpecificMalloc(bufferSize); 00037 str.copyToBuffer(buffer, bufferSize); 00038 00039 for (size_t i = 0; i < bufferSize-1; i++) 00040 if (buffer[i] != '\t' && buffer[i] != '\n') 00041 buffer[i] = ' '; 00042 00043 SimpleString result(buffer); 00044 PlatformSpecificFree(buffer); 00045 return result; 00046 } 00047 00048 static SimpleString addMarkerToString(const SimpleString& str, int markerPos) 00049 { 00050 size_t bufferSize = str.size()+1; 00051 char* buffer = (char*) PlatformSpecificMalloc(bufferSize); 00052 str.copyToBuffer(buffer, bufferSize); 00053 00054 buffer[markerPos] = '^'; 00055 00056 SimpleString result(buffer); 00057 PlatformSpecificFree(buffer); 00058 return result; 00059 00060 } 00061 00062 TestFailure::TestFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& theMessage) : 00063 testName_(test->getFormattedName()), fileName_(fileName), lineNumber_(lineNumber), testFileName_(test->getFile()), testLineNumber_(test->getLineNumber()), message_(theMessage) 00064 { 00065 } 00066 00067 TestFailure::TestFailure(UtestShell* test, const SimpleString& theMessage) : 00068 testName_(test->getFormattedName()), fileName_(test->getFile()), lineNumber_(test->getLineNumber()), testFileName_(test->getFile()), testLineNumber_(test->getLineNumber()), message_(theMessage) 00069 { 00070 } 00071 00072 TestFailure::TestFailure(UtestShell* test, const char* fileName, int lineNum) : 00073 testName_(test->getFormattedName()), fileName_(fileName), lineNumber_(lineNum), testFileName_(test->getFile()), testLineNumber_(test->getLineNumber()), message_("no message") 00074 { 00075 } 00076 00077 TestFailure::TestFailure(const TestFailure& f) : 00078 testName_(f.testName_), fileName_(f.fileName_), lineNumber_(f.lineNumber_), testFileName_(f.testFileName_), testLineNumber_(f.testLineNumber_), message_(f.message_) 00079 { 00080 } 00081 00082 00083 TestFailure::~TestFailure() 00084 { 00085 } 00086 00087 SimpleString TestFailure::getFileName() const 00088 { 00089 return fileName_; 00090 } 00091 00092 SimpleString TestFailure::getTestFileName() const 00093 { 00094 return testFileName_; 00095 } 00096 00097 SimpleString TestFailure::getTestName() const 00098 { 00099 return testName_; 00100 } 00101 00102 int TestFailure::getFailureLineNumber() const 00103 { 00104 return lineNumber_; 00105 } 00106 00107 int TestFailure::getTestLineNumber() const 00108 { 00109 return testLineNumber_; 00110 } 00111 00112 SimpleString TestFailure::getMessage() const 00113 { 00114 return message_; 00115 } 00116 00117 bool TestFailure::isOutsideTestFile() const 00118 { 00119 return testFileName_ != fileName_; 00120 } 00121 00122 bool TestFailure::isInHelperFunction() const 00123 { 00124 return lineNumber_ < testLineNumber_; 00125 } 00126 00127 SimpleString TestFailure::createButWasString(const SimpleString& expected, const SimpleString& actual) 00128 { 00129 return StringFromFormat("expected <%s>\n\tbut was <%s>", expected.asCharString(), actual.asCharString()); 00130 } 00131 00132 SimpleString TestFailure::createDifferenceAtPosString(const SimpleString& actual, size_t position) 00133 { 00134 SimpleString result; 00135 const size_t extraCharactersWindow = 20; 00136 const size_t halfOfExtraCharactersWindow = extraCharactersWindow / 2; 00137 00138 SimpleString paddingForPreventingOutOfBounds (" ", halfOfExtraCharactersWindow); 00139 SimpleString actualString = paddingForPreventingOutOfBounds + actual + paddingForPreventingOutOfBounds; 00140 SimpleString differentString = StringFromFormat("difference starts at position %lu at: <", (unsigned long) position); 00141 00142 result += "\n"; 00143 result += StringFromFormat("\t%s%s>\n", differentString.asCharString(), actualString.subString(position, extraCharactersWindow).asCharString()); 00144 00145 SimpleString markString = actualString.subString(position, halfOfExtraCharactersWindow+1); 00146 markString = removeAllPrintableCharactersFrom(markString); 00147 markString = addMarkerToString(markString, halfOfExtraCharactersWindow); 00148 00149 result += StringFromFormat("\t%s%s", SimpleString(" ", differentString.size()).asCharString(), markString.asCharString()); 00150 return result; 00151 } 00152 00153 EqualsFailure::EqualsFailure(UtestShell* test, const char* fileName, int lineNumber, const char* expected, const char* actual) : 00154 TestFailure(test, fileName, lineNumber) 00155 { 00156 message_ = createButWasString(StringFromOrNull(expected), StringFromOrNull(actual)); 00157 } 00158 00159 EqualsFailure::EqualsFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& expected, const SimpleString& actual) 00160 : TestFailure(test, fileName, lineNumber) 00161 { 00162 message_ = createButWasString(expected, actual); 00163 } 00164 00165 static SimpleString StringFromOrNan(double d) 00166 { 00167 if (PlatformSpecificIsNan(d)) 00168 return "Nan - Not a number"; 00169 return StringFrom(d); 00170 } 00171 00172 DoublesEqualFailure::DoublesEqualFailure(UtestShell* test, const char* fileName, int lineNumber, double expected, double actual, double threshold) : TestFailure(test, fileName, lineNumber) 00173 { 00174 message_ = createButWasString(StringFromOrNan(expected), StringFromOrNan(actual)); 00175 message_ += " threshold used was <"; 00176 message_ += StringFromOrNan(threshold); 00177 message_ += ">"; 00178 00179 if (PlatformSpecificIsNan(expected) || PlatformSpecificIsNan(actual) || PlatformSpecificIsNan(threshold)) 00180 message_ += "\n\tCannot make comparisons with Nan"; 00181 } 00182 00183 CheckEqualFailure::CheckEqualFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& expected, const SimpleString& actual) : TestFailure(test, fileName, lineNumber) 00184 { 00185 size_t failStart; 00186 for (failStart = 0; actual.asCharString()[failStart] == expected.asCharString()[failStart]; failStart++) 00187 ; 00188 message_ = createButWasString(expected, actual); 00189 message_ += createDifferenceAtPosString(actual, failStart); 00190 00191 } 00192 00193 ContainsFailure::ContainsFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& expected, const SimpleString& actual) : 00194 TestFailure(test, fileName, lineNumber) 00195 { 00196 message_ = StringFromFormat("actual <%s>\n\tdid not contain <%s>", actual.asCharString(), expected.asCharString()); 00197 } 00198 00199 CheckFailure::CheckFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& checkString, const SimpleString& conditionString, const SimpleString& text) : TestFailure(test, fileName, lineNumber) 00200 { 00201 message_ = ""; 00202 if (!text.isEmpty()) { 00203 message_ += "Message: "; 00204 message_ += text; 00205 message_ += "\n\t"; 00206 } 00207 message_ += checkString; 00208 message_ += "("; 00209 message_ += conditionString; 00210 message_ += ") failed"; 00211 } 00212 00213 FailFailure::FailFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& message) : TestFailure(test, fileName, lineNumber) 00214 { 00215 message_ = message; 00216 } 00217 00218 LongsEqualFailure::LongsEqualFailure(UtestShell* test, const char* fileName, int lineNumber, long expected, long actual) : TestFailure(test, fileName, lineNumber) 00219 { 00220 SimpleString aDecimal = StringFrom(actual); 00221 SimpleString aHex = HexStringFrom(actual); 00222 SimpleString eDecimal = StringFrom(expected); 00223 SimpleString eHex = HexStringFrom(expected); 00224 00225 SimpleString::padStringsToSameLength(aDecimal, eDecimal, ' '); 00226 SimpleString::padStringsToSameLength(aHex, eHex, '0'); 00227 00228 SimpleString actualReported = aDecimal + " 0x" + aHex; 00229 SimpleString expectedReported = eDecimal + " 0x" + eHex; 00230 message_ = createButWasString(expectedReported, actualReported); 00231 } 00232 00233 UnsignedLongsEqualFailure::UnsignedLongsEqualFailure(UtestShell* test, const char* fileName, int lineNumber, unsigned long expected, unsigned long actual) : TestFailure(test, fileName, lineNumber) 00234 { 00235 SimpleString aDecimal = StringFrom(actual); 00236 SimpleString aHex = HexStringFrom(actual); 00237 SimpleString eDecimal = StringFrom(expected); 00238 SimpleString eHex = HexStringFrom(expected); 00239 00240 SimpleString::padStringsToSameLength(aDecimal, eDecimal, ' '); 00241 SimpleString::padStringsToSameLength(aHex, eHex, '0'); 00242 00243 SimpleString actualReported = aDecimal + " 0x" + aHex; 00244 SimpleString expectedReported = eDecimal + " 0x" + eHex; 00245 message_ = createButWasString(expectedReported, actualReported); 00246 } 00247 00248 00249 StringEqualFailure::StringEqualFailure(UtestShell* test, const char* fileName, int lineNumber, const char* expected, const char* actual) : TestFailure(test, fileName, lineNumber) 00250 { 00251 size_t failStart; 00252 for (failStart = 0; actual[failStart] == expected[failStart]; failStart++) 00253 ; 00254 message_ = createButWasString(expected, actual); 00255 message_ += createDifferenceAtPosString(actual, failStart); 00256 } 00257 00258 StringEqualNoCaseFailure::StringEqualNoCaseFailure(UtestShell* test, const char* fileName, int lineNumber, const char* expected, const char* actual) : TestFailure(test, fileName, lineNumber) 00259 { 00260 size_t failStart; 00261 for (failStart = 0; PlatformSpecificToLower(actual[failStart]) == PlatformSpecificToLower(expected[failStart]); failStart++) 00262 ; 00263 message_ = createButWasString(expected, actual); 00264 message_ += createDifferenceAtPosString(actual, failStart); 00265 } 00266 00267
Generated on Tue Jul 12 2022 21:37:56 by
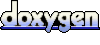