
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "TFT_4DGL.h" 00021 00022 TFT_4DGL ecran(p9,p10,p11); // serial tx, serial rx, reset pin; 00023 00024 int main() { 00025 char s[500]; 00026 int x = 0, y = 0, status, xc = 0, yc = 0; 00027 00028 ecran.baudrate(115200); 00029 ecran.background_color(DGREY); 00030 ecran.circle(120, 160, 80, 0xFF00FF); 00031 ecran.triangle(120, 100, 40, 300, 200, 270, 0x0000FF); 00032 ecran.line(0, 0, 239, 319, 0xFF0000); 00033 ecran.rectangle(50, 50, 200, 90, 0x00FF00); 00034 ecran.ellipse(100, 250, 80, 30, 0xFFFF00); 00035 ecran.pixel(120, 160, BLACK); 00036 ecran.read_pixel(120, 170); 00037 ecran.screen_copy(50, 50, 200, 200, 100, 100); 00038 ecran.pen_size(WIREFRAME); 00039 ecran.circle(120, 160, 60, BLACK); 00040 ecran.set_font(FONT_8X8); 00041 ecran.text_mode(TRANSPARENT); 00042 ecran.text_char('B', 9, 8, BLACK); 00043 ecran.text_char('I',10, 8, BLACK); 00044 ecran.text_char('G',11, 8, BLACK); 00045 ecran.graphic_char('G', 120, 120, BLACK, 4, 4); 00046 ecran.text_string("This is a test of string", 2, 12, FONT_8X8, WHITE); 00047 ecran.graphic_string("This is a test of graphic string", 20, 200, FONT_8X8, WHITE, 2, 2); 00048 ecran.text_button("OK", UP, 40, 260, 0xFF0000, FONT_8X8, BLACK, 2, 2); 00049 00050 // les's play with touch screen now 00051 00052 ecran.set_font(FONT_12X16); 00053 ecran.pen_size(SOLID); 00054 ecran.text_mode(OPAQUE); 00055 ecran.display_control(TOUCH_CTRL, ENABLE); 00056 ecran.set_touch(0, 0, 239, 319); 00057 ecran.wait_touch(50000); 00058 00059 while (1) { 00060 ecran.locate(0,18); 00061 status = ecran.touch_status(); 00062 printf(s, "Status:%d",status); 00063 ecran.puts(s); 00064 ecran.get_touch(&x, &y); 00065 ecran.locate(0,19); 00066 sprintf(s, "X:%03d Y:%03d",x, y); 00067 ecran.puts(s); 00068 switch (status) { 00069 case 1 : 00070 xc = x; 00071 yc = y; 00072 ecran.circle(xc,yc,20,WHITE); 00073 break; 00074 case 2 : 00075 ecran.circle(xc,yc,20,BLACK); 00076 break; 00077 case 3 : 00078 ecran.circle(xc,yc,20,BLACK); 00079 xc = x; 00080 yc = y; 00081 ecran.circle(xc,yc,20,WHITE); 00082 break; 00083 } 00084 } 00085 }
Generated on Wed Jul 13 2022 14:04:39 by
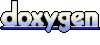