
Demonstrates crash on memory allocation.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 00004 Serial pc(USBTX, USBRX); 00005 BusOut leds(LED1, LED2, LED3, LED4); 00006 00007 extern "C" void HardFault_Handler() 00008 { 00009 pc.printf("Hard fault!\r\n"); 00010 while(1) { 00011 leds = 0x09; 00012 wait(0.4); 00013 leds = 0x06; 00014 wait(0.4); 00015 } 00016 } 00017 00018 00019 void fail() 00020 { 00021 pc.printf("Caught the failure\r\n"); 00022 while(1) { 00023 leds = 0x09; 00024 wait(0.4); 00025 leds = 0x06; 00026 wait(0.4); 00027 } 00028 } 00029 00030 00031 class Test 00032 { 00033 public: 00034 int something; 00035 char woot[28]; 00036 }; 00037 00038 00039 int main() { 00040 Test *dummy; 00041 int index = 0; 00042 00043 while(1) { 00044 dummy = new Test; 00045 if (dummy == NULL) fail(); 00046 pc.printf("%u\r\n", index); 00047 ++index; 00048 } 00049 }
Generated on Wed Jul 13 2022 13:00:05 by
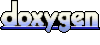