
Prototype
Dependencies: mbed ButtonEventDemo
Lauflicht.cpp
00001 #include "mbed.h" 00002 00003 //#define BUTTON1 p14 00004 #define INIT 0x03; 00005 00006 00007 int modifyBit(int x, unsigned char position, bool state); 00008 int lauflicht(bool richtung, int time, int &anz); 00009 void nibbleLeds(int value); 00010 void printb(uint8_t x); 00011 00012 BusOut myleds(LED1, LED2, LED3, LED4); 00013 DigitalIn button(BUTTON1); 00014 00015 int main(){ 00016 uint8_t value=INIT; 00017 int anzahl, anz; 00018 00019 anzahl=lauflicht(0,400,anz=0); 00020 printf("anzahl= %d\n", anzahl); 00021 nibbleLeds(value); 00022 printb(value); 00023 value=modifyBit(value,2,1); 00024 printb(myleds); 00025 printb(modifyBit(INIT,3,1)); 00026 lauflicht(0,400,anz=0); 00027 wait(0.1); 00028 } 00029 00030 int lauflicht(bool richtung, int time, int &anz){ 00031 int i=0; 00032 uint8_t lauf=0x01; 00033 00034 00035 if(!richtung){ 00036 lauf= 0x08; 00037 } 00038 while(1){ 00039 nibbleLeds(lauf&0x0F); 00040 if(richtung){ 00041 lauf=lauf<<1; 00042 if(lauf>8){ 00043 lauf=0x01; 00044 } 00045 } 00046 else { 00047 lauf=lauf>>1; 00048 if(lauf==0){ 00049 lauf=0x08; 00050 } 00051 } 00052 if(button){ 00053 break; 00054 } 00055 wait_ms(time); 00056 anz++; 00057 } 00058 return anz; 00059 } 00060 00061 int modifyBit(int x, uint8_t position, bool State){ 00062 int mask=1<<position; 00063 int state =int(State); 00064 return (x&~mask)|((state<<position)&mask); 00065 } 00066 00067 void printb(uint8_t x){ 00068 for(int i=sizeof(x)<<3; i; i--){ 00069 putchar('0'+((x>>(i-1))&1)); 00070 } 00071 printf("\n"); 00072 } 00073 00074 void nibbleLeds(int value){ 00075 myleds=value%16; 00076 }
Generated on Mon Jul 18 2022 09:53:45 by
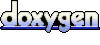