
部内ロボコンで使う回路のサンプルプログラム
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //***************************************** 00002 // メイン基板のサンプルプログラム 00003 //***************************************** 00004 00005 #include "mbed.h" 00006 00007 //デジタル読み取りピンの指定 00008 DigitalInOut Abutton(dp10); 00009 DigitalInOut Bbutton(dp14); 00010 00011 //デジタル出力ピン(空気圧シリンダー)の指定 00012 DigitalOut valve(dp11); 00013 00014 //アナログ読み取りピンの指定 00015 AnalogIn JOYstick_LR(dp13); 00016 00017 //PWM出力ピン(モーター)の指定 00018 PwmOut motorLRplus(dp1); 00019 PwmOut motorLRminus(dp2); 00020 00021 //シリアル入出力ピンの指定 00022 Serial Controller(dp16, dp15); 00023 00024 int main(void){ 00025 00026 //初期設定 00027 valve = 0; 00028 motorLRplus = 0.5; 00029 motorLRminus = 0.5; 00030 00031 //出力レートの指定 00032 Controller.baud(9600); 00033 00034 //デジタル入出力ピンの動作設定 00035 Abutton.input(); 00036 Bbutton.input(); 00037 00038 int l=0; 00039 //なにかPCでシリアルを送るまでは動作しない 00040 while(1){ 00041 wait(0.5); 00042 if(Controller.readable()){ 00043 break; 00044 } 00045 l ^= 1; 00046 } 00047 00048 Controller.printf("LR UD\n"); 00049 00050 int toggle = 0; 00051 float tmp = 0.5; 00052 while(1){ 00053 00054 //ジョイスティックの左右方向を読み取ってモータに投げる 00055 tmp = JOYstick_LR.read(); 00056 Controller.printf("%f\n",tmp); 00057 motorLRplus = tmp; 00058 motorLRminus = 1-tmp; 00059 00060 //タクトスイッチ(Aボタン)が押されていれば"A"を表示.シリンダをONに 00061 if(Abutton == 1 && Bbutton == 0 && toggle == 0){ 00062 Controller.printf("A \n"); 00063 valve = 1; 00064 toggle = 1; 00065 wait(0.05); 00066 } 00067 //タクトスイッチ(Bボタン)が押されていれば"B"を表示.シリンダをOFFに 00068 else if(Abutton == 0 && Bbutton == 1 && toggle == 1){ 00069 Controller.printf("B \n"); 00070 valve = 0; 00071 toggle = 0; 00072 wait(0.05); 00073 } 00074 00075 wait(0.05); 00076 00077 } 00078 00079 }
Generated on Mon Aug 1 2022 21:55:50 by
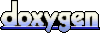