This is a data logger program to be implemented with an instrument amplifier.
Embed:
(wiki syntax)
Show/hide line numbers
Timeout.h
00001 /* mbed Microcontroller Library - Timeout 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 * sford 00004 */ 00005 00006 #ifndef MBED_TIMEOUT_H 00007 #define MBED_TIMEOUT_H 00008 00009 #include "Ticker.h" 00010 00011 namespace mbed { 00012 00013 /* Class: Timeout 00014 * A Timeout is used to call a function at a point in the future 00015 * 00016 * You can use as many seperate Timeout objects as you require. 00017 * 00018 * Example: 00019 * > // Blink until timeout. 00020 * > 00021 * > #include "mbed.h" 00022 * > 00023 * > Timeout timeout; 00024 * > DigitalOut led(LED1); 00025 * > 00026 * > int on = 1; 00027 * > 00028 * > void attimeout() { 00029 * > on = 0; 00030 * > } 00031 * > 00032 * > int main() { 00033 * > timeout.attach(&attimeout, 5); 00034 * > while(on) { 00035 * > led = !led; 00036 * > wait(0.2); 00037 * > } 00038 * > } 00039 */ 00040 class Timeout : public Ticker { 00041 00042 #if 0 // For documentation 00043 00044 /* Function: attach 00045 * Attach a function to be called by the Timeout, specifiying the delay in seconds 00046 * 00047 * Variables: 00048 * fptr - pointer to the function to be called 00049 * t - the time before the call in seconds 00050 */ 00051 void attach(void (*fptr)(void), float t) { 00052 attach_us(fptr, t * 1000000.0f); 00053 } 00054 00055 /* Function: attach 00056 * Attach a member function to be called by the Timeout, specifiying the delay in seconds 00057 * 00058 * Variables: 00059 * tptr - pointer to the object to call the member function on 00060 * mptr - pointer to the member function to be called 00061 * t - the time before the calls in seconds 00062 */ 00063 template<typename T> 00064 void attach(T* tptr, void (T::*mptr)(void), float t) { 00065 attach_us(tptr, mptr, t * 1000000.0f); 00066 } 00067 00068 /* Function: attach_us 00069 * Attach a function to be called by the Timeout, specifiying the delay in micro-seconds 00070 * 00071 * Variables: 00072 * fptr - pointer to the function to be called 00073 * t - the time before the call in micro-seconds 00074 */ 00075 void attach_us(void (*fptr)(void), unsigned int t) { 00076 _function.attach(fptr); 00077 setup(t); 00078 } 00079 00080 /* Function: attach_us 00081 * Attach a member function to be called by the Timeout, specifiying the delay in micro-seconds 00082 * 00083 * Variables: 00084 * tptr - pointer to the object to call the member function on 00085 * mptr - pointer to the member function to be called 00086 * t - the time before the call in micro-seconds 00087 */ 00088 template<typename T> 00089 void attach_us(T* tptr, void (T::*mptr)(void), unsigned int t) { 00090 _function.attach(tptr, mptr); 00091 setup(t); 00092 } 00093 00094 /* Function: detach 00095 * Detach the function 00096 */ 00097 void detach(); 00098 00099 #endif 00100 00101 protected: 00102 00103 virtual void handler(); 00104 00105 }; 00106 00107 } // namespace mbed 00108 00109 #endif
Generated on Wed Jul 13 2022 05:38:48 by
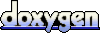