This is a data logger program to be implemented with an instrument amplifier.
Embed:
(wiki syntax)
Show/hide line numbers
DigitalOut.h
00001 /* mbed Microcontroller Library - DigitalOut 00002 * Copyright (c) 2006-2009 ARM Limited. All rights reserved. 00003 * sford 00004 */ 00005 00006 #ifndef MBED_DIGITALOUT_H 00007 #define MBED_DIGITALOUT_H 00008 00009 #include "platform.h" 00010 #include "PinNames.h" 00011 #include "PeripheralNames.h" 00012 #include "Base.h" 00013 00014 namespace mbed { 00015 00016 /* Class: DigitalOut 00017 * A digital output, used for setting the state of a pin 00018 * 00019 * Example: 00020 * > // Toggle a LED 00021 * > #include "mbed.h" 00022 * > 00023 * > DigitalOut led(LED1); 00024 * > 00025 * > int main() { 00026 * > while(1) { 00027 * > led = !led; 00028 * > wait(0.2); 00029 * > } 00030 * > } 00031 */ 00032 class DigitalOut : public Base { 00033 00034 public: 00035 00036 /* Constructor: DigitalOut 00037 * Create a DigitalOut connected to the specified pin 00038 * 00039 * Variables: 00040 * pin - DigitalOut pin to connect to 00041 */ 00042 DigitalOut(PinName pin, const char* name = NULL); 00043 00044 /* Function: write 00045 * Set the output, specified as 0 or 1 (int) 00046 * 00047 * Variables: 00048 * value - An integer specifying the pin output value, 00049 * 0 for logical 0 and 1 (or any other non-zero value) for logical 1 00050 */ 00051 void write(int value) { 00052 if(value) { 00053 _gpio->FIOSET = _mask; 00054 } else { 00055 _gpio->FIOCLR = _mask; 00056 } 00057 } 00058 00059 /* Function: read 00060 * Return the output setting, represented as 0 or 1 (int) 00061 * 00062 * Variables: 00063 * returns - An integer representing the output setting of the pin, 00064 * 0 for logical 0 and 1 for logical 1 00065 */ 00066 int read() { 00067 return ((_gpio->FIOPIN & _mask) ? 1 : 0); 00068 } 00069 00070 00071 #ifdef MBED_OPERATORS 00072 /* Function: operator= 00073 * A shorthand for <write> 00074 */ 00075 DigitalOut& operator= (int value) { 00076 write(value); 00077 return *this; 00078 } 00079 00080 DigitalOut& operator= (DigitalOut& rhs) { 00081 write(rhs.read()); 00082 return *this; 00083 } 00084 00085 00086 /* Function: operator int() 00087 * A shorthand for <read> 00088 */ 00089 operator int() { 00090 return read(); 00091 } 00092 00093 #endif 00094 00095 #ifdef MBED_RPC 00096 virtual const struct rpc_method *get_rpc_methods(); 00097 static struct rpc_class *get_rpc_class(); 00098 #endif 00099 00100 protected: 00101 00102 PinName _pin; 00103 LPC_GPIO_TypeDef *_gpio; 00104 uint32_t _mask; 00105 00106 00107 }; 00108 00109 } // namespace mbed 00110 00111 #endif
Generated on Wed Jul 13 2022 05:38:48 by
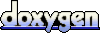