Programme BUS CAN
Fork of Grove_LCD_RGB_Backlight by
Embed:
(wiki syntax)
Show/hide line numbers
Grove_LCD_RGB_Backlight.h
00001 #include "mbed.h" 00002 00003 // I2C addresses for LCD and RGB 00004 #define LCD_ADDRESS (0x7c) 00005 #define RGB_ADDRESS (0xc4) 00006 00007 #define RED_REG 0x04 00008 #define GREEN_REG 0x03 00009 #define BLUE_REG 0x02 00010 00011 // commands 00012 #define LCD_CLEARDISPLAY 0x01 00013 #define LCD_DISPLAYCONTROL 0x08 00014 #define LCD_FUNCTIONSET 0x20 00015 00016 // flags for display on/off control 00017 #define LCD_DISPLAYON 0x04 00018 #define LCD_DISPLAYOFF 0x00 00019 00020 // flag for entry mode 00021 #define LCD_ENTRYLEFT 0x02 00022 00023 // flags for function set 00024 #define LCD_8BITMODE 0x10 00025 #define LCD_2LINE 0x08 00026 #define LCD_5x10DOTS 0x04 00027 00028 00029 class Grove_LCD_RGB_Backlight 00030 { 00031 00032 public: 00033 00034 //Contructor 00035 Grove_LCD_RGB_Backlight(PinName sda, PinName scl); 00036 00037 00038 00039 //Set RGB Color of backglight 00040 void setRGB(char r, char g, char b); 00041 00042 //Initialize device 00043 void init(); 00044 00045 //Turn on display 00046 void displayOn(); 00047 00048 //Clear all text from display 00049 void clear(); 00050 00051 //Print text to the lcd screen 00052 void print(char *str); 00053 00054 //Move cursor to specified location 00055 void locate(char col, char row); 00056 00057 00058 private: 00059 00060 //Send command to display 00061 void sendCommand(char value); 00062 00063 //Set register value 00064 void setReg(char addr, char val); 00065 00066 //MBED I2C object used to transfer data to LCD 00067 I2C i2c; 00068 00069 };
Generated on Thu Aug 4 2022 06:47:27 by
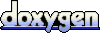