
Some code for a eavesdropper device.
Dependencies: FastIO FastPWM mbed
main.cpp
00001 #include "mbed.h" 00002 #include "FastIO.h" 00003 #include "FastPWM.h" 00004 00005 FastOut<LED1> led; 00006 00007 //Pin 29 and 30 are connected to pin 21 00008 FastIn<p29> CAP_2_1_input; 00009 FastIn<p30> CAP_2_0_input; 00010 FastPWM pwm(p21); 00011 00012 00013 Serial pc(USBTX, USBRX); // tx, rx 00014 00015 #define TIM2CR0 LPC_TIM2->CR0 00016 #define TIM2CR1 LPC_TIM2->CR1 00017 00018 //void _cap_2_1_falling() { 00019 // pc.printf("Interrupt: %X\n", LPC_TIM2->IR); 00020 //led = 1; 00021 //} 00022 00023 int main() 00024 { 00025 00026 unsigned int ticks = 226; 00027 00028 // This signal should give a manchester encoded 101010101 00029 pwm.period_ticks(ticks); 00030 pwm.pulsewidth_ticks(ticks / 2); 00031 00032 // Setup TIM2 00033 00034 // SC 00035 LPC_SC->PCONP |= (1<<22); // Power on the Timer2 00036 LPC_SC->PCLKSEL1 &= ~(3<<12); // Select CCLK/4 for TIM2 (whipes previous clock setting) 00037 LPC_SC->PCLKSEL1 |= (1<<12); // Select CCLK for TIM2 00038 00039 // CCR 00040 LPC_TIM2->CCR |= (3 << 1); // Set CAP2.0 on falling edge with interrupt 00041 LPC_TIM2->CCR |= (1 << 3); // Set CAP2.1 on rising edge 00042 00043 pc.printf("CCR: %X\n", LPC_TIM2->CCR); 00044 00045 // TCR 00046 LPC_TIM2->TCR = 0x0002; // Reset TIM2 00047 LPC_TIM2->TCR = 0x0001; // Enable TIM2 00048 00049 bool buffer [16]; 00050 int bits = 0; 00051 00052 //NVIC_DisableIRQ(TIMER2_IRQn); 00053 //NVIC_SetVector(TIMER2_IRQn, (uint32_t)&_cap_2_1_falling); 00054 //NVIC_EnableIRQ(TIMER2_IRQn); 00055 00056 while(1) { 00057 00058 //pc.printf("Interrupt: %X\n", LPC_TIM2->IR); 00059 00060 // Poll CRO (falling edge) intterupt 00061 if (LPC_TIM2->IR & (1 << 4)) { 00062 00063 led = 1; 00064 00065 wait(100); 00066 00067 led = 0; 00068 00069 int diff = (TIM2CR0 - TIM2CR1) % 0xFFFFFFFF; 00070 00071 if (diff > 90 && diff < 136) { // Check if diff is equal to T=1/848kHz with 20% error 00072 // bit transition 00073 00074 if (bits == 0) { // Start of sequence 00075 buffer[0] = 1; 00076 } else { 00077 buffer[bits] = !buffer[bits-1]; 00078 } 00079 00080 pc.printf("Trasition: %u\n", diff); 00081 00082 } else if (diff > 45 && diff < 68) { // Check if diff is equal to T/2 00083 // repeat last bit 00084 00085 if (bits == 0) { // Start of sequence 00086 buffer[0] = 1; 00087 } else { 00088 buffer[bits] = buffer[bits-1]; 00089 } 00090 00091 pc.printf("Repeat: %u\n", diff); 00092 00093 } else { 00094 // error, default to 1 00095 pc.printf("Error: %u\n", diff); 00096 } 00097 00098 bits = bits + 1 % 16; 00099 00100 LPC_TIM2->IR |= (1 << 4); // Clear IR by writing a one 00101 00102 } 00103 00104 // if (bits == 0) { 00105 // 00106 // for (int i = 0; i< 16; i++) { 00107 // pc.printf("%u", (int) buffer[i]); 00108 // } 00109 // 00110 // pc.printf("\n"); 00111 // 00112 // } 00113 00114 // // Poll CRO (rising edge) intterupt 00115 // if (LCP_TIM2-IR != (1 << 5)) { 00116 // 00117 // int diff = (TIM2CR1 - TIM2CR2) % 0xFFFFFFFF; 00118 // 00119 // // Do checks 00120 // 00121 // // End checks 00122 // 00123 // 00124 // LCP_TIM2->IR &= ~(1 << 5); 00125 // } 00126 00127 00128 00129 } 00130 00131 }
Generated on Tue Jul 12 2022 20:19:21 by
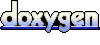