reverted HTTPCLient debug back to defaulted off
Fork of MTS-Socket by
TCPSocketConnection.h
00001 #ifndef TCPSOCKETCONNECTION_H 00002 #define TCPSOCKETCONNECTION_H 00003 00004 #include "Socket.h" 00005 #include "Endpoint.h" 00006 00007 /** 00008 TCP socket connection 00009 */ 00010 class TCPSocketConnection: public Socket, public Endpoint { 00011 00012 public: 00013 /** TCP socket connection 00014 */ 00015 TCPSocketConnection(); 00016 00017 /** Connects this TCP socket to the server 00018 \param host The host to connect to. It can either be an IP Address or a hostname that will be resolved with DNS. 00019 \param port The host's port to connect to. 00020 \return 0 on success, -1 on failure. 00021 */ 00022 int connect(const char* host, const int port); 00023 00024 /** Check if the socket is connected 00025 \return true if connected, false otherwise. 00026 */ 00027 bool is_connected(void); 00028 00029 /** Send data to the remote host. 00030 \param data The buffer to send to the host. 00031 \param length The length of the buffer to send. 00032 \return the number of written bytes on success (>=0) or -1 on failure 00033 */ 00034 int send(char* data, int length); 00035 00036 /** Send all the data to the remote host. 00037 \param data The buffer to send to the host. 00038 \param length The length of the buffer to send. 00039 \return the number of written bytes on success (>=0) or -1 on failure 00040 */ 00041 int send_all(char* data, int length); 00042 00043 /** Receive data from the remote host. 00044 \param data The buffer in which to store the data received from the host. 00045 \param length The maximum length of the buffer. 00046 \return the number of received bytes on success (>=0) or -1 on failure 00047 */ 00048 int receive(char* data, int length); 00049 00050 /** Receive all the data from the remote host. 00051 \param data The buffer in which to store the data received from the host. 00052 \param length The maximum length of the buffer. 00053 \return the number of received bytes on success (>=0) or -1 on failure 00054 */ 00055 int receive_all(char* data, int length); 00056 }; 00057 00058 #endif
Generated on Wed Jul 13 2022 10:29:47 by
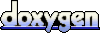