added prescaler for 16 bit pwm in LPC1347 target
Fork of mbed-dev by
FileBase.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "FileBase.h" 00017 00018 namespace mbed { 00019 00020 FileBase *FileBase::_head = NULL; 00021 SingletonPtr<PlatformMutex> FileBase::_mutex; 00022 00023 FileBase::FileBase(const char *name, PathType t) : _next(NULL), 00024 _name(name), 00025 _path_type(t) { 00026 _mutex->lock(); 00027 if (name != NULL) { 00028 // put this object at head of the list 00029 _next = _head; 00030 _head = this; 00031 } else { 00032 _next = NULL; 00033 } 00034 _mutex->unlock(); 00035 } 00036 00037 FileBase::~FileBase() { 00038 _mutex->lock(); 00039 if (_name != NULL) { 00040 // remove this object from the list 00041 if (_head == this) { // first in the list, so just drop me 00042 _head = _next; 00043 } else { // find the object before me, then drop me 00044 FileBase *p = _head; 00045 while (p->_next != this) { 00046 p = p->_next; 00047 } 00048 p->_next = _next; 00049 } 00050 } 00051 _mutex->unlock(); 00052 } 00053 00054 FileBase *FileBase::lookup(const char *name, unsigned int len) { 00055 _mutex->lock(); 00056 FileBase *p = _head; 00057 while (p != NULL) { 00058 /* Check that p->_name matches name and is the correct length */ 00059 if (p->_name != NULL && std::strncmp(p->_name, name, len) == 0 && std::strlen(p->_name) == len) { 00060 _mutex->unlock(); 00061 return p; 00062 } 00063 p = p->_next; 00064 } 00065 _mutex->unlock(); 00066 return NULL; 00067 } 00068 00069 FileBase *FileBase::get(int n) { 00070 _mutex->lock(); 00071 FileBase *p = _head; 00072 int m = 0; 00073 while (p != NULL) { 00074 if (m == n) { 00075 _mutex->unlock(); 00076 return p; 00077 } 00078 00079 m++; 00080 p = p->_next; 00081 } 00082 _mutex->unlock(); 00083 return NULL; 00084 } 00085 00086 const char* FileBase::getName(void) { 00087 // Constant read so no lock needed 00088 return _name; 00089 } 00090 00091 PathType FileBase::getPathType(void) { 00092 // Constant read so no lock needed 00093 return _path_type; 00094 } 00095 00096 } // namespace mbed 00097
Generated on Tue Jul 12 2022 13:38:58 by
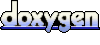