
A basic example of two treads for TCP and UDP. The TCP server in its thread waiting for a command (!start or !stop) from a client. That control the UDP thread and it start or stop sending a dummy message.
main.cpp
00001 #include "mbed.h" //MbedOS 5.15 on Nucleo-F767ZI 00002 #include "NetworkInterface.h" 00003 00004 #define BUFFSIZE 50 00005 00006 #define ROUTER 00007 #ifndef ROUTER 00008 #define IP "192.168.1.1" //Here place your Static IP of Mbed, when you want it to connect directly to PC 00009 #define GATEWAY "0.0.0.0" 00010 #define MASK "255.255.255.0" 00011 #endif 00012 #define ADDRESS "192.168.1.10" /*Here place IP of your PC. Run cmd.exe and write command ipconfig. 00013 If the address will be incorrect, the first message will be lost 00014 and connection will be established with first received message from opposite side.*/ 00015 #define UDPPORT 20 00016 #define UDPREMOTEPORT 2000 00017 #define TCPPORT 80 00018 00019 00020 DigitalOut led1(LED1); 00021 DigitalOut led2(LED2); 00022 DigitalOut led3(LED3); 00023 Thread threadUDP; 00024 Thread threadTCP; 00025 Ticker ticker; 00026 NetworkInterface *net = NetworkInterface::get_default_instance(); 00027 SocketAddress ip; 00028 volatile bool startFlag = false; 00029 volatile int dummyValue = 0; 00030 00031 void tickerFunc(); 00032 void taskTCP(); 00033 void taskUDP(); 00034 00035 int main() { 00036 printf("Example of UDP and TCP at once\n"); 00037 int net_stat; 00038 #ifndef ROUTER 00039 net->disconnect(); 00040 net_stat = net->set_network((SocketAddress)IP,(SocketAddress)MASK,(SocketAddress)GATEWAY); 00041 printf("Network set IP status: %s\n", net_stat ? "Error": "OK"); 00042 #endif 00043 printf("Connecting..."); 00044 net_stat = net->connect(); 00045 printf("%s\n",net_stat ? "Error": "OK"); 00046 net->get_ip_address(&ip); 00047 const char *p_ip = ip.get_ip_address(); 00048 printf("Device IP address: %s\n", p_ip ? p_ip : "None"); 00049 if(ip){ 00050 SocketAddress mask; 00051 net->get_netmask(&mask); 00052 const char *p_mask = mask.get_ip_address(); 00053 printf("Netmask: %s\n", p_mask ? p_mask : "None"); 00054 SocketAddress gateway; 00055 net->get_gateway(&gateway); 00056 const char *p_gateway = gateway.get_ip_address(); 00057 printf("Gateway: %s\n", p_gateway ? p_gateway : "None"); 00058 ticker.attach(&tickerFunc, 1); 00059 printf("Starting of TCP and UDP threads...\n"); 00060 ThisThread::sleep_for(200); 00061 threadUDP.start(callback(taskUDP)); 00062 ThisThread::sleep_for(200); 00063 threadTCP.start(callback(taskTCP)); 00064 ThisThread::sleep_for(200); 00065 }else{ 00066 printf("No IP\n"); 00067 if(net != nullptr) net->disconnect(); 00068 printf("Program End\n"); 00069 } 00070 00071 while(1) { 00072 led1 =! led1; 00073 ThisThread::sleep_for(1000); 00074 } 00075 } 00076 00077 void tickerFunc(){ 00078 dummyValue = rand() % 100; //random 0-99 00079 } 00080 00081 void taskTCP(){ 00082 printf("TCP Server starting...\n"); 00083 TCPSocket server; 00084 TCPSocket *client; 00085 SocketAddress clientAddress; 00086 char in_buffer[BUFFSIZE]; 00087 const char *selection[] = {"!stop","!start"}; 00088 server.open(net); 00089 server.bind(TCPPORT); 00090 server.listen(1); 00091 printf("TCP Server bound and listening at port: %d\n", TCPPORT); 00092 while (1) { 00093 client = server.accept(); 00094 client->getpeername(&clientAddress); 00095 const char *p_clientAddress = clientAddress.get_ip_address(); 00096 printf("Client connected from IP address: %s\n", p_clientAddress ? p_clientAddress : "None"); 00097 bool b = true; 00098 while (b) { 00099 led2 =! led2; 00100 int n = client->recv(in_buffer, BUFFSIZE); 00101 if (n == 0) { 00102 printf("Client disconnected\n"); 00103 b = false; 00104 }else{ 00105 in_buffer[n] = '\0'; 00106 char *result = nullptr; 00107 for(int i = 0; i<2; i++){ 00108 result = strstr(in_buffer,selection[i]); 00109 if(result != nullptr){ 00110 startFlag = i; 00111 break; 00112 } 00113 } 00114 printf("Received message from Client :'%s'\n", in_buffer); 00115 } 00116 } 00117 client->close(); 00118 } 00119 server.close(); 00120 printf("Thread end\n"); 00121 } 00122 00123 void taskUDP(){ 00124 printf("UDP starting...\n"); 00125 UDPSocket sock; 00126 SocketAddress addr; 00127 SocketAddress targetAddr(ADDRESS,UDPREMOTEPORT); 00128 sock.open(net); 00129 sock.bind(UDPPORT); 00130 printf("UDP listens on the local port: %d and send to the remote port %d\n", UDPPORT, UDPREMOTEPORT); 00131 char buffer[BUFFSIZE]; 00132 while(1){ 00133 while(!startFlag) ThisThread::sleep_for(100); 00134 sprintf(buffer,"Dummy value %d\n",dummyValue); 00135 sock.sendto(targetAddr, buffer, sizeof(buffer)); 00136 printf("Send back: %s", buffer); 00137 led3 =! led3; 00138 ThisThread::sleep_for(500); 00139 } 00140 }
Generated on Thu Jul 14 2022 16:29:44 by
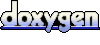