
Example of using the LVGL (8.3.4) with the MbedOS (6.16 - bare metal) on the Disco-F746NG board
Dependencies: BSP_DISCO_F746NG
lv_conf.h
00001 /** 00002 * @file lv_conf.h 00003 * Configuration file for v8.3.4 00004 */ 00005 00006 /* 00007 * Copy this file as `lv_conf.h` 00008 * 1. simply next to the `lvgl` folder 00009 * 2. or any other places and 00010 * - define `LV_CONF_INCLUDE_SIMPLE` 00011 * - add the path as include path 00012 */ 00013 00014 /* clang-format off */ 00015 #if 1 /*Set it to "1" to enable content*/ 00016 00017 #ifndef LV_CONF_H 00018 #define LV_CONF_H 00019 00020 #include <stdint.h> 00021 00022 /*==================== 00023 COLOR SETTINGS 00024 *====================*/ 00025 00026 /*Color depth: 1 (1 byte per pixel), 8 (RGB332), 16 (RGB565), 32 (ARGB8888)*/ 00027 #define LV_COLOR_DEPTH 16 00028 00029 /*Swap the 2 bytes of RGB565 color. Useful if the display has an 8-bit interface (e.g. SPI)*/ 00030 #define LV_COLOR_16_SWAP 0 00031 00032 /*Enable features to draw on transparent background. 00033 *It's required if opa, and transform_* style properties are used. 00034 *Can be also used if the UI is above another layer, e.g. an OSD menu or video player.*/ 00035 #define LV_COLOR_SCREEN_TRANSP 0 00036 00037 /* Adjust color mix functions rounding. GPUs might calculate color mix (blending) differently. 00038 * 0: round down, 64: round up from x.75, 128: round up from half, 192: round up from x.25, 254: round up */ 00039 #define LV_COLOR_MIX_ROUND_OFS 0 00040 00041 /*Images pixels with this color will not be drawn if they are chroma keyed)*/ 00042 #define LV_COLOR_CHROMA_KEY lv_color_hex(0x00ff00) /*pure green*/ 00043 00044 /*========================= 00045 MEMORY SETTINGS 00046 *=========================*/ 00047 00048 /*1: use custom malloc/free, 0: use the built-in `lv_mem_alloc()` and `lv_mem_free()`*/ 00049 #define LV_MEM_CUSTOM 0 00050 #if LV_MEM_CUSTOM == 0 00051 /*Size of the memory available for `lv_mem_alloc()` in bytes (>= 2kB)*/ 00052 #define LV_MEM_SIZE (48U * 1024U) /*[bytes]*/ 00053 00054 /*Set an address for the memory pool instead of allocating it as a normal array. Can be in external SRAM too.*/ 00055 #define LV_MEM_ADR 0 /*0: unused*/ 00056 /*Instead of an address give a memory allocator that will be called to get a memory pool for LVGL. E.g. my_malloc*/ 00057 #if LV_MEM_ADR == 0 00058 #undef LV_MEM_POOL_INCLUDE 00059 #undef LV_MEM_POOL_ALLOC 00060 #endif 00061 00062 #else /*LV_MEM_CUSTOM*/ 00063 #define LV_MEM_CUSTOM_INCLUDE <stdlib.h> /*Header for the dynamic memory function*/ 00064 #define LV_MEM_CUSTOM_ALLOC malloc 00065 #define LV_MEM_CUSTOM_FREE free 00066 #define LV_MEM_CUSTOM_REALLOC realloc 00067 #endif /*LV_MEM_CUSTOM*/ 00068 00069 /*Number of the intermediate memory buffer used during rendering and other internal processing mechanisms. 00070 *You will see an error log message if there wasn't enough buffers. */ 00071 #define LV_MEM_BUF_MAX_NUM 16 00072 00073 /*Use the standard `memcpy` and `memset` instead of LVGL's own functions. (Might or might not be faster).*/ 00074 #define LV_MEMCPY_MEMSET_STD 0 00075 00076 /*==================== 00077 HAL SETTINGS 00078 *====================*/ 00079 00080 /*Default display refresh period. LVG will redraw changed areas with this period time*/ 00081 #define LV_DISP_DEF_REFR_PERIOD 30 /*[ms]*/ 00082 00083 /*Input device read period in milliseconds*/ 00084 #define LV_INDEV_DEF_READ_PERIOD 30 /*[ms]*/ 00085 00086 /*Use a custom tick source that tells the elapsed time in milliseconds. 00087 *It removes the need to manually update the tick with `lv_tick_inc()`)*/ 00088 #define LV_TICK_CUSTOM 0 00089 #if LV_TICK_CUSTOM 00090 #define LV_TICK_CUSTOM_INCLUDE "Arduino.h" /*Header for the system time function*/ 00091 #define LV_TICK_CUSTOM_SYS_TIME_EXPR (millis()) /*Expression evaluating to current system time in ms*/ 00092 #endif /*LV_TICK_CUSTOM*/ 00093 00094 /*Default Dot Per Inch. Used to initialize default sizes such as widgets sized, style paddings. 00095 *(Not so important, you can adjust it to modify default sizes and spaces)*/ 00096 #define LV_DPI_DEF 130 /*[px/inch]*/ 00097 00098 /*======================= 00099 * FEATURE CONFIGURATION 00100 *=======================*/ 00101 00102 /*------------- 00103 * Drawing 00104 *-----------*/ 00105 00106 /*Enable complex draw engine. 00107 *Required to draw shadow, gradient, rounded corners, circles, arc, skew lines, image transformations or any masks*/ 00108 #define LV_DRAW_COMPLEX 1 00109 #if LV_DRAW_COMPLEX != 0 00110 00111 /*Allow buffering some shadow calculation. 00112 *LV_SHADOW_CACHE_SIZE is the max. shadow size to buffer, where shadow size is `shadow_width + radius` 00113 *Caching has LV_SHADOW_CACHE_SIZE^2 RAM cost*/ 00114 #define LV_SHADOW_CACHE_SIZE 0 00115 00116 /* Set number of maximally cached circle data. 00117 * The circumference of 1/4 circle are saved for anti-aliasing 00118 * radius * 4 bytes are used per circle (the most often used radiuses are saved) 00119 * 0: to disable caching */ 00120 #define LV_CIRCLE_CACHE_SIZE 4 00121 #endif /*LV_DRAW_COMPLEX*/ 00122 00123 /** 00124 * "Simple layers" are used when a widget has `style_opa < 255` to buffer the widget into a layer 00125 * and blend it as an image with the given opacity. 00126 * Note that `bg_opa`, `text_opa` etc don't require buffering into layer) 00127 * The widget can be buffered in smaller chunks to avoid using large buffers. 00128 * 00129 * - LV_LAYER_SIMPLE_BUF_SIZE: [bytes] the optimal target buffer size. LVGL will try to allocate it 00130 * - LV_LAYER_SIMPLE_FALLBACK_BUF_SIZE: [bytes] used if `LV_LAYER_SIMPLE_BUF_SIZE` couldn't be allocated. 00131 * 00132 * Both buffer sizes are in bytes. 00133 * "Transformed layers" (where transform_angle/zoom properties are used) use larger buffers 00134 * and can't be drawn in chunks. So these settings affects only widgets with opacity. 00135 */ 00136 #define LV_LAYER_SIMPLE_BUF_SIZE (24 * 1024) 00137 #define LV_LAYER_SIMPLE_FALLBACK_BUF_SIZE (3 * 1024) 00138 00139 /*Default image cache size. Image caching keeps the images opened. 00140 *If only the built-in image formats are used there is no real advantage of caching. (I.e. if no new image decoder is added) 00141 *With complex image decoders (e.g. PNG or JPG) caching can save the continuous open/decode of images. 00142 *However the opened images might consume additional RAM. 00143 *0: to disable caching*/ 00144 #define LV_IMG_CACHE_DEF_SIZE 0 00145 00146 /*Number of stops allowed per gradient. Increase this to allow more stops. 00147 *This adds (sizeof(lv_color_t) + 1) bytes per additional stop*/ 00148 #define LV_GRADIENT_MAX_STOPS 2 00149 00150 /*Default gradient buffer size. 00151 *When LVGL calculates the gradient "maps" it can save them into a cache to avoid calculating them again. 00152 *LV_GRAD_CACHE_DEF_SIZE sets the size of this cache in bytes. 00153 *If the cache is too small the map will be allocated only while it's required for the drawing. 00154 *0 mean no caching.*/ 00155 #define LV_GRAD_CACHE_DEF_SIZE 0 00156 00157 /*Allow dithering the gradients (to achieve visual smooth color gradients on limited color depth display) 00158 *LV_DITHER_GRADIENT implies allocating one or two more lines of the object's rendering surface 00159 *The increase in memory consumption is (32 bits * object width) plus 24 bits * object width if using error diffusion */ 00160 #define LV_DITHER_GRADIENT 0 00161 #if LV_DITHER_GRADIENT 00162 /*Add support for error diffusion dithering. 00163 *Error diffusion dithering gets a much better visual result, but implies more CPU consumption and memory when drawing. 00164 *The increase in memory consumption is (24 bits * object's width)*/ 00165 #define LV_DITHER_ERROR_DIFFUSION 0 00166 #endif 00167 00168 /*Maximum buffer size to allocate for rotation. 00169 *Only used if software rotation is enabled in the display driver.*/ 00170 #define LV_DISP_ROT_MAX_BUF (10*1024) 00171 00172 /*------------- 00173 * GPU 00174 *-----------*/ 00175 00176 /*Use Arm's 2D acceleration library Arm-2D */ 00177 #define LV_USE_GPU_ARM2D 0 00178 00179 /*Use STM32's DMA2D (aka Chrom Art) GPU*/ 00180 #define LV_USE_GPU_STM32_DMA2D 0 00181 #if LV_USE_GPU_STM32_DMA2D 00182 /*Must be defined to include path of CMSIS header of target processor 00183 e.g. "stm32f769xx.h" or "stm32f429xx.h"*/ 00184 #define LV_GPU_DMA2D_CMSIS_INCLUDE 00185 #endif 00186 00187 /*Use SWM341's DMA2D GPU*/ 00188 #define LV_USE_GPU_SWM341_DMA2D 0 00189 #if LV_USE_GPU_SWM341_DMA2D 00190 #define LV_GPU_SWM341_DMA2D_INCLUDE "SWM341.h" 00191 #endif 00192 00193 /*Use NXP's PXP GPU iMX RTxxx platforms*/ 00194 #define LV_USE_GPU_NXP_PXP 0 00195 #if LV_USE_GPU_NXP_PXP 00196 /*1: Add default bare metal and FreeRTOS interrupt handling routines for PXP (lv_gpu_nxp_pxp_osa.c) 00197 * and call lv_gpu_nxp_pxp_init() automatically during lv_init(). Note that symbol SDK_OS_FREE_RTOS 00198 * has to be defined in order to use FreeRTOS OSA, otherwise bare-metal implementation is selected. 00199 *0: lv_gpu_nxp_pxp_init() has to be called manually before lv_init() 00200 */ 00201 #define LV_USE_GPU_NXP_PXP_AUTO_INIT 0 00202 #endif 00203 00204 /*Use NXP's VG-Lite GPU iMX RTxxx platforms*/ 00205 #define LV_USE_GPU_NXP_VG_LITE 0 00206 00207 /*Use SDL renderer API*/ 00208 #define LV_USE_GPU_SDL 0 00209 #if LV_USE_GPU_SDL 00210 #define LV_GPU_SDL_INCLUDE_PATH <SDL2/SDL.h> 00211 /*Texture cache size, 8MB by default*/ 00212 #define LV_GPU_SDL_LRU_SIZE (1024 * 1024 * 8) 00213 /*Custom blend mode for mask drawing, disable if you need to link with older SDL2 lib*/ 00214 #define LV_GPU_SDL_CUSTOM_BLEND_MODE (SDL_VERSION_ATLEAST(2, 0, 6)) 00215 #endif 00216 00217 /*------------- 00218 * Logging 00219 *-----------*/ 00220 00221 /*Enable the log module*/ 00222 #define LV_USE_LOG 0 00223 #if LV_USE_LOG 00224 00225 /*How important log should be added: 00226 *LV_LOG_LEVEL_TRACE A lot of logs to give detailed information 00227 *LV_LOG_LEVEL_INFO Log important events 00228 *LV_LOG_LEVEL_WARN Log if something unwanted happened but didn't cause a problem 00229 *LV_LOG_LEVEL_ERROR Only critical issue, when the system may fail 00230 *LV_LOG_LEVEL_USER Only logs added by the user 00231 *LV_LOG_LEVEL_NONE Do not log anything*/ 00232 #define LV_LOG_LEVEL LV_LOG_LEVEL_WARN 00233 00234 /*1: Print the log with 'printf'; 00235 *0: User need to register a callback with `lv_log_register_print_cb()`*/ 00236 #define LV_LOG_PRINTF 0 00237 00238 /*Enable/disable LV_LOG_TRACE in modules that produces a huge number of logs*/ 00239 #define LV_LOG_TRACE_MEM 1 00240 #define LV_LOG_TRACE_TIMER 1 00241 #define LV_LOG_TRACE_INDEV 1 00242 #define LV_LOG_TRACE_DISP_REFR 1 00243 #define LV_LOG_TRACE_EVENT 1 00244 #define LV_LOG_TRACE_OBJ_CREATE 1 00245 #define LV_LOG_TRACE_LAYOUT 1 00246 #define LV_LOG_TRACE_ANIM 1 00247 00248 #endif /*LV_USE_LOG*/ 00249 00250 /*------------- 00251 * Asserts 00252 *-----------*/ 00253 00254 /*Enable asserts if an operation is failed or an invalid data is found. 00255 *If LV_USE_LOG is enabled an error message will be printed on failure*/ 00256 #define LV_USE_ASSERT_NULL 1 /*Check if the parameter is NULL. (Very fast, recommended)*/ 00257 #define LV_USE_ASSERT_MALLOC 1 /*Checks is the memory is successfully allocated or no. (Very fast, recommended)*/ 00258 #define LV_USE_ASSERT_STYLE 0 /*Check if the styles are properly initialized. (Very fast, recommended)*/ 00259 #define LV_USE_ASSERT_MEM_INTEGRITY 0 /*Check the integrity of `lv_mem` after critical operations. (Slow)*/ 00260 #define LV_USE_ASSERT_OBJ 0 /*Check the object's type and existence (e.g. not deleted). (Slow)*/ 00261 00262 /*Add a custom handler when assert happens e.g. to restart the MCU*/ 00263 #define LV_ASSERT_HANDLER_INCLUDE <stdint.h> 00264 #define LV_ASSERT_HANDLER while(1); /*Halt by default*/ 00265 00266 /*------------- 00267 * Others 00268 *-----------*/ 00269 00270 /*1: Show CPU usage and FPS count*/ 00271 #define LV_USE_PERF_MONITOR 0 00272 #if LV_USE_PERF_MONITOR 00273 #define LV_USE_PERF_MONITOR_POS LV_ALIGN_BOTTOM_RIGHT 00274 #endif 00275 00276 /*1: Show the used memory and the memory fragmentation 00277 * Requires LV_MEM_CUSTOM = 0*/ 00278 #define LV_USE_MEM_MONITOR 0 00279 #if LV_USE_MEM_MONITOR 00280 #define LV_USE_MEM_MONITOR_POS LV_ALIGN_BOTTOM_LEFT 00281 #endif 00282 00283 /*1: Draw random colored rectangles over the redrawn areas*/ 00284 #define LV_USE_REFR_DEBUG 0 00285 00286 /*Change the built in (v)snprintf functions*/ 00287 #define LV_SPRINTF_CUSTOM 0 00288 #if LV_SPRINTF_CUSTOM 00289 #define LV_SPRINTF_INCLUDE <stdio.h> 00290 #define lv_snprintf snprintf 00291 #define lv_vsnprintf vsnprintf 00292 #else /*LV_SPRINTF_CUSTOM*/ 00293 #define LV_SPRINTF_USE_FLOAT 0 00294 #endif /*LV_SPRINTF_CUSTOM*/ 00295 00296 #define LV_USE_USER_DATA 1 00297 00298 /*Garbage Collector settings 00299 *Used if lvgl is bound to higher level language and the memory is managed by that language*/ 00300 #define LV_ENABLE_GC 0 00301 #if LV_ENABLE_GC != 0 00302 #define LV_GC_INCLUDE "gc.h" /*Include Garbage Collector related things*/ 00303 #endif /*LV_ENABLE_GC*/ 00304 00305 /*===================== 00306 * COMPILER SETTINGS 00307 *====================*/ 00308 00309 /*For big endian systems set to 1*/ 00310 #define LV_BIG_ENDIAN_SYSTEM 0 00311 00312 /*Define a custom attribute to `lv_tick_inc` function*/ 00313 #define LV_ATTRIBUTE_TICK_INC 00314 00315 /*Define a custom attribute to `lv_timer_handler` function*/ 00316 #define LV_ATTRIBUTE_TIMER_HANDLER 00317 00318 /*Define a custom attribute to `lv_disp_flush_ready` function*/ 00319 #define LV_ATTRIBUTE_FLUSH_READY 00320 00321 /*Required alignment size for buffers*/ 00322 #define LV_ATTRIBUTE_MEM_ALIGN_SIZE 1 00323 00324 /*Will be added where memories needs to be aligned (with -Os data might not be aligned to boundary by default). 00325 * E.g. __attribute__((aligned(4)))*/ 00326 #define LV_ATTRIBUTE_MEM_ALIGN 00327 00328 /*Attribute to mark large constant arrays for example font's bitmaps*/ 00329 #define LV_ATTRIBUTE_LARGE_CONST 00330 00331 /*Compiler prefix for a big array declaration in RAM*/ 00332 #define LV_ATTRIBUTE_LARGE_RAM_ARRAY 00333 00334 /*Place performance critical functions into a faster memory (e.g RAM)*/ 00335 #define LV_ATTRIBUTE_FAST_MEM 00336 00337 /*Prefix variables that are used in GPU accelerated operations, often these need to be placed in RAM sections that are DMA accessible*/ 00338 #define LV_ATTRIBUTE_DMA 00339 00340 /*Export integer constant to binding. This macro is used with constants in the form of LV_<CONST> that 00341 *should also appear on LVGL binding API such as Micropython.*/ 00342 #define LV_EXPORT_CONST_INT(int_value) struct _silence_gcc_warning /*The default value just prevents GCC warning*/ 00343 00344 /*Extend the default -32k..32k coordinate range to -4M..4M by using int32_t for coordinates instead of int16_t*/ 00345 #define LV_USE_LARGE_COORD 0 00346 00347 /*================== 00348 * FONT USAGE 00349 *===================*/ 00350 00351 /*Montserrat fonts with ASCII range and some symbols using bpp = 4 00352 *https://fonts.google.com/specimen/Montserrat*/ 00353 #define LV_FONT_MONTSERRAT_8 0 00354 #define LV_FONT_MONTSERRAT_10 0 00355 #define LV_FONT_MONTSERRAT_12 0 00356 #define LV_FONT_MONTSERRAT_14 1 00357 #define LV_FONT_MONTSERRAT_16 0 00358 #define LV_FONT_MONTSERRAT_18 0 00359 #define LV_FONT_MONTSERRAT_20 0 00360 #define LV_FONT_MONTSERRAT_22 0 00361 #define LV_FONT_MONTSERRAT_24 0 00362 #define LV_FONT_MONTSERRAT_26 0 00363 #define LV_FONT_MONTSERRAT_28 0 00364 #define LV_FONT_MONTSERRAT_30 0 00365 #define LV_FONT_MONTSERRAT_32 0 00366 #define LV_FONT_MONTSERRAT_34 0 00367 #define LV_FONT_MONTSERRAT_36 0 00368 #define LV_FONT_MONTSERRAT_38 0 00369 #define LV_FONT_MONTSERRAT_40 0 00370 #define LV_FONT_MONTSERRAT_42 0 00371 #define LV_FONT_MONTSERRAT_44 0 00372 #define LV_FONT_MONTSERRAT_46 0 00373 #define LV_FONT_MONTSERRAT_48 0 00374 00375 /*Demonstrate special features*/ 00376 #define LV_FONT_MONTSERRAT_12_SUBPX 0 00377 #define LV_FONT_MONTSERRAT_28_COMPRESSED 0 /*bpp = 3*/ 00378 #define LV_FONT_DEJAVU_16_PERSIAN_HEBREW 0 /*Hebrew, Arabic, Persian letters and all their forms*/ 00379 #define LV_FONT_SIMSUN_16_CJK 0 /*1000 most common CJK radicals*/ 00380 00381 /*Pixel perfect monospace fonts*/ 00382 #define LV_FONT_UNSCII_8 0 00383 #define LV_FONT_UNSCII_16 0 00384 00385 /*Optionally declare custom fonts here. 00386 *You can use these fonts as default font too and they will be available globally. 00387 *E.g. #define LV_FONT_CUSTOM_DECLARE LV_FONT_DECLARE(my_font_1) LV_FONT_DECLARE(my_font_2)*/ 00388 #define LV_FONT_CUSTOM_DECLARE 00389 00390 /*Always set a default font*/ 00391 #define LV_FONT_DEFAULT &lv_font_montserrat_14 00392 00393 /*Enable handling large font and/or fonts with a lot of characters. 00394 *The limit depends on the font size, font face and bpp. 00395 *Compiler error will be triggered if a font needs it.*/ 00396 #define LV_FONT_FMT_TXT_LARGE 0 00397 00398 /*Enables/disables support for compressed fonts.*/ 00399 #define LV_USE_FONT_COMPRESSED 0 00400 00401 /*Enable subpixel rendering*/ 00402 #define LV_USE_FONT_SUBPX 0 00403 #if LV_USE_FONT_SUBPX 00404 /*Set the pixel order of the display. Physical order of RGB channels. Doesn't matter with "normal" fonts.*/ 00405 #define LV_FONT_SUBPX_BGR 0 /*0: RGB; 1:BGR order*/ 00406 #endif 00407 00408 /*Enable drawing placeholders when glyph dsc is not found*/ 00409 #define LV_USE_FONT_PLACEHOLDER 1 00410 00411 /*================= 00412 * TEXT SETTINGS 00413 *=================*/ 00414 00415 /** 00416 * Select a character encoding for strings. 00417 * Your IDE or editor should have the same character encoding 00418 * - LV_TXT_ENC_UTF8 00419 * - LV_TXT_ENC_ASCII 00420 */ 00421 #define LV_TXT_ENC LV_TXT_ENC_UTF8 00422 00423 /*Can break (wrap) texts on these chars*/ 00424 #define LV_TXT_BREAK_CHARS " ,.;:-_" 00425 00426 /*If a word is at least this long, will break wherever "prettiest" 00427 *To disable, set to a value <= 0*/ 00428 #define LV_TXT_LINE_BREAK_LONG_LEN 0 00429 00430 /*Minimum number of characters in a long word to put on a line before a break. 00431 *Depends on LV_TXT_LINE_BREAK_LONG_LEN.*/ 00432 #define LV_TXT_LINE_BREAK_LONG_PRE_MIN_LEN 3 00433 00434 /*Minimum number of characters in a long word to put on a line after a break. 00435 *Depends on LV_TXT_LINE_BREAK_LONG_LEN.*/ 00436 #define LV_TXT_LINE_BREAK_LONG_POST_MIN_LEN 3 00437 00438 /*The control character to use for signalling text recoloring.*/ 00439 #define LV_TXT_COLOR_CMD "#" 00440 00441 /*Support bidirectional texts. Allows mixing Left-to-Right and Right-to-Left texts. 00442 *The direction will be processed according to the Unicode Bidirectional Algorithm: 00443 *https://www.w3.org/International/articles/inline-bidi-markup/uba-basics*/ 00444 #define LV_USE_BIDI 0 00445 #if LV_USE_BIDI 00446 /*Set the default direction. Supported values: 00447 *`LV_BASE_DIR_LTR` Left-to-Right 00448 *`LV_BASE_DIR_RTL` Right-to-Left 00449 *`LV_BASE_DIR_AUTO` detect texts base direction*/ 00450 #define LV_BIDI_BASE_DIR_DEF LV_BASE_DIR_AUTO 00451 #endif 00452 00453 /*Enable Arabic/Persian processing 00454 *In these languages characters should be replaced with an other form based on their position in the text*/ 00455 #define LV_USE_ARABIC_PERSIAN_CHARS 0 00456 00457 /*================== 00458 * WIDGET USAGE 00459 *================*/ 00460 00461 /*Documentation of the widgets: https://docs.lvgl.io/latest/en/html/widgets/index.html*/ 00462 00463 #define LV_USE_ARC 1 00464 00465 #define LV_USE_BAR 1 00466 00467 #define LV_USE_BTN 1 00468 00469 #define LV_USE_BTNMATRIX 1 00470 00471 #define LV_USE_CANVAS 1 00472 00473 #define LV_USE_CHECKBOX 1 00474 00475 #define LV_USE_DROPDOWN 1 /*Requires: lv_label*/ 00476 00477 #define LV_USE_IMG 1 /*Requires: lv_label*/ 00478 00479 #define LV_USE_LABEL 1 00480 #if LV_USE_LABEL 00481 #define LV_LABEL_TEXT_SELECTION 1 /*Enable selecting text of the label*/ 00482 #define LV_LABEL_LONG_TXT_HINT 1 /*Store some extra info in labels to speed up drawing of very long texts*/ 00483 #endif 00484 00485 #define LV_USE_LINE 1 00486 00487 #define LV_USE_ROLLER 1 /*Requires: lv_label*/ 00488 #if LV_USE_ROLLER 00489 #define LV_ROLLER_INF_PAGES 7 /*Number of extra "pages" when the roller is infinite*/ 00490 #endif 00491 00492 #define LV_USE_SLIDER 1 /*Requires: lv_bar*/ 00493 00494 #define LV_USE_SWITCH 1 00495 00496 #define LV_USE_TEXTAREA 1 /*Requires: lv_label*/ 00497 #if LV_USE_TEXTAREA != 0 00498 #define LV_TEXTAREA_DEF_PWD_SHOW_TIME 1500 /*ms*/ 00499 #endif 00500 00501 #define LV_USE_TABLE 1 00502 00503 /*================== 00504 * EXTRA COMPONENTS 00505 *==================*/ 00506 00507 /*----------- 00508 * Widgets 00509 *----------*/ 00510 #define LV_USE_ANIMIMG 1 00511 00512 #define LV_USE_CALENDAR 1 00513 #if LV_USE_CALENDAR 00514 #define LV_CALENDAR_WEEK_STARTS_MONDAY 0 00515 #if LV_CALENDAR_WEEK_STARTS_MONDAY 00516 #define LV_CALENDAR_DEFAULT_DAY_NAMES {"Mo", "Tu", "We", "Th", "Fr", "Sa", "Su"} 00517 #else 00518 #define LV_CALENDAR_DEFAULT_DAY_NAMES {"Su", "Mo", "Tu", "We", "Th", "Fr", "Sa"} 00519 #endif 00520 00521 #define LV_CALENDAR_DEFAULT_MONTH_NAMES {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"} 00522 #define LV_USE_CALENDAR_HEADER_ARROW 1 00523 #define LV_USE_CALENDAR_HEADER_DROPDOWN 1 00524 #endif /*LV_USE_CALENDAR*/ 00525 00526 #define LV_USE_CHART 1 00527 00528 #define LV_USE_COLORWHEEL 1 00529 00530 #define LV_USE_IMGBTN 1 00531 00532 #define LV_USE_KEYBOARD 1 00533 00534 #define LV_USE_LED 1 00535 00536 #define LV_USE_LIST 1 00537 00538 #define LV_USE_MENU 1 00539 00540 #define LV_USE_METER 1 00541 00542 #define LV_USE_MSGBOX 1 00543 00544 #define LV_USE_SPAN 1 00545 #if LV_USE_SPAN 00546 /*A line text can contain maximum num of span descriptor */ 00547 #define LV_SPAN_SNIPPET_STACK_SIZE 64 00548 #endif 00549 00550 #define LV_USE_SPINBOX 1 00551 00552 #define LV_USE_SPINNER 1 00553 00554 #define LV_USE_TABVIEW 1 00555 00556 #define LV_USE_TILEVIEW 1 00557 00558 #define LV_USE_WIN 1 00559 00560 /*----------- 00561 * Themes 00562 *----------*/ 00563 00564 /*A simple, impressive and very complete theme*/ 00565 #define LV_USE_THEME_DEFAULT 1 00566 #if LV_USE_THEME_DEFAULT 00567 00568 /*0: Light mode; 1: Dark mode*/ 00569 #define LV_THEME_DEFAULT_DARK 0 00570 00571 /*1: Enable grow on press*/ 00572 #define LV_THEME_DEFAULT_GROW 1 00573 00574 /*Default transition time in [ms]*/ 00575 #define LV_THEME_DEFAULT_TRANSITION_TIME 80 00576 #endif /*LV_USE_THEME_DEFAULT*/ 00577 00578 /*A very simple theme that is a good starting point for a custom theme*/ 00579 #define LV_USE_THEME_BASIC 1 00580 00581 /*A theme designed for monochrome displays*/ 00582 #define LV_USE_THEME_MONO 1 00583 00584 /*----------- 00585 * Layouts 00586 *----------*/ 00587 00588 /*A layout similar to Flexbox in CSS.*/ 00589 #define LV_USE_FLEX 1 00590 00591 /*A layout similar to Grid in CSS.*/ 00592 #define LV_USE_GRID 1 00593 00594 /*--------------------- 00595 * 3rd party libraries 00596 *--------------------*/ 00597 00598 /*File system interfaces for common APIs */ 00599 00600 /*API for fopen, fread, etc*/ 00601 #define LV_USE_FS_STDIO 0 00602 #if LV_USE_FS_STDIO 00603 #define LV_FS_STDIO_LETTER '\0' /*Set an upper cased letter on which the drive will accessible (e.g. 'A')*/ 00604 #define LV_FS_STDIO_PATH "" /*Set the working directory. File/directory paths will be appended to it.*/ 00605 #define LV_FS_STDIO_CACHE_SIZE 0 /*>0 to cache this number of bytes in lv_fs_read()*/ 00606 #endif 00607 00608 /*API for open, read, etc*/ 00609 #define LV_USE_FS_POSIX 0 00610 #if LV_USE_FS_POSIX 00611 #define LV_FS_POSIX_LETTER '\0' /*Set an upper cased letter on which the drive will accessible (e.g. 'A')*/ 00612 #define LV_FS_POSIX_PATH "" /*Set the working directory. File/directory paths will be appended to it.*/ 00613 #define LV_FS_POSIX_CACHE_SIZE 0 /*>0 to cache this number of bytes in lv_fs_read()*/ 00614 #endif 00615 00616 /*API for CreateFile, ReadFile, etc*/ 00617 #define LV_USE_FS_WIN32 0 00618 #if LV_USE_FS_WIN32 00619 #define LV_FS_WIN32_LETTER '\0' /*Set an upper cased letter on which the drive will accessible (e.g. 'A')*/ 00620 #define LV_FS_WIN32_PATH "" /*Set the working directory. File/directory paths will be appended to it.*/ 00621 #define LV_FS_WIN32_CACHE_SIZE 0 /*>0 to cache this number of bytes in lv_fs_read()*/ 00622 #endif 00623 00624 /*API for FATFS (needs to be added separately). Uses f_open, f_read, etc*/ 00625 #define LV_USE_FS_FATFS 0 00626 #if LV_USE_FS_FATFS 00627 #define LV_FS_FATFS_LETTER '\0' /*Set an upper cased letter on which the drive will accessible (e.g. 'A')*/ 00628 #define LV_FS_FATFS_CACHE_SIZE 0 /*>0 to cache this number of bytes in lv_fs_read()*/ 00629 #endif 00630 00631 /*PNG decoder library*/ 00632 #define LV_USE_PNG 0 00633 00634 /*BMP decoder library*/ 00635 #define LV_USE_BMP 0 00636 00637 /* JPG + split JPG decoder library. 00638 * Split JPG is a custom format optimized for embedded systems. */ 00639 #define LV_USE_SJPG 0 00640 00641 /*GIF decoder library*/ 00642 #define LV_USE_GIF 0 00643 00644 /*QR code library*/ 00645 #define LV_USE_QRCODE 0 00646 00647 /*FreeType library*/ 00648 #define LV_USE_FREETYPE 0 00649 #if LV_USE_FREETYPE 00650 /*Memory used by FreeType to cache characters [bytes] (-1: no caching)*/ 00651 #define LV_FREETYPE_CACHE_SIZE (16 * 1024) 00652 #if LV_FREETYPE_CACHE_SIZE >= 0 00653 /* 1: bitmap cache use the sbit cache, 0:bitmap cache use the image cache. */ 00654 /* sbit cache:it is much more memory efficient for small bitmaps(font size < 256) */ 00655 /* if font size >= 256, must be configured as image cache */ 00656 #define LV_FREETYPE_SBIT_CACHE 0 00657 /* Maximum number of opened FT_Face/FT_Size objects managed by this cache instance. */ 00658 /* (0:use system defaults) */ 00659 #define LV_FREETYPE_CACHE_FT_FACES 0 00660 #define LV_FREETYPE_CACHE_FT_SIZES 0 00661 #endif 00662 #endif 00663 00664 /*Rlottie library*/ 00665 #define LV_USE_RLOTTIE 0 00666 00667 /*FFmpeg library for image decoding and playing videos 00668 *Supports all major image formats so do not enable other image decoder with it*/ 00669 #define LV_USE_FFMPEG 0 00670 #if LV_USE_FFMPEG 00671 /*Dump input information to stderr*/ 00672 #define LV_FFMPEG_DUMP_FORMAT 0 00673 #endif 00674 00675 /*----------- 00676 * Others 00677 *----------*/ 00678 00679 /*1: Enable API to take snapshot for object*/ 00680 #define LV_USE_SNAPSHOT 0 00681 00682 /*1: Enable Monkey test*/ 00683 #define LV_USE_MONKEY 0 00684 00685 /*1: Enable grid navigation*/ 00686 #define LV_USE_GRIDNAV 0 00687 00688 /*1: Enable lv_obj fragment*/ 00689 #define LV_USE_FRAGMENT 0 00690 00691 /*1: Support using images as font in label or span widgets */ 00692 #define LV_USE_IMGFONT 0 00693 00694 /*1: Enable a published subscriber based messaging system */ 00695 #define LV_USE_MSG 0 00696 00697 /*1: Enable Pinyin input method*/ 00698 /*Requires: lv_keyboard*/ 00699 #define LV_USE_IME_PINYIN 0 00700 #if LV_USE_IME_PINYIN 00701 /*1: Use default thesaurus*/ 00702 /*If you do not use the default thesaurus, be sure to use `lv_ime_pinyin` after setting the thesauruss*/ 00703 #define LV_IME_PINYIN_USE_DEFAULT_DICT 1 00704 /*Set the maximum number of candidate panels that can be displayed*/ 00705 /*This needs to be adjusted according to the size of the screen*/ 00706 #define LV_IME_PINYIN_CAND_TEXT_NUM 6 00707 00708 /*Use 9 key input(k9)*/ 00709 #define LV_IME_PINYIN_USE_K9_MODE 1 00710 #if LV_IME_PINYIN_USE_K9_MODE == 1 00711 #define LV_IME_PINYIN_K9_CAND_TEXT_NUM 3 00712 #endif // LV_IME_PINYIN_USE_K9_MODE 00713 #endif 00714 00715 /*================== 00716 * EXAMPLES 00717 *==================*/ 00718 00719 /*Enable the examples to be built with the library*/ 00720 #define LV_BUILD_EXAMPLES 1 00721 00722 /*=================== 00723 * DEMO USAGE 00724 ====================*/ 00725 00726 /*Show some widget. It might be required to increase `LV_MEM_SIZE` */ 00727 #define LV_USE_DEMO_WIDGETS 1 00728 #if LV_USE_DEMO_WIDGETS 00729 #define LV_DEMO_WIDGETS_SLIDESHOW 0 00730 #endif 00731 00732 /*Demonstrate the usage of encoder and keyboard*/ 00733 #define LV_USE_DEMO_KEYPAD_AND_ENCODER 0 00734 00735 /*Benchmark your system*/ 00736 #define LV_USE_DEMO_BENCHMARK 0 00737 #if LV_USE_DEMO_BENCHMARK 00738 /*Use RGB565A8 images with 16 bit color depth instead of ARGB8565*/ 00739 #define LV_DEMO_BENCHMARK_RGB565A8 0 00740 #endif 00741 00742 /*Stress test for LVGL*/ 00743 #define LV_USE_DEMO_STRESS 0 00744 00745 /*Music player demo*/ 00746 #define LV_USE_DEMO_MUSIC 0 00747 #if LV_USE_DEMO_MUSIC 00748 #define LV_DEMO_MUSIC_SQUARE 0 00749 #define LV_DEMO_MUSIC_LANDSCAPE 0 00750 #define LV_DEMO_MUSIC_ROUND 0 00751 #define LV_DEMO_MUSIC_LARGE 0 00752 #define LV_DEMO_MUSIC_AUTO_PLAY 0 00753 #endif 00754 00755 /*--END OF LV_CONF_H--*/ 00756 00757 #endif /*LV_CONF_H*/ 00758 00759 #endif /*End of "Content enable"*/
Generated on Sat Feb 4 2023 21:01:57 by
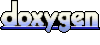