MODULO TEMPORIZADOR
Dependents: IngresoHORA Tarea_5
Fork of RTC-DS1307 by
Rtc_Ds1307.h
00001 /* Rtc_Ds1307.h */ 00002 /* 00003 Copyright (c) 2013 Henry Leinen (henry[dot]leinen [at] online [dot] de) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 #ifndef __RTC_DS1307_H__ 00024 #define __RTC_DS1307_H__ 00025 00026 00027 00028 /** Class Rtc_Ds1307 implements the real time clock module DS1307 00029 * 00030 * You can read the clock and set a new time and date. 00031 * It is also possible to start and stop the clock. 00032 * Rtc_Ds1307 allows you to display the time in a 12h or 24h format 00033 */ 00034 class Rtc_Ds1307 00035 { 00036 public: 00037 /** Structure which is used to exchange the time and date 00038 */ 00039 typedef struct { 00040 int sec ; /*!< seconds [0..59] */ 00041 int min ; /*!< minutes {0..59] */ 00042 int hour ; /*!< hours [0..23] */ 00043 int wday ; /*!< weekday [1..7, where 1 = sunday, 2 = monday, ... */ 00044 int date ; /*!< day of month [0..31] */ 00045 int mon ; /*!< month of year [1..12] */ 00046 int year ; /*!< year [2000..2255] */ 00047 } Time_rtc; 00048 00049 /** RateSelect specifies the valid frequency values for the square wave output 00050 */ 00051 typedef enum { 00052 RS1Hz = 0, 00053 RS4kHz = 1, 00054 RS8kHz = 2, 00055 RS32kHz = 3 00056 } SqwRateSelect_t; 00057 00058 protected: 00059 I2C* m_rtc; 00060 00061 static const char *m_weekDays[]; 00062 00063 public: 00064 /** public constructor which creates the real time clock object 00065 * 00066 * @param sda : specifies the pin for the SDA communication line. 00067 * 00068 * @param scl : the pin for the serial clock 00069 * 00070 */ 00071 Rtc_Ds1307(PinName sda, PinName scl); 00072 00073 ~Rtc_Ds1307(); 00074 00075 /** Read the current time from RTC chip 00076 * 00077 * @param time : reference to a struct tm which will be filled with the time from rtc 00078 * 00079 * @returns true if successful, otherwise an acknowledge error occured 00080 */ 00081 virtual bool getTime(Time_rtc& time); 00082 00083 /** Write the given time onto the RTC chip 00084 * 00085 * @param time : refereence to a struct which contains valid date and time information 00086 * 00087 * @param start : contains true if the clock shall start (or keep on running). 00088 * 00089 * @param thm : 12-hour-mode if set to true, otherwise 24-hour-mode will be set. 00090 * 00091 * @returns true if successful, otherwise an acknowledge error occured 00092 */ 00093 virtual bool setTime(Time_rtc& time, bool start, bool thm); 00094 00095 /** Start the clock. Please note that the seconds register need to be read and 00096 * written in order to start or stop the clock. This can lead to an error 00097 * in the time value. The recommended way of starting and stoping the clock is 00098 * to write the actual date and time and set the start bit accordingly. 00099 * 00100 * @returns true if the clock was started, false if a communication error occured 00101 */ 00102 bool startClock(); 00103 00104 /** Stop the clock. Please note that the seconds register need to be read and 00105 * written in order to start or stop the clock. This can lead to an error 00106 * in the time value. The recommended way of starting and stoping the clock is 00107 * to write the actual date and time and set the start bit accordingly. 00108 * 00109 * @returns true if the clock was stopped, false if a communication error occured 00110 */ 00111 bool stopClock(); 00112 00113 /** Service function to convert a weekday into a string representation 00114 * 00115 * @param wday : day of week to convert (starting with sunday = 1, monday = 2, ..., saturday = 7 00116 * 00117 * @returns the corresponding string representation 00118 */ 00119 const char* weekdayToString( int wday ) { 00120 return m_weekDays[wday%7]; 00121 } 00122 00123 /** Enable Square Wave output. The function enables or disables the square wave output 00124 * of the module and sets the desired frequency. 00125 * 00126 * @param ena : if set to true, the square wave output is enabled. 00127 * 00128 * @param rs : rate select, can be either one of the four values defined by type /c RateSelect_t 00129 * 00130 * @return true if the operation was successful or false otherwise 00131 */ 00132 bool setSquareWaveOutput(bool ena, SqwRateSelect_t rs); 00133 00134 private: 00135 bool read(int address, char* buffer, int len); 00136 bool write(int address, char* buffer, int len); 00137 00138 static int bcdToDecimal(int bcd) { 00139 return ((bcd&0xF0)>>4)*10 + (bcd&0x0F); 00140 } 00141 00142 static int decimalToBcd(int dec) { 00143 return (dec%10) + ((dec/10)<<4); 00144 } 00145 }; 00146 00147 00148 00149 typedef void (*RtcCallback_t) (void); 00150 00151 00152 class RtcCls : public Rtc_Ds1307 00153 { 00154 protected: 00155 InterruptIn m_sqw; 00156 bool m_bUseSqw; 00157 time_t m_time; // Only used in case SQW is used 00158 00159 bool m_bAlarmEnabled; 00160 RtcCallback_t m_alarmfunc; 00161 time_t m_alarmTime; 00162 00163 public: 00164 RtcCls(PinName sda, PinName scl, PinName sqw, bool bUseSqw); 00165 00166 protected: 00167 void _callback(void); 00168 00169 public: 00170 time_t getTime(); 00171 virtual bool getTime(Time_rtc& time) { return Rtc_Ds1307::getTime(time); } 00172 void setTime(time_t time); 00173 virtual bool setTime(Time_rtc& time, bool start, bool thm) { return Rtc_Ds1307::setTime(time, start, thm); } 00174 public: 00175 void setAlarm(int nSeconds, RtcCallback_t alarmfunc) { 00176 m_alarmfunc = alarmfunc; 00177 m_alarmTime = m_time + nSeconds; 00178 m_bAlarmEnabled = (alarmfunc == NULL) ? false : true; 00179 } 00180 }; 00181 00182 #endif // __RTC_DS1307_H__
Generated on Sun Jul 17 2022 01:37:25 by
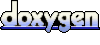