
Done
Dependencies: HTU21D Hexi_KW40Z Hexi_OLED_SSD1351 MAX30101
Fork of Hexiwear by
main.cpp
00001 #include "mbed.h" 00002 #include "Hexi_OLED_SSD1351.h" 00003 #include "Ticker.h" 00004 #include "Hexi_KW40Z.h" 00005 #include "HTU21D.h" 00006 #include "string.h" 00007 #include "MAX30101.h" 00008 00009 #define LED_ON 0 00010 #define LED_OFF 1 00011 00012 void StartHaptic(void); 00013 void StopHaptic(void const *n); 00014 void ButtonUp(void); 00015 void ButtonDown(void); 00016 void ButtonRight(void); 00017 void ButtonLeft(void); 00018 void ButtonSlide(void); 00019 void attime(void); 00020 00021 DigitalOut redLed(LED1); 00022 DigitalOut greenLed(LED2); 00023 DigitalOut blueLed(LED3); 00024 DigitalOut haptic(PTB9); 00025 00026 MAX30101 heart(PTB1, PTB0); 00027 00028 /* Instantiate the SSD1351 OLED Driver */ 00029 SSD1351 oled(PTB22,PTB21,PTC13,PTB20,PTE6, PTD15); /* (MOSI,SCLK,POWER,CS,RST,DC) */ 00030 00031 /* Instantiate the Hexi KW40Z Driver (UART TX, UART RX) */ 00032 KW40Z kw40z_device(PTE24, PTE25); 00033 00034 /* Define timer for haptic feedback */ 00035 RtosTimer hapticTimer(StopHaptic, osTimerOnce); 00036 00037 /* Instantiate the HTU21D Sensor */ 00038 HTU21D temphumid(PTB1,PTB0); 00039 DigitalOut powerEN (PTB12); // Power Enable HTU21D Sensor 00040 00041 int sample_ftemp; //values for the humidity/temp sensors 00042 int sample_ctemp; 00043 int sample_ktemp; 00044 int sample_humid; 00045 int sample_HR; 00046 00047 char text[20]; 00048 int flag=0; 00049 int counter=0; 00050 Ticker timer; 00051 00052 int main() 00053 { 00054 powerEN =0; 00055 00056 /* Register callbacks to application functions */ 00057 kw40z_device.attach_buttonUp(&ButtonUp); 00058 kw40z_device.attach_buttonDown(&ButtonDown); 00059 kw40z_device.attach_buttonLeft(&ButtonLeft); 00060 kw40z_device.attach_buttonRight(&ButtonRight); 00061 kw40z_device.attach_buttonSlide(&ButtonSlide); 00062 00063 heart.enable(); 00064 00065 /* Get OLED Class Default Text Properties */ 00066 oled_text_properties_t textProperties = {0}; 00067 oled.GetTextProperties(&textProperties); 00068 00069 /* Turn on the backlight of the OLED Display */ 00070 oled.DimScreenON(); 00071 00072 /* Fills the screen with solid black */ 00073 oled.FillScreen(COLOR_BLACK); 00074 00075 /* Display Text at (x=7,y=0) */ 00076 strcpy((char *) text,"Welcome"); 00077 oled.Label((uint8_t *)text,7,0); 00078 00079 /* Display text at (x=5,y=40) */ 00080 strcpy(text,"Initializing.."); 00081 oled.Label((uint8_t *)text,5,40); 00082 /* 00083 Thread::wait(3000); 00084 00085 oled.FillScreen(COLOR_BLACK); 00086 00087 strcpy((char *) text,"Functions List:"); 00088 oled.Label((uint8_t *)text,7,0); 00089 00090 textProperties.fontColor = COLOR_BLUE; 00091 oled.SetTextProperties(&textProperties); 00092 00093 00094 strcpy((char *) text,"Left:Bike Menu"); 00095 oled.Label((uint8_t *)text,0,15); 00096 00097 00098 strcpy((char *) text,"Right:Biometrics"); 00099 oled.Label((uint8_t *)text,0,35); 00100 */ 00101 Thread::wait(3000); 00102 00103 00104 oled.FillScreen(COLOR_BLACK); 00105 00106 textProperties.fontColor = COLOR_RED; 00107 oled.SetTextProperties(&textProperties); 00108 00109 00110 strcpy((char *) text,"Temp:"); 00111 oled.Label((uint8_t *)text,5,15); 00112 00113 //display Humidity-linked to while loop 00114 strcpy((char *) text,"Humidity:"); 00115 oled.Label((uint8_t *)text,5,30); 00116 00117 //display timer-linked to while loop for continuous readings 00118 strcpy((char *) text,"Timer:"); 00119 oled.Label((uint8_t *)text,5,45); 00120 00121 //display Humidity-linked to while loop 00122 // strcpy((char *) text,"HR:"); 00123 // oled.Label((uint8_t *)text,5,60); 00124 00125 00126 00127 00128 while(true) 00129 { 00130 sample_ftemp = temphumid.sample_ftemp(); 00131 sample_humid = temphumid.sample_humid(); 00132 sample_ftemp-=10; 00133 // sample_HR = heart.getRevisionID(); 00134 00135 textProperties.fontColor = COLOR_WHITE; 00136 textProperties.alignParam = OLED_TEXT_ALIGN_RIGHT; 00137 oled.SetTextProperties(&textProperties); 00138 00139 /* Format the value */ 00140 sprintf(text,"%d F",sample_ftemp); 00141 /* Display time reading in 35px by 15px textbox at(x=55, y=40) */ 00142 oled.TextBox((uint8_t *)text,55,15,35,15); //Increase textbox for more digits 00143 00144 /* Format the value */ 00145 sprintf(text,"%d %%",sample_humid); 00146 /* Display time reading in 35px by 15px textbox at(x=55, y=40) */ 00147 oled.TextBox((uint8_t *)text,55,30,35,15); //Increase textbox for more digits 00148 00149 sprintf(text,"%d s",counter); 00150 // Display time reading in 35px by 15px textbox at(x=55, y=40) 00151 oled.TextBox((uint8_t *)text,55,45,35,15); //Increase textbox for more digits 00152 00153 // sprintf(text,"%d bpm",sample_HR); 00154 // Display time reading in 35px by 15px textbox at(x=55, y=40) 00155 //oled.TextBox((uint8_t *)text,55,60,35,15); //Increase textbox for more digits 00156 //Thread::wait(300); 00157 } 00158 } 00159 void attime(void) 00160 { 00161 counter++; 00162 } 00163 void ButtonUp(void) 00164 { 00165 StartHaptic(); 00166 Thread::wait(50); 00167 StartHaptic(); 00168 00169 redLed = LED_ON; 00170 greenLed = LED_OFF; 00171 blueLed = LED_OFF; 00172 00173 00174 if(flag==0) 00175 { 00176 timer.attach(&attime,1); 00177 flag=1; 00178 } 00179 else 00180 { 00181 timer.detach(); 00182 counter=0; 00183 flag=0; 00184 } 00185 } 00186 00187 void ButtonDown(void) 00188 { 00189 StartHaptic(); 00190 Thread::wait(50); 00191 StartHaptic(); 00192 00193 redLed = LED_OFF; 00194 greenLed = LED_ON; 00195 blueLed = LED_OFF; 00196 00197 if(flag==0) 00198 { 00199 timer.attach(&attime,1); 00200 flag=1; 00201 } 00202 else 00203 { 00204 timer.detach(); 00205 counter=0; 00206 flag=0; 00207 } 00208 } 00209 00210 void ButtonRight(void) 00211 { 00212 StartHaptic(); 00213 00214 redLed = LED_OFF; 00215 greenLed = LED_OFF; 00216 00217 blueLed = LED_ON; 00218 } 00219 00220 void ButtonLeft(void) 00221 { 00222 StartHaptic(); 00223 00224 redLed = LED_ON; 00225 greenLed = LED_ON; 00226 blueLed = LED_OFF; 00227 } 00228 00229 void ButtonSlide(void) 00230 { 00231 StartHaptic(); 00232 00233 redLed = LED_ON; 00234 greenLed = LED_ON; 00235 blueLed = LED_ON; 00236 } 00237 00238 void StartHaptic(void) 00239 { 00240 hapticTimer.start(50); 00241 haptic = 1; 00242 } 00243 00244 void StopHaptic(void const *n) { 00245 haptic = 0; 00246 hapticTimer.stop(); 00247 }
Generated on Tue Jul 12 2022 21:44:21 by
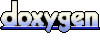